Zod File Upload validation
I'm currently making a file upload form
is there any way to validate a file in zod?
I tried this https://github.com/colinhacks/zod/issues/387#issuecomment-1191390673
but it didn't seem to work
No matter what file i upload it just says image is required
https://rentry.co/ndimv
A gist of my code
// Imports go here
const MAX_FILE_SIZE = 500000
const ACCEPTED_IMAGE_TYPES = [
"image/jpeg",
"image/jpg",
"image/png",
"image/webp",
]
const formSchema = z.object({
course_id: z.string().min(1, { message: "Course name is required" }),
year: z.string().m...
GitHub
Validating file input · Issue #387 · colinhacks/zod
Coming from Yup. I'm trying to validate a required file input with no success: file: z.any().refine(val => val.length > 0, "File is required") Any tips?
43 Replies
that error message is defined in the formSchema
isn't that enough?
When I was doing this I had to do it in a slightly different way
The api for attaching files was a file list actually so I had to refine the inputs
I’ll share what works for me in a second when I’m at my pc
If I forget plz @ me
guess you forgot
could you please share that?
Just using an Input with type file to prompt the user
works well 😄
Am I missing something?
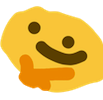
chrome
The comment you linked in OP is basically the same way I do it aside from using z.custom instead of z.any. Did you try this one? https://github.com/colinhacks/zod/issues/387#issuecomment-1535668926
GitHub
Validating file input · Issue #387 · colinhacks/zod
Coming from Yup. I'm trying to validate a required file input with no success: file: z.any().refine(val => val.length > 0, "File is required") Any tips?
I don't know
I just took the project from the shelf (basically I did touched it for a month or so)
maybe its older
lemme check
zod - v3.21.4
react - 18.2.0
next - 13.4.4
This wasnt fun when I was implementing it. Weird to see it happen to you to but my solution not working
. The solution I shared above didn't work for me so I was hoping it would for you
All the "thumbs-up" reactions on the commetn and it isn't actually working 😭
versions look fine
What is your handle upload doing?
Thats probably it
The form state doesn't have a value set so thats why its erroring even tho locally the input might actually have something.
check the state using react dev tools to see if the input has anything
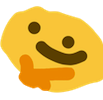
const handleUpload = (e: any) => {
setFiles(e.target.files)
}
thats it
I'm assuming you want to let the use upload a file and submit. I do the same thing but I leave the upload in the handle submit portion and the form state holds my file
it just sets the state
Well thats why
Your formstate doesn't have it
Thats why its erroring
console.log(form)
and you'll be able to see it
you might have to dig thru the properties
or just set up a
Then try uploading and see what happens
iirc it logged the file path
i did this long ago