❔ Recursive Multiplier
Hi, when I try to write this example by modifying the method return type to int, I don't get the same output as the void return type. Shouldn't this give the same result?
Also, why can't I use Console.WriteLine(RepeatMultiply(1,2,4000)); to output the result in the void method example when calling the method from Main?
Sorry if there is something obvious that I don't get. I'm very new to programming.
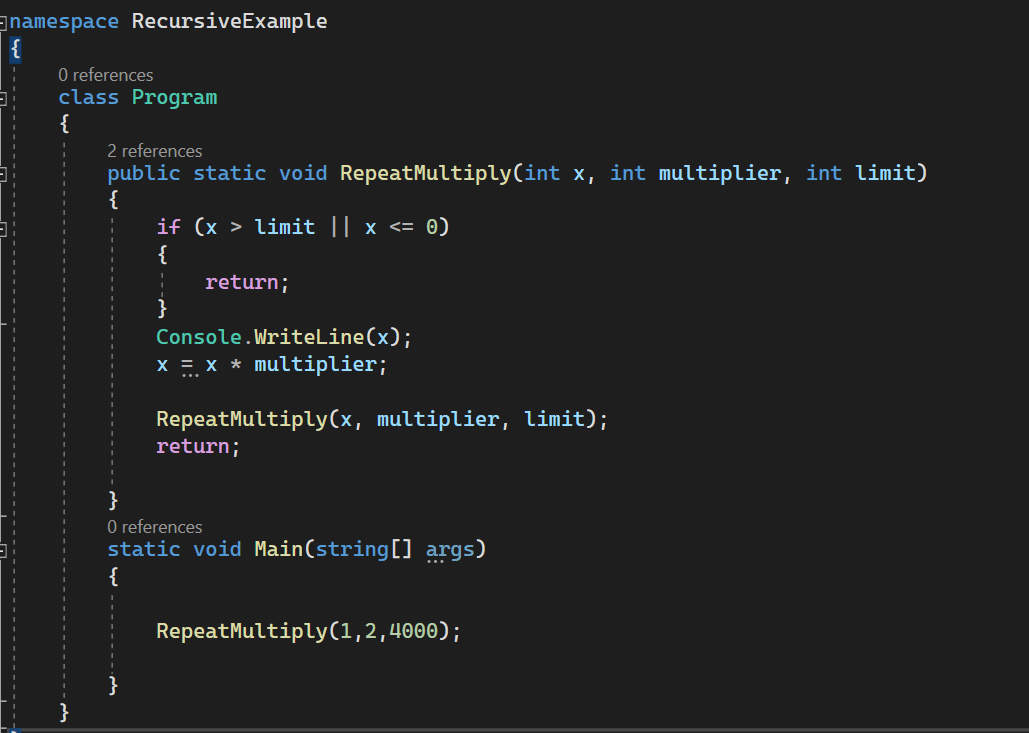
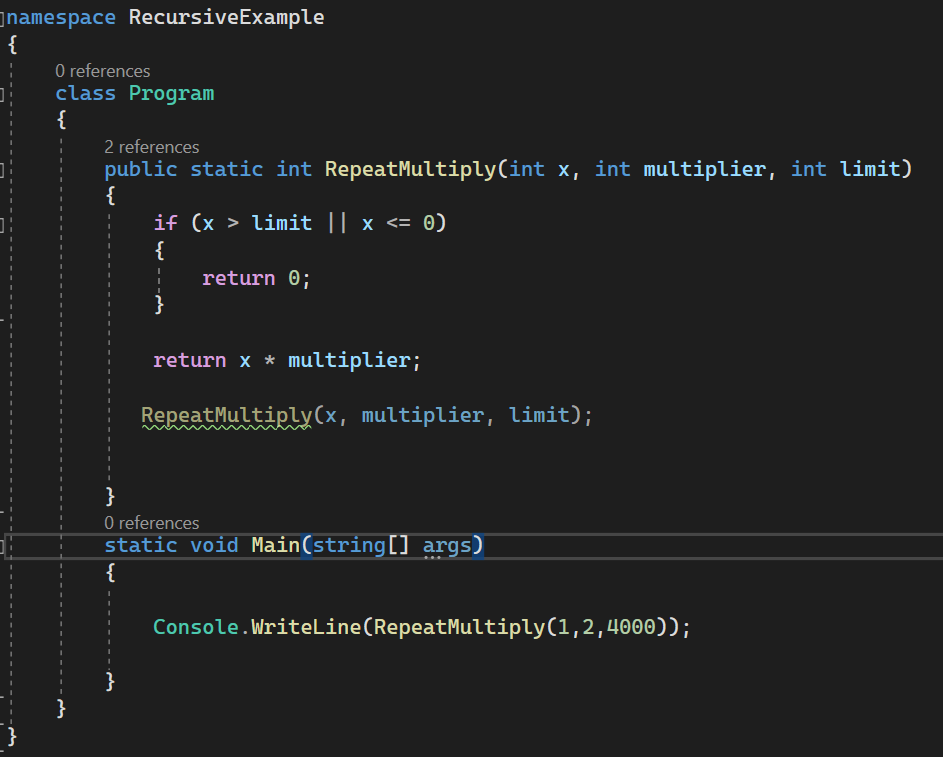
42 Replies
Do you see that green squiggle?
Hover your mouse over it
And read what it says
Then look at your code again
Yes, CS0162: unreachable code. Does that mean code exits before reaching this part?
It's saying that it is impossible for that line of code to ever execute.
Do you see why?
Is it because of the return code above?
Yes.
Jump statements - break, continue, return, and goto
C# jump statements (break, continue, return, and goto) unconditionally transfer control from the current location to a different statement.
return exits the function
A recursive function usually returns the result of calling itself.
Ohh now I understand. Is it possible to get the same result without using the void method?
Sure
Did you figure it out?
I tried without returning anything, but now it says the method needs a return value.
Just tried to call RepeatMultiply(x,multiplier, limit) inside the method
How do you return the result of a function calling itself?
I am returning the repeatmultiply(x, multiplier, liit)
Show the code
Use $code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/$codegif
Do you know what a base case is in a recursive function?
kind off
isn't my if statement the base case here?
The base case is what causes the recursive function to terminate and return the final value that was calculated. And yes, that's your if statement.
But is your base case returning the final value?
No, it's returning 0.
So once you get to the base case the function just always return 0.
You might want to make two if statements - one to check for less than or equal to zero, a second to handle the base case of reaching the limit.
Maybe that will make it more clear.
Ask in #help-0 , this channel is for @Kobra 's question 🙂
I tried breaking down the if statement into two ifs but can't understand what to do if the limit is reached.
I am basically doing the same thing before breaking the if statements
Don't return 0 in the base case.
return limit?
Sorry, I feel so dumb right now
Recursion is a little tricky at first, don't worry about it.
But ultimately the point of this function is to do what? Compute a result (in this case, the value of doing a series of multiplications.)
In the base case, return the result.
limit
is not the result.
It never changes.so return x?
🎉
But it still prints out 4096
What's your final code and what did you expect it to print out?
I want the last value before reaching 4000 to be printed out and then terminate itself
exactly like the example with void method
Ok, so think about how you might accomplish that.
One approach would be something like: check if multiplying will satisfy your base case and return the accumulated result (not including the latest multiplication) if it does. Only assign the result of the mulitplication to x and recurse if it does not satisfy the base case.
You might find a temporary variable useful.
Thanks for the tip
@mtreit Thank you for your help and patience. I am so glad for people like you, who have the patience to learn newbies like me. I really appreciate it
Your temp variable isn't necessary there.
I was suggesting using a temp variable so you don't do the multiplication twice.
(Which is a very small performance optimization)
Also, I assume hard-coding 4000 was just for debugging, and you should really be checking against
limit
Since right now you ignore limit
`
Yes, that was the idea.
Now that I think about it, I would probably use some better variable names and write it something like this:
Small optimization in there is: don't multiply if you don't have to (like if the value is 0)
(rename
x
to currentValue
)
But that's just polishing. The code as you wrote it works.You are right, looks much more tidier and understandable.
why not use a loop
I think they are learning recursion.
oh
i 

If I used a loop, would that be iterative? Is there any advantage over the other approach?
Yes, iterative. Recursion is actually usually worse if there is a straightforward iterative solution.
It's slower and dangerous in languages like c# that don't have tail call optimizations, because if you recurse too deeply you can stack overflow
Thank you @mtreit
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.