❔ ✅ ListView Annoyances.
I am trying to use await/async to pull all files from all folders in a chosen path. The issue I have is when trying to add them to a listview, and it keeps erroring out with the message:
:'Cannot add or insert the item 'bakedfile00/AI/AIGOSGameObject.jsfb' in more than one place. You must first remove it from its current location or clone it. (Parameter 'item')'
I am close to solving it, been trying various things but no luck. Can anyone help me? Thank you.
My code is at this URL due to Discord message limit.
https://pastebin.com/Eab8dP9F
Thank you! ❤️
Pastebin
CODE-BEHIND private async void PollDirectories_Click(object ...
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
89 Replies
I don't do UI programming so not sure about list views, but is this just a matter of calling Clear() on the list view outside of the for loop?
If I keep clear in there, my listview just clears, populats over and over
if i remove the clear, it never does anything :/
Outside the loop, not inside the loop
I moved it above the loop
now i get this again:
System.ArgumentException: 'Cannot add or insert the item 'bakedfile00/AI/AIGOSGameObject.jsfb' in more than one place. You must first remove it from its current location or clone it. (Parameter 'item')'
So you're adding the same string multiple times?
No, I'm going through every file in a folder(s), putting em in a list, making a hash
Well, the error says that item is already in the listview
items
keeps containing the items of the previous iterationugh
what am i missing? 😂
You'd thing the ListViewItem new would make a new item for it
Make a HashSet<string> to track which items you've added and if you check the HashSet and the string is already in there, figure out why you are getting duplicates.
Well, yeah, you are adding stuff into items and you add items again and again
I had it working fine before I got mad and ripped it out for a DataGrid which made things worse XD
well, theoretically u would need to clear
items
after adding that range, but then u have a race conditionhmmm
Oh
u could simply forget about a global
items
outside the for loop and create one for reach chunkClear items at the start of each for loop iteration (the outer loop)
also do I really need both?
then its just fire and forget and throw the reference away after that
I invoked due to async/await
simply move
List<ListViewItem> items = new List<ListViewItem>();
inside the for loop and its fixed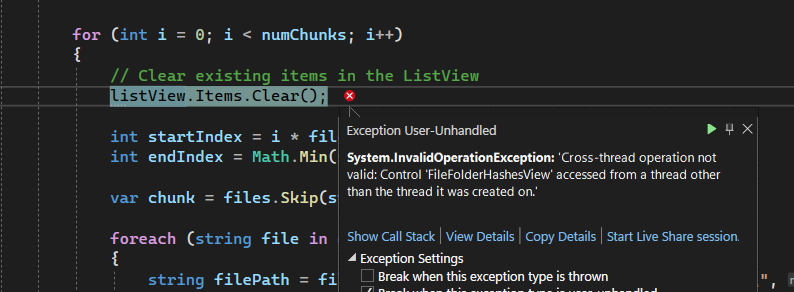
ok
sec
You're not doing async await anywhere in that code
I'd actually prefer a virtual listview. It's a better to work with imo
oh, yeah that u will have to do via
Invoke
as well
ok but do i need item.SubItems.Add(hashValue);
items.Add(item);?
this is so damn sloppy, a infant could do better 😦
What's the hash value for anyway?
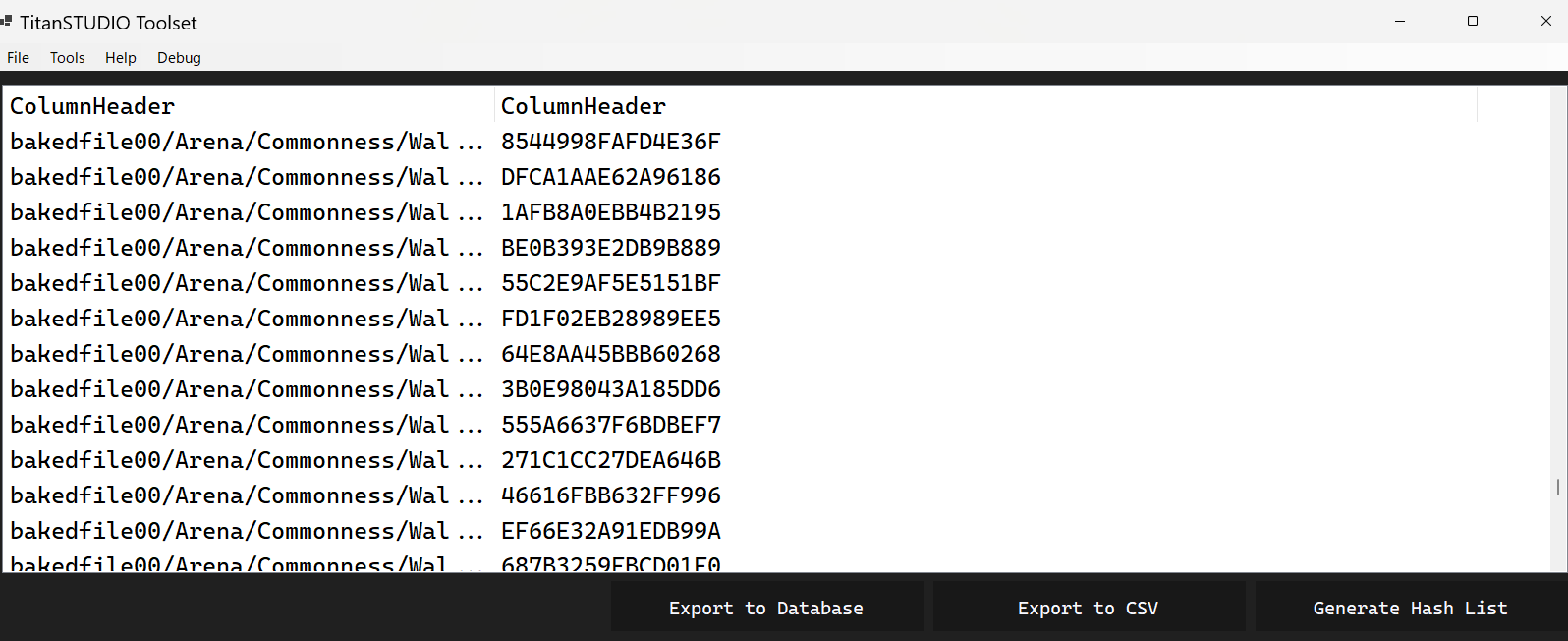
FIXED
😮
Final code:
I like your extremely inconsistent use of
var
😄listView.Items.Clear();
needs to be executed on the UI thread as wellBecause the game uses FNV1A to encode file names/folders as GUID's pretty much
im lazy i guess
// Update the ListView on the main thread
listView.Invoke(new Action(() =>
{
// Clear existing items in the ListView
listView.Items.Clear();
// Add the items to the ListView
listView.Items.AddRange(items.ToArray());
}));
now I have TWO items.clear
and half my list in the listview is missing 😂
dont do it before adding each chunk, do it before adding all chunks (outside the for loop), in its own invoke
the outer loop or inner?
basically the first appearence of
listView.Items.Clear();
before the for loop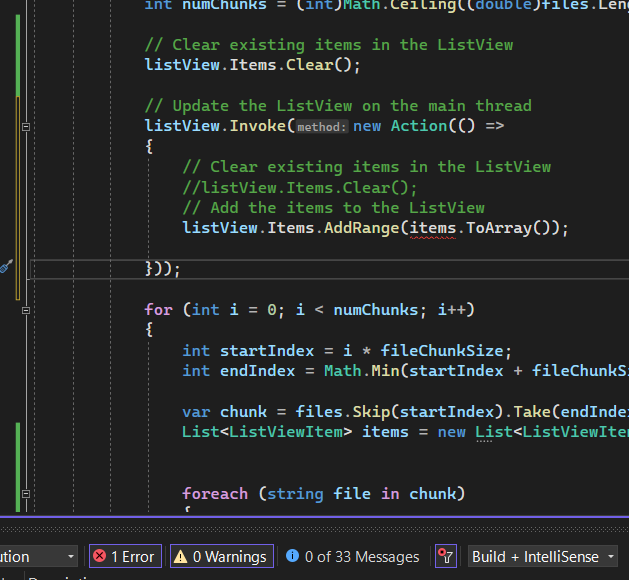
now it's just broken, because items wasnt defined yet
are u kidding me ... i said that
Clear()
call has to be in the invokeok
not the chunk stuff
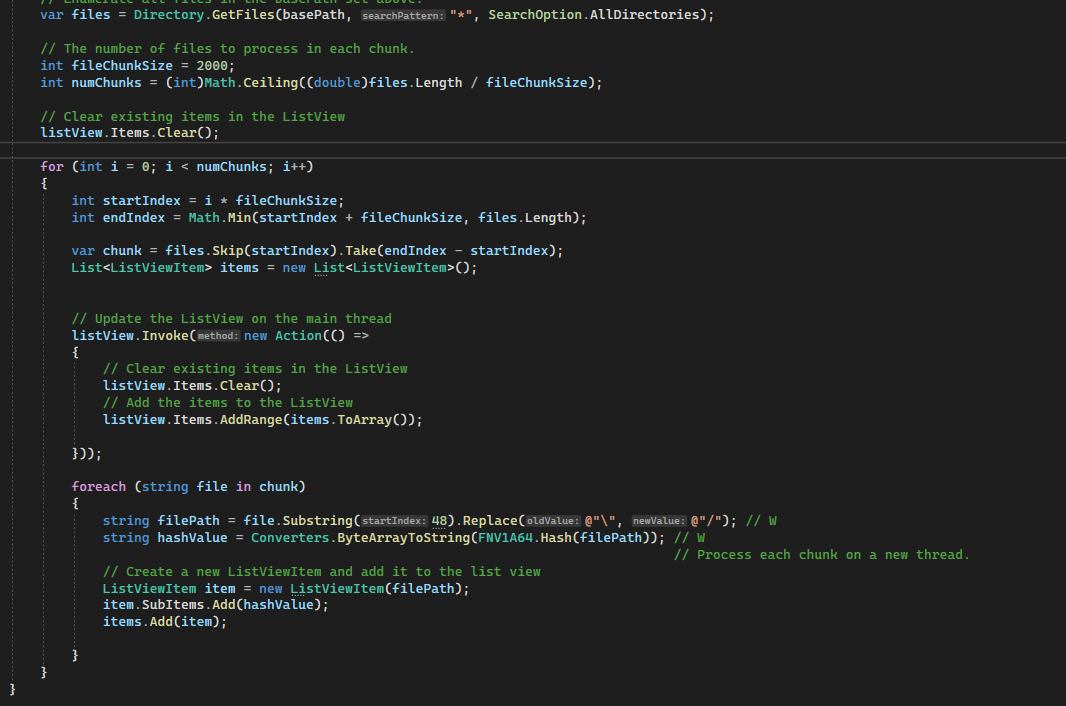
closer?
my head hurts and having asd isnt helping me :"|
now is it good? :x
I like how Discord chokes on lol
no its not, because u r still clearing the whole list inside the invoke which is inside the for loop as well
and then u r adding an empty list in that invoke
and after that u fill the list
ffs
Im lost
Oh the invoke thing...
Well it works, now, I feel dumb 😐
I was close
can probably be reduced to
listView.Invoke(() => listView.Items.AddRange(items));
listView.Invoke(new Action(() => listView.Items.AddRange(items));
cant convert to listviewitem
as im using a list
did u call
Add
instead of AddRange
?listView.Invoke(() => listView.Items.AddRange(items.ToArray())); works
has to be an array as I have a List<T>
what is the parameter type? i expected it to be
IEnumerable<ListViewItem>
List<ListViewItem> items = new List<ListViewItem>();
aaah, yeah has to be
ToArray()
, it doesnt have an IEnumerable<ListViewItem>
overload (https://learn.microsoft.com/en-us/dotnet/api/system.windows.forms.listview.listviewitemcollection.addrange?view=windowsdesktop-7.0)
expected it to be more similar to a List<T>
from the api surfaceListView.ListViewItemCollection.AddRange Method (System.Windows.Forms)
Adds an array of items to the collection.
yeah 🙂
btw u can simplify the chunking a lot
Enumerable.Chunk(IEnumerable, Int32) Method (System.Linq)
Splits the elements of a sequence into chunks of size at most size.
I only am chunking because its over 10,000 files
oh ok
I will have to play with that new idea when im focused more
Can I add a .sort to the invoke arrowfunction?
listView.Invoke(() => listView.Items.AddRange(items.ToArray()) => listView.Sort());
nm got it
basically the whole method could be just:
listView.Invoke(() => { listView.Items.AddRange(items.ToArray()); listView.Sort(); });
much better
oh..
do I even need to chunk? was from a tutorial
I assume yes due to having 10K+ files to go through lol
well, yeah if u have a lot of entries added at once, it would freeze the whole UI when adding all at once
i think even 2000 might be too many
fair, even with threads
hmmm, 1000 then? was on 1000 before, i increased it
this is sadly from case to case differently
i'll try 1000 then 500 if needed
usually u would even add some delay inbetween:
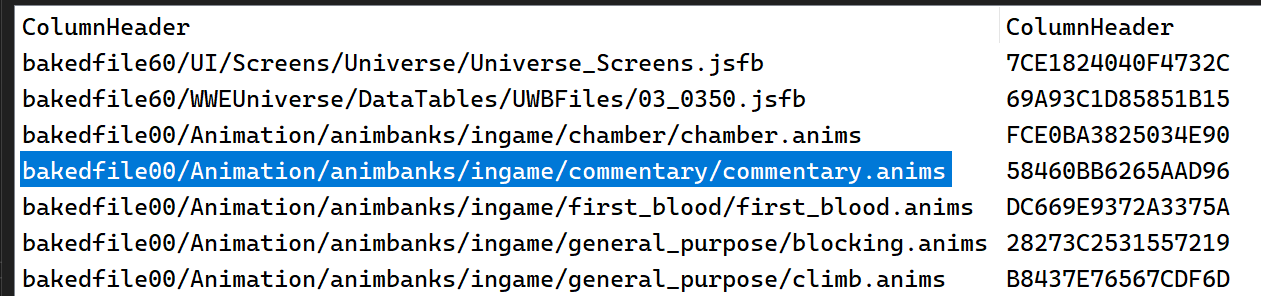
nice "sort"
how does 00 go after 60
oh nm its going by the entire line
i mgiht need to rewrite this portion 😄
that makes more sense
btw, do the sort on the linq part, not sorting after adding each chunk
basically before the
.Select(...)
u could add a .OrderBy(file => file)
to sort by the path itselfthanks
glad i could help o7
complete w your help
im sure in WPF it will be different but this works for now
in wpf its quite similar, how u execute the the update on the ui thread should be the key difference
yeah true, DataContext, Source, all different
but the "skeleton" is the same for almost any ui system
I'm not ready to move my app there yet
I do notice one thing, the code doesn't add the directory too, only files.
well, i guess u r talking about the MVVM pattern here actually, then u wouldnt add it to the list component like this, but to ur view model's observable collection like this
yeah mvvm
then its basically just a different list u add to ;p
as long as i can add it o the same listview 😛
i will try adding directories, thanks again 🙂
⤴️
also is listview underkill for this or should i use datagridview?
i have no clue about winforms besides how to do a quick google xD
fair enough, it works, thats what matters, its a free app thing 🙂
thanks again o7
if ur problem is solved now, please dont forget to $close the thread
Use the
/close
command to mark a forum thread as answeredwill it be archived?
in case i need to re read it
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.