✅ What's the easiest type of information storage?
I'm new to C# and when I was starting out with Python, the first type of data storage I learned was working with json files and I'm curious to know what is the easiest file storage to learn with C#? After learning how to create a math game and that involved database storage, I can't seem to replicate what I've done and get the database to work when I work and create a different project, so I'm wanting to learn an easier data storage thing. Any ideas? Thanks.
320 Replies
Honestly, json is a pretty common and easy way to do data storage in C# too. Here's some Microsoft docs on how to use System.Text.Json https://learn.microsoft.com/en-us/dotnet/standard/serialization/system-text-json/how-to?pivots=dotnet-8-0
How to serialize and deserialize JSON using C# - .NET
Learn how to use the System.Text.Json namespace to serialize to and deserialize from JSON in .NET. Includes sample code.
ty 🙂
In general, the storage type is not language specific, and you can use the very same with Python or C#, or even Java.
JSON is a valid and performant choice.
What it comes to JSON and C# you can either use a popular third-party library called Newtonsoft JSON, or the native System.Text.Json
However, if you e.g. wish to write a JSON schema and validate incoming JSON against it, it is only possible with Newtonsoft.
Then you can always use XML for storing data. Or, you can store your data in databass
I definitely appreciate that. The big problem that I'm coming into with C# in general is balancing between the scripting files
.cs
and the window pages .xaml
. I get the window pages pretty easy as it's really similar (in my opinion) to web design on the front end. I've picked that up rather simply, but when it comes to moving around the .cs
files and making them match up to the window files, and making the schemas in the .cs
files like
like this one, and knowing when and where and how to use it, it just becomes a nightmare for me. I guess that's the step that Python took out because I don't need files like this in Python unless I'm wanting to be super nit-picky in my code. Otherwise, I just write my stuff right into my logic and continue on about my day. Sometimes I'll write helper modules like
if I'm going to be writing stuff to my json file frequently and then i'll just add in a trigger word with an if statement like
but in C# everything is on a much lower language level aspect and it's honestly a lot annoying to me. Same with Java. I built a number guessing game in java using swing and dropped the project and forgot about java because of how nit-picky the langauge was and how much of a nightmare it was to use a json fileDo yourself and everyone else a favor and use
System.Text.Json
instead of Newtonsoft as a beginner.
It's literally two lines of code to serialize an object and write it to a JSON file on diskKudos for starting out with WPF and not WinForms
and I really don't doubt that, however, I started learning code with someone sitting next to me going "no bitch that's wrong" and "there you go chick" and if I just couldn't figure it out, I'd slide the keyboard over and now I'm on my own so like trying to use docs and such is like really difficult even though I understand how they work. I figured out how to read the docs with developing discord bots in Python, but using that knowledge and trying to do other languages just kills it lol
don't honestly know that acronym....I just open visual studio, click new project, select .net maui project and go from there....I can do console apps like no ones business though if they're strictly in the console
Hopefully I don't get roasted for this, but ChatGPT is a great pair programmer. Only you also have to be conscious and know when it generated nonsense
Oh maui, that's different, but also a valid choice
but that's soon retired as I'm running out of space on my computer and therefore am going to spend some time getting vscode prepped for c# programming
just over the last few years, being able to determine bad code (for me) is as simple as putting it into a test file and seeing if it 1) works and 2) does what I need it to do
Vscode is a subpar experience for C# compared to a full-blown IDE
I really recommend getting a larger hard drive. Vs code is a nightmare for beginners.
you're not wrong there, I'm just running out of space on this poor laptop lol I've been setup to use
VSCode for
- Web Design
- Python projects
- Java Projects
and Visual Studio for
- C# projects
As for C# and ease of use. Yes, you do tend to do a bit more than python. However, it is so much less than in Java. That language makes you write a lot of unnecessary code. While C# is sweet with a ton of syntax sugar that hides away the complicated stuff and makes programming easier
not that I disagree, but for me I learned how to use VSCode faster and more easily than I did visual studio
Yep, bigger tool, steeper learning curve. But look on the bright side, you are stepping outside of your comfort zone
Be a masochist like me, and go a step further by installing the VIM extension
oh absolutely. Java is just full of boiler plate code that honestly is useless. Why should I have to instantiate objects like they want you to do when in Python it would be like instantiating everything in your init method and then having to call
self.something
everywhere you need it. It's globalizing variables that don't need to be globalized
haha I'm definitely a sadist. So this will be a new trick haha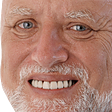
https://github.com/mekasu0124/MeksDiary
https://github.com/mekasu0124/MeksPeriodicTableGame
https://github.com/mekasu0124/MeksWordle
https://github.com/mekasu0124/MeksBattleShip
these are the python projects that I've started, retired, or am currently working on that I'd love to learn how to do in C#. C# is better for game design and desktop applications, but it's just so......complicated between c# and xaml
Stack Overflow
How to read JSON file into string specifying values and using Syste...
I have a .Net Core 3.1 console application (not an ASP.NET Core) with SQL Server connection, where connection string is stored in appsettings.json file
{"ConnectionStrings": { "DefaultConnection":"
You'll get used to it
I moved from python to C#, and I'm never touch that snake again.
ok so let's say on my console app, it prompts the user for their username
how would I store that username into a json file like this
in Python I would just do
Make a class/record with a
Username
string property
Lets call this UserInfo
Then, create a new class/record with a UserInfo UserInfo
property. Now create an instance of these recordshere's what I Have
Then simply feed that outer instance to
JsonSerializer.Serialize
you 100% frenched me
Je ne suis pas francais
yo hablo espanol
See, unlike python we don't do untyped json very well, or at all
Indeed you create a class to represent your data. Don't view this as an overcomplication, but as an improved maintenability of code. You represent your data with a class, and have the class in one place - more readablility
We instead create classes that model our data
whew.....yea the basics taught me nothing
Well yeah... They are literally the basics
I'm on mobile ATM so can't really write up an example :p
it's all good. I just want to get good at some type of data storage. That's my major lacking component to being able to do advanced console apps
if write a full example of your desired JSON, you can paste it into ChatGPT and tell it to write a C# model representing it
https://github.com/mekasu0124/MeksMathGame this is my math game. Did I write this alone? No. I followed https://www.thecsharpacademy.com/ dudes tutorial (which went extremely fast) and learned it his way. The way I initially wrote it was just omg so ugly and full of spaghetti. I then followed his tutorial to turn it into a GUI application https://github.com/mekasu0124/MeksMathGameGUI and I've tried to use this GUI program and replicate how he did database storage using SQLite and build my diary app (which is now built in python) because I couldn't get the database to work correctly.
so write it in python, copy/paste, and it'll transpose it to c#?
Not what I meant directly, but sure that can also work.ig
The goal is to have a desired json - how you want your data to look like
And copy that into ChatGPT
can't use it unless I create an account, and I've created so many accounts on so many different things in my programming time, that I'm honestly burnt out on doing that
And what about bing?
Or paste the JSON here I'll get it for you
ok but where's the dictionary indexing? How does it know to put the username with the data["username"] key,value pair?
It looks at your object structure
like the search engine bing?
Bing chat
ok you've bought my interest here
We dobt treat json as a dictionary
never heard of it
It uses something wonderful called reflection
ok this is new. there's other ways to treat it? Python had me thinking it was strictly just dictionaries
No it represents an object structure
It's just that in python, all objects are dictionaries
It would really make more sense if python was called librarian and not python
ok. I'm redownloading visual studio and once it launches, I'll create a new console app and give this json thing a try. I doubt I'm going to understand it right off the bat, but I'll give it a shot.
And get yourself an external drive.
Store data in a class. The class you give to the serializer is the outer object, represented by the outmost brackets
Cheaper than a new laptop
I have a 1TB. I just get tired of plugging it in and unplugging it. I don't have a desk and do most of my programming outside as for some ungodly reason I get better internet connection outside than I do in my room on the other side of the wall from the router.
ok so what would be an example of writing this as a class?
This.depends on what you want your JSON to look like
written words help understand from context pov, but code displays help even more
if that makes sense
If you don't care, then here's an example
if I don't care?
Wait a few Hours, I'm typing this on a phone
lmao not a few hours xD
Phone coding isn't great :p
oh trust me. it's even worse on iphone
This should then produce the following JSON
oh getter and setters. never understood those either
don't use those in python
They are different in python
🫤
Username and Score in that example is what we call "Properties"
oh like
Kinda, but since we are not loosely typed, you need to declare them ahead of time
We can't just make an ad-hoc object like python
like a global username = ""
You can avoid writing those properties if you use some syntax sugar of C# and write a record:
We declare types
Definitely not like
global
:pWith properties you are in control of what is going on with your data. You know when it is accessed and when it is changed
You can, of course use public fields and lose than control. But that is not considered good C#.
And with the auto-property syntax it's not much shorter anyways
like that? *edited
Yup
Almost
Yes wonderful
Ah missing semicolon
You need to use the
new
keyword
When creating new objects
A d you want to save the object reference
So `UserData data = new UserData(...)
And you need a constructor to assign the property 🙂
(or use a record)Or you can skip the constructor.
UserData data = new UserData { Username=username};
Records are very nice, but can be hard to understand as a beginner. They are immutable for one
You can also replace the UserData on the left with var
Yup
Assuming a constructor exists in the class
But remember, the data variable will exist in the scope of your method. Once the method has exited, you data is thrown.away.
what if I did
You can.
Except it's not a string
It's a UserData
so string would be var
Or UserData, yes
I'm a big fan of var, but since you are new it might be a good idea to be explicit about your types
This is bad code, and it is not a string.
But don't do this. Read the line separately
ok so what's the import for using the json library?
System.Text.Json
ok so visual studio finished downloading, I created a new console app and here's where I'm at. The
UserData(username);
is throwing an error due to the class not having a constructor with that parameter so what did I miss?Making the constructor.
You could also change to the initializer syntax Denis showed
I put public in front of the get/set
Write your code like a plain old boring story. Everything is clearly stated. Don't do any plot twists like surprising the reader with a Console readline directly in the object initialisation.
That works
Except it should give you warning about the naming
Nice. Only with records you write the constructor parameters with capital letters
But that's just a warning 🙂
Ha beat you to it
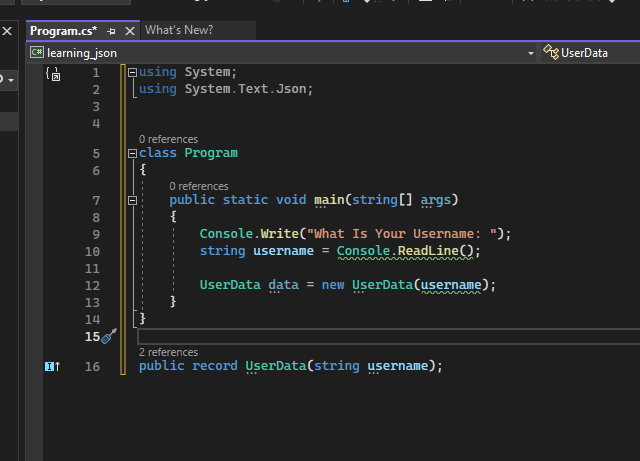
Yus
just says that username may not be null
on line 12
Ignore that for now
It's a warming only
ok
Readline can in theory return null, but it's a very special case. Ignore
ok ignoring
when I hover over UserName on line 16 it says to declare types in namespace
Correct
You can force ignore it in the code, but I'll keep that feature a secret for.now, so that you don't use it in places you shouldn't
Add a namespace declaration to the top of your file
so all of this should be wrapped in a namespace
Wrapped and wrapped...
Just
namespace YourNamespace;
Under your usingsYou have probably made a .NET Framework console project, because the new .NET console doesn't have main
ok did that
But it doesn't matter
Or am I wrong and you pasted the main in there?
Oh yeah, for the future make sure you are using .net 7
I created a new project, and in the next screen I named it and then selected C# for the language and Console for the project type
Because why otherwise would you have the namespace missing...
Hmm
Prob copypasted
Yep
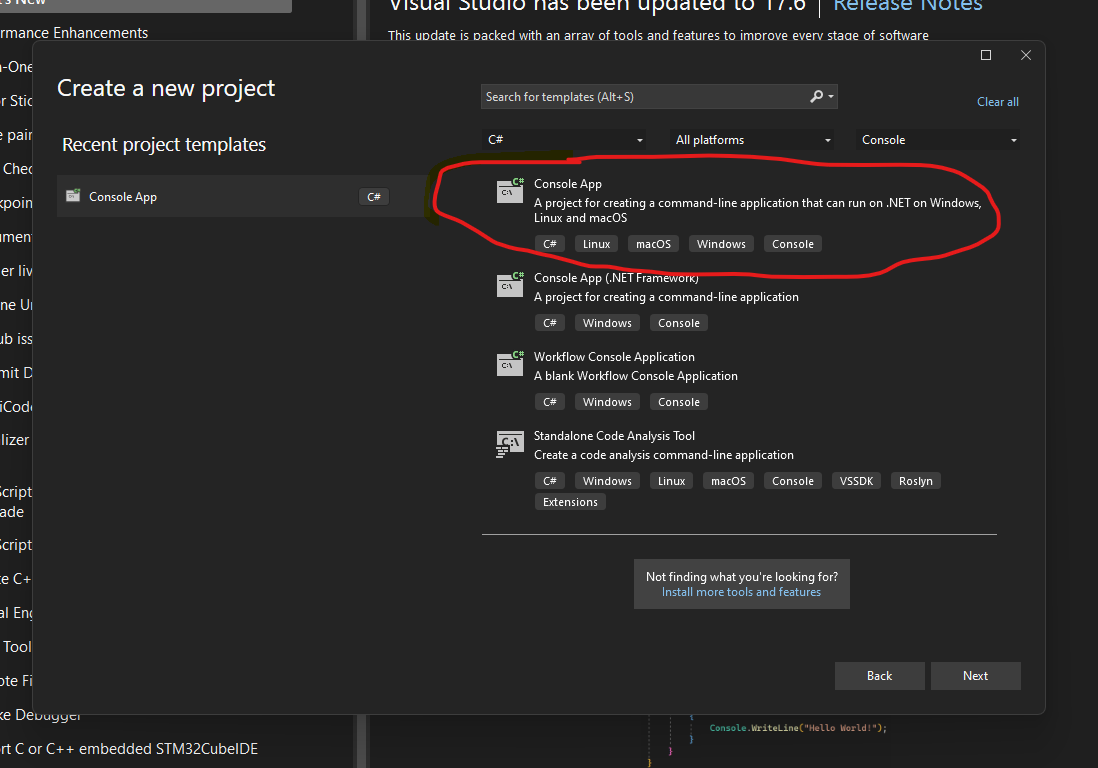
no I re-typed everything. no copy/paste
Ah well you've got the correct project type
Good you picked the right thing
ok so I'm here now
Okay, now use the two lines I showed tou
so when the user enters their username and presses Enter, I need to store the data to the json file, so should I do it in the main function or should I create a separate function to do that?
For now in main is okay..we can move it around later
these, right?
Yup, that and the File.WriteAllText
ok so small question. I pass the first line the
data
variable correct?
string json = JsonSerializer.Serialize(data);Ok I'm about to get on my plane now, best of luck
I'll be on the PC in a couple of mins
and then a comprehension question. What does the UserData class do with the username exactly? I could just as easily have
and it do the same thing right?
best of luck! be safe!
Have a safe flight
thanks for your help ❤️
The UserData class defines the name of the JSON field. And once you decide to have more entries for your json, you can add then to the class
so it could wind up to be something like
public record UserData(string Username, int Age, string Address);
and just a long list of parameters...
or would I revert back to using a proper class of getters and setters if I had multiple parameters like that
ok gotchaAnd what is cool is that you can define e.g., a class called Address and have that as a parameter of the UserData
Then would generate something like this
using this code here, and fixing main to be Main, I ran the program, put in a test username, but the json file didn't create
Did you add File.WriteAllText ?
I used this code. It added in the extra import all by itself. I didn't put
using SystemIO;
in there at all
idk why it even did thatBecause it needed it for File
oh ok
That seems fine, except try just using "test.json" for your pth
Then look in your bin directory
Bin/debug/net7.0/
it did make it and it did save it. It was in the bin folder which doesn't show up in my file explorer....odd
and I haven't made this change yet
why doesn't the bin or obj folder show in the file explorer?
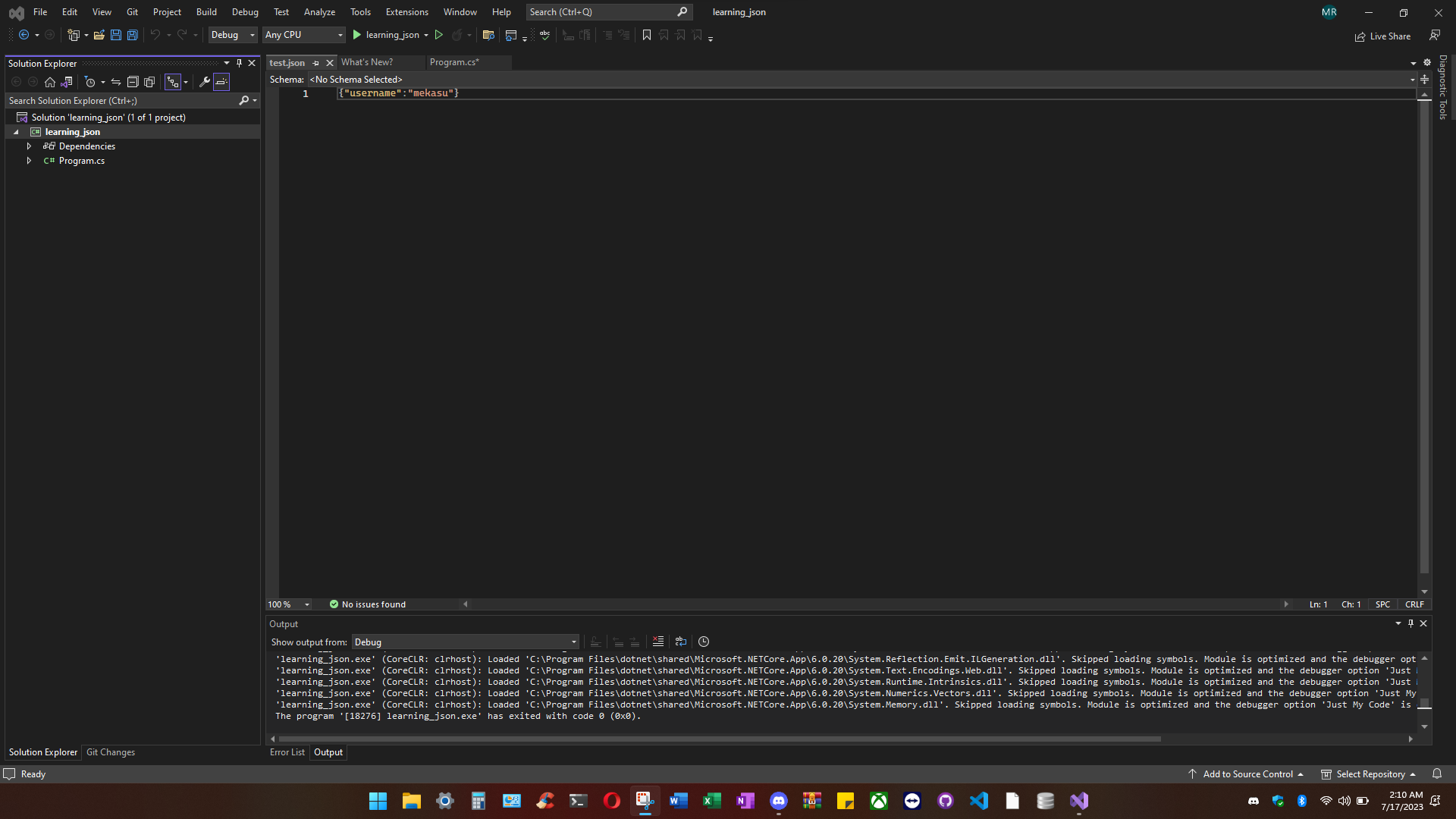
and how can I get it to put the file in the directory with the main.cs file instead of the bin folder?
Eh, that isn't really something you do
The bin folder contains your compiled application
oh ok
So yes, you can change the target directory of your JSON file
but you don't put that near your cs
because, once you want to share that program with your friends, they won't have the folder with the cs file
ok so going off your idea here, I tried to incorporate asking the address of the user. I got this far on my own, but am not sure how to proceed.
the address is not a string
ok cool. gotcha
ok I fixed that parameter
a slight aesthetic recommendation (because who doesn't like an instagram-grade look), use
int.Parse
instead of Converter.ToInt32
and added location into
UserData data = new UserData(username, location);
almoooost
ok made that change
you successfully created a new
Address
class, and you put it as a parameter for the UserData
that is wonderfulso I shouldn't try to bring it all together with one variable?
right
however, you did not set any of the
Address
object properties. You read the city, street, etc. into separate variables
So, you can change your code into the following:
of, you could also do
And that should be just fine
Ok, now another little itty bitty detail
in a very special case, your code will fail. And it is because you inherently cannot trust users to provide you correct data.so how do I get my json file to pretty print with an indent of 4?
ah, just a moment, I will find that
right so we would do data validation i.e. checking that the zip code is actually an integer
yes, that can be done quite easily
write int, then press
.
and check out what other methods are available
and try and use the popping up documentation to figure out how to use itlike what I did in my math game
int.TryParse(whatTheUserProvidedViaConsoleRead, out int theParsingResultThatYouThenSaveIntoTheAddressClass)
and the method TryParse returns a bool, indicating whether the parsing was a success or not
A small note
Convert.ToInt32
has a slight different behavior to int.Parse
/int.TryParse
Convert.ToInt32
will return 0
if the value passed to it is null
int.Parse
will throw an error, and int.TryParse
will return falseso
location.Zip = int.TryParse(location.Zip, zipCode);
?
or did I just wreck that whole thingok....so then should I use something different?
No no, keep using
int.TryParse
, just wanted to point out why they are different and why int.TryParse
is preferred over Convert.ToInt32
This how you'd enable indentation. Couldn't find how to set the indent size, tho
OMG NO
I left my icecream on the table and it melted
I totally forgot
Iirc there is a setting on the options class that sets indent size
It seems that it is not
this is perfect so far. it's a natural indent of 2 which I can definitely live with (client side web designer talking lol) and the script works with the integer validation. So now, here's a fantastic question lol what can I use to validate if an address is real or not?
oh boy
oh nevermind lol
we'll save that for a later date
easier question then
you'd probably have to find some API that provides that functionality, connect to it, send your address to it, and get a result
let's say I want to move my getters and setters to their own file, and put my input validation into it's own file. How would I do that, and how would i use that in this main file to clean up the code some?
Move the userdats class to it's own file..should be a quick action to do so already
It seems that Google provides an address validation API, but last time I worked with googles API I almost pulled my hair out
place cursor on the UseData class, and press...oh my, is it Ctrl+.?
should be
because like for my input validation (address speaking) I would want to check that the address starts with 1-6 digits, and the rest is a string and ends with a road extension (dr, st, ave, etc) and with my username input, I'd like to validate that it's a username with only letters and numbers
Ctrl+. Indeed
that'll get you a context menu for quickly moving it to a different file.
you would write your own method for that, or write a regex
Might I suggest static bool methods on your UserData class, with names such as ValidateUsername?
Then you can use regex or whatever you want inside them
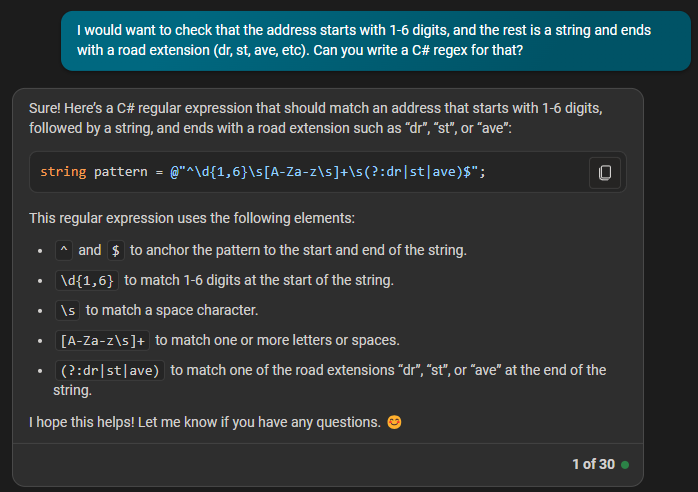
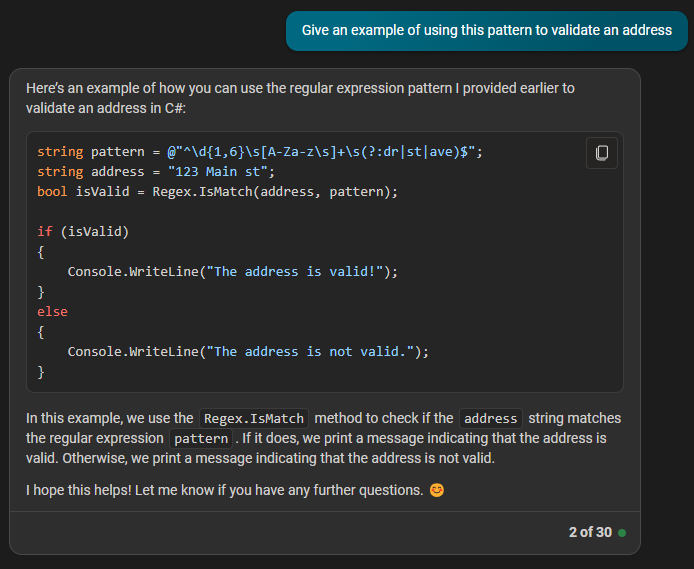
this worked, and I was able to copy/paste my address getter setter to the same file and it work just fine as well
um.....wut
Copy pasta leads to disasta
so what did I mess up?
Well, the UserData should be in its own separate file, same for the address. Not together
ok so if I move the address getter and setter to it's own file, then how would I deconstruct that one-liner for user data to incorporate address and write it like address is written?
Files are not super important in c#, if they share a namespace they are accessible to eachother by default
how would I put address into this?
The same way you did with username
because UserData is what is used to write tot he json file
But with your own type instead of string
just a side note, it is an
Address
class. Why did you change how the UserData looks? Keep the record
Just move it to a different file, called UserData.csyep
and that works just fine
ok so taking what I've learned here, I'm going to try and create my diary app and use the json file as storage (not ideal, but am still beginner)
It's perfectly fine to use a local JSON file as local data storage
I'd say JSON is a perfectly fine choice
ok so then I'm sticking with json
C# is all about keeping everything organized, separated into namespaces, files, classes, etc.
You keep your code organized, and you'll thank yourself once you need to fix some treaturous bug
becuase you'll know that the class that does that thing is here, and that thing seems to be failing.
Otherwise, you'd have spaghetti code, which sounds tasty, but is a nightmare to untangle. It should be called
Christmas lights code
insteadlmao I agree
ok so I'm using Visual Studio for this diary project. I have built the home page with 1 button
I'm not too fancy on styling and colors until I can understand functionality. So how do I create a new
.xaml
and .cs
file like what I have here to design my NewEntry page which will ask for a title, date&time, and the users entry with a button to submit or go back?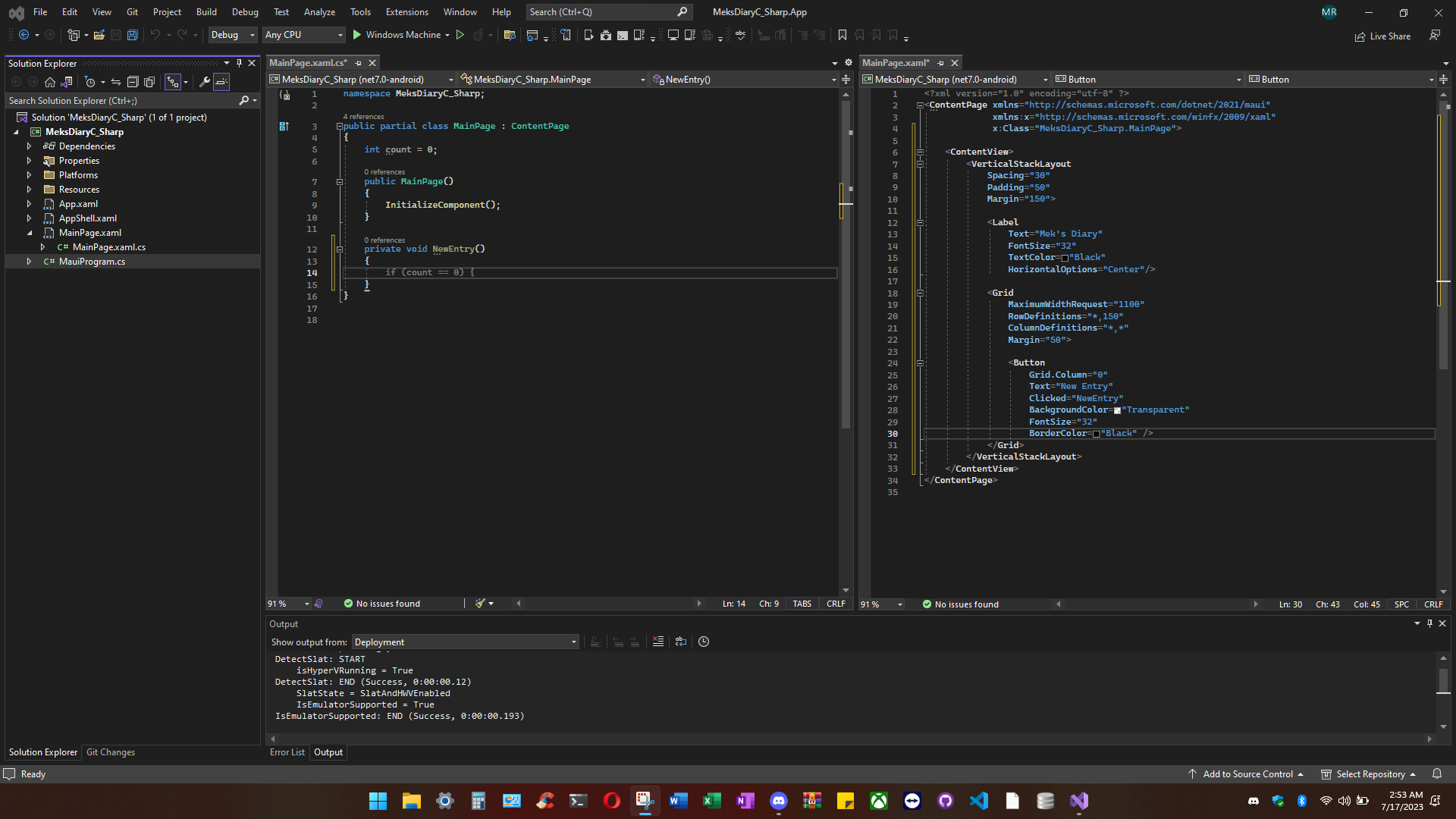
I remember from the tutorial on the math game that I right click the root project and select Add. I thought it was
class
that I selected to add and then selected a new content page from there, but that seems wrongNot class this time
I don't know Maui very well, but probably window?
what are you trying to add exaclty? Didn't quite understand
I'm creating a new page. So when the user clicks "New Entry" it will navigate to the new entry page
ah, just a sec, loading a new project
something like this
or however it's done. I can't fully remember tbh
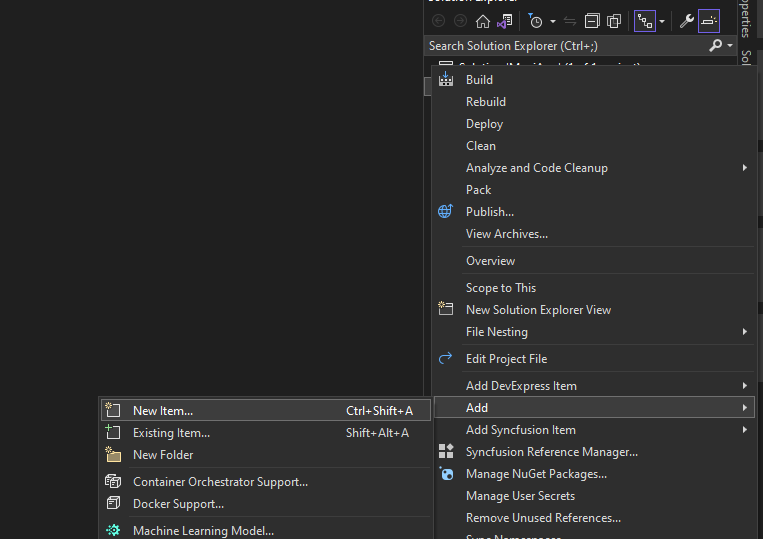
you right click the project, the one with the green
C#
icon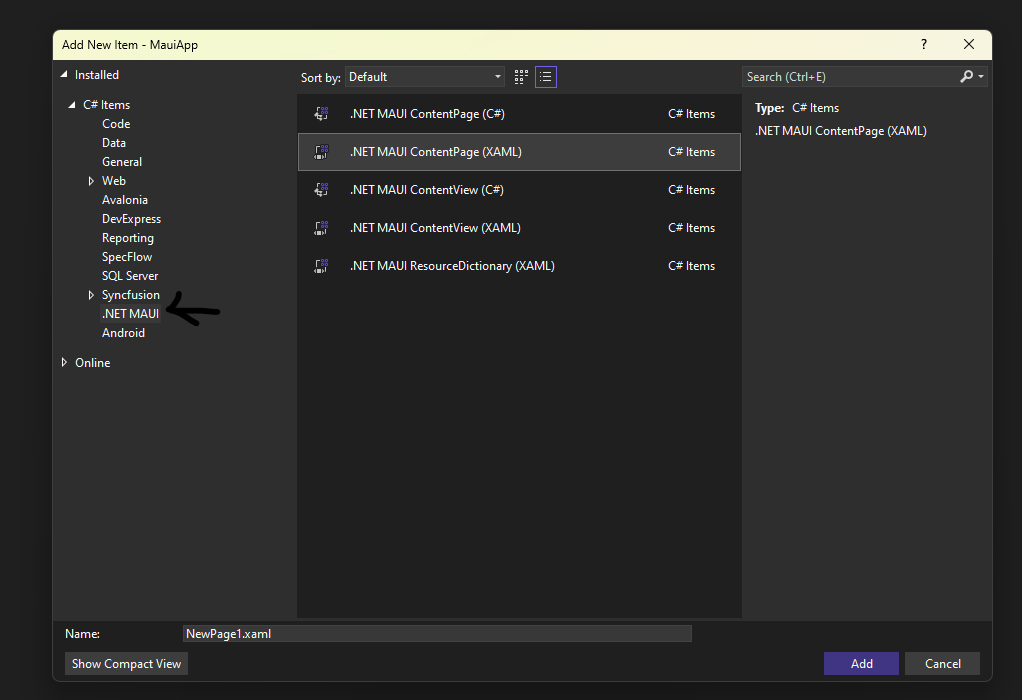
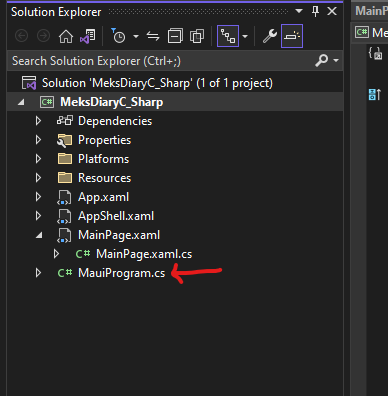
right-click that one?
Nope, that is a csharp file
oh the top one
The project is the second thing in this list, from the top
ok then select add
yes
ok then new item?
yes, just like the screenshot
then filter out for .NET MAUI
and select the context page XAML
ok that doesn't happen with the new item option. For me, that filtering is done when I select Add > class and a window pops up for me to do that
should be the same
Add New Item
window, but okwhen I right-click the project, and hover over Add and select class, I can then click MAUI on the left and select Content Page (xaml) and click ok
Yes, that is what you want
that gives me this so then how do I make the button on the home screen open this new entry screen?
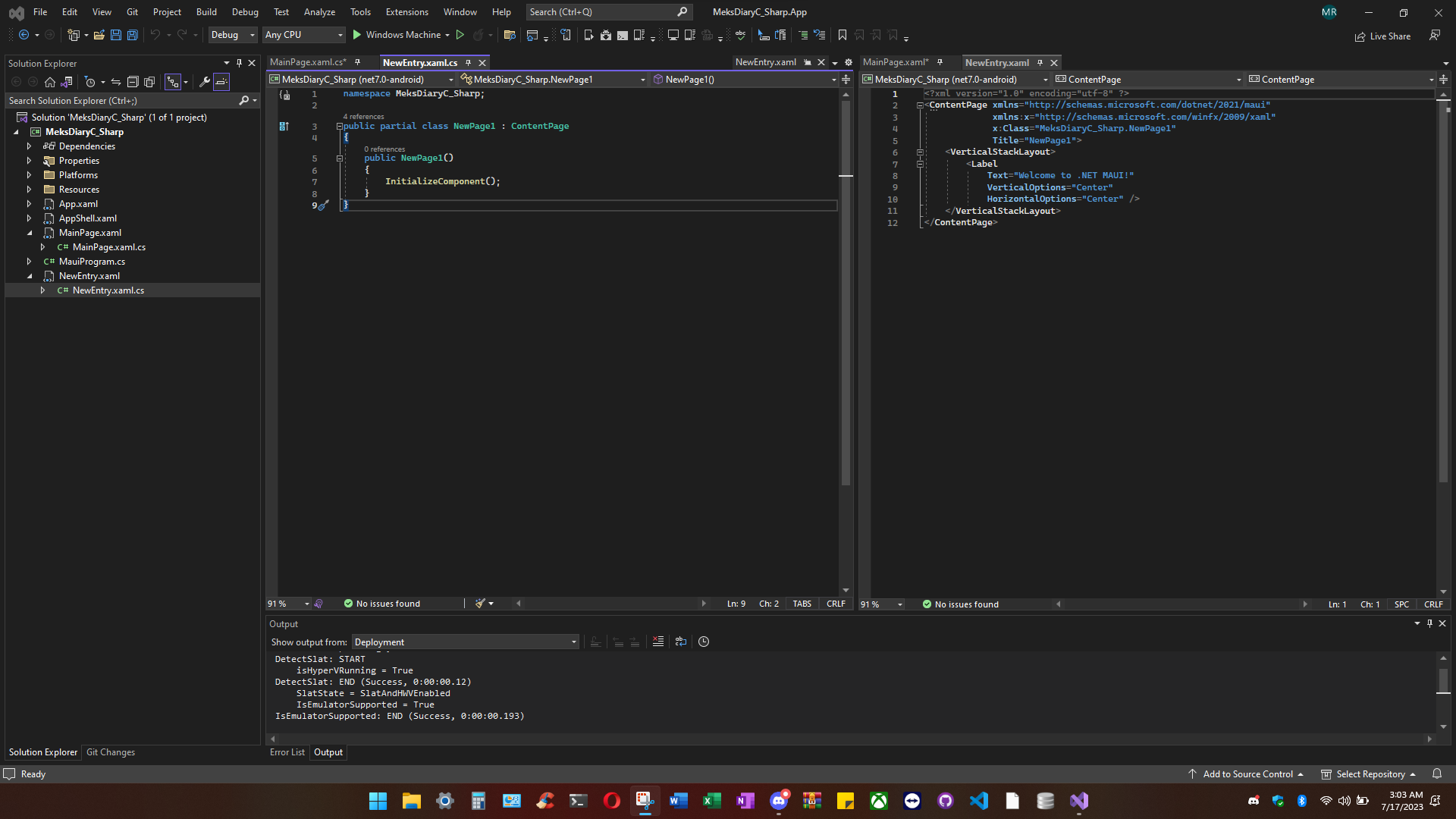
well, I've never done MAUI, but give me a second
let's try and google this
and you put that into your button click event
notice the async, before the void, that should be there
Just to be frank, I don't think MAUI is a great place to start. It's a new and very complicated framework
Is it really that complicated?
ok what's a better framework to start with?
I\ve never touched it
maybe WPF?
What are your goals with this program? Windows desktop?
it's very similar, I'd say
Then WPF for sure
Maui is for mobile apps primarily
yes. That's the only OS I want to start with in developing desktop apps. i know how to build executables using Visual Studio so I can build the .exe's for the other two OS's once I have the project finished
Thou its acronym is for Multi app.
WPF then imho
WPF is a wonderful, mature GUI framework.
Uhm, that second part of your message confuses me
there are tons of resources available for it
The "other two OS" being Mac and Linux I assume?
i know how to build executables using Visual Studio so I can build the .exe's for the other two OS's once I have the project finishedSo, you want to have a cross-platform application? You want it to run on Mac, linux and windows?
To support those, you have no option but Avalonia
.NET MAUI is cross-platform, but it does not support Linux
which is a controversial decision from Microsoft
Linux desktop
Has to do with the fact that linux has three or more different gui apis
But yes, it's a glaring omission
Yeah... they do love their freedom of choice and lack of consistency...
Yup
Ups and downs 🙂
ok so basically
- Mobile app dev is beyond down the line so I don't even want to think about that.
- This application is going to be developed for Windows first and the others down the line
- This application is only going to have 5 basic functionalities.
- - 1) Setup screen: get user's first and last name and email address -> store to json
- - 2) Creating a new entry: Title, Date & Time, Diary Entry, Save Button -> store to json, Back Button -> go to home page
- - 3) Editing an existing entry: Auto populate (same screen layout as new entry), Save/Back (to json/home page) buttons
- - 4) Deleting an existing entry: Shows a scroll view list of all entries in the format of
#) date/time - title
, Save/Back (update json/home page) buttons
- - 5) Sharing an entry: Email: Subject format Users First and Last Name - Journal Entry
Body = entry details date/time \n journal entry
, Send/Back (send it to desired email/Home Page) buttons
it's a simple app with a little bit of logicIf you are fine with having to rewrite the entire GUI down the line, you can start with WPF and later move to Avalonia
Or you bite the bullet and use Avalonia right now
Higher initial threshold but you will have full Mac/Linux support from the start
Your actual program should be fairly easy to make thou, based on your above requirements
I do not understand why everytime I close the solution and then close visual studio, and go into my file explorer and delete the project, it always tells me that the project is opened in another application and I can't delete it. I've closed the IDE completely....every time I have to restart my computer as a whole in order to delete a project from visual studio and I don't get that. I'll brb
it is. I've done all but the delete and share functionalities of it in python already
ok I'm back. I'm going to give avalonia a try
that's not an option when creating a new project, so how do I create a new avalonia project?
It's a third party frameworl
yes
You'll need to go through their setup instructions
in the long run, yes and I'll eventually turn it into a mobile app for ios and android too but that's a long ways down the road
https://avaloniaui.net/GettingStarted
Here's my project that uses avalonia, maybe it helps: https://github.com/hailstorm75/ModularDoc
And here's a list of other projects: https://github.com/AvaloniaCommunity/awesome-avalonia
for linux, I can just eat dust and build it in python and have the users compile it from source by cloning the github repo
Python and GUI development 💀
I thought you said you were a sadist, and not a masochist
I use PySide6. The only reason I hate it is because they have a GUI builder named QDesigner or QtDesigner. It allows you to build the screens, but you have to use spacers and it's so annoying because I couldn't figure out how to resize the spacers. Once you have the screens built, you save them into your project and then right-click and convert them into python files. After that, you just call the screens into your logic, access the child components and do what you need to do. I couldn't figure all that out, so I hand wrote out my screens which you can see in https://github.com/mekasu0124/MeksDiary which is the PySide6 framework in Python of what I'm attempting to do now in C#
Qt is horrible
😅 this is my little bit of masochism lmao
I do not disagree
oh my. avalonia has xaml and cs files just like maui....oh boy
All the modern gui frameworks do
Maui, wpf, Avalonia, winui, uwp
oh yay T_T
ok so the first difference I've found is the avalonia xaml page does not have
<ContentView></ContentView>
lolafk for a couple mins, in a meeting
ok so I don't have the preview window like it shows in the tutorial. How do I get the preview window?
Iirc you don't. You run the program and use hotreload
Xaml is a bit like HTML
Think of it like having a live server
ok so start the program and just alt tab back and forth. cool
how do I change the background color of the project? I tried doing
BackgroundColor="Black"
inside of the <Window></Window>
tag on the main pages xaml script, but it didn't like thatNo idea. Check Avalonia docs
okie dokie
as far as I know you don't get that
or you need an extension for visual studio for it
check the docs
I'm looking around, and am not finding anything. I'd be sad to know I can't change the background color lol right this very second, I'm trying to figure out how to change the text color of a label and a button
this is my issue rn
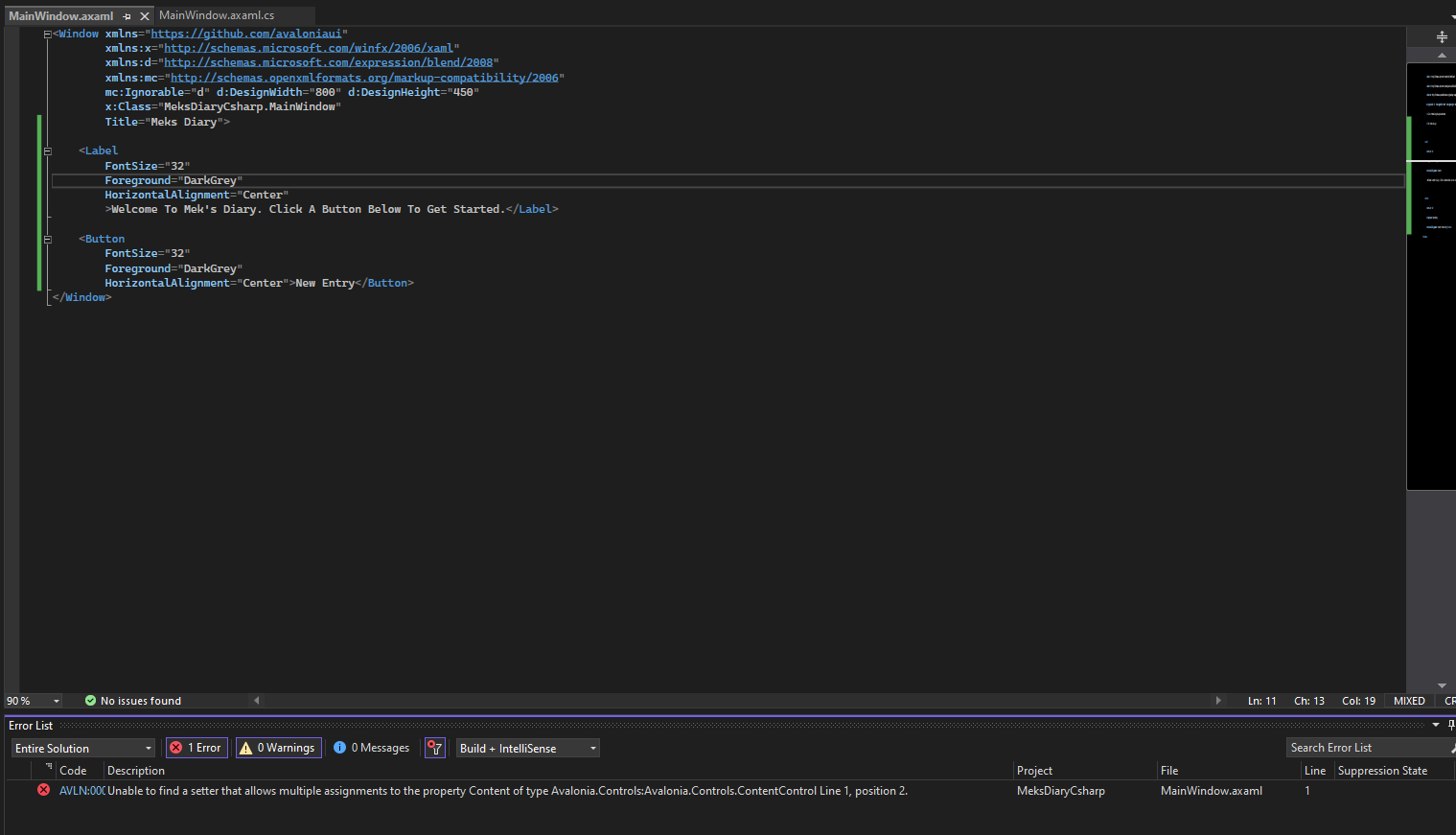
Well foreground and background are exaclty what you are looking for
I'm starting to get pissed off. I have tried foreground/background -> error. I've tried following https://docs.avaloniaui.net/docs/styling/ -> error. I have zero idea how to get this crap to style so I'm just going to quit trying to style it for now before I get aggravated at it
But I see the issue you have
Read the error message
you cannot have multiple content entries in the Window tag
This means that 🥁 you must wrap your label and button into some element container. E.g.,
Grid
, or StackPanel
omg. is it really that simple.....
You'd do the same in WPF, .NET MAUI...
the error message are very helpful
this isn't like C where all you get is
SegFault
. And you're just sitting there, staring at the screen not knowing what the hell is wrongI read that error message, but I can't say that I understood it. That just.....oh I wanna scream lol so now I have my black background and some styles on my label and button. grrrrrrrrrrrrrrrr
Keep reading them, don't outright ignore them. They'll start to make more and more sense
I hope so
Afaik, Rust has the best error messages. They literally tell you where you have an issue and suggest a fix
Code Golf Stack Exchange
Generate the longest error message in C++
Write a short program, that would generate the longest possible error message, in a standard C++ compiler (gcc, cl.exe, icc, or clang).
The score of each entry is the number of characters in the l...
oh jesus 21300 lines for an error message...
and here's the home page 🙂 the 4 basic functionalities, but this will be send to the home page file. I have to create a brain file that will check the json file if
data["setup"] = 0
and if that variable = 0 then it launches the setup screen, otherwise, it'll launch this screen.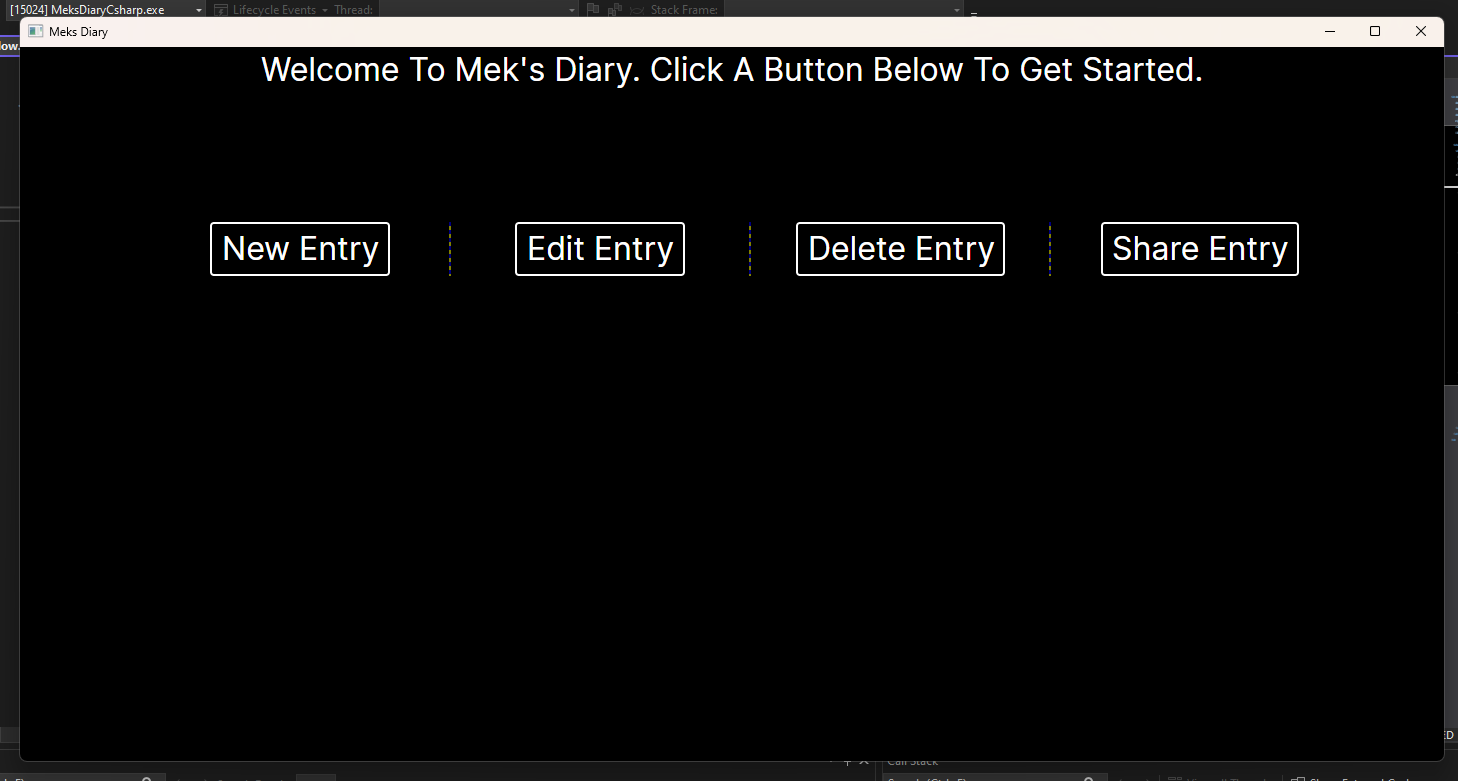
Brain file
sounds cool
business logic
But why check for "setup", if you can check if the json file exists?
And create a json for each diary entry?
This way, whenever the file does not exist, you start the creation wizard. Otherwise, you just load the file and display its contents
https://github.com/amwx/FluentAvalonia I use this for my theme
GitHub
GitHub - amwx/FluentAvalonia: Control library focused on fluent des...
Control library focused on fluent design and bringing more WinUI controls into Avalonia - GitHub - amwx/FluentAvalonia: Control library focused on fluent design and bringing more WinUI controls int...
ok so the label went to the center of the screen with no issues, but I'd like for it to come down some and I cannot get the grid to center within the window at all. What am I doing wrong that I can't get the label to come down and the grid to center?
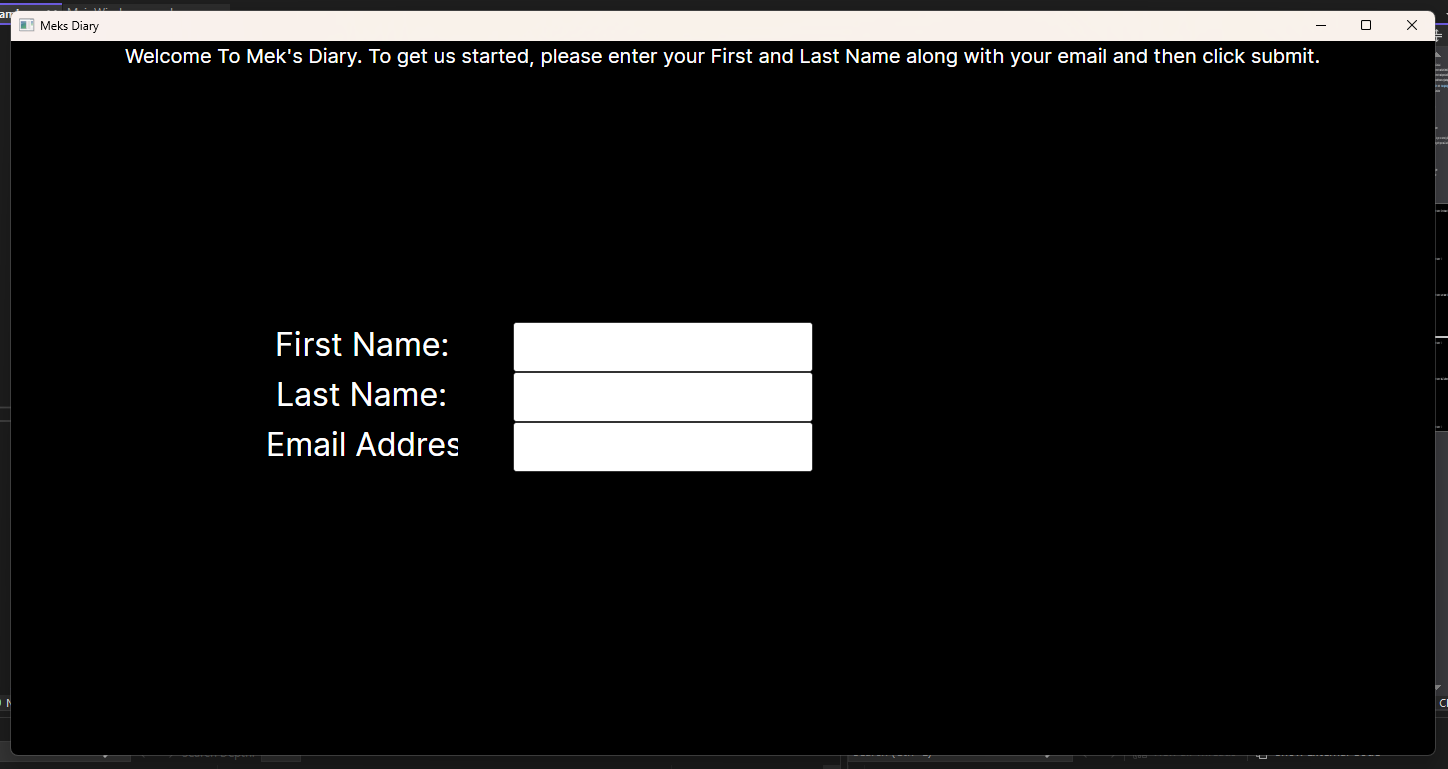
First things first
Avoid using specific values for the column and row sizes
Use
*
for using the maximum width/height
Auto
for using only what the controls within the cell need
you can also prepend the *
with a number. This is useful when you have multiple rows/cols with the *
size, and you want one of them to be bigger/smallerthat fixed that issue 😛 ty. so how do I increase the height of the rows
if I'm using the
*
Well, you could do
*, Auto, *
for the row definition
This will use a maximum height row, then a row of the height needed by the controls within it, then another maximum height row
To center the top label, try VerticalAlignment?that didn't do anything, but it's ok. It's not an important feature at the moment. What is kind of important, is how do I get a button to span the width of 2 columns?
like I'm trying to get these two buttons at the bottom to both be matching widths which would be 2 columns each and I'm trying to center the text within the button to the center of the button
I got it figured out 🙂
Try to add a parameter to the button:
Grid.ColumnSpan
google is your friend
and ChatGPT is your peer programmerI tried the column span and it didn't work so I did this instead
I promise I'm not after just quick answers to my issues. It took me 20 minutes of looking through google and asking my question 5 different way to get what I needed to solve that. I promise I'm googling lol so now. With your idea of checking if a file exists, how would I go about doing that? Right now, my setup screen is in my MainWIndow file. Where would I put my logic for checking if the json file existed and launching this screen if it didn't?
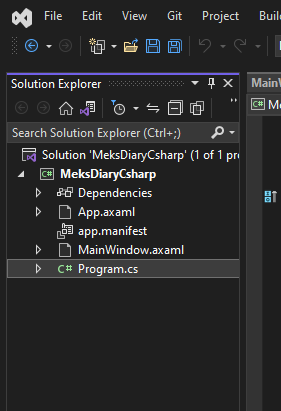
like would my logic for that go in the MainWindow's cs file and then move all of this to a setup screen file?
So, when it comes to these modern UI frameworks, the correct way of organizing your code is using MVVM:
Model - the classes that represent your data, e.g.,
DiaryEntry
View - the axaml
, the UI that the user interacts with. It is the MainWindow.axaml and the MainWindow.axaml.cs. The cs file is responsible for doing UI related things.
ViewModel - the backend for the UI. It fetches data, wraps it up in Models and provides those models to the View.
This is a very watered down description of what MVVM is. This separation helps you keep everything organized. But....getting into MVVM can be quite overwhelming. So, if you want to do it properly, get yourself some migraine pills. But once you understand it, it's a very powerful tool.
If you skip MVVM and it the "quick and dirty" way, then I suggest writing most of the stuff in the MainWindow.axaml.cs
And create special classes for, e.g., handling the JSON.
I'd create a class called DataManager. It would handle loading, saving, and checking data.
This class should be static, so that you can access it from anywhere without creating an instance of it - same thing as Console
You do not create a new instance of Console
when you need to ReadLine
or WriteLine
To make a static class, do the following:
notice how the class and the methods have static
keyword
when a class has the keyword, all of its members, i.e., methods, properties, fields, have to be also static
You can remove the static keyword from the class, but in this case I'd keep it
The most important thing is to have the methods static - they will be accessible without doing new DataManager()
And can simply access the method like so DataManager.EntryRecordExists(pathToRecord)
This tutorial series might help: https://www.youtube.com/watch?v=YhKuZImznEE
AngelSix
YouTube
001. Avalonia UI - Getting Started Environment Setup
Support me in my journey to giving back to the industry all my knowledge and helping the world with what I do. Spreading knowledge to those who cannot afford an education, and helping those who want to better themselves.
Avalonia UI
https://www.avaloniaui.net
Support what I do and pay for me to survive
https://www.angelsix.com/donate
Live Cha...
The MVVM confuses the hell out of me. I’m not even fixing to lie. I’ll check out that tutorial tomorrow after work. I’m a bit tied up this evening.
Feel free to ask questions whenever
Ok bet 🙂 tyvm
ok so my first question would be, in an avalonia application, where would I put my logic for checking if the json file exists when the program first starts?
would it go in Program.cs?
so technically, the first thing I want to do is make a loading screen. This loading screen will be the first thing to launch and it checks all the dependencies and such to "load" them. After "loading" everything, it'll then check if the json file exists. I'd like for this loading screen to be as realistic as possible so as it iterates through all the directories in the project, it'll show them on the label with the
{Binding LoadingText}
as the string being passed to the label. The ProgressBar
will go from 0% to 100% respectively and once the application is done "loading" it'll launch the appropriate screen -> Setup if json file doesn't exist, and home screen if it does exist. Here's what I have so far and I'm not sure how to progress from here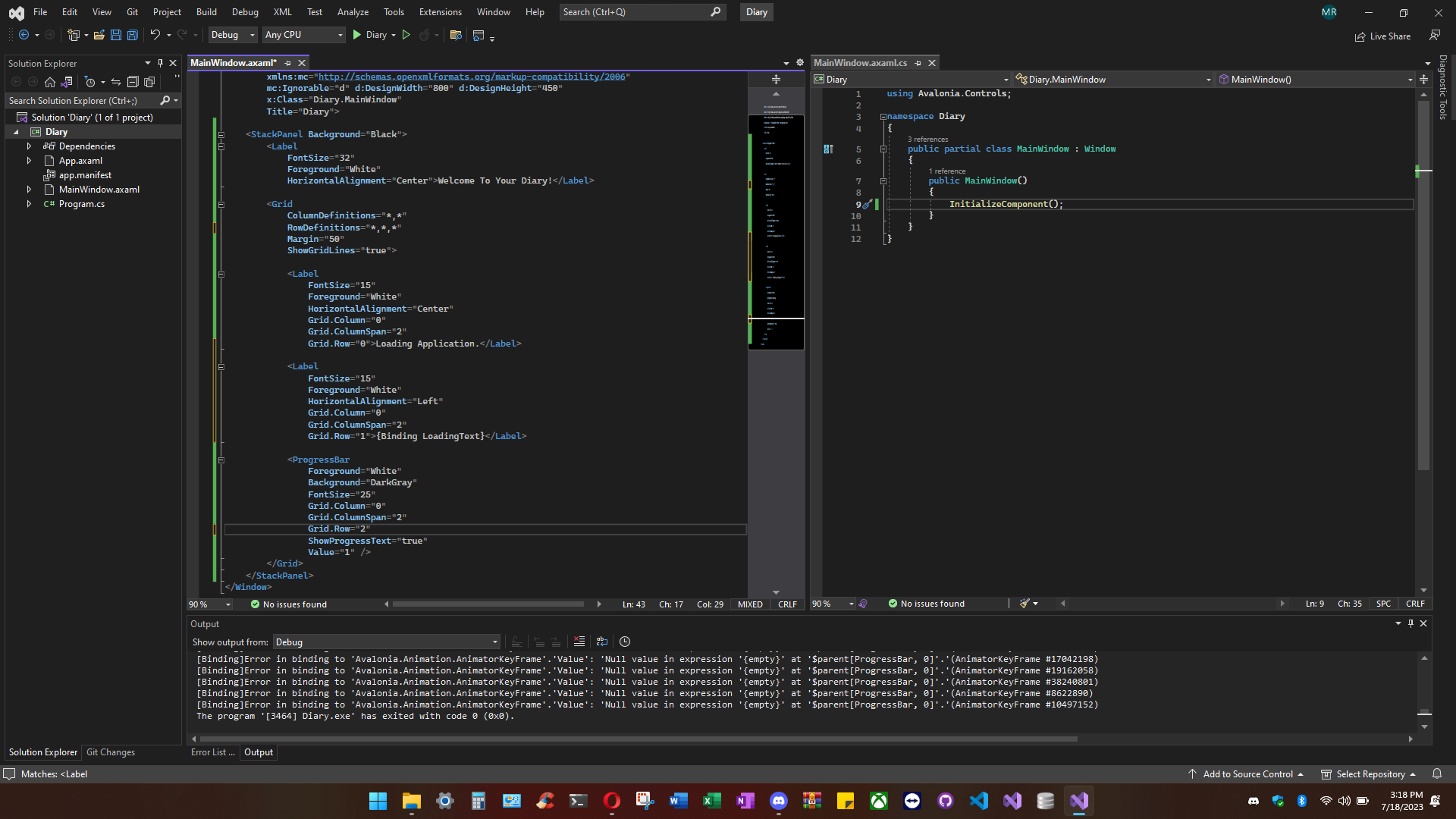
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.