Cannot play .mp3 user attachments
Hi there, this command is supposed to allow the user to upload a .mp3 file for the bot to play, but I cannot get it to work.
Here's how I wanted to work.
1. User requests /playmp3 and has the option to upload an attachment (.mp3) to play (see photo for example).
2. The bot then plays that given .mp3 file in the voice channel.
I have already gotten this to work for raw .mp3 file links, but I also want attachment support.
However, I am getting this error:
I'm assuming Discord.js doesn't support attachments for playing? How could I approach this? Maybe be downloading the .mp3 locally to a folder temporarily and playing it through there? Here is my current code, thanks in advance!
Error executing playmp3
TypeError [ERR_INVALID_ARG_TYPE]: The "body" argument must be of type function or an instance of Blob, ReadableStream, WritableStream, Stream, Iterable, AsyncIterable, or Promise or { readable, writable } pair. Received an instance of Attachment
Error executing playmp3
TypeError [ERR_INVALID_ARG_TYPE]: The "body" argument must be of type function or an instance of Blob, ReadableStream, WritableStream, Stream, Iterable, AsyncIterable, or Promise or { readable, writable } pair. Received an instance of Attachment
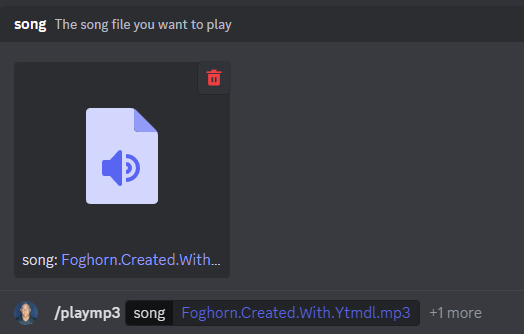
1 Reply
const {SlashCommandBuilder} = require('discord.js');
const {joinVoiceChannel, getVoiceConnections, createAudioPlayer, NoSubscriberBehavior, createAudioResource } = require('@discordjs/voice');
const data = new SlashCommandBuilder()
.setName('playmp3')
.setDescription('Play some music from an .mp3 file! 🎶')
.addAttachmentOption(option =>
option.setName('song')
.setDescription('The song file you want to play')
)
.addStringOption(option =>
option.setName('url')
.setDescription('The url of the song you want to play (must be .mp3 file)')
);
const {SlashCommandBuilder} = require('discord.js');
const {joinVoiceChannel, getVoiceConnections, createAudioPlayer, NoSubscriberBehavior, createAudioResource } = require('@discordjs/voice');
const data = new SlashCommandBuilder()
.setName('playmp3')
.setDescription('Play some music from an .mp3 file! 🎶')
.addAttachmentOption(option =>
option.setName('song')
.setDescription('The song file you want to play')
)
.addStringOption(option =>
option.setName('url')
.setDescription('The url of the song you want to play (must be .mp3 file)')
);
module.exports = {
data: data,
async execute(interaction) {
if (!interaction.member.voice.channelId) {
await interaction.reply('You must be in a voice channel to use this command!');
return;
}
if (!getVoiceConnections().has(interaction.guildId)) { // if bot is not in a voice channel
joinVoiceChannel({
channelId: interaction.member.voice.channelId,
guildId: interaction.guildId,
adapterCreator: interaction.guild.voiceAdapterCreator
});
}
if (interaction.options.getAttachment('song') === null) { // only url provided
if (interaction.options.getString('url') === null) { // no user input at all
await interaction.reply('Please provide a .mp3 file!');
return;
}
const resource = createAudioResource(interaction.options.getString('url'));
resource.metadata = {
title: interaction.options.getString('url').split('/').pop(),
url: interaction.options.getString('url'),
};
if (!resource.metadata.title.includes('.mp3')) {
await interaction.reply('Please provide a raw .mp3 file!');
return;
}
module.exports = {
data: data,
async execute(interaction) {
if (!interaction.member.voice.channelId) {
await interaction.reply('You must be in a voice channel to use this command!');
return;
}
if (!getVoiceConnections().has(interaction.guildId)) { // if bot is not in a voice channel
joinVoiceChannel({
channelId: interaction.member.voice.channelId,
guildId: interaction.guildId,
adapterCreator: interaction.guild.voiceAdapterCreator
});
}
if (interaction.options.getAttachment('song') === null) { // only url provided
if (interaction.options.getString('url') === null) { // no user input at all
await interaction.reply('Please provide a .mp3 file!');
return;
}
const resource = createAudioResource(interaction.options.getString('url'));
resource.metadata = {
title: interaction.options.getString('url').split('/').pop(),
url: interaction.options.getString('url'),
};
if (!resource.metadata.title.includes('.mp3')) {
await interaction.reply('Please provide a raw .mp3 file!');
return;
}
const player = createAudioPlayer({
behaviors: { // music will pause if nobody is in channel
noSubscriber: NoSubscriberBehavior.Pause,
},
});
const connection = getVoiceConnections().get(interaction.guildId);
connection.subscribe(player); //
player.play(resource);
await interaction.reply('Playing ' + resource.metadata.title + '!');
} else if (interaction.options.getString('url') === null) { // only file provided
const resource = createAudioResource(interaction.options.getAttachment('song'));
resource.metadata = {
title: interaction.options.getAttachment('song').name
};
if (!resource.metadata.title.includes('.mp3')) {
await interaction.reply('Please provide a raw .mp3 file!');
return;
}
const player = createAudioPlayer({
behaviors: { // music will pause if nobody is in channel
noSubscriber: NoSubscriberBehavior.Pause,
},
});
const connection = getVoiceConnections().get(interaction.guildId);
connection.subscribe(player); //
player.play(resource);
await interaction.reply('Playing ' + resource.metadata.title + '!');
}
}
};
const player = createAudioPlayer({
behaviors: { // music will pause if nobody is in channel
noSubscriber: NoSubscriberBehavior.Pause,
},
});
const connection = getVoiceConnections().get(interaction.guildId);
connection.subscribe(player); //
player.play(resource);
await interaction.reply('Playing ' + resource.metadata.title + '!');
} else if (interaction.options.getString('url') === null) { // only file provided
const resource = createAudioResource(interaction.options.getAttachment('song'));
resource.metadata = {
title: interaction.options.getAttachment('song').name
};
if (!resource.metadata.title.includes('.mp3')) {
await interaction.reply('Please provide a raw .mp3 file!');
return;
}
const player = createAudioPlayer({
behaviors: { // music will pause if nobody is in channel
noSubscriber: NoSubscriberBehavior.Pause,
},
});
const connection = getVoiceConnections().get(interaction.guildId);
connection.subscribe(player); //
player.play(resource);
await interaction.reply('Playing ' + resource.metadata.title + '!');
}
}
};