❔ Number guessing game.
I'm trying to make a program that generates a random number between 1-100, let's the user guess till they get the correct number, tells the use if they guessed too high/low and also let's them know if they guess correctly and if so, tell's them how many guesses it took. But I want to make it more complicated by letting the user know when they are close to the number.
This is how my code looks so far:
using System.Diagnostics.CodeAnalysis;
Random random = new Random();
bool playAgain = true;
int min = 1;
int max = 101;
int guess;
int number;
int guesses;
while (playAgain)
{
guess = 0;
guesses = 0;
number = random.Next(min, max);
while (guess != number)
{
Console.WriteLine("Try to guess the randomly generated number " + min + "-" + max + ":");
guess = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Guess: " + guess);
if (guess < number)
{
Console.WriteLine("Number " + guess + " is too low...");
}
else if (guess > number)
{
Console.WriteLine("Number " + guess + " is too high!");
}
guesses++;
}
Console.WriteLine("You won! Good job!");
Console.WriteLine("Total guesses; " + guesses);
}
29 Replies
If the correct number is 45 and the user guesses 44, i want the program to say "It's getting really warm" but not tell the user if the number is higher or lower.
just get a abs value of the delta
and have a switch case that deals with close values
probably easiest way i can think of
and use the default just the standard response
return "You are basically on fire";

in python I did it like this:
print("Hi, welcome to the number guessing game.")
import random
number = random.randint(1,100)
guess = 0
while guess !=number:
guess = int(input("Guess:"))
if abs(guess - number) <=3 and guess !=number:
print("You're really close, keep going.")
elif guess == number:
print("You guessed correct!")
elif guess < number:
print("Higher!")
else:
print("Lower!")
Can I do it in a similar way on C#?
yes?
abs
is Math.Abs
in C#i'm asuming else if (c#) is python's variant of elif
can I use else if multiple times?
yes
however, consider using a switch instead
switches in C# are very powerful, as they can do pattern matching
I've never used that before. :/
its similar in concept to
if+elseif+elseif+...+else
but its a nicer syntax
Look at icePhoenix message above for an exampleIcyPhoenix
Quoted by
<@105026391237480448> from #Number guessing game. (click here)
React with ❌ to remove this embed.
How would that look in my program? I've been studying c# for just a few hours total so not any good at it yet
I'll try playing around with switches a bit
I'm completely lost 💀
Can you indicate where you are lost?
This entire part so far. I want to find a tutorial on it but so far none of the tutorials I've found use numbers
why do you need a tutorial?
that example covers it all
how do i write the so it's stands out from my normal text on discord btw? it was something like '''code'''
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/tripple backtick, the letters
cs
, then end with tripple backtick
for a whole block
for inline, use a single backtick for start and end
inline code
it shows an error when i write (absDelta)
sure,
absDelta
is just a placeholder variable hereso it's normal?
assuming you didn't make a variable with that name... yes?
its just a variable name
in this example, probably containing the absolute delta value
between the answer and your guess
i regret not going with if statements rn
entire code is red and i have no clue what i'm doing.
Show me.
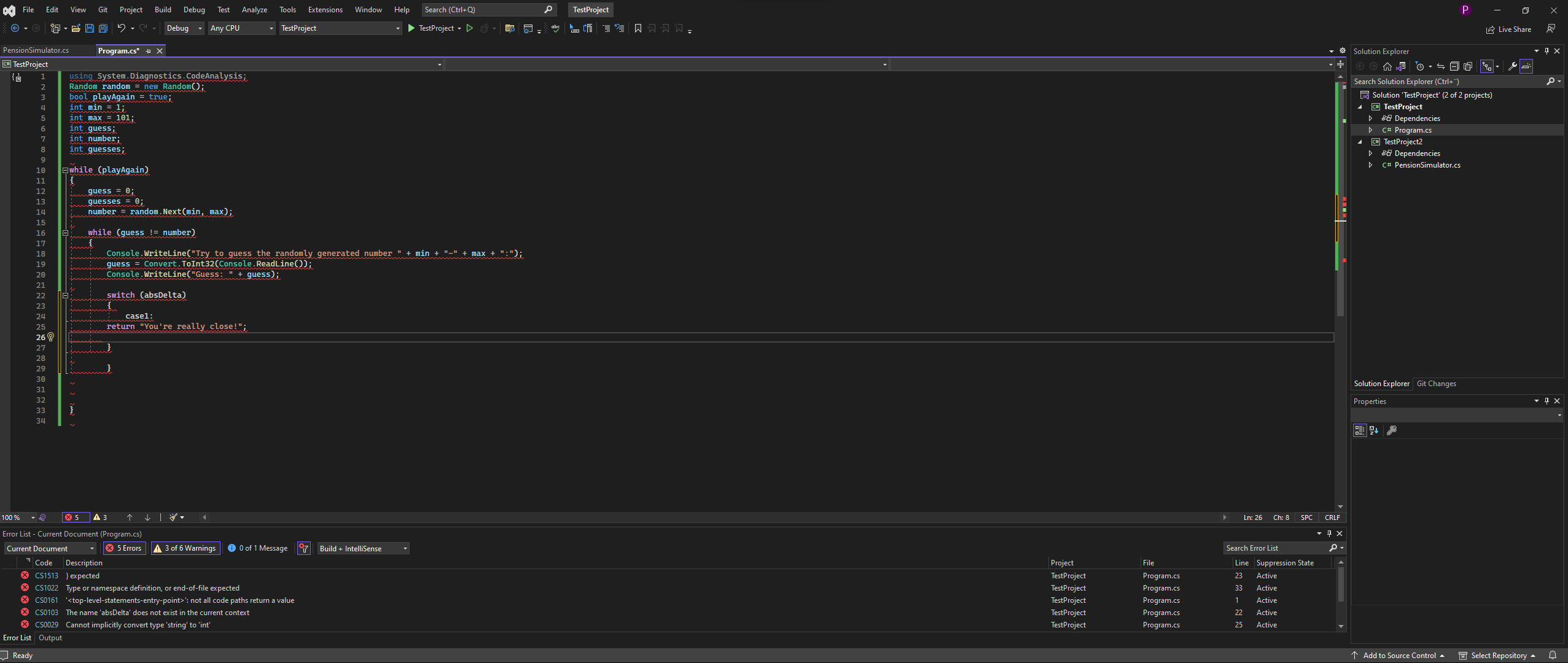
case1:
should be case 1:
you have red everywhere because the compiler can't understand what you are trying to do
Also part of the reason why I suggested you don't use top-level statements
u can do this as well
the number (or what they refer to as abs) would be the difference between the user input and the correct value (so shouldnt be hardcoded
top level statements are really confusing yeah lol
thanks for the help!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.