❔ Interfaces, IEnumerator and IEnumerable
Hello! I just started learning about these topics today and from looking at answers and explanations of these terms I am even more confused. So far, for interfaces I understand that there are like classes that cannot be used to create objects but force other classes to have their methods or fields contained in them. For IEnumerator and IEnumerable, i'm just completely lost. I think im a little dumb but I understand that this is basically explains how the foreach functions by turning our into something else that the compiler turns our code into. The IEnumerator has two functions of like movenext() and current and the ienumerable calls a method (GetEnumerator()) which gives an IEnumerator? So in brief, my two questions are this:
1. I was wondering when we would actually use interfaces compared to just adding another method under a class or using a switch case or other functions. I know that interfaces is kind of a work around for the non-existent multi-inheritance which doesn't exist in C# but I can't figure out a good reason for them.
2. How does the IEnumerator and IEnumerable works because I see that you also have to implement this piece of code as well.
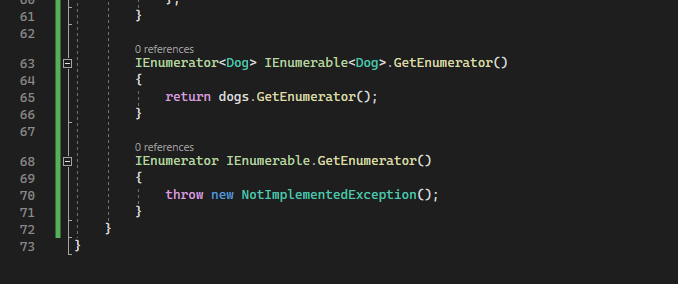
22 Replies
for #1, interfaces allow you to define certain requirements for an object without tying your code to any specific implementation
a common use case could be testing when you want to replace a service with a fake version that gives you specific results to test against
For most custom collections, all you need is a backing private collection type and return it's GetEnumerator
take all of the IEnumerable<T> extension methods for example, they work on any class that implements IEnumerable<T> without knowing what the actual object type is
Sorry, I'm just a little confused by what you mean on the case where you have to replace a service with a fake version that gives you specific results to test against
By custom collections, does that mean like collections that aren't generic?
Like the one in your screenshot
Implementing IEnumerable<T>
say you want to test a class that has a dependency on some other service, like an email service. when you're testing your code you don't actually want to send out an email, so if you define an IEmailService interface it's easy to provide your class a "dummy" service just for testing. you may want it to just save the email as a string so you can check that your class is trying to send the right message, and if your class just depends on an IEmailService interface you can give it any implementation you want without having to change the class itself (this relates to dependency injection, not sure if you've seen anything like that yet)
If you check the members of IEnumerator, its an object that handles the state of an ongoing enumeration
Can be useful to enumerate manually with it in some cases
Ohh so like you have an interface called IEmailServices and a class that uses that inerface. Inside this inteface, we place like an empty method but acts like a placeholder until we want to send the actual email?
And yea I haven't seen dependency injection yet 😭
So this IEnumerator allows me to loop through my object collection?
Yes, the dogs colleciton you have already provided an enumerator so you can just use that
For the non generic method, either call dogs.GetEnumerator again or your class's GetEnumerator
I see, so if it were a non generic, I would use the one at the bottom because that would account for the types of objects inside that collection. For the one that returns the dog collection, Does the IEnumerable<Dog> part allow the .GetEnumerator() to function? So like without INumerable<Dog> we wouldn't be able to use .GetEnumerator()? as well, the IEnumerator just allows us to check the current object in the collection and move on to the next object in the collection
The implementation of generic IEnumerable gets called in almost all cases. The non-generic one is implemented explicitely and will only be called if the object is referenced as a non-generic IEnumerable
The enumerator is the same except the Current property is System.Object in the non-generic case
So depending on if you know the type of items in the collection, the enumeration will either return that type or Object
By object in this case, it refers to various types of datatypes?
The literal System.Object that can hold anything
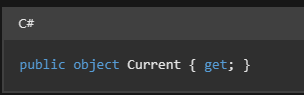
Ok i'll try looking more into that
I appreciate your help and responses! Thank you!
I would like to share my own explanation of why Interfaces are useful.
Interfaces allows you define the signature of methods and property. When I say define the signature I mean that you only set the method's name and parameters. For example:
There is my
IMathOperations
interface (Note that the name starts with I
it's a naming convection). My interface could now be implemented by any class. And any class that implements my interface will have to define what the sum()
method does.
So, what all this means is that I might have a bunch of classes implementing the IMathOperations
interface, and I can be sure that all of them have the method sum()
. That's clean code. It will help when you are coding using the IDE intelligence.I see, but wouldn't it be better to just add a method for that in the class without writing an interface?
Cause if you write an interface, you would have to write the sum method inside the class regardless right?
So wouldn't it be cleaner if you just don't state the interface?
Well, It doesn't make sense if you are not defining the same method in multiple classes. Anyway, it's a good practice to define certain type of methods using interfaces. Just in case you want to implement it in another class in the future. And also keep in mind that the definition of the method that the interface sets is required in the class that implements it. As I said
I can be sure that all classes that implement the IMathOperations interface have a definition for the method sum().
.Ohh I see what you mean, by having that there, it's much more clear to the user and me on what the code is actually doing, Got it, thank you so much for the tip
That's noting, bro. I happy you can see clearly now. I will find lots of implementations with Interfaces.
Haha thanks, I appreciate the help!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.