next auth jwt session
Hello im having some problems with making the session for credentials provider i managed to make it for google provider using the Prisma adapter but since there's no adapters for the credentials i tried to make it with jwt but i don't have any idea if this is the right implementation
That's the github repo : https://github.com/CLOG9/PuzChess
And that's the auth code im having doubts about (from
app/api/auth/[... nextauth]/options.ts
):
GitHub
GitHub - CLOG9/PuzChess: a new chess puzzles platform made with nex...
a new chess puzzles platform made with next js. Contribute to CLOG9/PuzChess development by creating an account on GitHub.
158 Replies
May I ask, why JWT.
Idk im new to this tbh
Should i use database session instead?
JWT is not really recommended unless you're doing state-less auth.
Like B2B, stuff where you just need to know if they're allowed or not.
(B2B, Business To Business)
OWASP recommends them for authentication yeah.
OWASP is huge for anything application security.
I couldn't find a good ressource to knw how to make database session
so basically i used the database strategy, it works as excpected for the google provider but i doesn't save the session for the credentials provider, when i changed it to jwt it works fine for both except that it doesn't saving the session in db for the google provider (and thats obvious)
here's the block of code
@thatbarryguy u here?
@CLOG, I've ran into this issue before. For some reason next-auth restricts the credentials provider to only JWT (so no db sessions) unfortunately. The second paragraph on the Credentials Overview page states the following: "It comes with the constraint that users authenticated in this manner are not persisted in the database, and consequently that the Credentials provider can only be used if JSON Web Tokens are enabled for sessions."
So either using jwt for both providers or just droping the credentials provider
@0xahmad
thats the code until now
when using credentials it return user undifined and the account has no token_id
it works fine for the google provider
This is my set of callbacks for custom login
it should return undefined, except for the time you hit
signin
even when i hit sign in it returns it
nah it should return it one time
i'll try ur code
put console logs in there and see when it does/does not return
you'll understand it's flow
sure what about the sign in callback? should i use it if im using the middleware?
here's my provider for context
yes you should use it, middleware should not interfere with your sign in process
for example here's my implementation of using the signin hook
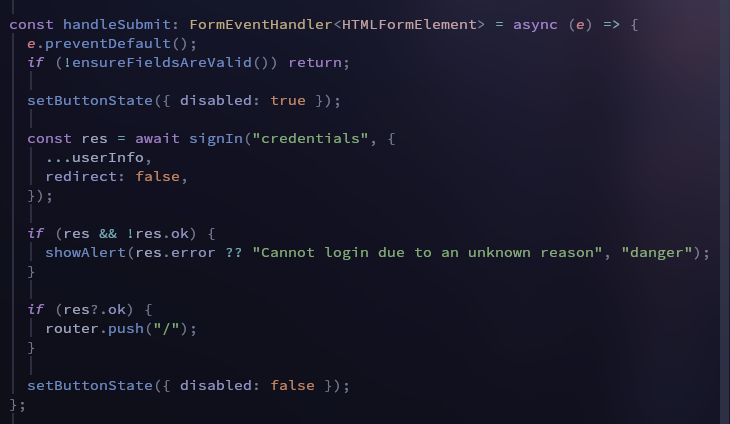
but in this project, I hadn't needed to use any middleware, so I can't show a middleware example atm as running this project requires some prior setup
thats what im having in the middleware :
so it's not even catching for
/auth
(if you have it setup under /auth
like any normal setup)
what is the default
middleware? 🤔i don't understand purpose of the sign in callback, so i have to make it look like this
I've always setup a function if I wanted to use middleware, never used default
? what do you mean ?
you shouldn't need any middleware for this
unless next auth has changed
im protecting routes
I see
so then you should call default and set it up according to the docs
i will change it to a function later im going to make user based role
I handled the route protecting myself so I didn't set it up that way oddly
u protect it with server session?
well they setup http cookies, so I utilized my own middleware function and serverside validation,
Plus I had setup a hook at the base of the page which ran with an interval and revalidated by hitting a
/auth/session/verify
endpoint. Logging the user out if they were not validatedwell to make things clear to you because im kinda lost i followed this tut :
https://www.youtube.com/watch?v=w2h54xz6Ndw
Dave Gray
YouTube
Next-Auth Login Authentication Tutorial with Next.js App Directory
Web Dev Roadmap for Beginners (Free!): https://bit.ly/DaveGrayWebDevRoadmap
In this Next-Auth login authentication tutorial with Next.js app directory, you will learn how to protect all of your pages with credentials or an OAuth login from providers like GitHub. Learn how to protect any page in your app.
Get CodiumAI for FREE: https://mark...
i see, but is my way good or should i make it like u did?
you should stick with them if you're not familiar with handling sessions properly, but it's not really a big thing to handle so if you want to you can do it on your own
So to answer your question : it depends
so u used HOC here?
yep
and what about user based role?
here's an olderr example, which I don't recommend you do it this way, but it gets the meaning through
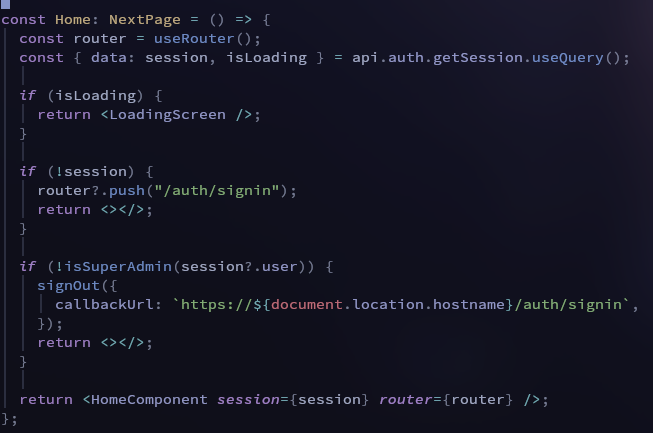
middleware handles it
I apply patterns via next's middleware function
plus my projects are literally separated by roles
I have a monorepo as so:
- apps
- user front-end
- admin front-end
which are deployed to separate domains
this is more extreme, yes, but the client requires it and I got the hang of doing it
but
for normal projects, it's just more work to handle.
so I just setup a simple middleware to handle it
which does like 95% of the work
im having an error with the callback code u provided
how are you returning your payload from the
signin
handleraaaaa i remember the second option u told me about, the route protection by seperating the project
Good? well if you can do it, then it's more secure as it's decoupled and there's no chance of unauthorized admin access unless the attacker has your admin info
But for the most part middlewares handle it really well also.
check it properly, this should not be occuring
i think im missing something, where should i find this handler?
^ this
aaa the authorize method
you're returning nulls, which is raising an error
that's bad practice
you should raise the appropriate http errors
how can i fix this?
take a look at my implementation
for example
you should be throwing these responses
wait a second, the code works fine for credentials provider but its throwing this error for the google one
*agreed this is not a trpc function, but the correct http error is still raised regardless, I just use them everywhere
hmmmmmm I think then you'll have to handle it for the google provider accordingly
as the
jwt
and session
handlers catch all typesalso i dont see the access token in the response
lemme show u
first i logged the user and the token in the jwt function
i tried to log the session but its not triggerd so no session is created
so the user is being properly set into the token by the looks of it
btw remove any unnecessary info
that password hash does not belong in the token
its' a major security hole
anyhow, then what's the output for the google provider?
yes but without the access token or the token id
the error i showed ya
..
then show me the console log before setting the user in the token
in the session func?
something like this:
both?
sure
I forgot how google provider returns it so
thats the code so u can follow the logs :
and thats the output
I wanted to see the jwt one before the session one
so in the jwt function put the console logs above the
user && (token.user = user);
sensor the unnecessary info if you want to I only want to inspect the structure, not the values
AHHHH
wait
@CLOG
I just readits not returning the jwt
here
the modification is in the session function
as
token from session undefined
is the output in the case of the google providerokay i will log the output
no need
I just read the last line of this so I figured out where it's throwing
the token form the session is undefined
it was throwing on the
token.user
and token
was undefined
yes
as google is already handling setting the user object, you don't seem to need to do anything there for setting the user object in the session
just make sure each authentication method has the same information in the user objectu mean i dont need acces token and other stuff like in the credentials one?
noooooo
you don't need to set any
session = token.user
for the google provider
that's what I meant
OR
this sufficiently handles it. Though you should double check your session data is present in the frontend after a signin
to be surei see, now in credentials its not returning the session, only the jwt with the user info
so its like the oppopsite of google
for the google provider, yes
what about credentials?
in the credientals? what do you mean
again my logic applies for both cases
its returning the jwt but no session is created
if it's google : there's no token so no
session.user
updates
if it's credentials : then there's a token and session.user
is made
?that's weird, it should be working normally how it was working a few mins ago
like thisit didn't work i told u but we skipped to google, i think it was missunderstanding
this one is with which provider?
credentials
and it's not setting a jwt?
it should be working as it's a valid console log
its returning the jwt but not the session
hmmmmmmmm
you're doing something wrong with somewhere else in that case I think
to make things clear, which strategy we are using here? jwt right?
if this is your setup
yes
then things should work properly for both
as both callbacks are properly implemented
so no session should be created in the db
that's upto you as in the case of jwt, it's not required. UNLESS you want to handle sessions on serverside, for the sake of invalidating them
but making a session is to be done in the authorize function
well pls dont curse🙂
. In this example, where I have
// ... some other business logic and session management
, I put my logic for it here
?i commented it by mistake
ahh
now every things is woking fine
👏
sessions, jwts
*slow 👏
well if u dont mind
anyhow fixed now right?
GGs
can u explain the proccess
which one
like how things should be done
cuz i feel i made a spaghetty code here nd im just following without understanding how it should be done in the right way
here's the flow in the case of next auth's functions, give me a minute
i think if im going touse only google i'll only setup the adapter and let it work by it self
sure
here's how it works in a nutshell according to my mind and what I've read
if you still don't understand, then ask specifically. Unless you want me to draw out some sort of flow diagram, which I'll do later
hey i think there's a problem in the solution
?
basically i have protected routes by the middleware but when i log in the middlware still require me to re-log
eh?
yes
is the middleware the same/
the default and the matchers
or did you put a custom one in?
nope, the same matchers
its just acting like your not logged in
but actually u have the session in the cookies
uuhuh
is it doing for both providers or just 1?
both
also, it's just redirecting you to the page correct?
u log in (either google, or the other guy) , redirects u to the home page (i defined the callback like that), if there is a session some new navlinks will appear in the navbar, if u click on one of them u'll get redirected to sign in page
so clicking on either of the links redirects to signin page?
except the unprotected ones
and you have not setup any sort of logic in those pages per say right? Other than the middleware?
no user based role, no extra layers only raw pages with the matcher
that's weird
after signing in, have you verified your session is present in the cookies?
and also in the db? (if you're using db auth)

we are using jwt
I see ok
wait, im not using the sign in callback in the options there
i only set the jwt nd session callbacks with you
about which part are you talking about?
this error is weird as I can't pinpoint it.
Send me a jwt as an example I want to inspect it's exp and iat
okay i will log the jwt from jwt callback
or
copy from the cookies tab
easier that way
no
like the signed jwt
or leave it
the hash?
I got the iat
and the exp
ahhhhh
your expiry is not set
I figured
i need to set the age
?
yes
the max age

in my case the SESSION_TTL is
3600 * 6
which is 6 hoursi'll check this
nothing changed
still blocking me
hmmmmmmmmm
when you're redirected, are the cookies present?
redirected to signin is what I meant
yep cookies are still there
hmmmm then I honestly don't know other it being the middleware, try removing it and seeing if you see the content properly. if you do, then there's something you're missing. Check the next-auth docs in that case
i'll do a final check
i did this and i guess its working :
nope its not
that's because you're returning
true
in any casesilly me
it's alright, play around with your code, and test it out. You'll find it eventually. Otherwise leave me a message, I'll check it out tomorrow 👋
or someone who's been using next-auth more than me, is more familiar with it might know
and answer you
sure have a great day.
i guess i found the issue but i don't know how to fix it, when i log in it redirects me to the home page but in the client side its not rendering the protected links in the first render until i navigate to another page, here's the client side code :
i have more than that /play route.
you can use a trpc function instead of useSession to mitigate that
im not using trpc in this project. is there any other solutions?
something like
then use getserverside props
to get the session from the cookie and pass it as a prop to the page
so no middleware?
oh wait it's next 13 right?
yes the app dir
then you can just use the server component to get the cookie from the
cookies().get()
it's very easy that way, no getserverside props, I was thinking in the old pages dir style
as the cookie is present, you don't have to do a specific fetch on the client side with useSession
the confusion is that it was working fine before
hmm I get that, but if you can't fix it. My solution still stands as valid 🤷♂️
the issue you're encountering is specific to your code currently, I've never encountered it in such a way before
well i can send u the repo if u don't mind
where i can find that in the docs?
I feel you pain nextauth too just stopped working for me as expected after a while
After much front and back i had to implement my own auth from scratch
I could, but I don't have enough time to gather enough context , test out the issue and help fix it. Sorry :/
It was more easier and straightforward
Just be sure you don't leave a gaping hole in your security. Making your own auth is easy, but also easy to shoot yourself in the foot
no worries at all
Lol even next auth has its own gaps
for example?
I have forgotten by it was a topic of discussion here at some point where cj also talked a bit on it
regarding what topic about auth?
I can't remember