React remove item from cart
once i open the cart the product is deleted and this error appears
this is the customhook i have
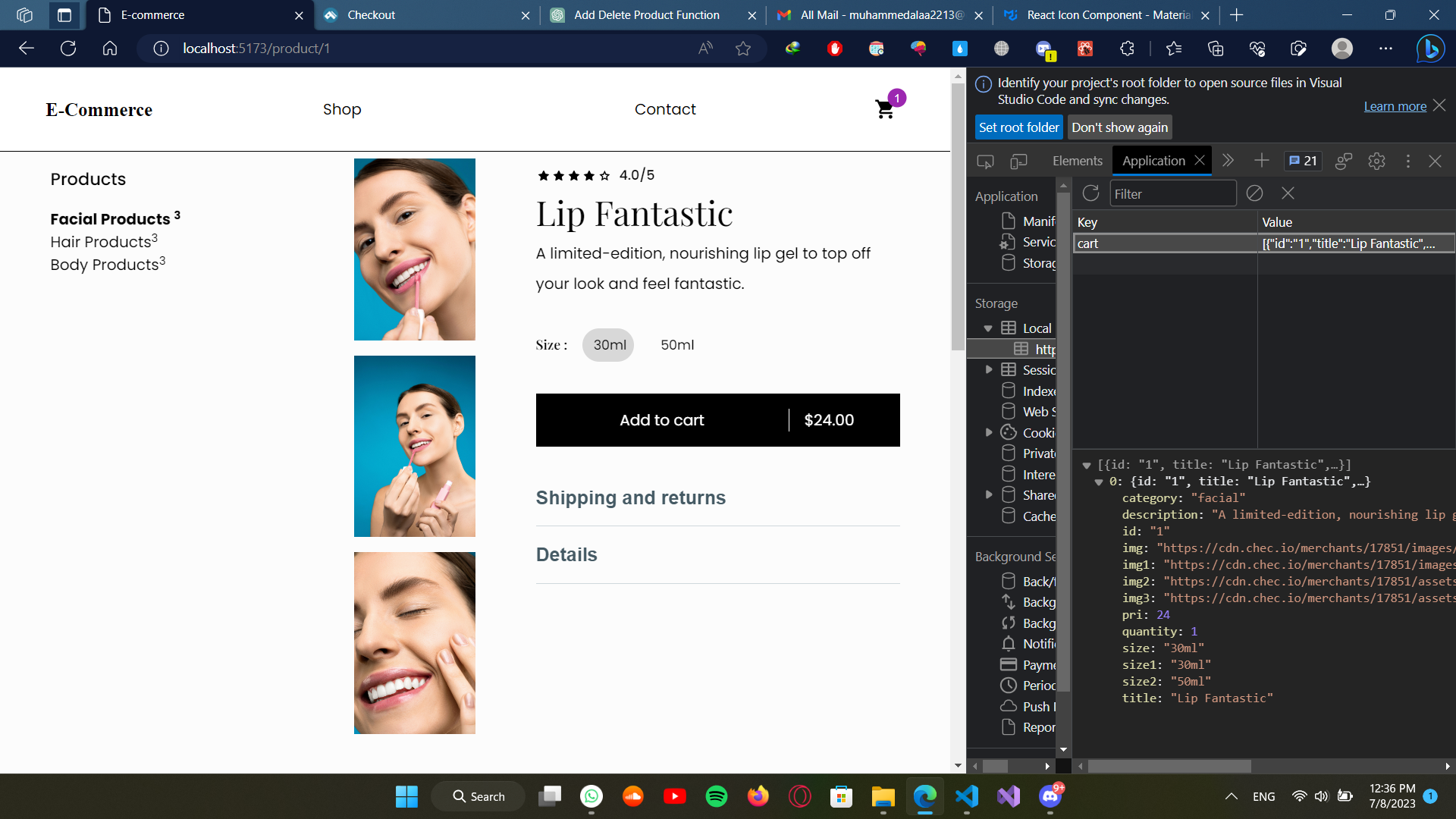
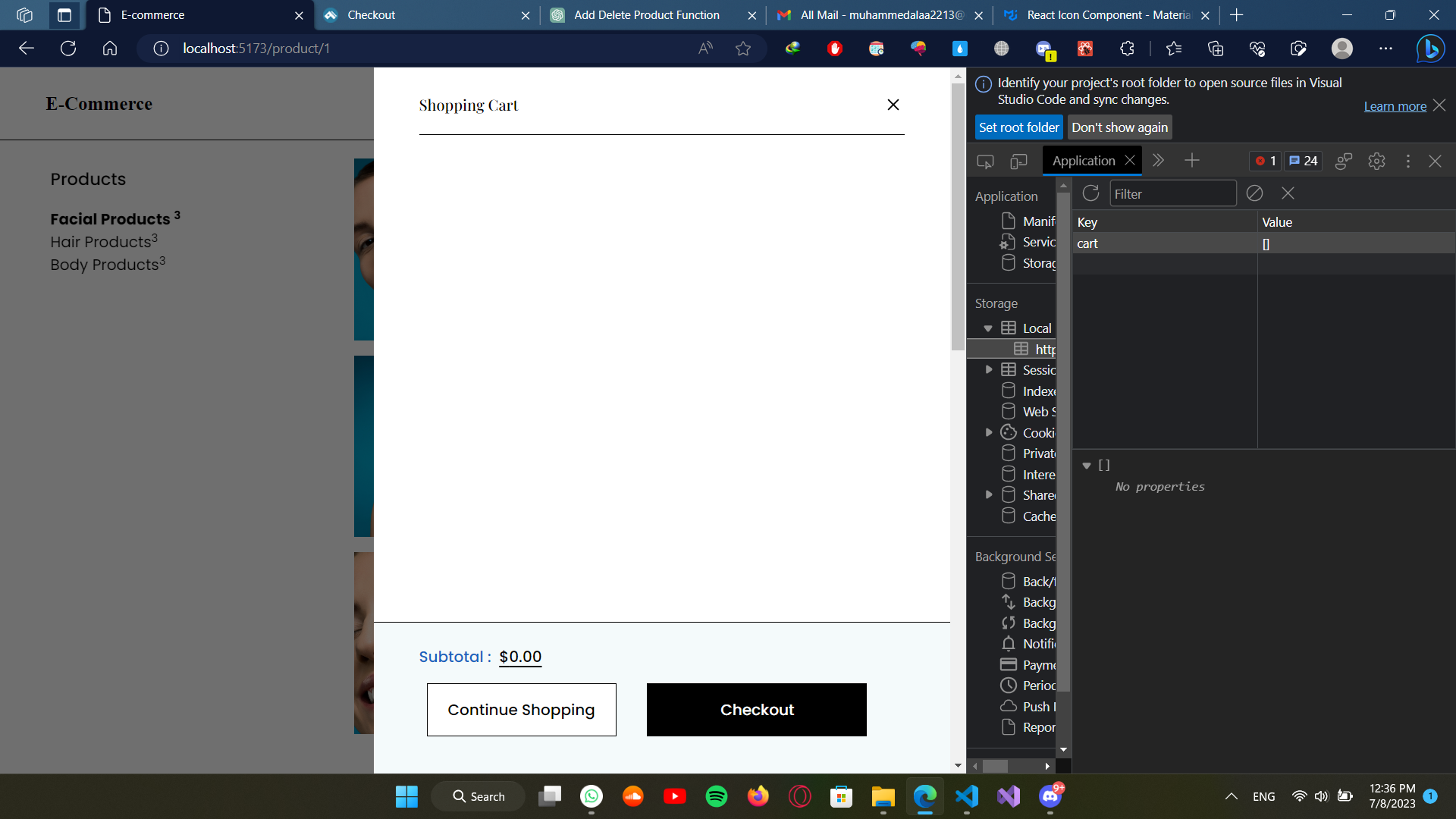
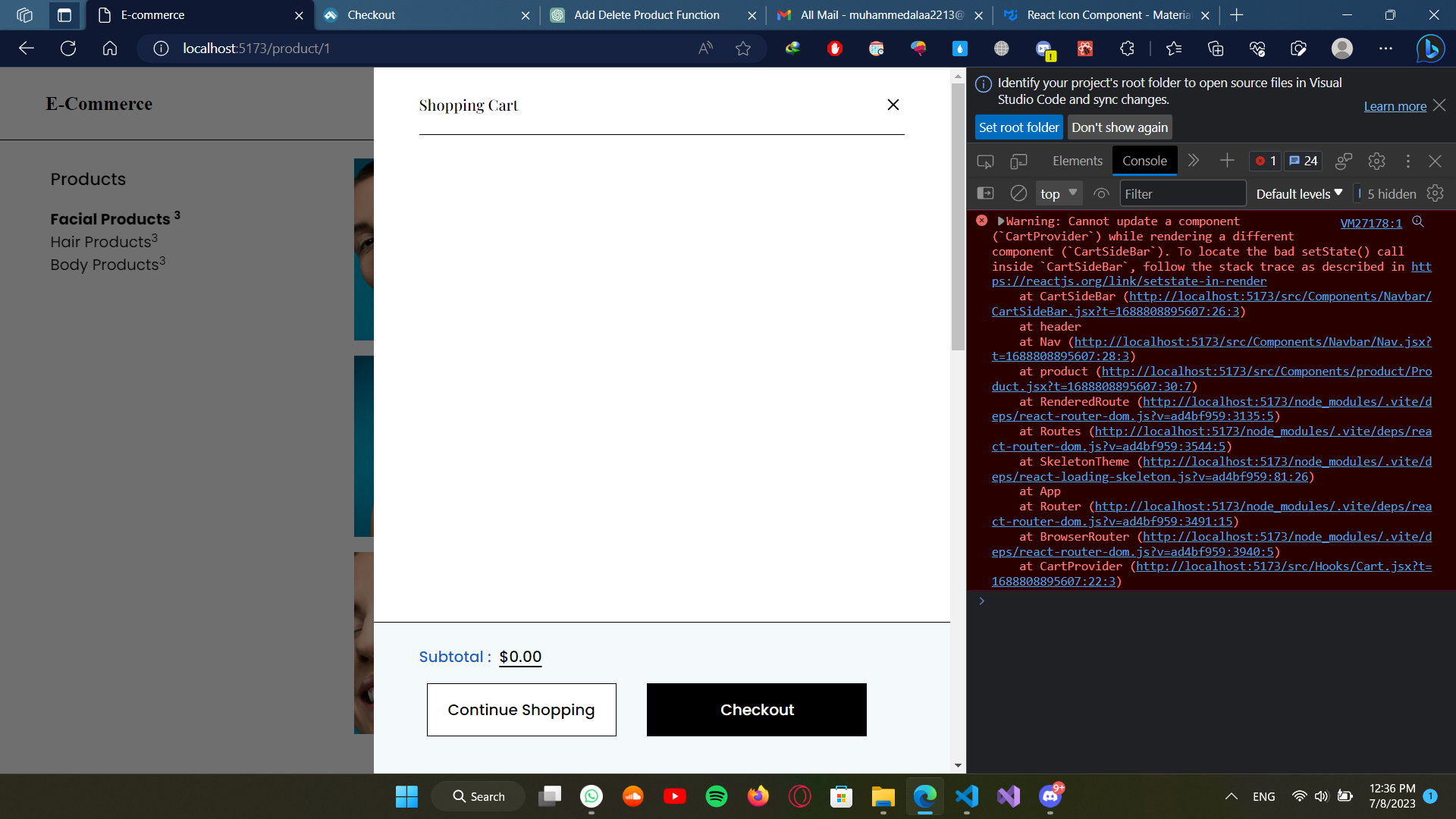
5 Replies
this is in the cartsidebar component
this is the cart side bar when i comment the onClick function on the delete icon
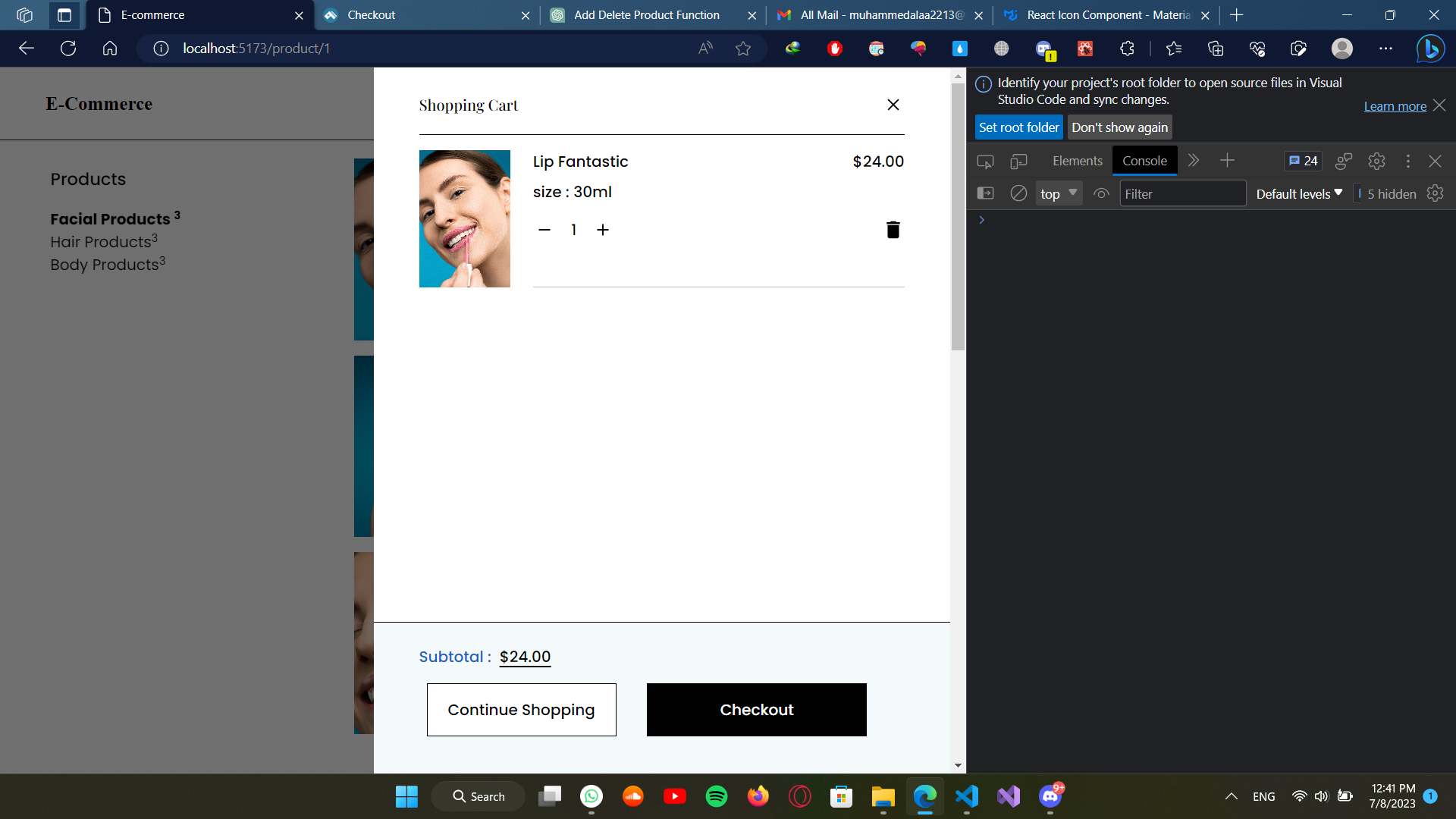
CodeSandbox
CodeSandbox is an online editor tailored for web applications.