Pagination problem
Hello, I have a problem with pagination. I'm working on some stock management commands, and here I would like to display the entire stock after the command.
The problem is that the embed is too large, so I thought about using pagination and only displaying 5 "data" on each embed page.
However, I have a problem because my code is not functional.
Regarding the error, my stock is displayed correctly, but only the first page. As soon as I try to navigate to another page, my bot doesn't crash and continues to function, but I get an "interaction failed" message.
Thank you.
Copy code
const Discord = require("discord.js");
module.exports = {
name: "pagination",
description: "Check the stock",
permission: Discord.PermissionFlagsBits.Administrator,
dm: false,
category: ":beginner:・Stockage",
options: [],
async run(bot, message, args, db) {
db.query("SELECT * FROM stock WHERE afficher = 1", async (err, results) => {
if (err) {
console.error("Error retrieving data:", err);
await message.reply("An error occurred while retrieving data.");
return;
}
// pagination embed
let embeds = [];
for (let i = 0; i < results.length; i += 5) {
let embed = new Discord.MessageEmbed()
.setColor("Blue")
.setTitle(`Stock - Page ${Math.floor(i / 5) + 1}`)
.setDescription(`Number of accounts on this page: **${Math.min(results.length - i, 5)}**`)
.setTimestamp()
.setFooter({
text: `${bot.user.username} - Page ${Math.floor(i / 5) + 1}`,
iconURL: bot.user.displayAvatarURL({ dynamic: true }),
});
for (let j = i; j < Math.min(i + 5, results.length); j++) {
embed.addFields({
name: `Account #${results[j].id}`,
value: `<:mail:1126445411683541103> Mail: \`${results[j].mail}\`
:password: Mail password: ||${results[j].mdpmail}||
:leagueoflegends: LOL Name: \`${results[j].nomlol}\`
:password: LOL password: ||${results[j].mdplol}||`,
});
}
embeds.push(embed);
}
// create buttons
let leftButton = new Discord.MessageButton().setLabel("Previous").setCustomId("previous").setStyle("SECONDARY");
let rightButton = new Discord.MessageButton().setLabel("Next").setCustomId("next").setStyle("SECONDARY");
// action row for buttons
let actionRow = new Discord.MessageActionRow().addComponents(leftButton, rightButton);
// send first embed
let msg = await message.reply({ embeds: [embeds[0]], components: [actionRow] });
// collector for 60 seconds
const collector = msg.createMessageComponentCollector({ componentType: 'BUTTON', time: 60000 });
let currentIndex = 0;
collector.on('collect', async (button) => {
await button.deferUpdate();
if (button.customId === 'next') {
currentIndex = currentIndex < embeds.length - 1 ? currentIndex + 1 : 0;
} else if (button.customId === 'previous') {
currentIndex = currentIndex > 0 ? currentIndex - 1 : embeds.length - 1;
}
await button.message.edit({ embeds: [embeds[currentIndex]], components: [actionRow] });
});
collector.on('end', collected => console.log(`Collected ${collected.size} interactions.`));
});
}}
Copy code
const Discord = require("discord.js");
module.exports = {
name: "pagination",
description: "Check the stock",
permission: Discord.PermissionFlagsBits.Administrator,
dm: false,
category: ":beginner:・Stockage",
options: [],
async run(bot, message, args, db) {
db.query("SELECT * FROM stock WHERE afficher = 1", async (err, results) => {
if (err) {
console.error("Error retrieving data:", err);
await message.reply("An error occurred while retrieving data.");
return;
}
// pagination embed
let embeds = [];
for (let i = 0; i < results.length; i += 5) {
let embed = new Discord.MessageEmbed()
.setColor("Blue")
.setTitle(`Stock - Page ${Math.floor(i / 5) + 1}`)
.setDescription(`Number of accounts on this page: **${Math.min(results.length - i, 5)}**`)
.setTimestamp()
.setFooter({
text: `${bot.user.username} - Page ${Math.floor(i / 5) + 1}`,
iconURL: bot.user.displayAvatarURL({ dynamic: true }),
});
for (let j = i; j < Math.min(i + 5, results.length); j++) {
embed.addFields({
name: `Account #${results[j].id}`,
value: `<:mail:1126445411683541103> Mail: \`${results[j].mail}\`
:password: Mail password: ||${results[j].mdpmail}||
:leagueoflegends: LOL Name: \`${results[j].nomlol}\`
:password: LOL password: ||${results[j].mdplol}||`,
});
}
embeds.push(embed);
}
// create buttons
let leftButton = new Discord.MessageButton().setLabel("Previous").setCustomId("previous").setStyle("SECONDARY");
let rightButton = new Discord.MessageButton().setLabel("Next").setCustomId("next").setStyle("SECONDARY");
// action row for buttons
let actionRow = new Discord.MessageActionRow().addComponents(leftButton, rightButton);
// send first embed
let msg = await message.reply({ embeds: [embeds[0]], components: [actionRow] });
// collector for 60 seconds
const collector = msg.createMessageComponentCollector({ componentType: 'BUTTON', time: 60000 });
let currentIndex = 0;
collector.on('collect', async (button) => {
await button.deferUpdate();
if (button.customId === 'next') {
currentIndex = currentIndex < embeds.length - 1 ? currentIndex + 1 : 0;
} else if (button.customId === 'previous') {
currentIndex = currentIndex > 0 ? currentIndex - 1 : embeds.length - 1;
}
await button.message.edit({ embeds: [embeds[currentIndex]], components: [actionRow] });
});
collector.on('end', collected => console.log(`Collected ${collected.size} interactions.`));
});
}}
5 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.(sorry i missclicked the version)
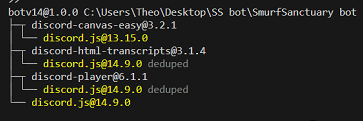
Node version : v18.15.0
for (let i = 0; i < results.length; i += 5) {
let embed = new Discord.MessageEmbed()
.setColor("Blue")
.setTitle(`Stock - Page ${Math.floor(i / 5) + 1}`)
.setDescription(`Number of accounts on this page: **${Math.min(results.length - i, 5)}**`)
.setTimestamp()
.setFooter({
text: `${bot.user.username} - Page ${Math.floor(i / 5) + 1}`,
iconURL: bot.user.displayAvatarURL({ dynamic: true }),
});
for (let j = i; j < Math.min(i + 5, results.length); j++) {
embed.addFields({
name: `Account #${results[j].id}`,
value: `:mail: Mail: \`${results[j].mail}\`
:password: Mail password: ||${results[j].mdpmail}||
:leagueoflegends: LOL Name: \`${results[j].nomlol}\`
:password: LOL password: ||${results[j].mdplol}||`,
});
}
embeds.push(embed);
}
for (let i = 0; i < results.length; i += 5) {
let embed = new Discord.MessageEmbed()
.setColor("Blue")
.setTitle(`Stock - Page ${Math.floor(i / 5) + 1}`)
.setDescription(`Number of accounts on this page: **${Math.min(results.length - i, 5)}**`)
.setTimestamp()
.setFooter({
text: `${bot.user.username} - Page ${Math.floor(i / 5) + 1}`,
iconURL: bot.user.displayAvatarURL({ dynamic: true }),
});
for (let j = i; j < Math.min(i + 5, results.length); j++) {
embed.addFields({
name: `Account #${results[j].id}`,
value: `:mail: Mail: \`${results[j].mail}\`
:password: Mail password: ||${results[j].mdpmail}||
:leagueoflegends: LOL Name: \`${results[j].nomlol}\`
:password: LOL password: ||${results[j].mdplol}||`,
});
}
embeds.push(embed);
}
Found the sollution, thanks 🙂
How can i close that channel?