✅ Capturing process output
https://learn.microsoft.com/en-us/dotnet/api/system.diagnostics.process.outputdatareceived?view=net-7.0#examples
I am using the following example to try and capture a process output and print it to a textbox at runtime. The process output is captured, but it is only printed after the process has terminated.
how do I make sure to print at runtime?
Process.OutputDataReceived Event (System.Diagnostics)
Occurs each time an application writes a line to its redirected StandardOutput stream.
45 Replies
process.WaitForExit()
does what it sayswhat is that suppose to mean?
i just tried not waiting for exit and i get no output at all
well sure
you have to have both running kind of in parallel
if you wait for the other process to exit, then your code will never read
tboxConsole.AppendText
before the process has exited
but if you don't wait, then your code will reach tboxConsole.AppendText
before any output has occurredi also tried capturing the process on a separate thread, but no luck.
what would you suggest?
i would probably just change the textbox contents in the
DataReceivedEventHandler
lol i tried that too
and?
no out put at all
not even when the process has terminated
is this what you mean?
something like that yeah
well that didnt work unfortunatly :C
what kind of applicationis this?
winforms?
if this is winforms, you probably need to call
Refresh
on the control to update it with the output:
https://learn.microsoft.com/en-us/dotnet/api/system.windows.forms.control.refresh?view=windowsdesktop-7.0Control.Refresh Method (System.Windows.Forms)
Forces the control to invalidate its client area and immediately redraw itself and any child controls.
but you should also be multithreading this
for example, try adding
i tried having the refresh run on a separate thread but no luck..
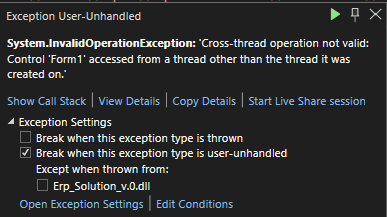
this is what i tried:
it sounds like it should be working then based on your comments. I don't think I can help without being able to replicate your issue. If you can share your code so we can test it for ourselves please do
I am pushing to github atm,
https://github.com/karim-daman/Replication_Test.git
really appretiate your attempt to help.
NOTE: adjust the exe path so that the form can reference it.
GitHub
GitHub - karim-daman/Replication_Test
Contribute to karim-daman/Replication_Test development by creating an account on GitHub.
you gotta be trippin if you think anyone's just gonna launch this exe
ur not suppose to be launching it lol
i mean u can
but the form would launch it when i hit start
it's a published .netcore web api as an exe
you can use a vm if ur sus
i mean you have to start it to do your replication
no u dont
u run the form, then click start, and the form would run the exe as a separate process
...which runs the exe
which is what i'm saying
i was clear about this since day one lol
anyway it works fine
what? u see output?
at run time?
yeah
wtf
no way
well no, i made changes
show me!
cause your code still didn't make sense
i dont see any output on my side..
show your code now
just push to the repo maybe
good one
i guess the using statement actually breaks it. makes sense
OMGOSH BRO
UR A GENIOUS!
@just_ero THANKS!!!
make sure to kill the process when your app shuts down
is there a more graceful way of shutting down the process?
don't think you need one
@ZP ░▒▓█├■̶˾̶͞■┤█▓▒░ Thanks Zach!
maybe clear the textbox when the api process is stopped
make the textbox readonly
and your info groupbox doesn't scale
i am going to look into that , i noiticed it (idk how to fix that)
also, Gutter Country is pretty dope
It's amazing
so raw!
I didn't create it if you think that
Also $close
Use the
/close
command to mark a forum thread as answered