✅ Serializing XML with diacritics/accents
Hi, I'm trying to serialize a class to xml that contains some strings, some of which may contain an accent (example:
genérica
) or other latin characters (example: diseño
). Currently I'm serializing it using the following code:
(I was previously trying to use a StringWriter but it also doesn't work)
It ends up replacing the characters with ??
instead of writing the actual characters.
The final XML needs to be formatted to UTF8 (and include encoding="utf-8"
at the top of the xml), so I'm not able to use iso-8859-1 (but even when I tried to use it as an encoding it replaced characters with "??")15 Replies
my guess is the default encoding for XmlWriter isn't any form of unicode
XmlWriterSettings.Encoding Property (System.Xml)
Gets or sets the type of text encoding to use.
hm, but it's UTF8 by default in .NET 7
what runtime version are you on?
net 7
what program are you using to view the output XML?
I'm currently printing it to console, but I initially checked it on a browser
I'm also checking the byte array and it shows 2 '?' characters
hmm
lemme try that code on my end
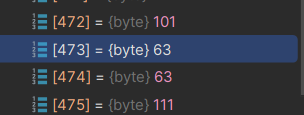
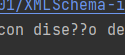
hm, it works here
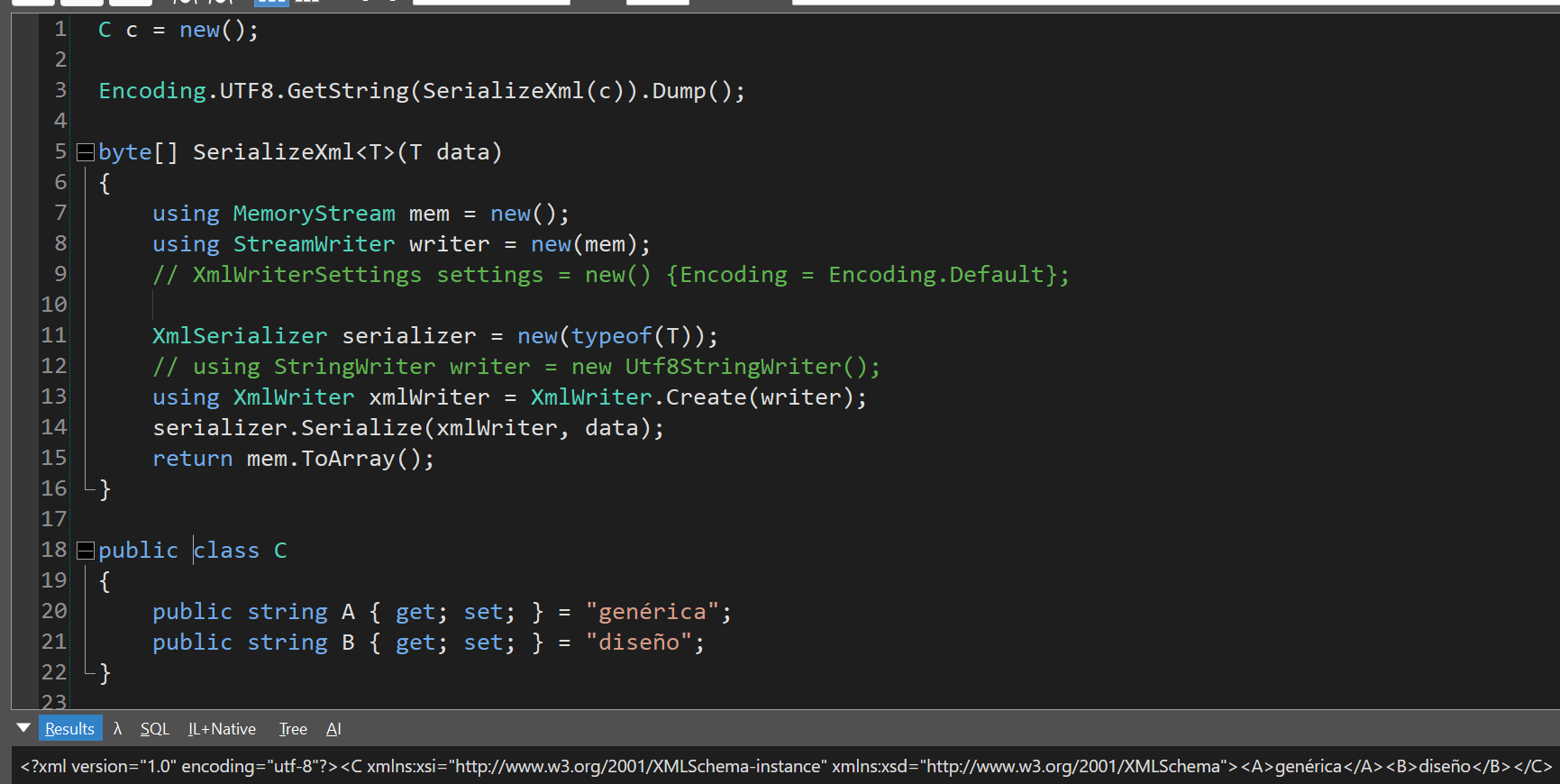
also .NET 7
what if you try explicitly specifying the Encoding as UTF8 on the writer settings?
like this?
it still doesn't work
have you tried printing out the values in the object you're serializing
maybe they're already messed up?
I tried setting it to a test value (
product.Description = "genérico diseño tést";
) but it's still replacing it
hm
can you produce a minimal example?
I discovered the issue. I have a
Request<T>
class to surround anything with <Request><T></T></Request>
(where T
is the class name of the generic T
) and it was done like this
It serialized the data so that the element name changes with the class name, but I made it use ascii instead of utf8, causing characters to be replaced with ?
. It's fixed now by changing Encoding.ASCII
to Encoding.UTF8
, although I should probably check if there's a better/correct way to do this
Thanks for the help