❔ Interactive both in y and x axis, in cmd
Hello :), I am trying to do an interactive menu in a command line application that use both the x and y axis, you navigate using keyboard arrows. I did a well working one that is only using the x axis, but with both this is way more complicated. I wrote a code that kind of works but for any reason the Console.Clear is not doing what i was expecting : Editing is the main code that run the menu and handle the user input
19 Replies
CustomCMD.GetValue() is used to get the correct string, depends of the language (this is not the actual problem so i won't explain much)
this is what is display without any input (and this is what i want) :
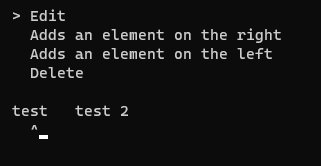
But once I do any input, the selected item is the good one, but the console is acting wierd :
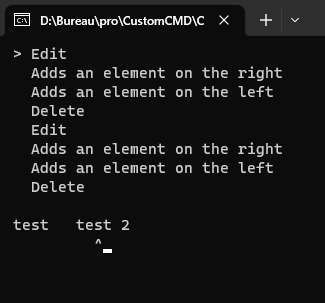
It duplicates the menu 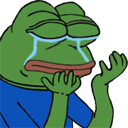
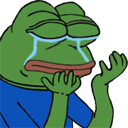
i didnt see where you clear the screen
in the begining of WriteComplexMenu which is called right after defining every option in the while loop
but every time you loop, dont you add more options? so on the second time you have 2x each option?
$debug is your friend
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
i can't debug because of the way i'm compiling
I need to read json files, that are in the CustomCMD folder, but the compiled exe goes to CustomCMD/Debug/net7.0
this is this 🥲
it has been 2 hours while I was reading everything about buffer, Console.Clear thing etc ... x)
add a sleep to your program. while it is sleeping, atach to the running process
wdym by atach?
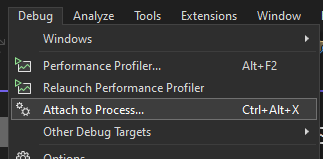
you can connect a debugger to a running process
nice, is there a way to do it automatically right after the build ?
right now it's moving the exe and other file automatically from net7.0 to the right folder usig a .bat script
you'd be better off making the location that json files are read-from a command line argument ( and dont use a bat script to move the exe to a 'special' location)
you can control the commandline args given when doing normal 'debug' from the project properties
or, load the json as
../../my_file.json
instead of just my_file.json
in your code
you could also include the json in the build and set it to be copied to the output directory (not sure what the exact steps are, but I believe I've used the correct terminology)I'll check that
But I've never find anything like that
I think it's usually right click on the file in Visual Studio
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.