β Access button from another script
So im trying to make a settings window for my app and i want a check box so when you click it 2 buttons on the main window become visible but i dont know how to access thos buttons from the settings script
98 Replies
we need more info. What kind of code base are we dealing with here? Winforms? WPF? Avalonia? Unity? etc.
but regardless, you generally have one class per file if you are following C# conventions. And if you want to access members f another class, you just need an instance/reference of that class and you need the members you need to access to be
public
.sorry forgot to add what i was using im using winforms
accessing code members from another file in winforms is no different from having multiple files in a console application or any C# project type. you just need an instance/reference of the object to edit and the member must be public.
the easiest way for us to help would be for you to share your code. if you can share your code please do
Main Form
Settings Form
You have quite a few problems with your code. but I'll focus on the problem you asked about in this thread.
If you want to edit your main form from the settings form you need a reference to the main form. For example you add this...
to your settings form. then you would have a constructor that would take in a reference to your main form. and in your main form change
to
then if you want to change anything in the main from from your settings form, you would just
MainForm.WhatYouWantToChange = WhatToChangeItTo;
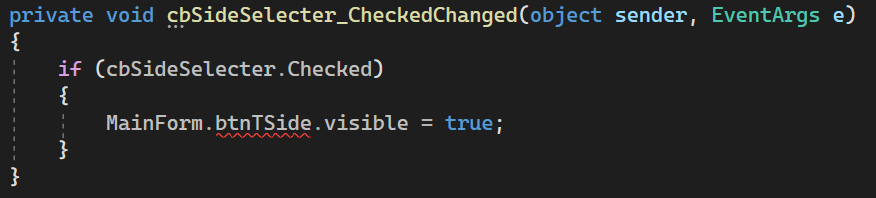
read the compiler errors
they will help you

and what do you think that error means?
idk how to change the protection level of a property...
what is happening is that
btnTSide
is defined as a private
field
and you cannot access private fields from other classes
cause that is how private worksi know that
so you need to expose it. I would not recommend editing the
Xxx.designer.cs
file, which is where the button is defined
instead...
I would make a public member to expose what you need to be public
for example you could add public Button ButtonTSide => btTSide;
to your code for the main form
then you have a public property that exposes the private button
and you could do MainForm.ButtonTSide.Visible = true;
instead of trying to access btnTSide
in your settings form
give it a shotSo theres no errors but it dose nothing when i check the box
put a breakpoint
Im still very new to C# so idk what that is yet
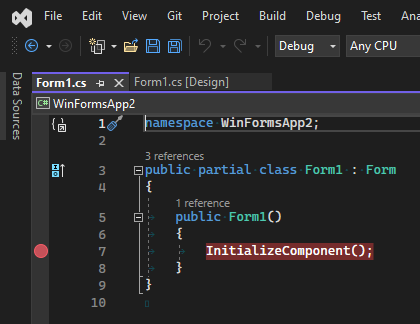
you click where that red dot it in my screencap
that will add what is called a "breakpoint"
when you debug your app in visuals studio by clicking the green start triangle at the top and your code reaches that breakpoint, it will stop executing your code at the breakpoint
so you can look at what your code is doing
another option is to start your code by pressing F10 or F11
those are the "Step Over" and "Step Into" hotkeys
they let you walk through your code line by line
and watch what it does
learning how to debug code is a vital skill for a software developer
so the sooner you get started learning how to debug, the better π
it will help you improve
here is a guide that you should review if you struggle with debugging:
https://learn.microsoft.com/en-us/visualstudio/get-started/csharp/tutorial-debugger?toc=%2Fvisualstudio%2Fdebugger%2Ftoc.json&view=vs-2022
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
the reason I'm encouraging you to debug your code is because it is likely your code isn't reaching your
MainForm.ButtonTSide.Visible = true;
line
you need to figure out why your code never reaches that line
I can't really help you because I don't have your full code in order to run it myself to see what it is doing
if you can share the entire code via a github repo then we could tell you exactly what is happening πim uploading the files now
is the obj file needed?
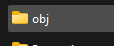
@ZP ββββββ ΜΆΛΎΜΆΝβ β€ββββ
no. you should have a ".gitignore" file that will exclude the bin and obj folders. had to step away from computer but will send screenshot when back explaining
GitHub
GitHub - PhoenixXeme/temp
Contribute to PhoenixXeme/temp development by creating an account on GitHub.
awesome. will give it a look once back at my pc in a few minutes
when i get to the check box
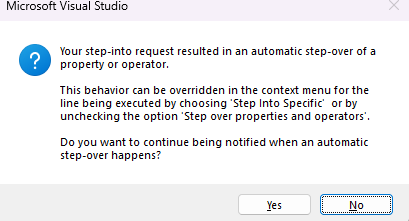
I would select to "no" and just keep going (ignore that)
so, based on the current code in your github repo, the "T" button is always visible
you want the checkbox to toggle it right?
if that is the case, I would recommend changing your settings form to:
then, when you construct your settings form, the checkbox will default to the current state of the button being visible or not, and when you check the checkbox, the button's visibility will toggle with the checkbox being toggled
and now i need it for CT button
XD
Is the "T" button working like you expect?
yea all works fine
but why is it so annoying to access it π
I'm not sure what you mean by "annoying to access it"
i mean why do you have to do all that why cant it be more simple
anyway
i just need to do one more thing
check if the check box from settings is checked
in form1 π¦
well keep in mind this is how you have decided to design the app. There are other ways to handle settings they might make it easier to handle. but don't worry about that right now. focus on finishing your app. you can always change it in the future if you end u wanting to
as for the "CT" button, are you wanting the same checkbox to toggle the visibility of both the "T" and "CT" buttons? or do you want another checkbox to handle the "CT" button?
no it all works when i uncheck it


the reson i want this is because i want to log the side but if the box is uncheck i want it to log nothing
sorry im not following what you the app to do. can you elaborate and/or rephrase? What is not working in your app currently?
when the box is unchecked i dont want it to write "T" or "CT"
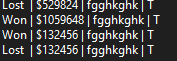
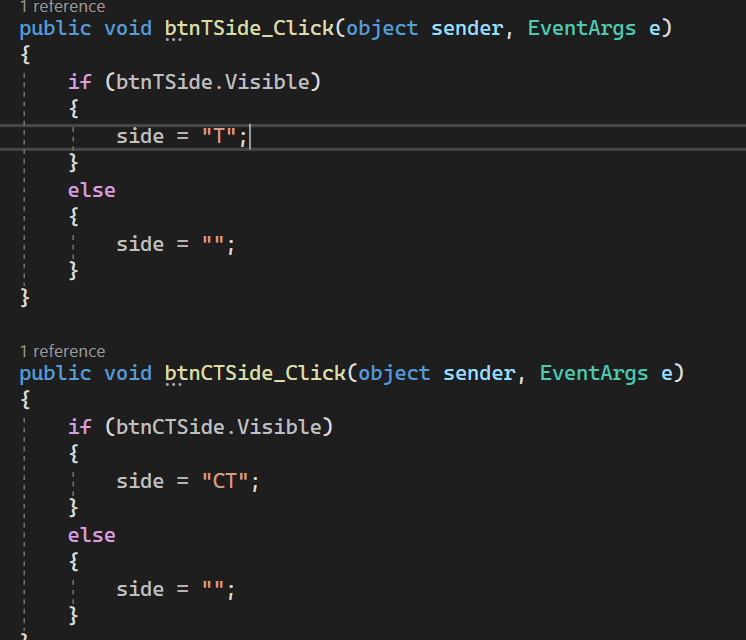
can you push the latest code to your github?
you have made changes on your PC that we cant see yet at your github repo
can we call and i screen share
?
I'm a little busy for a screen share at the moment (I'm technically still workign my day job currently π ) but I may have time in like 4 hours π
so no sorry not now
but if you update your code on Github we can probably assist still
GitHub
GitHub - PhoenixXeme/temp
Contribute to PhoenixXeme/temp development by creating an account on GitHub.
are you struggling to use github? when you make a repo you want to add a
.gitignore
. that will help you out a lot: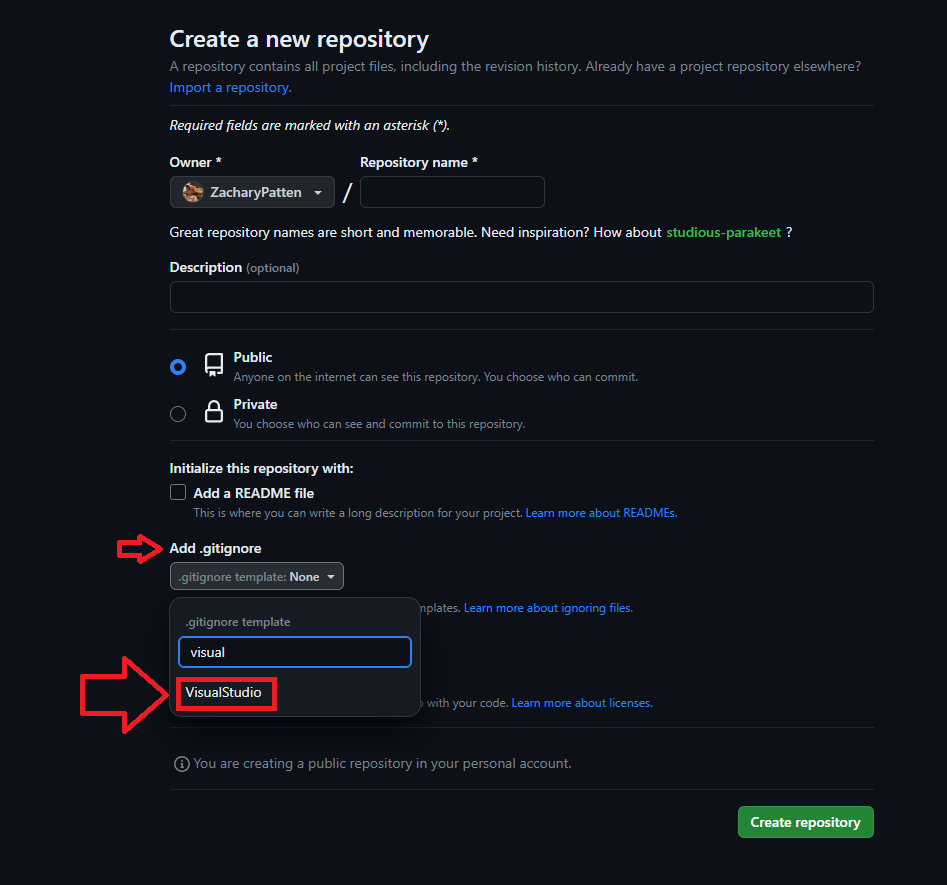
then you won't have to update individual files
ahhhh
still new to github XD
yeah you were making your life 1000x harder
π
bruh
and then what files do i upload?
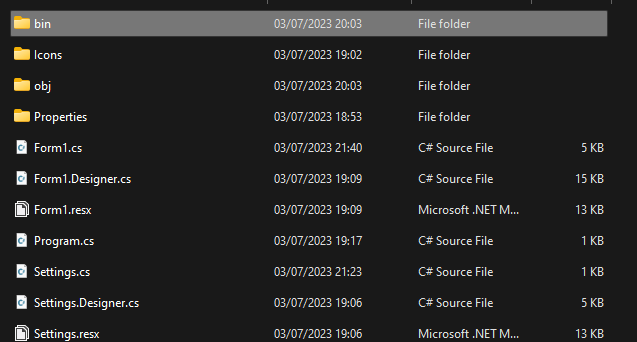
I have to go offline for a while now (getting busy with work)... but I will try to help out later... but there are plenty of people on this server besides me who can help you too
if you have a good
.gitignore
file then you can just push everything that is not ignored. The "Visual Studio" .gitignore
file on github will exclude everything that you don't need in your repo for C# applications. If you eventually try to learn other programming languages like Rust for example, then you would likely want a different .gitignore
specifically for Rust (not C#)are you dragging and dropping files to github?
yea
these are the files i drag and drop
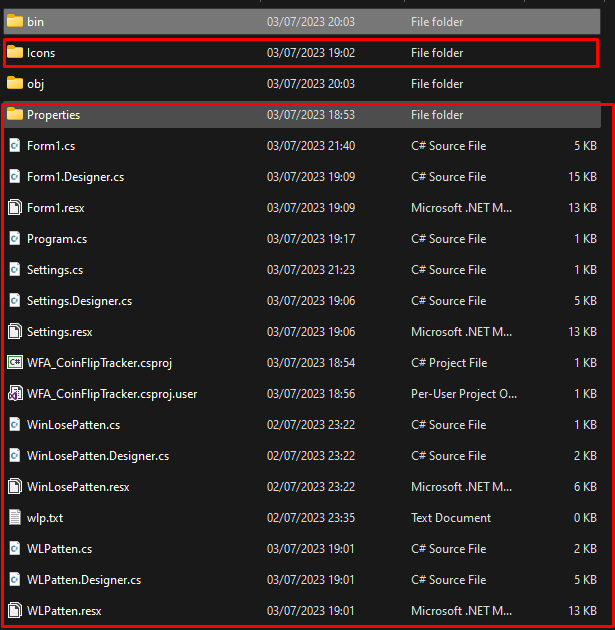
if you're using visual studio, it has a built in git control
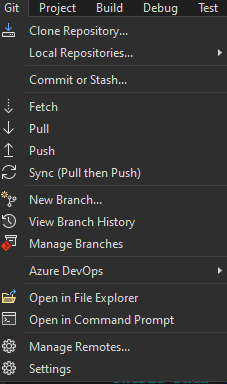
hmmmmmm
so you can push and pull without having to do it manually
ok
i didnt know that
dont need to learn the syntax either
recommended, but baby steps
wonder if there is a git tag $git
Git commands Cheat Sheet
Initialize a new qit repository:
git init
Set configuration values for your username and email:
git config --global user.name <your-name>
git config --global user.email <your-email>
Clone a repository:
git clone <repository-url>
Add a file to the staging area:
git add <file>
Add all files changes to the staging area:
git add .
Check the unstaged changes:
git diff
Commit the staged changes:
git commit -m "Message"
Reset staging area to the last commit:
git reset
Check the state of the working directory and the staging area:
git status
Remove a file from the index and working directory:
git rm βΉfile>
List the commit history:
git log
Check the metadata and content changes of the commit:
git show <commit-hash>
Lists all local branches:
git branch
Create a new branch:
git switch -c <branch-name>
git branch <branch-name>
Rename the current branch:
git branch -m <new-branch-name>
Delete a branch:
git branch -d <branch-name>
Switch to another branch:
git switch <branch-name>
git checkout <branch-name>
Merge specified branch into the current branch:
git merge <branch-name>
Create a new connection to a remote repository:
git remote add <name> <repository-url>
Push the committed changes to a remote repository:
git push <remote> <branch>
Download the content from a remote repository:
git pull <remote>
Cleanup unnecessary files and optimize the local repository:
git gc
Temporarily remove uncommitted changes and
save them for later use:
git stash
Reapply previously stashed changes
git stash apply
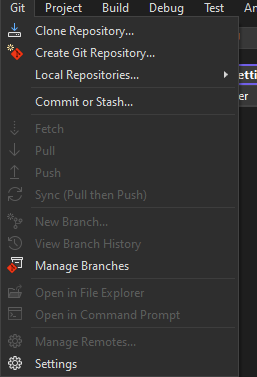
yeah so you wanna connect that to your current repo
so rightclick on your solution
and find the git
maybe itll be easier to create a new repo

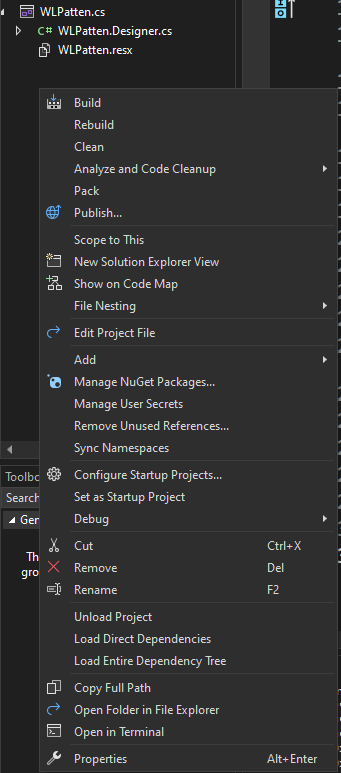
maybe try the project
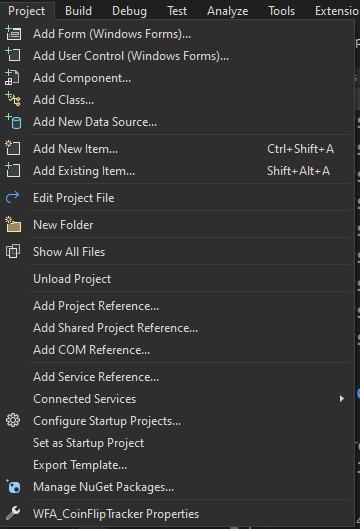
weird
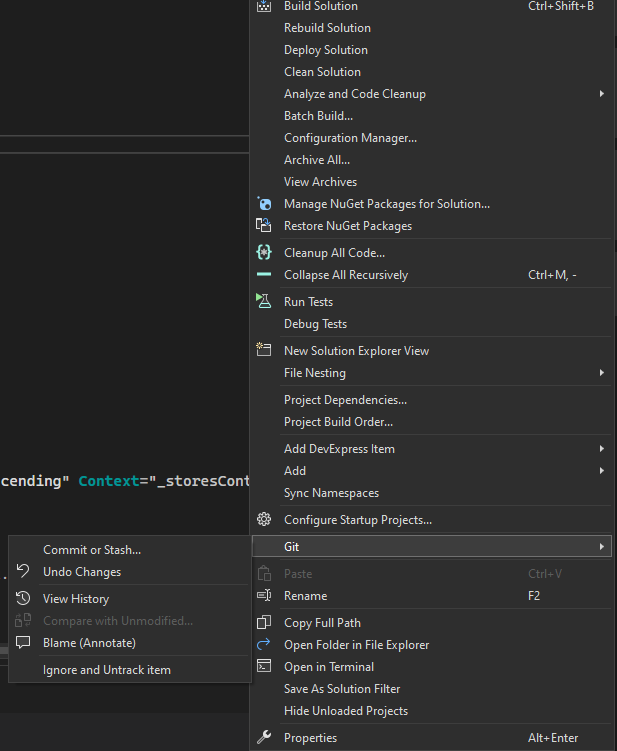
click create git repo here
i connected my account
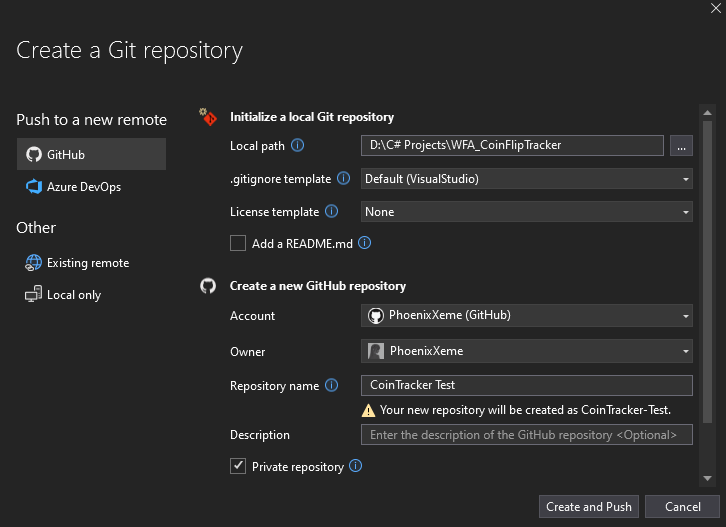
nice
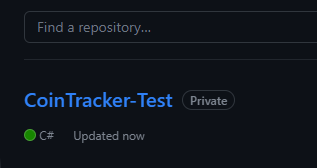
yeah make it a public repo so others can see it, the create and push
then you can now go into git changes and pull/push easier
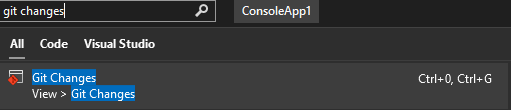
i deleted the repos on github but i still have this
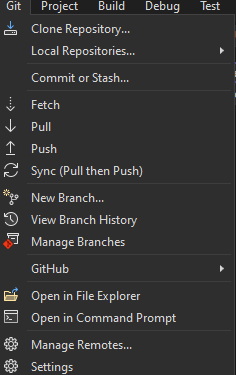
that should be fine?
now the flow should be every time you make a change, commit all + push on the master branch
ideally you would create a branch off of master then do a pull request for that but this is fine
no the repos is deleted so i cant
oh, did you need to make the repo public? you couldve done that on github
but how do i fix it
go into manage remotes and try manually removing the origins
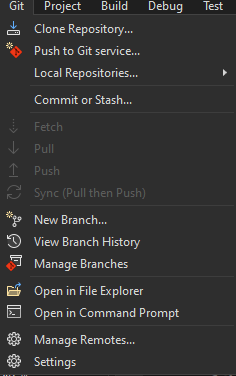
ok
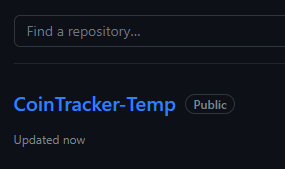
so now what dose push and pull and fetch do?
and new branch
do you see this on the bottom right? master is your current branch

git is a vcs, you can probably do more research on that
but basically you have a local version of master, and then there is the online version of master

you can do whatever you want in the local version of master, as long as you dont commit and push it, it wont affect the online version of master
ok
when you push your local version of master to online version of master, it will now become master
then when someone else pulls that version of master, they will now have your version of master
ok i get that
so what dose pull do?
pull just grabs the online version of master and merges it with the local code
the local version of master
so it just downloads online master and loads it?
it merges it but yeah
wdym "merge"
its kinda how merge conflicts happen. So user A works on file 1. User A pushes code to master. User B was also working on file 1. User B pulls from master. There is a merge conflict now because git doesnt know which is correct
im just not gonna touch pull then
what dose fetch do?
it just checks if there is a newer version of master avaible to pull
in your case you wont need to touch fetch or pull yet, but its good info if you ever do need it 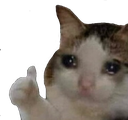
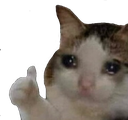
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.