❔ EF core querying issue
hello there i got a question around modeling... in short:
i have a Product model and a ProductRatings model i have for both of them a table and want to join in the average rating and user specific rating into the response when you request it via the api so i forward it from controller to service and from service to repository there i want then to create my queries
model classes:
tables that get created by ef (see image)
model creation override:
my issue then lies in the repo itself see here:
that includes the entire table to the nav property but does not assign the values into the properties i need to can convert into the response i need
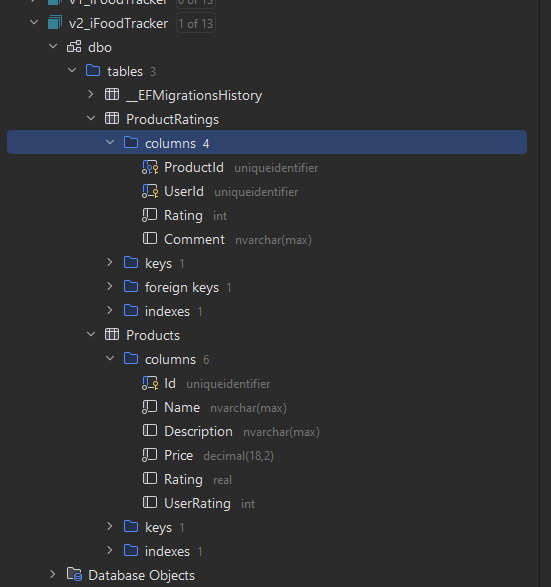
5 Replies
so, that
if (userId == null)
part...
i would avoid having that logic inside the query method
what does this mean
does not assign the values into the properties
property UserRating and Rating stays null as they arent assigned from the query (in the object of type Product)
we haven't got much to test
are you sure you are hitting the right query?
are you sure your data is complete?
did you look into the db context at debug time to see if there are all the fields
yes there are
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.