Implementing JsonConverter to simplify ser/de
I've been trying to implemement a JsonConverter for a simple class to simplify the default C# tagging using JsonSerializer. Here's the class I'm trying to convert:
And here's an example serialized output for what I need:
(FYI the default serializer outputs
{"Taken":"2020-06-21T00:00:00","Data":"this is some data here!!"}
). How do I implement a JsonConverter to Read & Write to my desired format? I need the generics to always work and this Snapshot will be used as an attribute of a parent class
I've wrote this so far which feels like it's going in the right direction, but I'm not sure if the JsonSerializer
line here is right and I'm not sure about how to implement Read
:
30 Replies
quite sure this is impossible
Are there any libraries to do this sort of thing? I'm basically reimplementing my rust client in C#
i mean i'll just be real, this is a really bad json layout
like, awful
Looks like a KeyValuePair entry for a Dictionary<DateTime, T>. Would that be an option instead of using Snapshot<T>?
God yeah I messed up
Thats the json for the Element
Haha hang on let me get the actual class
Sorry about that
dictionary is the only thing i can think of as well
but the key of a json entry must be a string so
I think I was debugging the wrong class for a little while, I'll check if it applies and send the right problem :)
the date should definitely never be the key
This looks like an answer to a similar issue https://stackoverflow.com/a/41503997
Stack Overflow
Serialize List> as JSON
I'm very new with JSON, please help!
I am trying to serialise a List<KeyValuePair<string, string>> as JSON
Currently:
[{"Key":"MyKey 1","Value":"MyValue 1"},{"Key":"MyKey 2","Value":"
Yeah the JSON is for a class with a dictionary inside (called Elements), posted the wrong bit but I was debugging assuming that JSON which is why I couldn't get it to work
Had the wrong test case
Yep seems to work
This is what I actually needed help with haha:
How do I flatten this so there's no
{"Values": [data here]}
so its only {[data here]}
I need it as a class because it's got related methods otherwise it'd just be a Dictionary. In rust this would look like
class Elements<T> : Dictionary<DateTime, T>
?
but again, the datetime being the key is really weird...Did not think about that
Timeseries data
don't know what that means
title here is Elements<string> for example
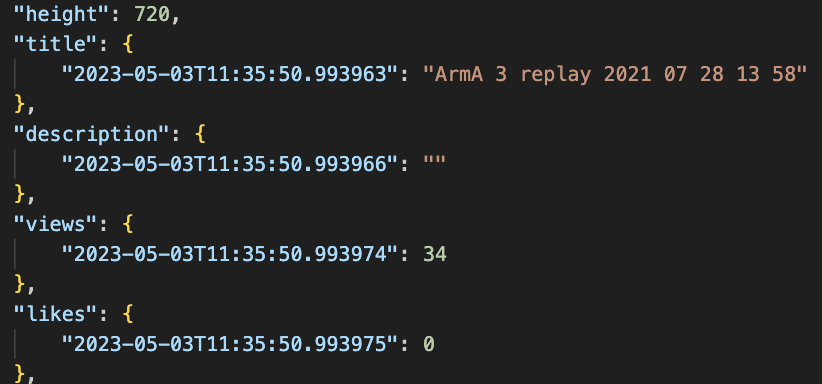
I'm writing a youtube archiver which can snapshot metadata at different places in time
this seems really backwards
i think with this you just disqualified yourself from getting anymore help, since to me to me it seems like you are scraping data from yt which iirc is against tos
the only other way to do it is like that original Snapshot class in a list but then you lose the hashtable
this would definitely be better
but yeah we can't help with scraping
metadata captures not perfect so sometimes only certain things are either updated or remain unchanged
sure
and Elements means each is generalised
what's it matter
title.current()
a lot easier with ISO alphabetically sorted dates
snapshots.orderby(date).first().title
rather than having to linear search through each snapshot and then see if theres a title in there or if its not been captured or not been updated
im tracking each update not each time ive possibly captured it
also btw currently all your dates are messed up
yep they should all use the same time
cause you fetch datetime.now anew every single time
any more critiques?
i guess not