❔ How to make a method returning type T but only taking subclasses of a certain class ?
I'd like to make a function that takes the type T as an "argument" (
T MyMethod<T>()
), but the type T must be equal to Type Z or subclass of Type Z, how do I do that ?
I'd like it search through defined dictionaries that are all typed as subclasses of Type Z.34 Replies
I don't know if it's clear but uh...
Basically this was the code I was planning to do, however it tells that some code will never be reached:
which code is it saying will be unreached
every single case of the switch
they are all told as unreachable
what is the exact error it tells you
Okay I changed a line and it deleted the error...
I don't know what happened
but it was telling word for word "this code is unreachable"
Although I need to know, can be the argument T type be omitted if it is specified before ?
what I did before is that I deleted the T type argument and my switch was
switch(default(T))
and there the code was unreachableyes, that will not work
null
will not match any of them
it will always go to default
because null
is not AudioClip
or GameObject
or Material
Oh sure..
Oh yeah I see
And can the type argument be omitted when T is putted before ? Or not ?
what do you mean by "if it's specified before"
Like uh
GetAsset<T>(m, x)
the <T>
can be omitted if m
has the type T
e.g.
there is no other circumstance in which the <T>
can be omittedno, because those are completely different things
Ahhh
T type
means "give me a T
"Yeah an instance...
"give me an
AudioClip
"that's not what I want...
i think you will have to give up the
switch
hereOh wait
if I do
new T()
?Ah I see
Is it possible to give several contraints to
where T : new() & where T : UnityEngine.Object
sorta ?
oh yeah my bad
im dumb lol
i just had to put a comma
Would this work ?
no, it would not
it's not making any error in my IDE
oh...
it will make an error when you do
GetAsset<Material>
or GetAsset<AudioClip>
Ah...
That's annoying...
Curious, why’s that?
because they do not meet the
new()
constraint
they do not have a public, parameterless constructor
it's probably true for other ones too but i didn't check all of themAh... I see...
Well then
I'll do your thing
And can't I make a
switch(true) { case true when typeof... }
?i suppose you could
Okay alright
Thanks, it does not look to bad
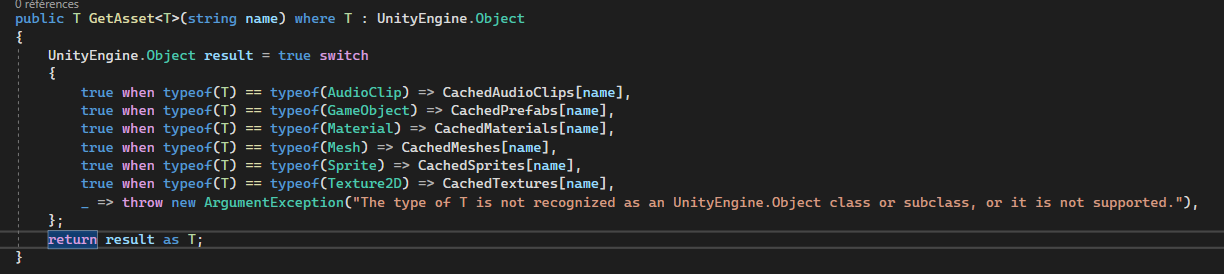
I'll obviously add a check if result is null
Ah
It will an exception before
lol
Thanks for help 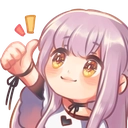
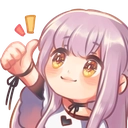
could go even further and maybe do something like
where CachedAssets is
This way if you have a new asset type you want to cache you just add a key-value pair to CachedAssets dictionary
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.