❔ Validating user input
I have created a project and I need to learn how to validate user inputs
324 Replies
What kind of project? What kind of user input?
I'll show you in a minute
this makes no sense
Ok
the class seems to be an entity/dto/model, and it has a static
ValidateUserInput
method that.. prints an optional message, and if no message was provided... what is going on?I just wanna say to the users you either enter a name or I will throw you an invalid message if leave it empty or null.
what kind of input could a single string hold that would be deemed "valid" or "invalid" in terms of a UserAccount anyways?
validation of user input usually means checking if the provided values are "okay" or not, this does not include checking if they match an existing item in your database
its stuff like "did the provided account ID match our expected format"
Well! It's just a console application that I'm creating, so it should only validate if user input is null or not that's all.
If you do it your self, how would you modify this logic?
There is already a method for that.
string.IsNullOrWhitespace
Ok! You're right. I've used it before.
Let me show you my implementation
It's working now. But the only issue is that I'm repeating the readLine method twice.@pobiega
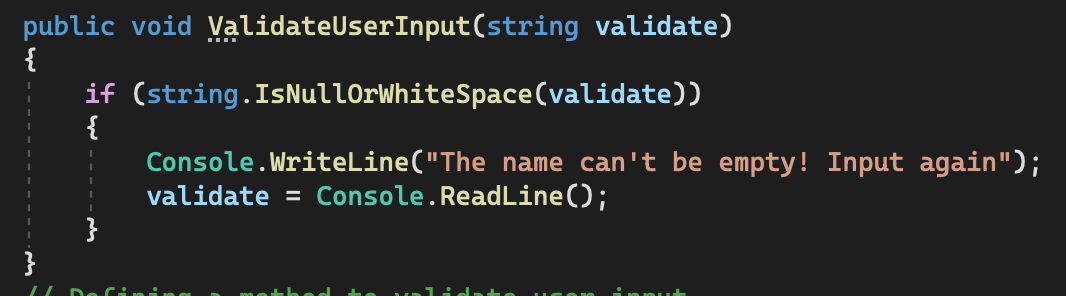
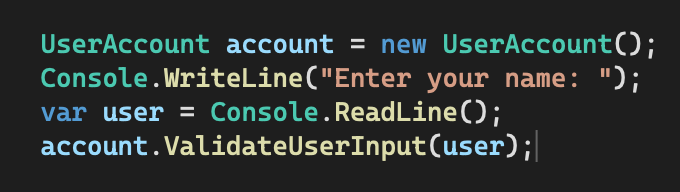
I'm out and about at the moment, I'll post a different solution when I get home. ETA 15
Ok! Sounds awesome mate, thanks a lot.
Okay, so, lets first talk a bit about validation vs input and how they co-relate
any method that does validation should either return a
bool
indicating if the validation passed or failed, or throw an exception on validation failure
I personally greatly prefer the bool
approach
second, we shouldnt allow invalid input, so we need to repeat the asking for a value until a valid value is passed
we also dont want to repeat ourselves, so we want to separate the input taking from the validation, so it can be reusedOK
Great to see you back again
Have you seen generic arguments before?
its stuff like
List<string>
I'm still reading your content
so our validator will look something like...
and we dont call it directly, instead we pass it to the input method
this guarantees a valid name
Sorry, I just had to wash some clothes. Now, I'm back again.
Yes
What do you mean by that?
look at the code I posted
Yeah! Did review it actually. It looks really good to me.
But you're using delegate like
Func<string, bool>
I'm not at this level yet.
I noticed that you're defining a second method outside the main program, right? Main
is a method, not a program
and its fairly unusual to declare methods inside other methodsSorry
even if its possible
so yeah, in this case I just declared it on
Program
itself.Yeah
in a real program, I'd have a
UserInput
static class with these methods in itHow come you didn't declare it in another class? Why underneath the main method a second method?
Ok
here is one of my favorite methods, possible because of recent .NET features
Wow, this looks even more complicated than other one 😃
so if you want the user to enter a number, this guarantees a valid number
I used this source and it looks like shit https://codeeasy.io/lesson/input_validation
Input Validations in C# at C Sharp for Beginners Course codeeasy.io
Discover how to perform validation in C Sharp applications to prevent a user from inputting wrong data at our interactive tutorial.
you can make the validator optional quite easily
Compare to what you showed me
Really
Like how?
Any tips?
optional validator doesnt really make sense for the string version, as there is no parsing
Ok
So mate, do you recommend me to use this code everytime I create a project and need a user input validation?
More or less?
Applying these methods?
input should be taken in a while(true) loop, returning only on a valid input or the user killing the program
validation should be a separate thing
the two methods I showed above do this quite nicely
you dont HAVE to use them as is, you can write something different
but imho, this is best practice when it comes to user input and validation
you could modify this so the validator returns a
ValidationResult
object instead, so you can customize the error message etc
but the core ideas here are solidOk! You're right. This is the right context for validating user input
Just like you mentioned, I would like to modify this logic, just for practicing purpose and also for validating multiple user inputs not only for the
FirstName
I think every property deserves its own method, because we should be validating user input individually and not wait until the user enters all the inputs and then throw an error messages, right?that's up to you and how you want your application to behave
Ok
That's correct
I'm gonna practice defining a similar method for each property then
Although this will create a whole bunch of code just for validating 😆
thats fine.
but yeah, for a console UI it makes a lot of sense to validate each input at a time
if your name isnt valid, you dont want to know that after 10 other fields
You're absolutely right bro.
As you can see I implemented another validation function, but this time I'm validating even the regex characters, although I copied your second method for looping, but I will try to figure out a way to do it differently.
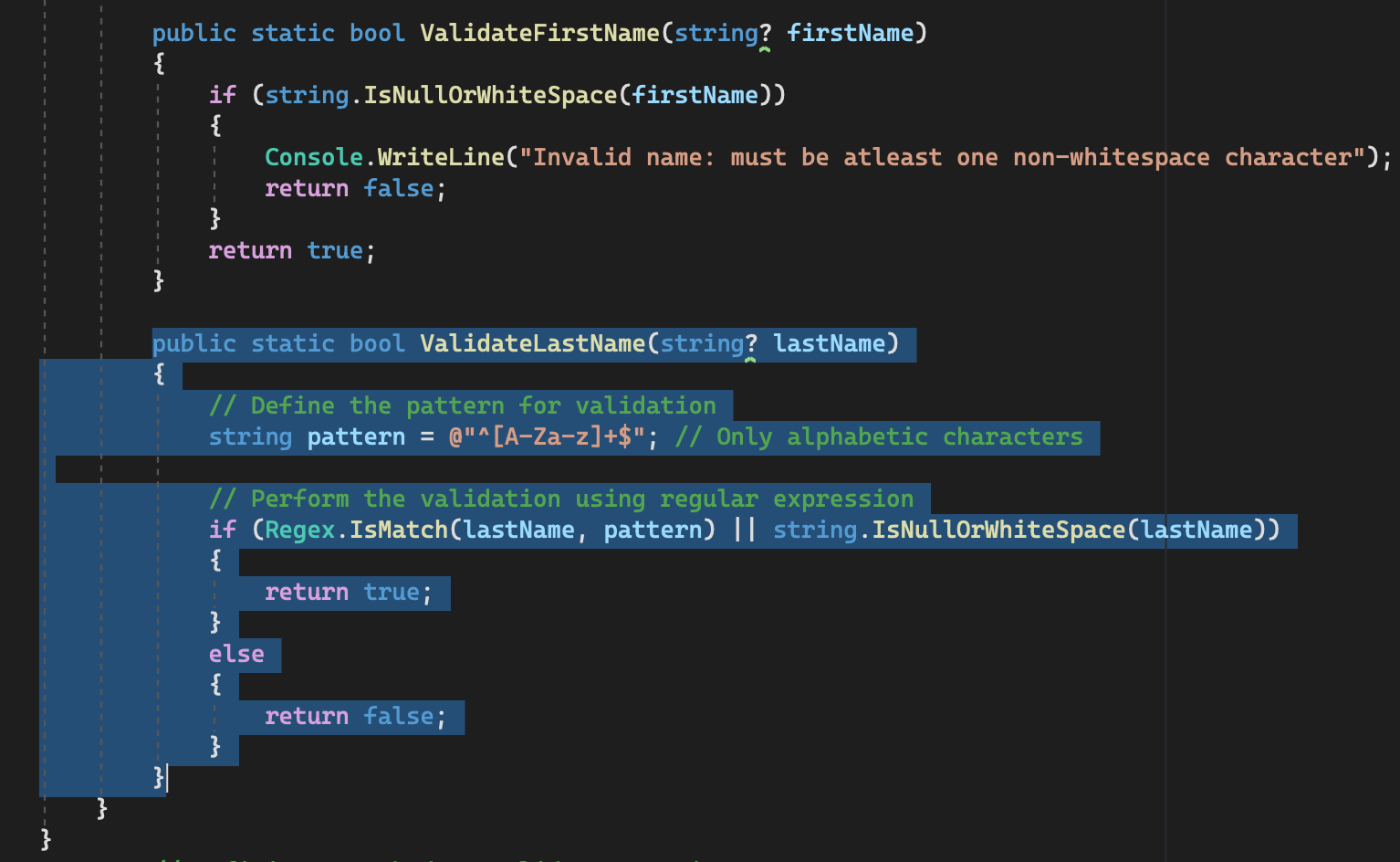
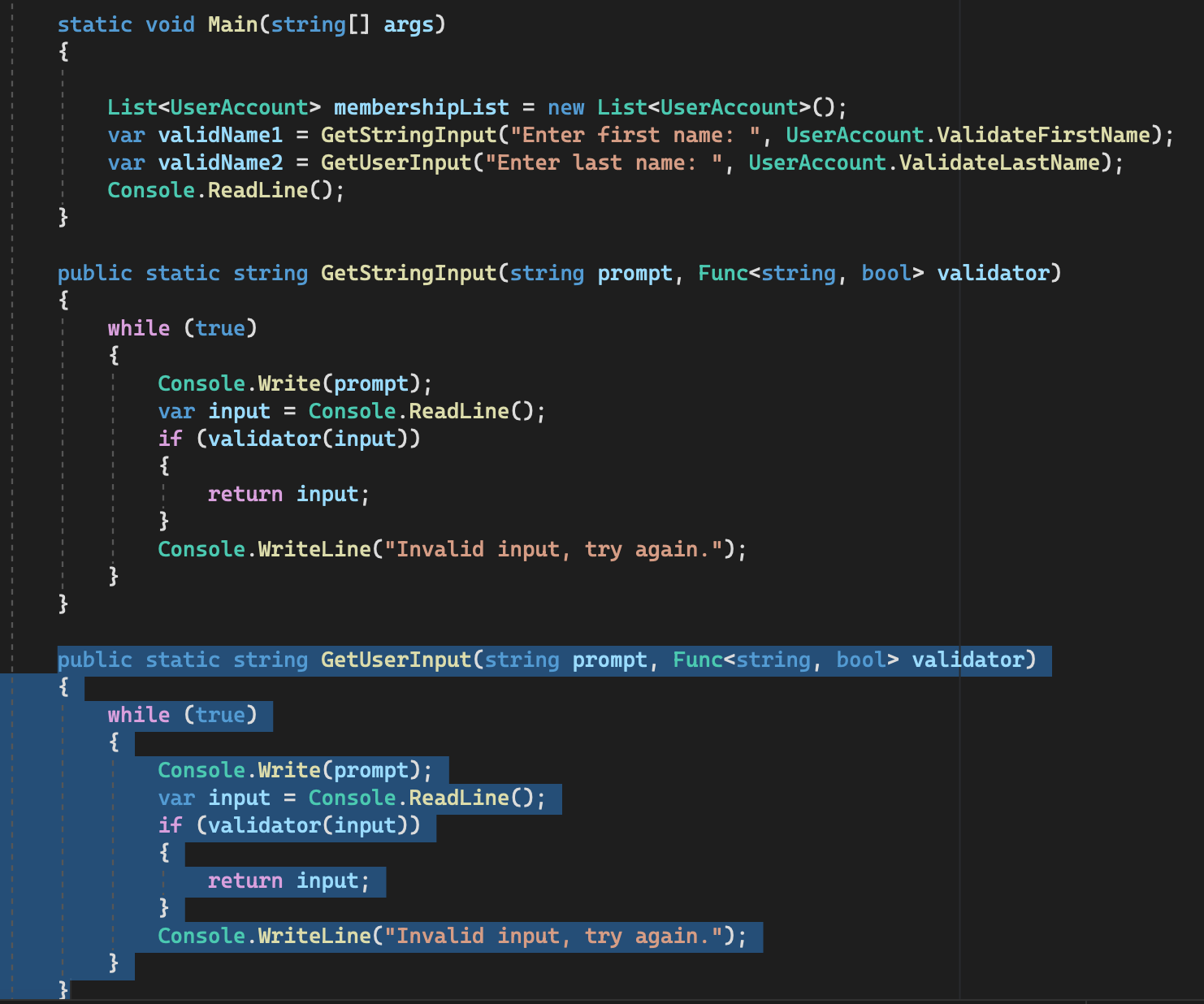
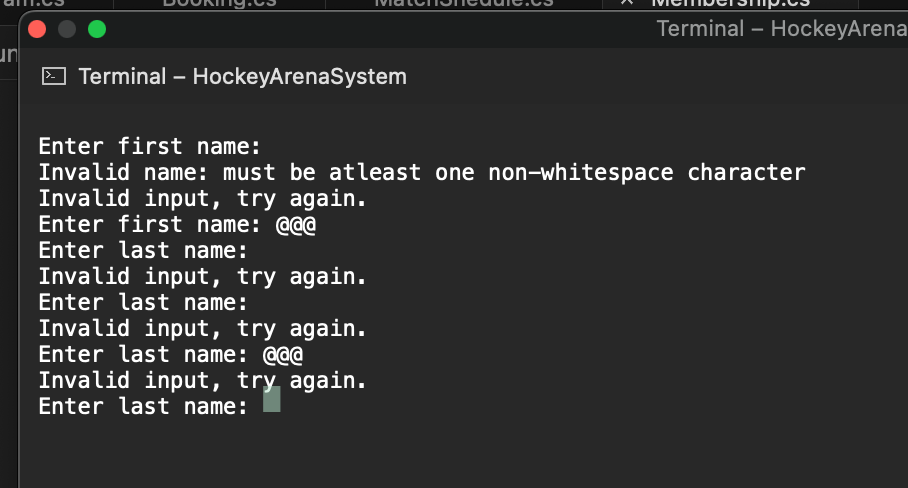
Although, I used ChatGPT since this helps me a lot to learn new implementations and understand them by reading the logic and notice what it's doing not like google examples which they suck and outdated.
you could just use
this will be a lot faster than using regex
Oh! Wow 😮 that's cool mate. This is even shorter than my logic.
Someone in the server advised me to always try writing a logic on my own without looking at any source and if I couldn't after multiple tries, then google it and if this doesn't solve it then the final solution is ask for help and this is exactly what I did with this code, so my question is how can I print out the invalid message until the user enters valid input?
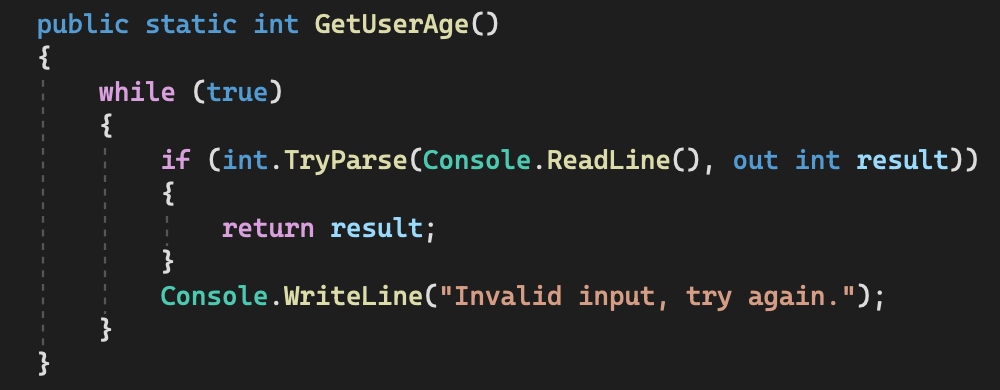
Another tip he gave me. He said write code even if it sucks, even if it's wrong, no one will laugh at and don't feel shy to share it 😉

Prompting for user input is outside the while loop, so it won't ask for user input again, so I need to figure out a way.
where would be a better place for your prompt?
Well! It would be better if it's defined insaide a loop.
Where every time the user inters invalid input, I should be re-prompting again and again.
how can you modify your current code to achieve that?
while not hardcoding the prompt
This is what I'm trying to figure out by re-reading and re-think it
This is interesting
I mean, if your method is going to be
GetUserAge
then its fine to hardcode the prompt, since it will only ever ask for an age... but then you'll have an almost identical method for another int
tooOk! Why should I have another identical method then? Can't I achieve both at the same time, prompting for user input & validate for invalid input.
exactly, why should you?
Imagine the following, you need to ask for 2 strings (name, adress) and 2 ints (account number, pin). You could do this with four different methods, hardcoding the prompt and the validation in each... or you make 2 methods, one that returns a string and one that returns an int, and you don't hardcode either the prompt or the validation.
@jimmacleI think this is answering the question
So, how would you create a method to take input from the user as an
int
, and not hardcode the prompt?But this can be a kind of tricky. How to prompt & validate for two strings and two ints in each separate methods?
its really not tricky
what changes between the two calls to the same method?
ie, what needs to change between asking for name and address?
you've already learned all the pieces you need to do this, you need to figure out how to put them together
this is a pretty critical point that you have to get past, you cannot progress in programming without being able to take previously learned concepts and rearrange them to solve a new problem
Ok
Trying to figure out these questions 🤔
it's a really simple answer
write out the code for both, as two separate methods
then compare the code line by line
You mean writing out two methods one for name and email address and second one for int age?
you're complicating the problem before solving the original one
pobiega#0000
ie, what needs to change between asking for name and address?
Quoted by
<@!901546879530172498> from #Validating user input (click here)
React with ❌ to remove this embed.
no age, just those two
Ok
Correct me if I'm wrong. I mean from my understanding point and something just clicked in my mind by reviewing your previous code is that you're defining a method for null or white space and this method can
GetStringInput
can be used for both user name and email address, but the second identical method is for prompting for user input until a valid input is entered, right?uhm, can you rephrase that?
that made me scroll up and now i'm really confused why this problem isn't solved yet 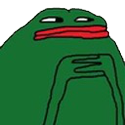
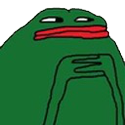
ignore the validation for now, just think about the rest
what separates a method asking for a name from one that asks for an address?
Parameters
yes!
I mean we could define two separate methods one for name and second for address, but we could combine both in one method public void GetName(string name)
so both combined with each other would be as the following:
no.
those dont get anything, they print
Ok! This opens a new question, I'll have to pass two arguments in this case for name and address and we should be asking in two separate invocation, right?
Yeah, you're right.
Our goal is as the following
yes!
using te same method
parameterize the prompt, thats the way
Aha
Like this
Before I show my thought
Is this what you mean?
almost
the method doesnt need to be called
GetFirstName
now
since we parameterized the prompt, it just gets a stringI feel like you're combining two methods using delegate?
Aha
Ok
kind of
It was my fault editing the signature name
we need to be able to change the validator, since what is valid is different depending on input
so it feels right to pass the validator as a delegate
a name might not have the same validation as an address, or a zipcode, or... whatever
but the rest is the same
ie, the prompting (with different prompts ofc), the loop, the getting of input
Trying to understand the logic behind what you just explained 🤔
I got it here
It makes sense to me now 😉
good
You're right. The only difference is how we validate the name is different than the address, but the rest of logic is the same. Man this is a bit deep logic for a beginner.
.so my logic is wrong implemented here
I could basically use the same parameterized logic for age input too, right?
yes
you need a different method for int compared to string
since one requires parsing and one doesnt
So is this what delegate does only or it has other uses too? Although, I haven't reached this level yet learning delegate, but now I got an idea on what delegate is from this example.
Ok! Clear enough.
delegate
the keyword can do more things
but we rarely use it
Action
and Func
is all you need in 99% of cases
they are generic delegatesI'll write some logic and show you even if it's not perfect, the most important is just get comfortable of writing code 😉
yup
Ok! Yeah! This is what I noticed too. Action and delegate is what most people talk about in the server.
Awesome, get back to you soon.
Waite minute, I thought about something here, why can't I use the same two methods invocation to take user input and validate for all the rest of properties except for the
int age
? If we could take user input and validate two strings using one method why can't we do it for all?
It should be possible
store in a different variables
@pobiegayou absolutely can. thats the whole point
Awesome. Yeah! I thought about it logically and reviewed your previous messages and thought, how can we do it like this? Why can't we do it like this? Good specific questions makes it clear to see what's going on.
and you can get the phonenumber, email and address the same way too, just with a different validation method
That's why we use method too 😉 so we don't repeat us many times, other wise the project will become spaghetti rather then understandable flow.
yes
Exactly, but should they stay as strings or modified to integers?
.
what do you mean, you want to store an email address as an integer?
Sigh.
I was so happy when I saw you using a string for phone number, because I thought you had thought about it and realized that its not actually a "number" as such
No, the email address will stay as string, since emails always contains letters, so even if they contain numbers too, we can't use any number data type for letters.
can you clarify this question then?
what is "they?"
I've seen before you mentioned that phone number should always be int type
i'm 100% sure he never did that
think about it
what is an int
and then, how does a swedish phone number look like
or a phone number with a country code
or one with an extension
I have, several times, stated that a phonenumber should NEVER be treated as an integer
I might asked the wrong question, but it's alright, it seems obvious to me now after having thought about it.
leading zeroes are important, for one. You have symbols like
+
, -
and ()
in themI mean a phone number is just like an address, it's stable and doesn't get change, doesn't get calculated, doesn't increase nor get reduced, so it makes sense to assign it as string.
yes
the more important distinction is that phone numbers have more information than can be represented by a simple number
int
is good because it lets us do things like math on itYep
but doing math on a phonenumber isnt really... relevant
Exactly
+46 123 123 123
would be a hard time to fit in an intNo it's not in any scenario
Yes, I think we use double for long numbers or high precision numbers.
but a phonenumber isnt a number
so stop thinking about it as a number type
its just a string mostly made up by digits
Yes
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.So now after being done with creating and validating user inputs, I would like to practice implementing the logic of writing these inputs from user to a text file and then reading these data and add them to a list called userAccountList, what do you think?@pobiega
Look at my planning
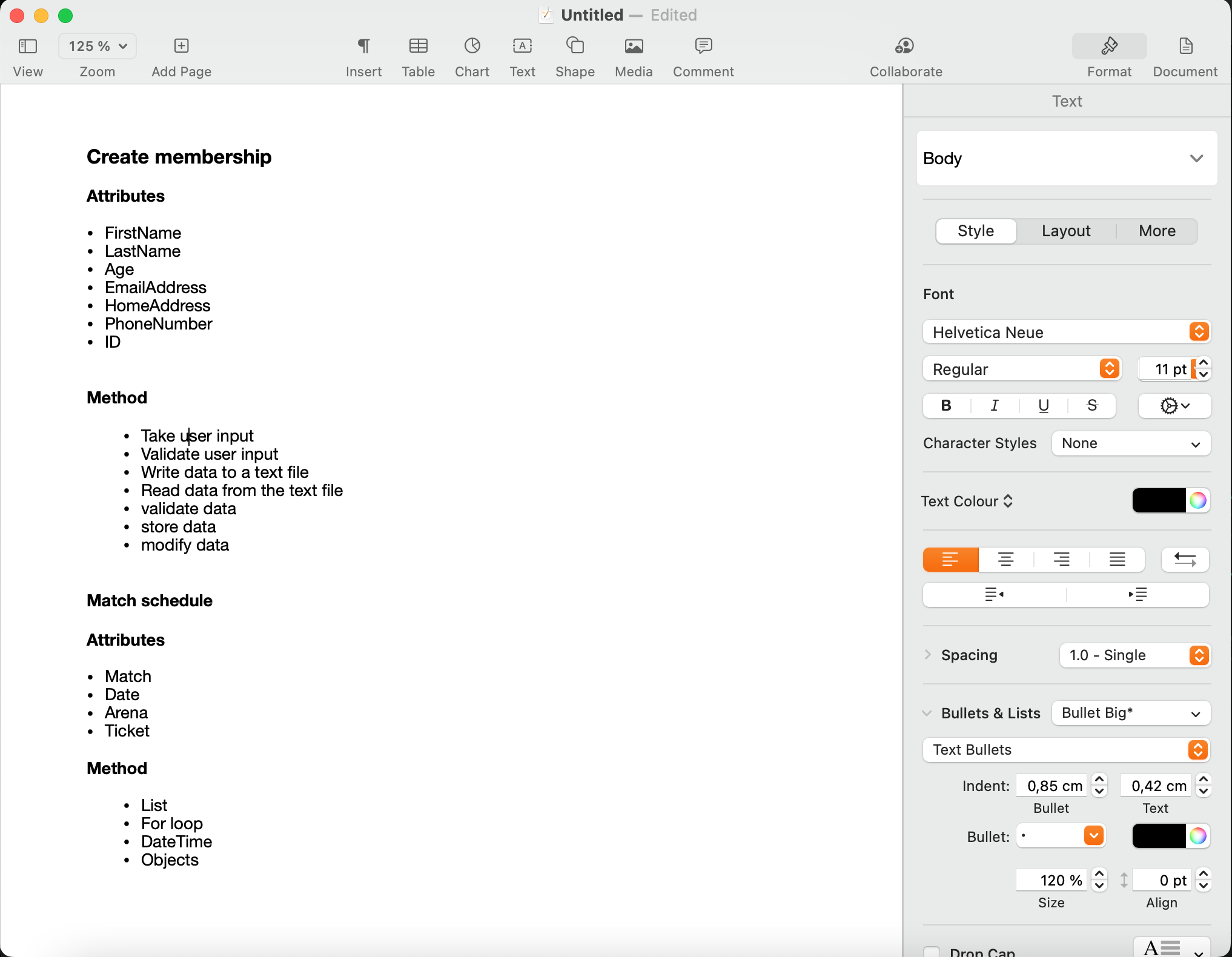
sure
Awesome
The first small step would be defining a static method writing and reading data, right?
I have a question here though! Should the method be defined as list type or should the list be defined separately in the main program?
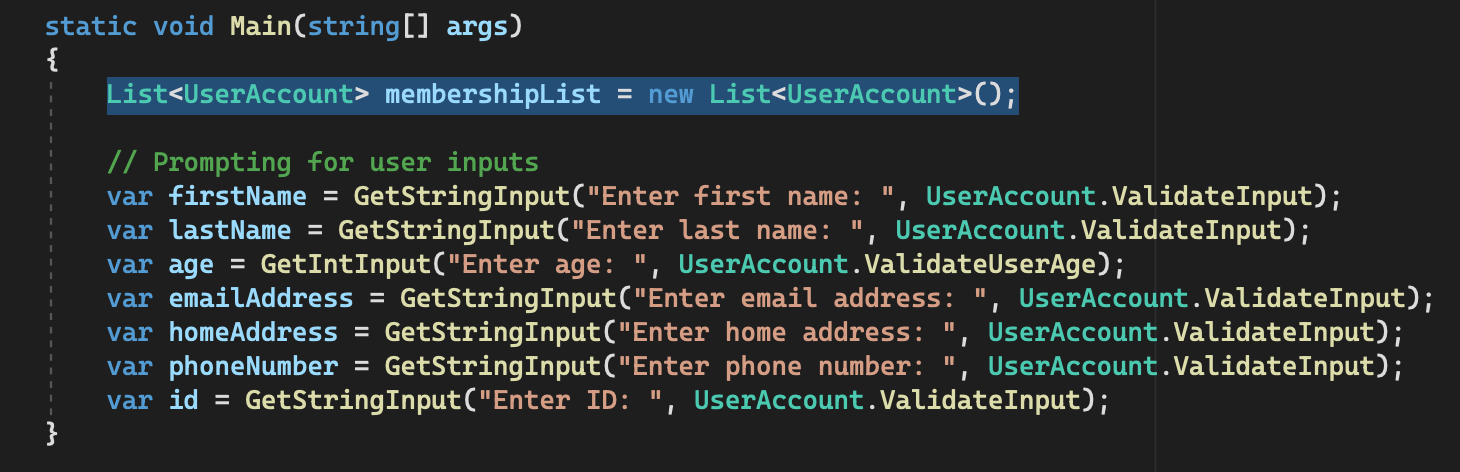
?
Or
what?
Sorry, I might confused you.
Ignore what I said earlier
what does that have to do with writing to a file?
Next question is should I create a class and do the writing and reading data inside a method?
Nothing, I said it wrongly.
Or can I implement the writing and reading here?
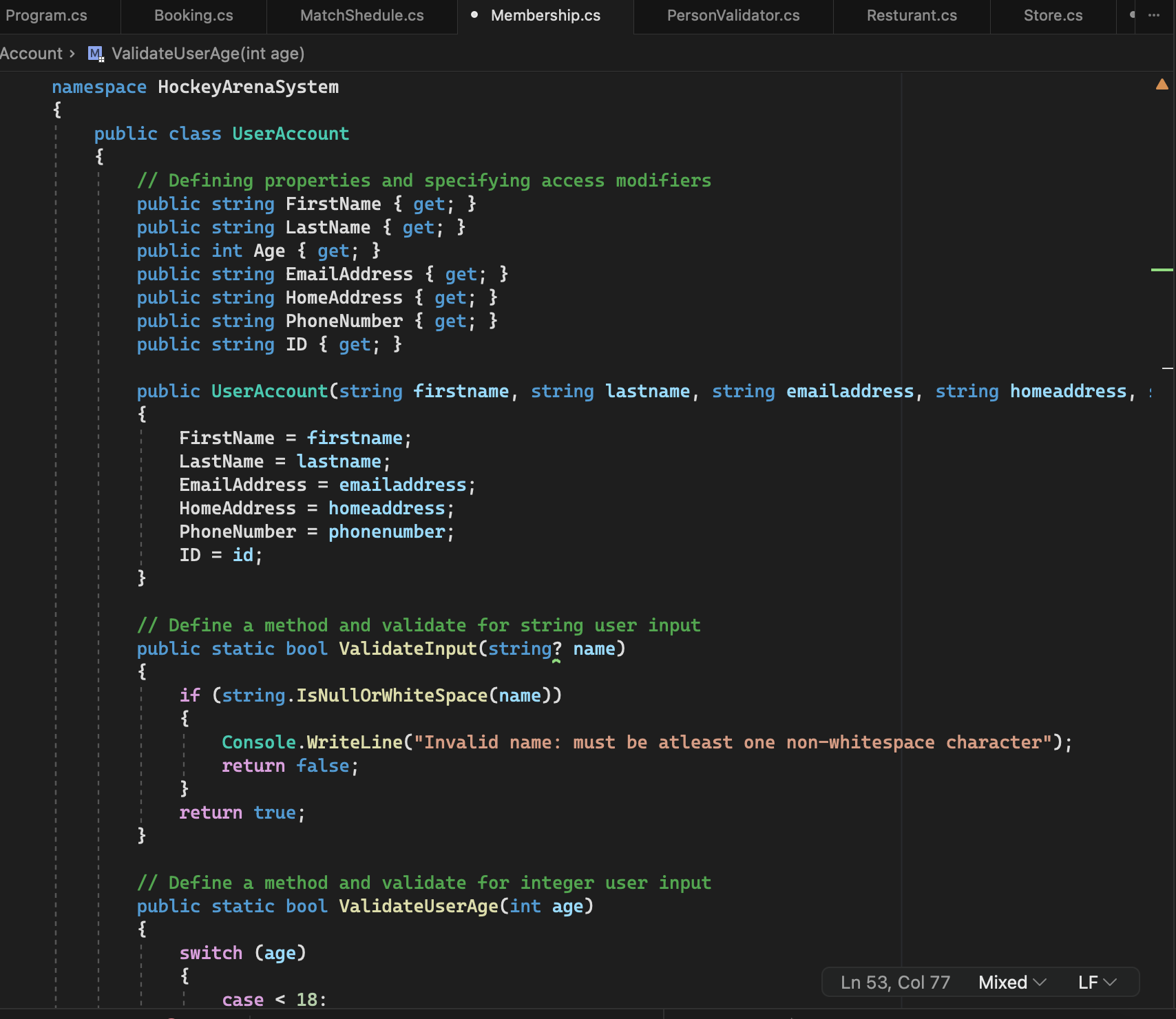
?
you can implement it anywhere you want
Ok! I know, but which one is best?
there is no "best".
a static load and a non-static save can work
You know what, it's easier to have all the logic that is related to creating user account in this class.
or you make a new class that is responsible for saving/loading all your data, instead of just one class
Ok
Great, I'll go with this option then. Thanks a lot bro
Should I call the class something like
UserData
?¯\_(ツ)_/¯
I have a question for you man?
When writing the date that are inputed by user inputs, how do I grab these data and write them to a text file? Should I create an empty notepad or something in my local MacBook, so these data will be saved in the file?
@pobiega
what
Well, I'd probably use json serialization
and you would serialize an entire "UserAccount" object
Ok! So these user input values should be stored in an object first, right?
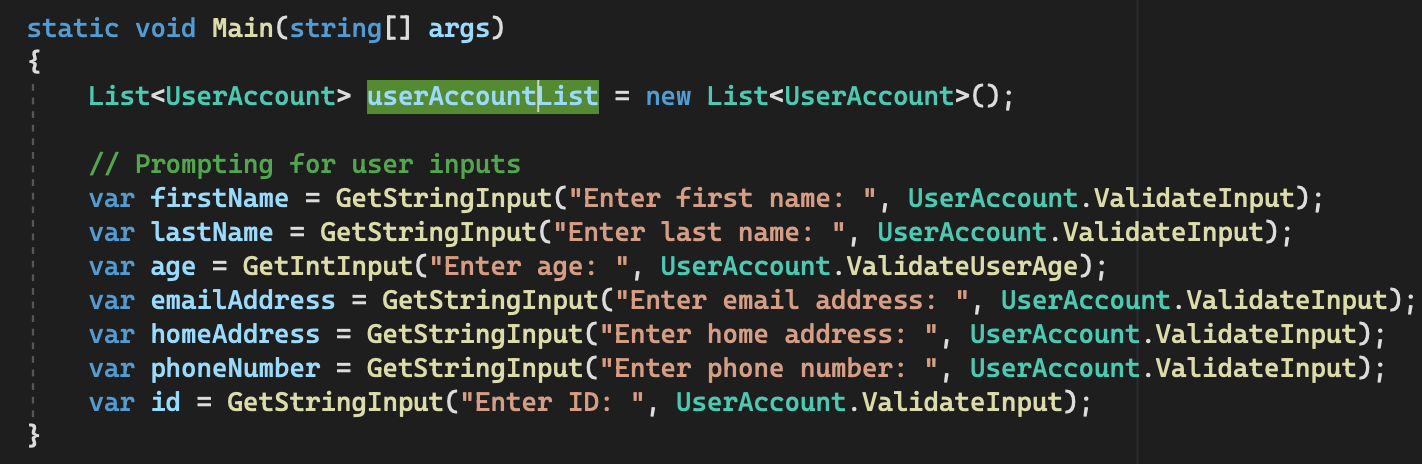
I haven't touched anything near to json 😆
You know I'm still a beginner 😁
Well, I thought that was the purpose of your
UserAccount
class?Ok! Well! The primary goal was just practicing the fundamentals, but then updating it to be used in a Windows Forms. So now pretty much just practicing write and read to a text file.
I'm reading about serialization right now https://learn.microsoft.com/en-us/dotnet/standard/serialization/
Serialization - .NET
This article provides information about .NET serialization technologies, including binary serialization, XML and SOAP serialization, and JSON serialization.
good
This article doesn't provides enough details about Serialization? How do I learn to implement this topic?
Should I follow a tutorial or similar?
.
@pobiega
did you follow the reference links?
the one for System.Text.Json in particular
How to serialize and deserialize JSON using C# - .NET
Learn how to use the System.Text.Json namespace to serialize to and deserialize from JSON in .NET. Includes sample code.
Yes, I've been reading it, understanding the context.
Thanks a lot for the link 🤗
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.@pobiegaI've been reading a lot about Json serialisation and I reviewed the code samples too.
I noticed when serializing, you would need to declare a variable and then use the method to pass an object inside the parentheses.
But not sure how this applies to my case, I mean I have variables and not an instantiated object!
you should have a C# model that matches the JSON you're trying to serialize and deserialize
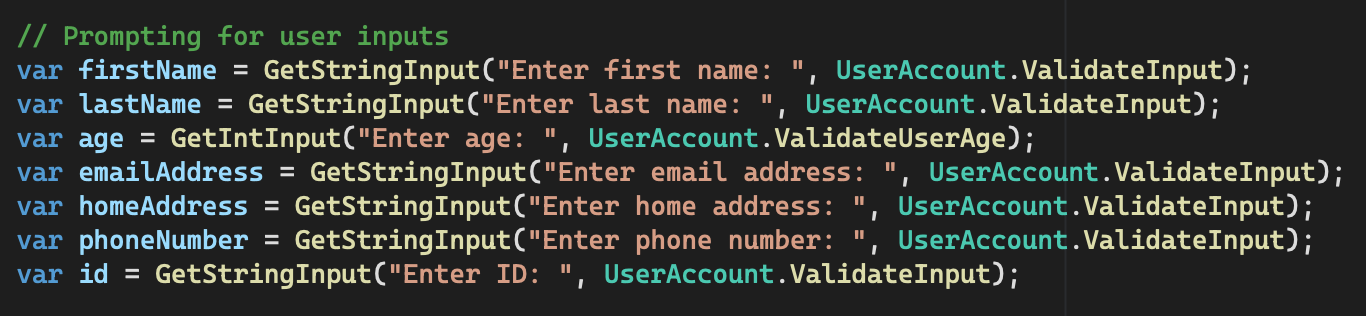
Could you make it more clear?
you need to create a class to hold the data you want to serialize
just like the
WeatherForecast
in your exampleAha! A separate class.
But my friend, it's shown in the example that weatherForecast is defined in the main Program and basically I'm doing the same too, why using another class?
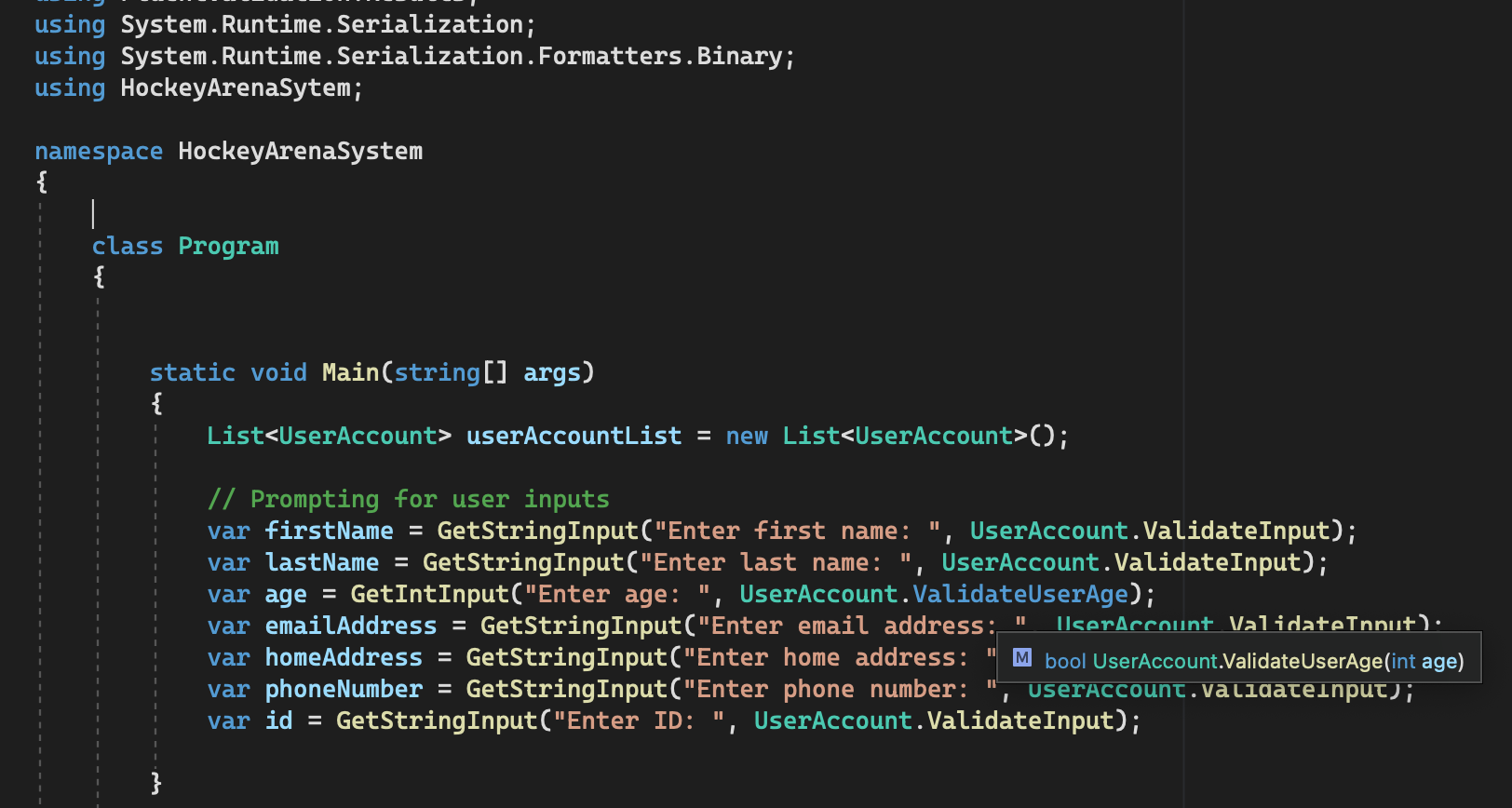
you're not doing basically the same thing, you don't have a single object to serialize
what are you trying to save?
No, I don't actually.
I'm trying to save the values that are stored in the variables from user inputs into an object and serialize that object to be able to write these data to a text file.
okay, so where is the object you're storing them into?
I would need to define one then
so just to clarify your thought process for me, did you get stuck here until i told you the answer?
Kind of
Just like I mentioned earlier, I read the docs these two daus off the work, but I felt a bti confused, cause the examples are using instantiated objects for serializing, but in my case, I don't have one.
right, so what's the solution to not having one?
Hint: look at your validation methods.
This is a good point that makes me realize what part I'm missing here
Think about all your variables. Together, what do they represent?
They are all parts of a bigger whole, no?
Ok! Yeah! I think this is where you referred in the hint ☝️
I've been reading this article too, but reading without knowing how to apply it particularly in mt project leads to no where, thus I need to use it. https://blog.postman.com/when-and-how-to-use-json-serialization-in-postman/
Postman Blog
When and How to Use JSON Serialization in Postman | Postman Blog
Get a quick review of serialization and why it’s used, and then learn how to work with JSON serialization in Postman.
Of course they represent the
UserAccount
Yes. But have you created your user account instance yet?
Nope, not yet.
You mean instantiating an object of the
UserAccount
?
I know I should be doing that based on the article
But I have one question for you that makes a bit curiousI know when serializing we are instantiating an object and then using the properties to serialize variables into string, but what's the relation between the variables that stores the user inputs and the properties? I mean I know I should be serializing the user inputs, but the examples in the docs are using properties to serialize not like in my context 🤷🏻♂️
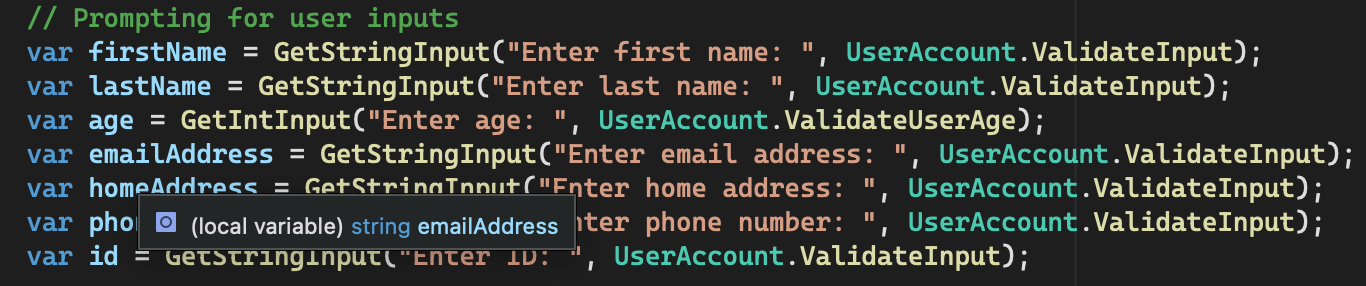
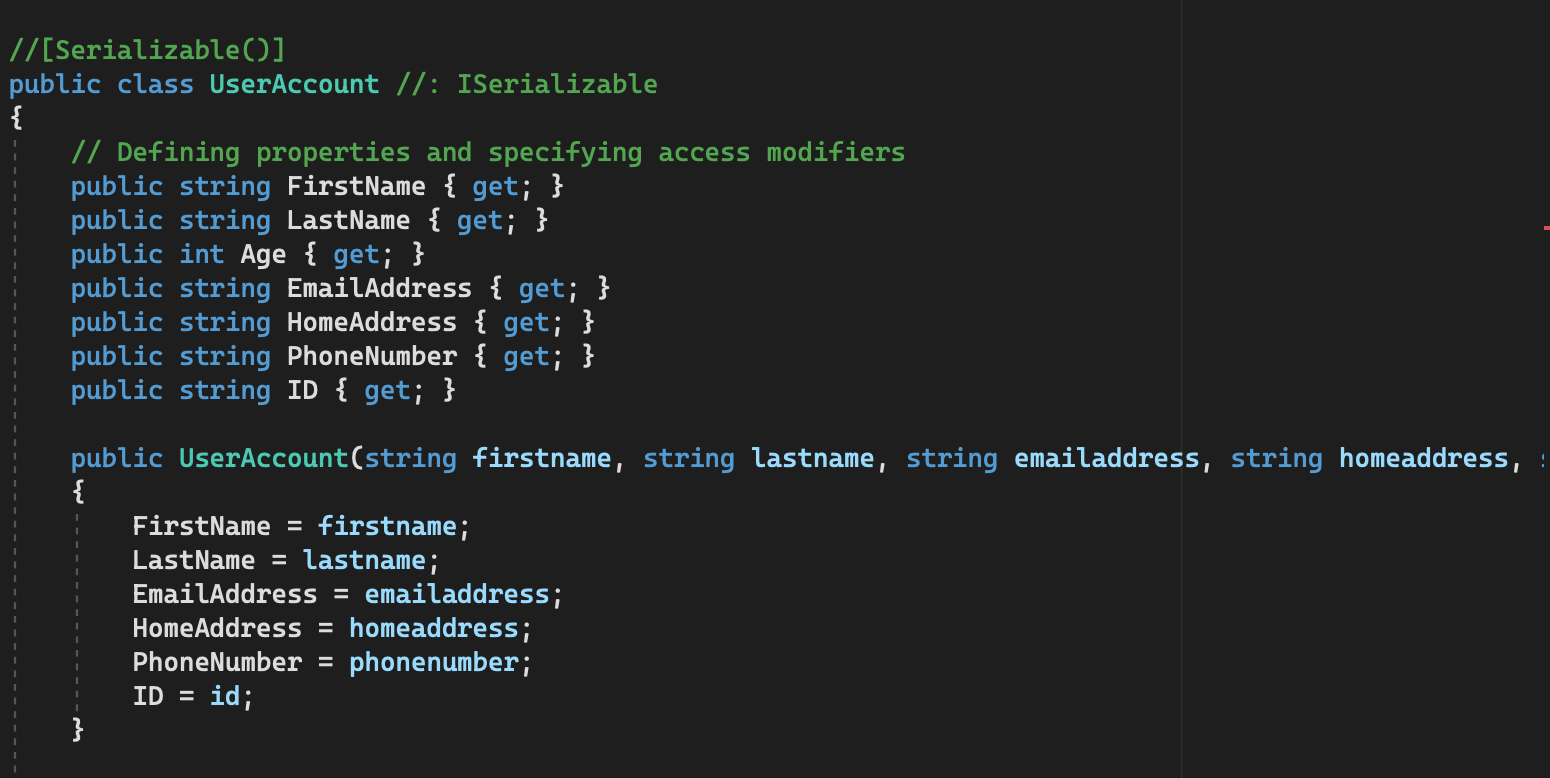
Questions like these makes me wonder why this? How is this doing this? Why this?
?
I do not understand the question.
you CAN serialize a single string, but there is no point in doing so
the strength of serialization is that it can handle more complex data shapes, like objects, or lists
Once, I learn how to create user account, serializing and take notes, then I won't need to re-ask again in future projects.
Ok
what do you mean "learn how to create user account"?
you already know how to instantiate objects
I mean the logic where I have already done like methods, user inputs, validating, serializing and etc..
?
Yes, I do.
So create a user account
Ok
What do you think?
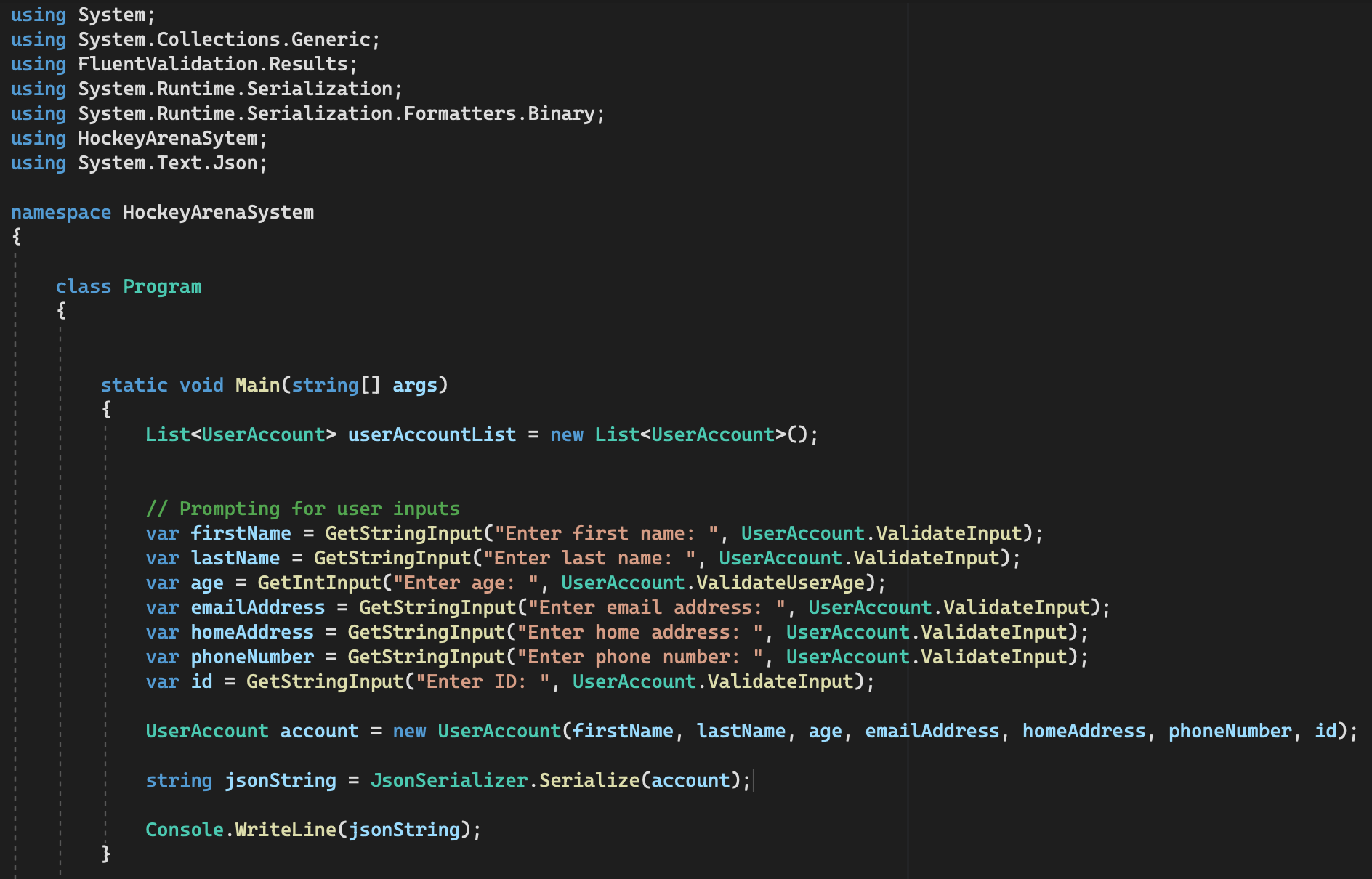
yup
Awesome
What is the plan for the list of user accounts btw?
If you are not going to use it, I'd remove that line
I thought you'll say "what the hell" you've been in c# for over a year now 😆
Well! At first my plan was just to create user accounts and add to a list, so I could just remove it.
okay so whats next?
you have your serialized json string.
Good question
My plan at the beginning was to write & read data to a text file
ChatGPT
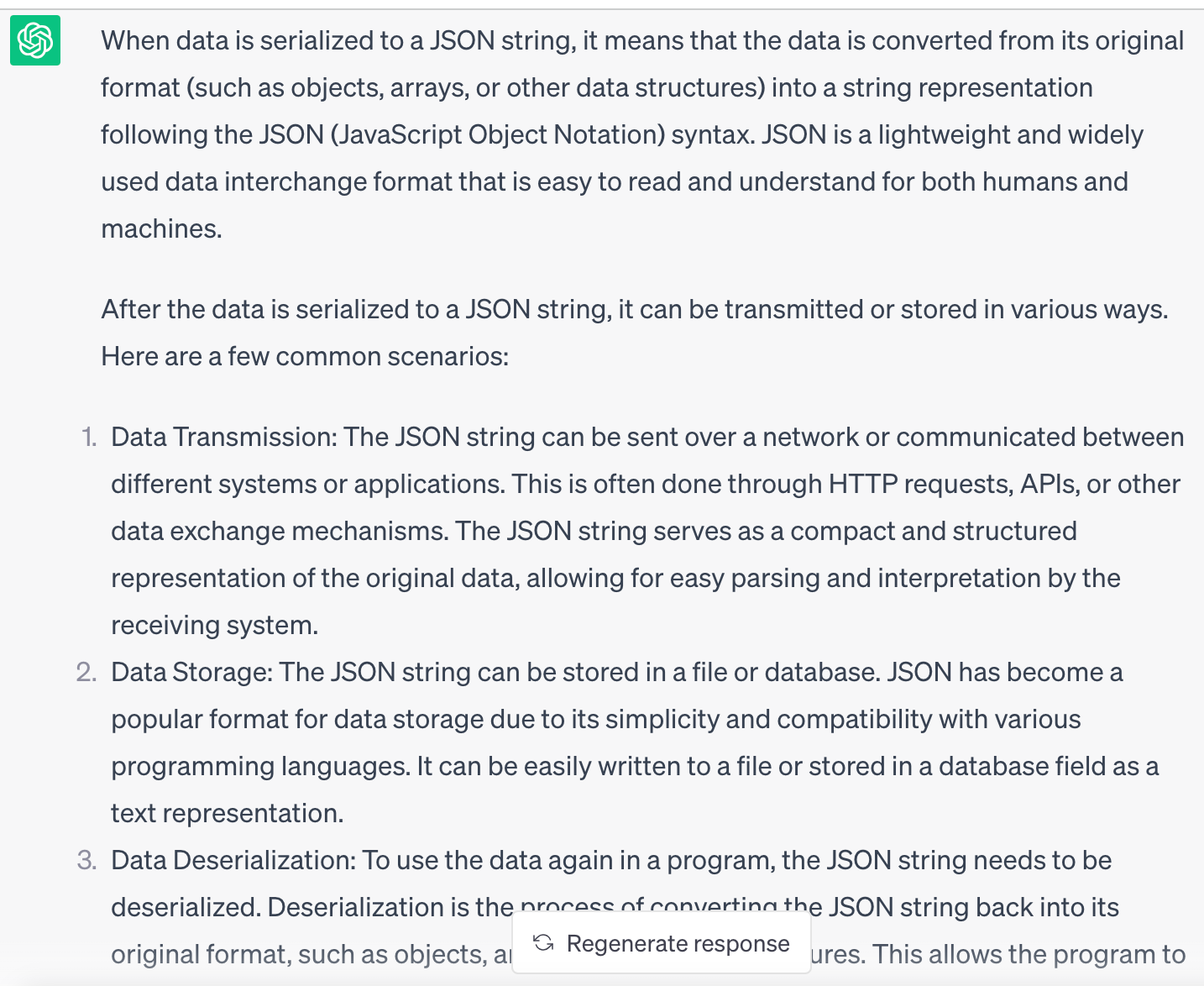
ok so do that.
what did you ask chat GPT and why?
Well! I was just curious to learn what you can do with the data after the Json serialization that's all, cause I'm new to this topic you know.
well, the important bit is that its a string
so you can do whatever you can do with strings
so you can easily write/read it to/from files
Ok!You're right. That makes very sense.
I could store the data even in database too, but since it's a console application project, I'll just use it with a text file.
you could, but for databases its usually a better idea to actually save the data as is, not serialized as json
Oh! Really. Didn't know that. Very interesting info.
databases dont store strings as such, they store things in a very different way
Ok! No idea how databeses like SQL or other types work yet.
I'm going through this post explaining how to write to Json file https://www.techiedelight.com/write-to-json-file-csharp/
Admin
Techie Delight
Write to JSON file in C# | Techie Delight
This post will discuss how to write to JSON file in C#... You can use the JsonSerializer class with custom types to serialize from and deserialize into.
@pobiega
why?
a JSON file isnt special
its just a text file
Ok
Pobiega
well, the important bit is that its a string
Quoted by
<@105026391237480448> from #❔ Validating user input (click here)
React with ❌ to remove this embed.
this hasnt changed
its still just a string
so you can write it to a text file, just as you can with other strings. its convention to have the file extension be
.json
for a file containing json information thouI mean this is the examples that are shown in the docs are the basic way fo writing to a text file, but in my case I have an object, should I pass it as a path? https://learn.microsoft.com/en-us/troubleshoot/developer/visualstudio/csharp/language-compilers/read-write-text-file
Read from and write to a text file by Visual C# - C#
This article describes how to read from and write to a text file by using Visual C#. This article also provides some sample steps to explain related information.
As you can see in this example a file path is passed as an argument, but in my case I have an serialized object.
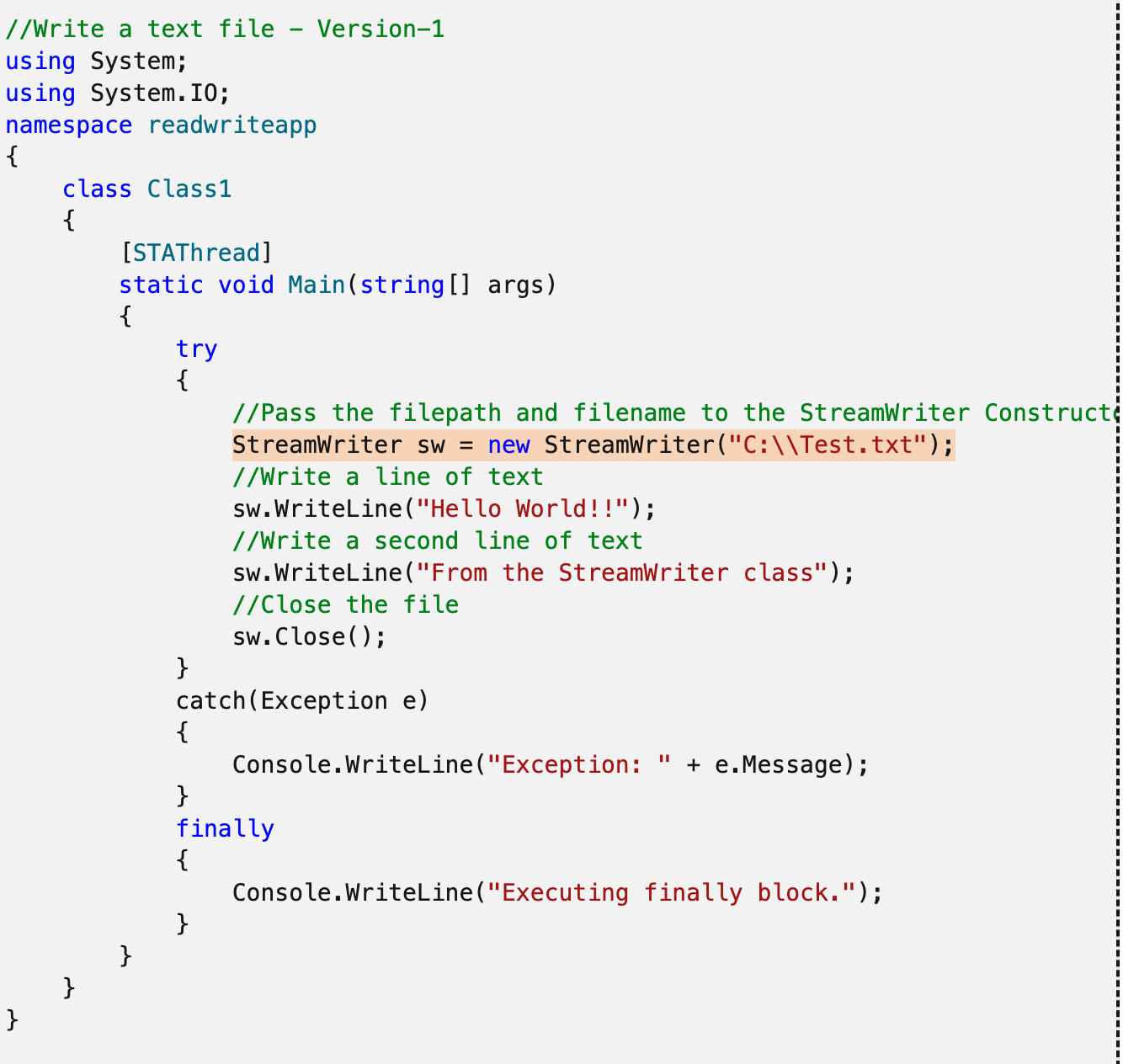
Should I pass it as an argument to be able to write to a text file?
You should probably be using this: https://learn.microsoft.com/en-us/dotnet/api/system.io.file.writealltext?view=net-7.0
File.WriteAllText Method (System.IO)
Creates a new file, write the contents to the file, and then closes the file. If the target file already exists, it is overwritten.
Ok! Awesome.
Yeah! I noticed that we have this method
File.WriteAllText
where we could write all strings at once.no, not "all strings"
it takes in a single string
or well, it takes two strings. one as path, one as content
Ok
I should read it all then
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.+
?
I just put a symbol so the thread won't be gone that's all 🤗
What do you think?
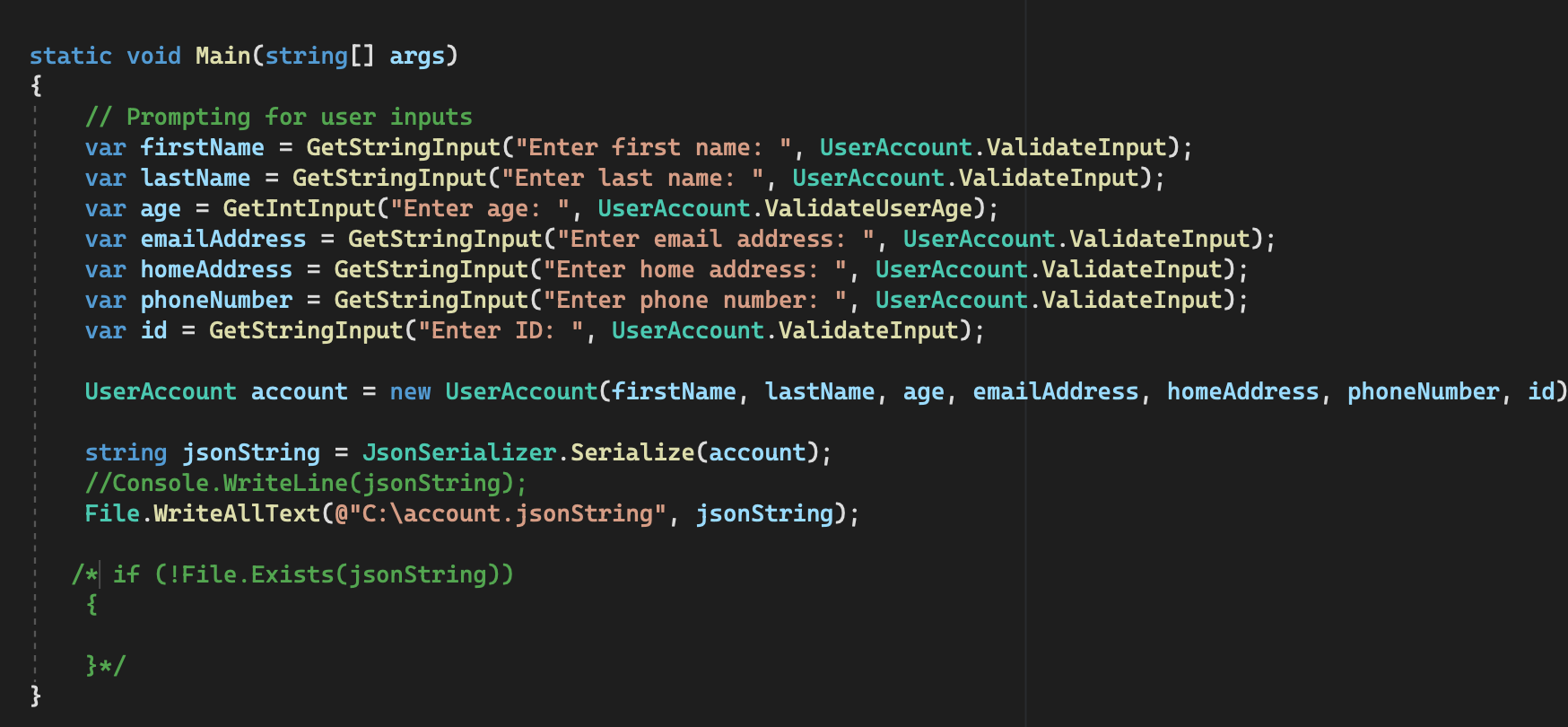
almost
why hardcode the absolute file path thou? and why
jsonString
instead of json
as your file extensionOK! That's a good point. You're right. The docs says it creates a new file, so we don't need to hardcode a path.
why would we need to hardcode a file if it didnt?
So I could use only json?
?
If it didn't exist youu mean?
yes
I was able to write it in this form following a tutorial
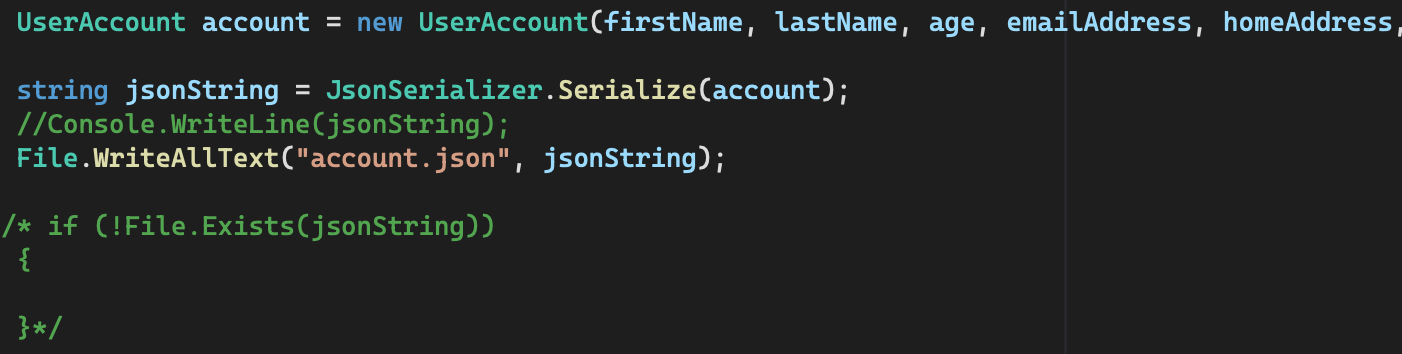
This docs is showing the very basic of writing to a file in my case I have an object and I shouldn't be hardcoding the path like it's shown in the examples.
Am I doing it correct now?
yes
Ok! Awesome man thanks a lot.
I'll continue then
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Hey man
Now that I'm done with writing all, next step is to read all text from a text file, right?
I guess I should be reading this docs https://learn.microsoft.com/en-us/dotnet/api/system.io.file.readalltext?view=net-7.0
File.ReadAllText Method (System.IO)
Opens a text file, reads all the text in the file into a string, and then closes the file.
sure
So currently it looks like this
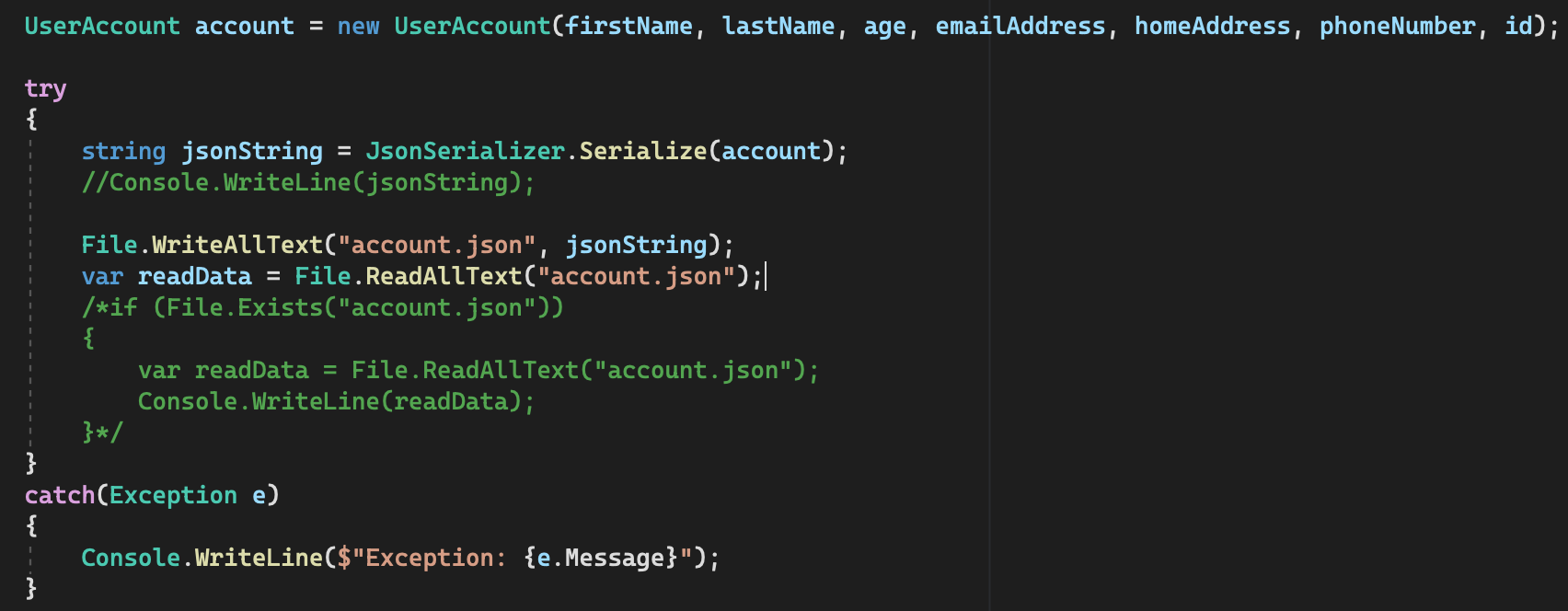
but why
read out the logic to me, spell it out
First I'm writing data that are converted to a jason string to a text file
Mhm.
and then reading all the data and store them in the readData variable
and this seems logical and sound to you?
Having data, saving it, then reading it again?
I'm using the try catch exception if the data doesn't exist
how is that possible? you save before you read, so it will always be there
Well! It's just a practicing reading and writing that's all.
Really
.. Sure, but think about when it makes sense to read and write
What is the entire point of saving the useraccount on disk?
No point what so ever
I think I could've practiced writing and reading in a different project and different context
And not only that, I should be separating the user accounts, so they're not mixed altogether, so it would get complicated.
Even when reading the data lines, they should be separated with a delimiter like # , "
Although the docs example is doing the same, doesn't it?
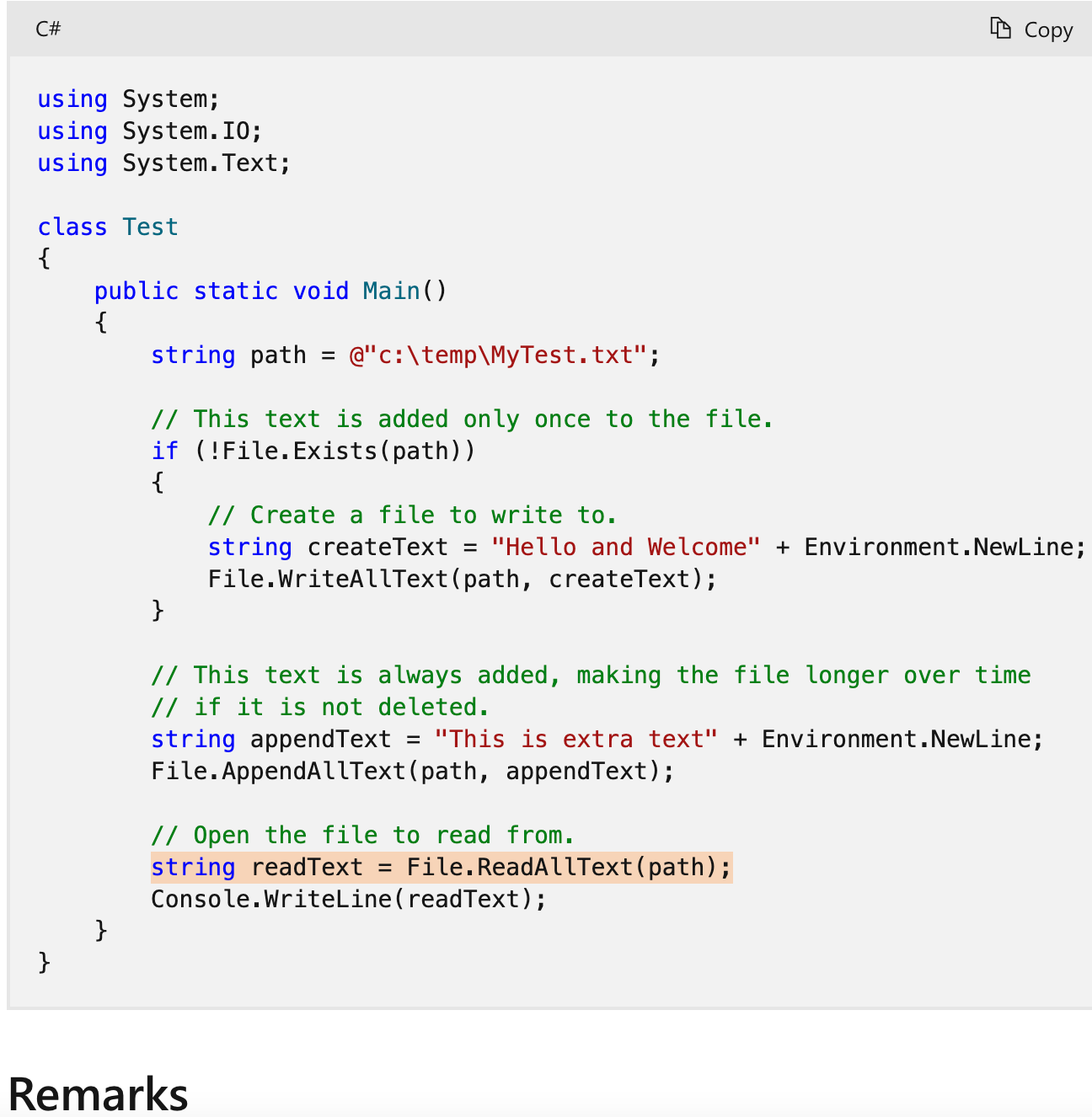
but thats not a real world app
its just a testprogram with no other purpose
You mean the docs example?
yes.
Yeah! You're right. They're just showing how to write & read.
So what you suggest I should be using?
Give up reading or?
no, but instead of randomly applying "reading and writing files" to a random project, how about applying them in a way that makes sense?
like, saving the users entered information and loading it from the file instead of asking the user to enter it again, if the file exists
Ok
if it didnt exist, ask the user and save the file
You really need to practice connecting the dots in your applications
so why not do just that
Ok
I try to get exactly what you mean, but it's a bit unclear to me?
Could you split what you mentioned into multiple steps or lines?
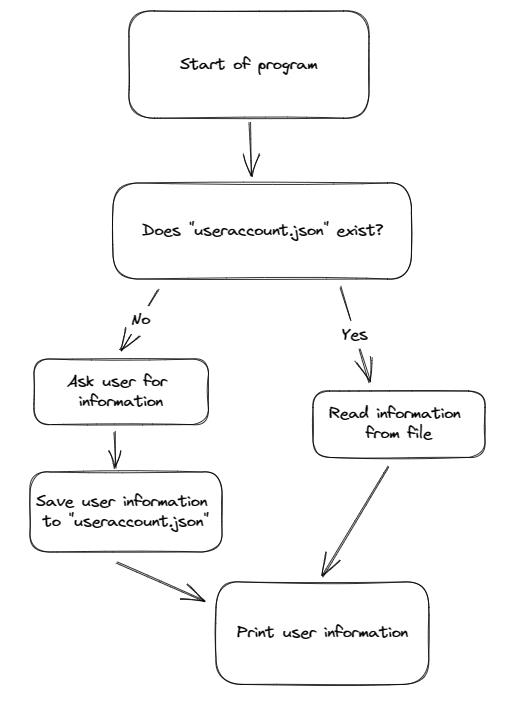
@Faraj does that make sense?
ngl, feels a bit rude to not even say anything when I went through the effort of actually making a flowchart for you
Sorry, I was sleepy last night when you made this flowchart.
Well! It does make sense to me, although I wonder why we should ask if the file does exist or not? Because Write all method creates a file and writes the data to a file, why should I ask then?
there is no asking
and if you READ THE FLOWCHART
where is the saving taking place?
Sorry, I meant
Does bla bla bla exist?
read the flowchart
where is the saving taking place?
It's right after taking user inputs
FARAJ
BASIC READING
What did I write?! TWICE
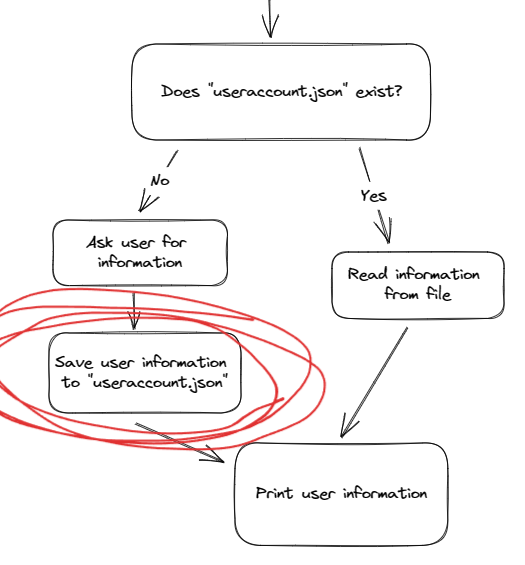
Well! This is what I meant too. But maybe I was wrong by showing you the code block.
Look at the flowchart
Look where save takes place
notice that its ONLY in the "no" branch of "does useraccount.json exist"
Yeah! That's right.
so we are no longer always saving
we've changed things around
Only if the file does NOT exist do we ask for information and save it to a file
<:picard_facepalm:616692703685509130>
I'm at a loss here Faraj
This is like.. extreme beginner level understanding
Ok
After reviewing your flowchart and thinking more deeply about it, I think it does not make sense.
How can we save user information if we don't even have a json file from the first place
O_O
What do you think saving does?
This is the current output that I'm getting
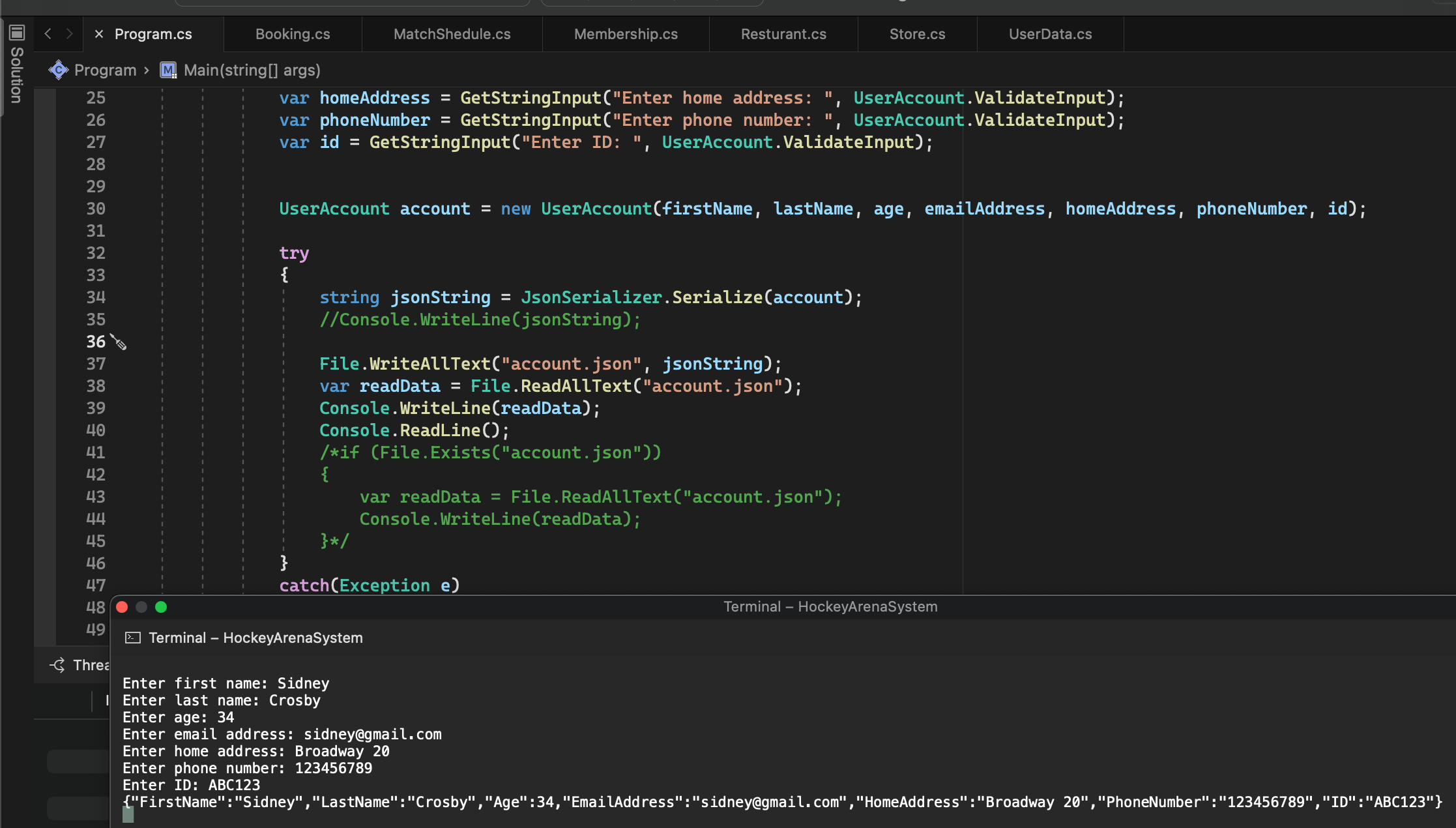
yes?
how is this relevant
Shouldn't this be writing all data to a json file?
we were talking about restructuring your program
I just wanted to show you the output that's all
Yes
so take your current code and break it up into smaller methods
its all in Main atm, which is bad
then in your blank and clean main, implement the flow according to the above flowchart
We should be saving in the json file
Ok
You mean I should be using these blocks inside a methods and then only calling them in the main method?
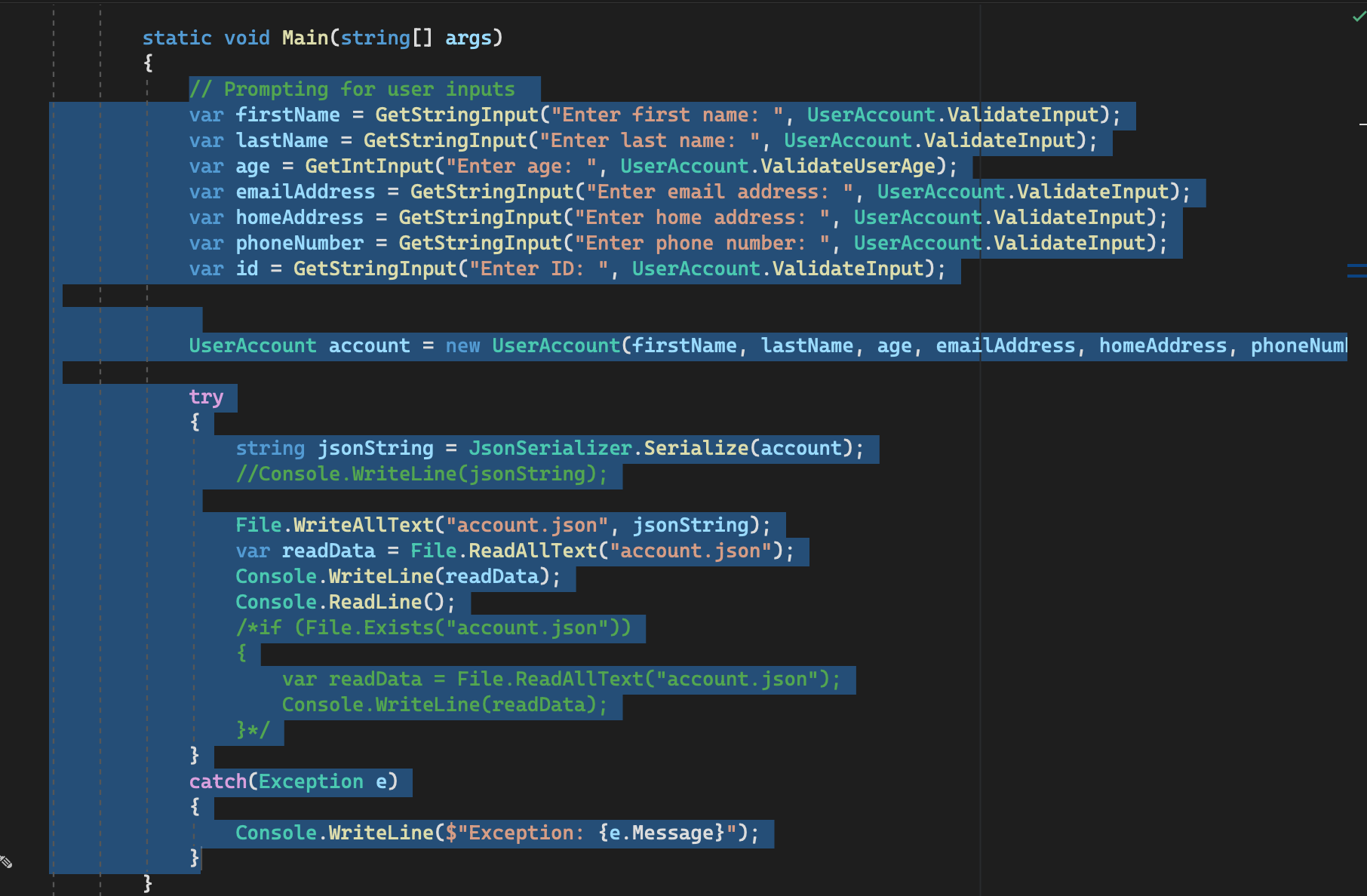
you should turn that code into methods that do distinct things
Ok
one that makes a user account, one that loads a user account from a file, one that saves a useraccount to a file
etc
Ok! By "load" you mean reading a text file? and by "save" you mean writing to a text file?
no shit?
of course thats what I mean
😁
Ok! Just making sure I get it right that's all lol.
Do you think I could define these methods here in this class?
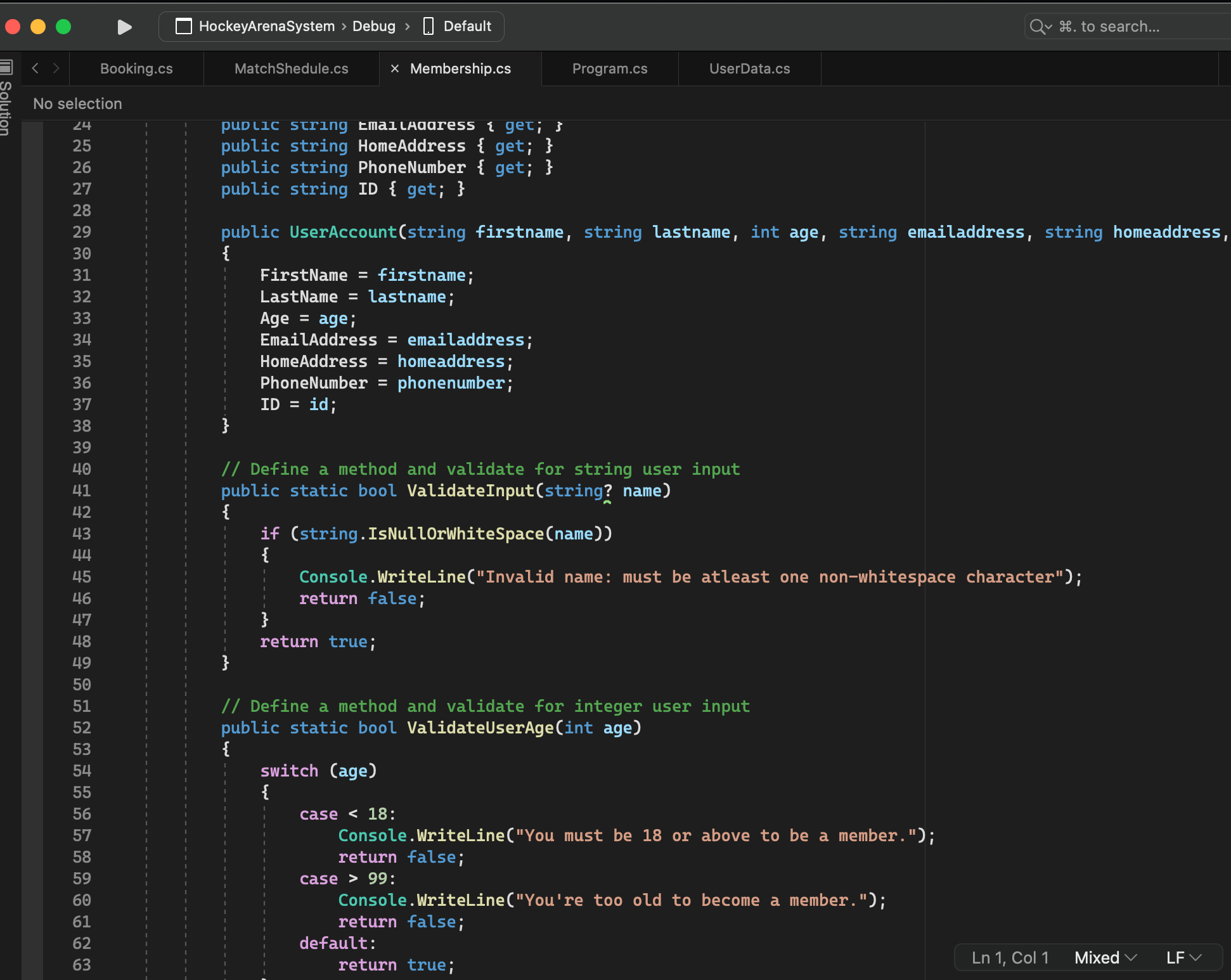
no
Is this bad too?
I think they should be in your
Program
classAha! Ok! Just outside the main method.
yes, because you shouldn't be writing huge methods that do many things
composition is important
Ok! Interesting information.
Just wanna check this with you, these methods should be static and return, right?
every method you ever write returns, asking if it should return doesn't make sense
just try it and see
Ok! But sometimes I try to find out what exactly should I return particularly in cases where I need to make a user account.
so you should know the answer to that question
return whatever you need that method to give you
OK
Let me figure it out
It seems confusing to me. Those blocks are attached to each other, I can't define them in a three separated methods.
they are related, not attached
I mean for example if I write the logic of making user account inside a method, how do I reference the variables when reading & writing from outside the block?
Yes
you don't, you return whatever data is needed elsewhere in the code
if you're making a user account in the method, you shouldn't need to access individual variables anywhere else
you just return the account that was created
same for loading, you read in the json file and return the user account that you deserialized from it
the rest of the program shouldn't be concerned with exactly how it's done
If the variable is declared inside a method block, I won't be able to use it other wise I'll get error saying there's no variable declared bla bla bla
yes
that is correct
Yes
you don't want to access the internals of a method, that defeats the purpose
the method should return the data you need
you're taking a complex operation like getting all the inputs and creating an account and representing it as a self contained unit that the rest of your code can use without knowing exactly what's happening
so whatever you're trying to access that's inside the method, return it
Aha! Ok! So this is the context that I should follow in order to implement each logic in a separated methods, very interesting to learn new stuff a long the way.
that's the whole purpose of methods
like i've said many times, you break the problem down into smaller pieces and solve them individually
that whole process leads to you developing different classes and methods that solve those smaller problems
OK
It's clear to me now. I'll use this method by breaking the whole process into small steps then.
The first step would be creating the user account in a method and returning the created user account
Currently, I'm backing off to re-learn how return type methods, using parameters and arguments in methods following this tutorial. I need to go back and learn the fundamentals again, so I can have a good solid foundation of the basic concepts. https://learn.microsoft.com/en-us/training/modules/write-first-c-sharp-method/2-understand-syntax-of-methods
Understand the syntax of methods - Training
Understand the syntax of methods
Plus following along this tutorial too https://learn.microsoft.com/en-us/shows/csharp-fundamentals-for-absolute-beginners/defining-and-calling-methods
Defining and Calling Methods
Create a helper method, create and call methods to retrieve a value, create and use input parameters, learn about string formatting, and create overloaded methods.
Methods are very important and considered as essential concept OOP
ignore OOP, they are probably the single most important concept in all of programming
Be it FP, OOP, procedural or what have you
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity..
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity..
just make a new thread the next time you have a question
i don't see the point in keeping this one open forever, nobody new is going to find it and it's far off the original topic
Yeah! You're right. I'll just dismiss this thread and just create a new one for new questions. The reason why I kept it, because I was going back here and re-read the useful info you guys wrote. But I could just take notes too.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.