❔ Mapping error
Hello, I have a crud application in ASP.NET and I need to add a new selectList, the options come from the db, on the add screen I managed to implement it smoothly, but on the update screen it breaks my application claiming that it cannot map, but my AddVehicleDto is the same as my UpdateVehicleDto, apart from the ID of course, and it only complains about this new attribute that I include,
my mappers:
// code here
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Ambipar.Response.Modules.Vehicles.Shared.Dtos;
using AutoMapper;
namespace Ambipar.Response.Modules.Vehicles.Application.Mappers
{
public class DomainToDtoMappingProfile : Profile
{
public DomainToDtoMappingProfile()
{
CreateMap<Vehicle, UpdateVehicleDto>()
.ForMember(dest => dest.Id, m => m.MapFrom(src => src.Id));
CreateMap<Vehicle, PagedVehicleDto>()
.ForMember(dest => dest.Id, m => m.MapFrom(src => src.Id));
}
}
}
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Ambipar.Response.Modules.Vehicles.Shared.Dtos;
using AutoMapper;
namespace Ambipar.Response.Modules.Vehicles.Application.Mappers
{
public class DtoToDomainMappingProfile : Profile
{
public DtoToDomainMappingProfile()
{
CreateMap<AddVehicleDto, Vehicle>();
CreateMap<UpdateVehicleDto, Vehicle>();
}
}
}
// code here
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Ambipar.Response.Modules.Vehicles.Shared.Dtos;
using AutoMapper;
namespace Ambipar.Response.Modules.Vehicles.Application.Mappers
{
public class DomainToDtoMappingProfile : Profile
{
public DomainToDtoMappingProfile()
{
CreateMap<Vehicle, UpdateVehicleDto>()
.ForMember(dest => dest.Id, m => m.MapFrom(src => src.Id));
CreateMap<Vehicle, PagedVehicleDto>()
.ForMember(dest => dest.Id, m => m.MapFrom(src => src.Id));
}
}
}
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Ambipar.Response.Modules.Vehicles.Shared.Dtos;
using AutoMapper;
namespace Ambipar.Response.Modules.Vehicles.Application.Mappers
{
public class DtoToDomainMappingProfile : Profile
{
public DtoToDomainMappingProfile()
{
CreateMap<AddVehicleDto, Vehicle>();
CreateMap<UpdateVehicleDto, Vehicle>();
}
}
}
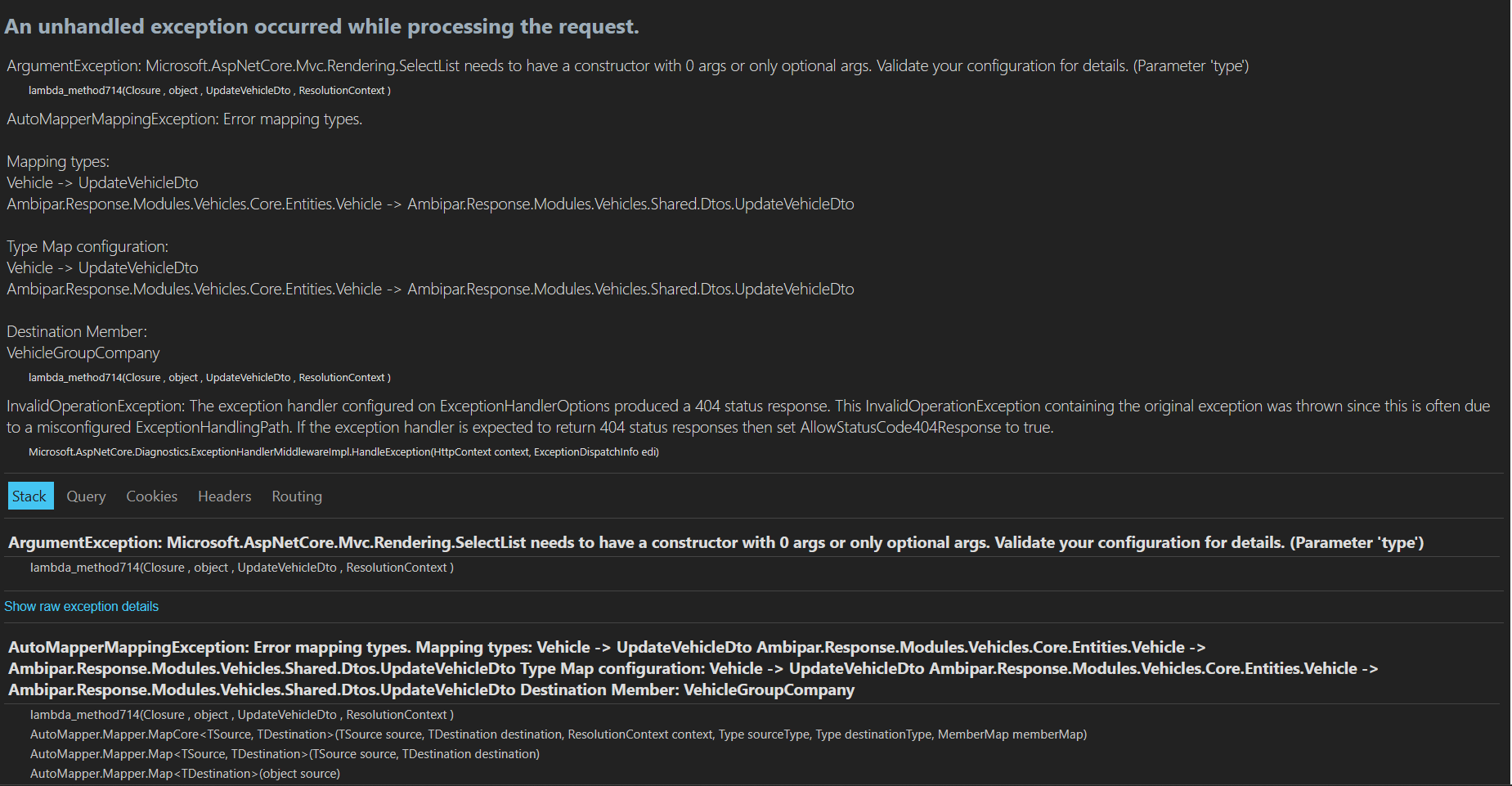
6 Replies
UpdateVehicleDto class:
Vehicle class:
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using System.ComponentModel.DataAnnotations;
using System.Globalization;
using System.Security.Principal;
namespace Ambipar.Response.Modules.Vehicles.Shared.Dtos;
public class UpdateVehicleDto
{
public Guid Id { get; set; }
public bool Active { get; set; }
public bool Maintenance { get; set; }
public bool Tracked { get; set; }
public string HasTrackingSystem { get; set; }
public string TrackingSystemName { get; set; }
public string Renavam { get; set; }
public string LicensePlate { get; set; }
public Guid VehicleCategoryId { get; set; }
public Guid VehicleFuelTypeId { get; set; }
public Guid VehicleGroupId { get; set; }
public Guid VehicleBrandId { get; set; }
public Guid VehicleModelId { get; set; }
public Guid VehicleOperationId { get; set; }
public VehicleOperation VehicleOperation { get; set; }
public Guid WorkBaseId { get; set; }
public int? ManufacturingYear { get; set; }
public int? ModelYear { get; set; }
public Guid BusinessUnityId { get; set; } = new Guid("e39ac9b5-652b-4838-a432-924cbfa1e9fb");
public SelectList GroupCompany { get; set; }
public Guid VehicleGroupCompanyId { get; set; }
public SelectList VehicleGroupCompany { get; set; }
public SelectList BusinessUnity { get; set; }
public SelectList Category { get; set; }
public string Type { get; set; }
public SelectList FuelType { get; set; }
public SelectList Group { get; set; }
public SelectList Operation { get; set; }
public SelectList Brand { get; set; }
public SelectList Model { get; set; }
public string FleetNumber { get; set; }
public string Chassis { get; set; }
public string HasSupplyTank { get; set; }
public string SupplyTankCapacity { get; set; }
}
using Ambipar.Response.Modules.Vehicles.Core.Entities;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using System.ComponentModel.DataAnnotations;
using System.Globalization;
using System.Security.Principal;
namespace Ambipar.Response.Modules.Vehicles.Shared.Dtos;
public class UpdateVehicleDto
{
public Guid Id { get; set; }
public bool Active { get; set; }
public bool Maintenance { get; set; }
public bool Tracked { get; set; }
public string HasTrackingSystem { get; set; }
public string TrackingSystemName { get; set; }
public string Renavam { get; set; }
public string LicensePlate { get; set; }
public Guid VehicleCategoryId { get; set; }
public Guid VehicleFuelTypeId { get; set; }
public Guid VehicleGroupId { get; set; }
public Guid VehicleBrandId { get; set; }
public Guid VehicleModelId { get; set; }
public Guid VehicleOperationId { get; set; }
public VehicleOperation VehicleOperation { get; set; }
public Guid WorkBaseId { get; set; }
public int? ManufacturingYear { get; set; }
public int? ModelYear { get; set; }
public Guid BusinessUnityId { get; set; } = new Guid("e39ac9b5-652b-4838-a432-924cbfa1e9fb");
public SelectList GroupCompany { get; set; }
public Guid VehicleGroupCompanyId { get; set; }
public SelectList VehicleGroupCompany { get; set; }
public SelectList BusinessUnity { get; set; }
public SelectList Category { get; set; }
public string Type { get; set; }
public SelectList FuelType { get; set; }
public SelectList Group { get; set; }
public SelectList Operation { get; set; }
public SelectList Brand { get; set; }
public SelectList Model { get; set; }
public string FleetNumber { get; set; }
public string Chassis { get; set; }
public string HasSupplyTank { get; set; }
public string SupplyTankCapacity { get; set; }
}
using Ambipar.Response.PortalServices.Shared.Entities;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace Ambipar.Response.Modules.Vehicles.Core.Entities
{
public class Vehicle : BaseEntity<Vehicle>
{
public bool Active { get; set; }
public bool Maintenance { get; set; }
public bool Tracked { get; set; }
public string HasTrackingSystem { get; set; }
public string TrackingSystemName { get; set; }
public string Renavam { get; set; }
public string LicensePlate { get; set; }
public int? ManufacturingYear { get; set; }
public int? ModelYear { get; set; }
public Guid BusinessUnityId { get; set; }
public Guid WorkBaseId { get; set; }
public string Type { get; set; }
public Guid VehicleCategoryId { get; set; }
public VehicleCategory VehicleCategory { get; set; }
public Guid VehicleFuelTypeId { get; set; }
public VehicleFuelType VehicleFuelType { get; set; }
public Guid VehicleGroupId { get; set; }
public VehicleGroup VehicleGroup { get; set; }
public Guid VehicleOperationId { get; set; }
public VehicleOperation VehicleOperation { get; set; }
public Guid VehicleModelId { get; set; }
public VehicleModel VehicleModel { get; set; }
public Guid VehicleBrandId { get; set; }
public VehicleBrand VehicleBrand { get; set; }
public Guid VehicleGroupCompanyId { get; set; }
public VehicleGroupCompany VehicleGroupCompany { get; set; }
public string FleetNumber { get; set; }
public string Chassis { get; set; }
public string HasSupplyTank { get; set; }
public string SupplyTankCapacity { get; set; }
}
}
using Ambipar.Response.PortalServices.Shared.Entities;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace Ambipar.Response.Modules.Vehicles.Core.Entities
{
public class Vehicle : BaseEntity<Vehicle>
{
public bool Active { get; set; }
public bool Maintenance { get; set; }
public bool Tracked { get; set; }
public string HasTrackingSystem { get; set; }
public string TrackingSystemName { get; set; }
public string Renavam { get; set; }
public string LicensePlate { get; set; }
public int? ManufacturingYear { get; set; }
public int? ModelYear { get; set; }
public Guid BusinessUnityId { get; set; }
public Guid WorkBaseId { get; set; }
public string Type { get; set; }
public Guid VehicleCategoryId { get; set; }
public VehicleCategory VehicleCategory { get; set; }
public Guid VehicleFuelTypeId { get; set; }
public VehicleFuelType VehicleFuelType { get; set; }
public Guid VehicleGroupId { get; set; }
public VehicleGroup VehicleGroup { get; set; }
public Guid VehicleOperationId { get; set; }
public VehicleOperation VehicleOperation { get; set; }
public Guid VehicleModelId { get; set; }
public VehicleModel VehicleModel { get; set; }
public Guid VehicleBrandId { get; set; }
public VehicleBrand VehicleBrand { get; set; }
public Guid VehicleGroupCompanyId { get; set; }
public VehicleGroupCompany VehicleGroupCompany { get; set; }
public string FleetNumber { get; set; }
public string Chassis { get; set; }
public string HasSupplyTank { get; set; }
public string SupplyTankCapacity { get; set; }
}
}
please edit your post to format the code.
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/fixed
yeah, so it seems automapper cannot default create any property on UpdateVehicleDto that is
SelectList
due to the lack of a default constructor.Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.