❔ Hello im making a recording software for fun and my code isnt really working
so im using visual studio 2022 and i use .net framework 4.7.2 and im trying to make it record for 5 seconds then open recording after that but litteraly nothing happens when i press the button and i have no idea why
51 Replies
Debug it, go step by step
how? im a newbie
even when i make new projects buttons do nothing i can do nothing nothing works
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
i dont understand much of it can you help?
Suggest you look up a tutorial. Debugging is a critical skill
whats debuggin used for?
To figure out problems in your code
but is it in my code or just my visual studio? cus the button should say "hej" right?
Why should it show that? You make a message box with that message. Not any button
but its in the button1_click
Yes
so it should say hej when pressed right?
The message box should show, yes
it doesnt
Then you didn't connect the event handler to the event, I guess
what...
whats that supposed to mean?
Sorry don't have time to dig into the basics. If you're following a tutorial then I suggest to retrace your steps
im not really following a tutorial
when i make a even that button dont work
The button exposes events. You '+=' on the event to add handlers, ie your button1_click
Are you just writing these methods?
no i also add to design the form1.cs
Show more details about that, maybe someone can help with it
I hate winforms and stay away from it
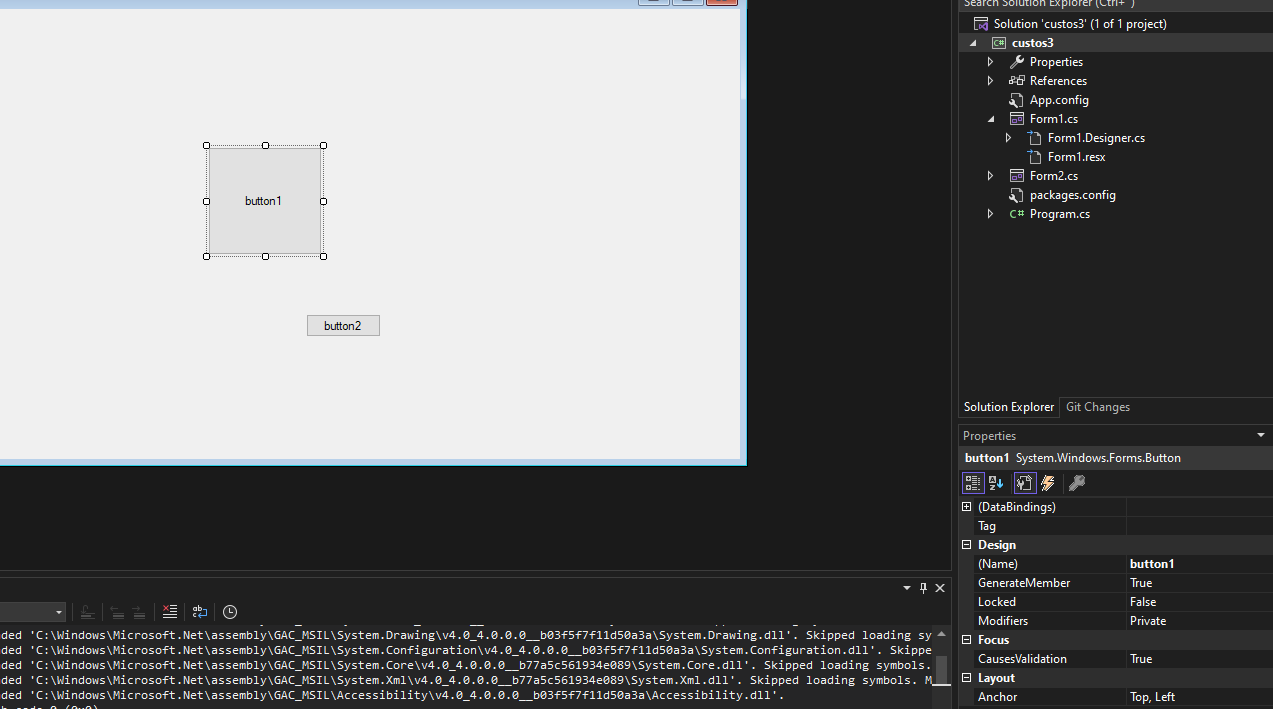
why?
is there a easier way of making windows applications?
Yeah, but where is the button event handler connected to the button?
uuh
how do i do that
when i press on view code it puts me to form1.cs where the other code is
Tell me how the button click method were created?
You wrote it? Or it was generated?
i dragged it in from toolbox and wrote code
Idk. Click the lightning bolt, what shows?
i havent changed anything in the propertys
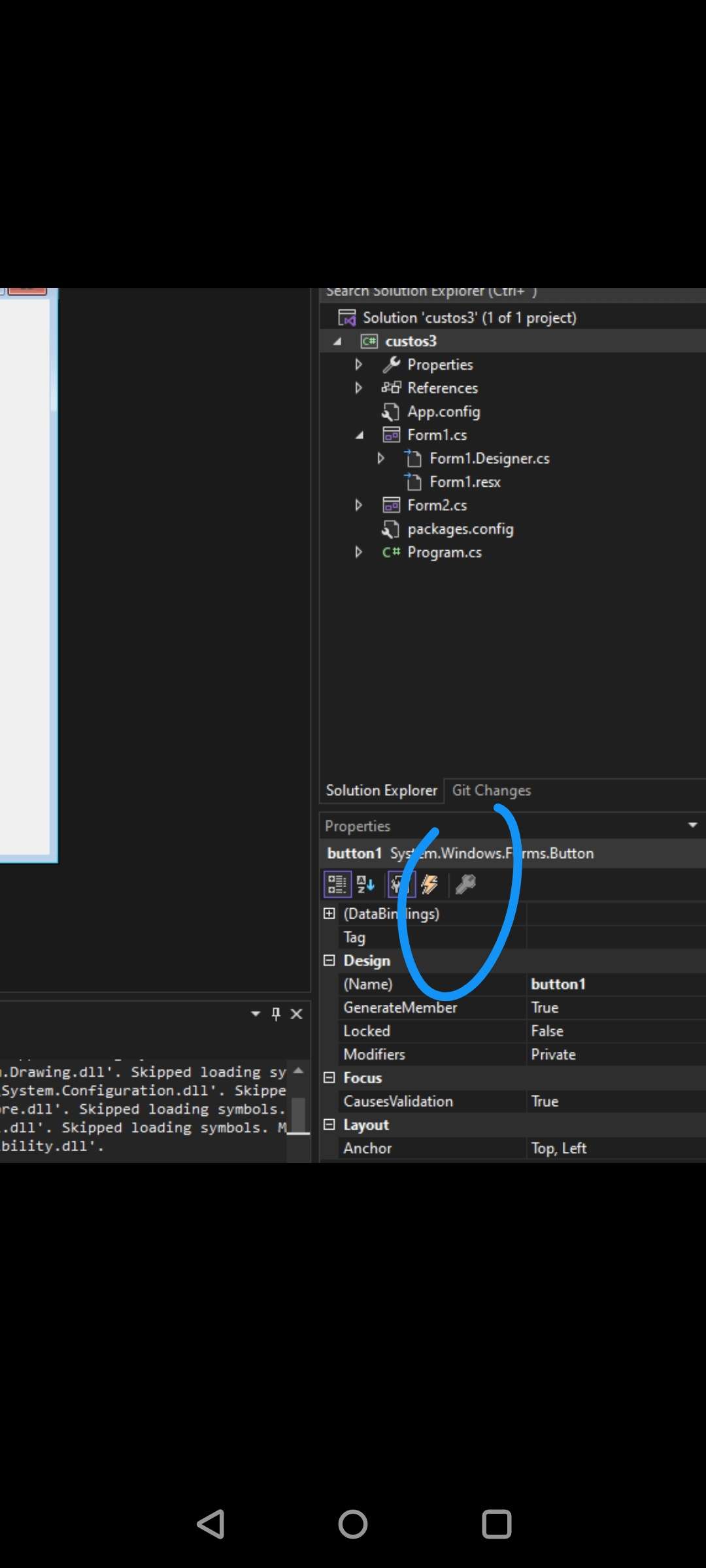
if ya click on that u see the events
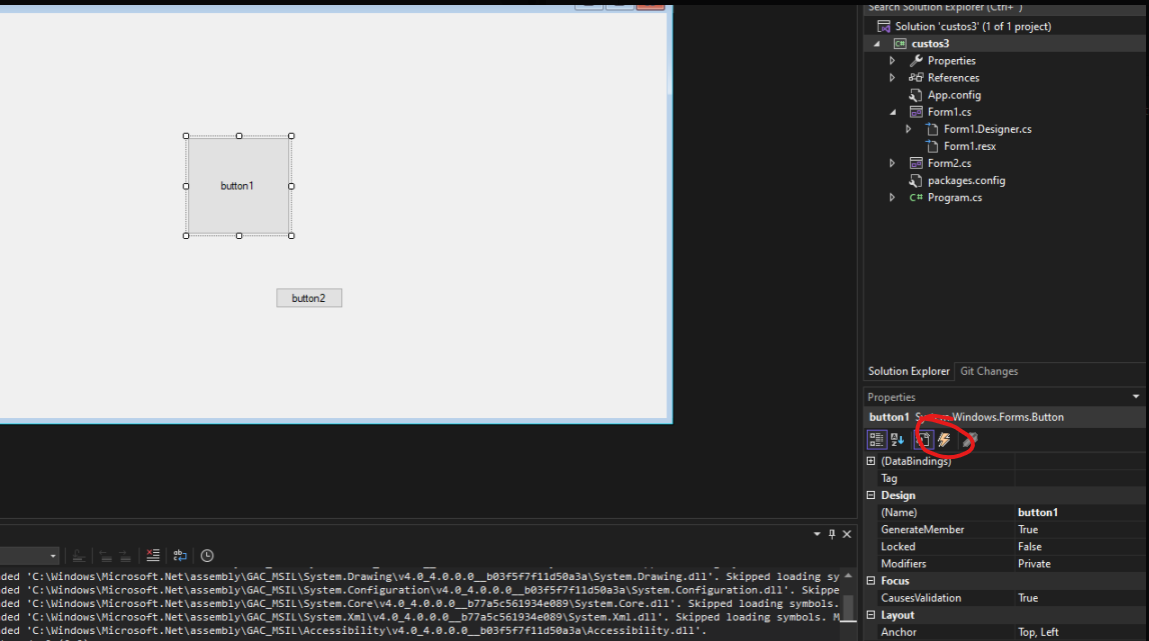
for that button the currently selected element
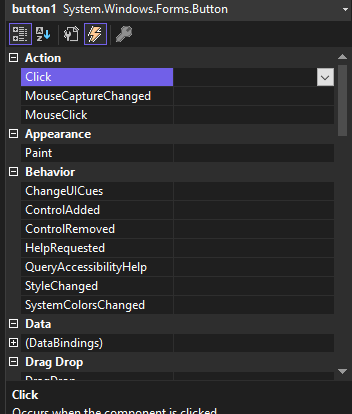
should i change something?
yeah, u would have to set it to ur button1_Click event handler method
There's no events handler set...
god damn.
?
this is so usefull
ok new error
after i run it it says exception unhandled at button.enabled = true;
would be good to know which exception
System.InvalidOperationException: 'Cross-thread operation not valid: Control 'button1' accessed from a thread other than the thread it was created on.'
yeah u cant update GUIs from outside their own thread
wich means?
i have to move the code?
to the thread
well, if u dont do the recording inside its own thread, the whole window would hang for that time
oh.
how do i fix
usually GUI frameworks provide some way to dispatch some callback to its GUI thread
dunno how its called in winforms tho
https://stackoverflow.com/questions/11211533/update-a-control-in-ui-with-running-background-thread-in-winforms#answer-11212222 seems to be the way
Stack Overflow
update a control in UI with running background Thread in Winforms
I am using Background Worker Thread in my Winform, inside my Do_Work Event I am calculating something, what I need the thing is that simultaneously I would like to update a label which is in main/UI
cap5slut how do i make it so the playbackspeed isnt sped up when i change framerate?
dunno i never worked with videos/recording at all
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.