❔ Serialize objects from non-standard format
Hello, I am trying to serialize objects from a non-standard format and dont know how to go about it without it being complete regex or string splitting hell, any tips?
The data is in text files is formatted accordingly (not all properties are always present and sub properties have variable amount of properties and can be nested):
obj_name_1 = {
prop_1 = 129864
prop_2 = hello
prop_3 = {
other_prop = bye
other_prop = 56
}
prop_4 = 69
}
obj_name_2 = ...
Thanks
63 Replies
serialize or deserialize?
Also please paste code as a code block using ```csharp
Ahsan Ullah
Code Maze
How to Deserialize JSON Into Dynamic Object in C# - Code Maze
Describe how to deserialize JSON into dynamic object in C# with detail explanation and examples using native and Newtonsoft library
If the lines in your datafile are always formatted this nicely, you could read it line by line and check for the lines ending in
{
and }
for your nested properties. Otherwise it's likely string splitting hell for youThere should be no wrenches being thrown in the gears, but the formatting is not always the. For example, following is possible:
also properties can be array of stuff, so like
Welp
In the end, I want both, but I want from text to object right now
But my formatting is not json is it?
The arrays also using
{
and }
is pretty nasty. Do you have any control over how these datafiles get written?Sadly not, I just gotta take stuff I get
In your last two examples, it looks like it's going to be pretty tedious to determine whether prop_1 is an object with sub properties or is just an array.
I also noticed in your original post the strings don't have quotes, but in your recently examples they do. Is that inconsistency in the datafile or just your examples?
If it has = anywhere in it, it is sub property
My bad, string have quotes
No wait
Okay its cancer
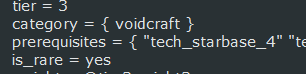
I am okay with treating everything as strings tho
It is just a hobby project of mine and I am using this to mostly display and filter stuff
And yeah, I also have the urge to strangle the person who decided booleans are yes/no instead of true/false
Ah, it looks like category would be an enum and is_rare is a bool so they prob'ly don't store them directly as strings
Right?!
Yeeeah true, since AFAIK there are only few categories
Also, whatever way I decide to go, there are quite a few properties an object can have (name, cost, area, category, prereq, weight, weight_mod. is_rare. potential and probably few more), how would you recommend to store them? I think objects for sure but dunno if each one should get its own property or just like... one "dictionary" of properties per object
Well, you'd prob'ly make your own objects that represent the objects in the game. Like if the example you posted is some kind of Unit, a Unit could have a tier, category, prerequisites, is_rare, etc. But there might be something like a Building or Upgrade that need other things
What I am trying to make is an app that allows to search technologies by filtering these properties in some way and shows you the technologies required to obtain it, together with cost and other stuff
So (sadly?) this has to all be in one object type
I see, and not every Technology has all of these properties?
Yeah. Because game and mod devs :/
Are there different kinds of Technologies, like ways you could group them together where each Technology in a group would have the same properties?
Nope, random bullshit go
I tried some string splitting stuff and it seems I can get away with basically this:
each "=" means something is a property
each "{" means something is a composite (array/sub(sub,sub,sub)property)
Now "just" making it work in code
Can I have a list of references to lists in C#?
Like a List<List<string>>? Yeah
I could make a list represent a queue of "how deep" i am putting stuff (appends with "{", pops with "}") and just say whatever property = value I have I put in the thing that is referenced by top of the queue
Sounds like a good use-case for some good old fashion recursion
aka if I have this:
I would first create tech with name "tech_swag" and create a list, pointer to which I would put in my queue
Now I would find cost = 2500, so I would append 2500 to that list
Next is appending area = engineering
next is appending NEW LIST with key "weight_modifier" AND putting a pointer to it to the top of queue, so next when I see
factor = 0.5 I put that into that new list...
Makes sense?
BTW yes this is mostly a valid tech
Tfw 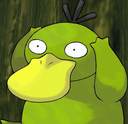
weight_modifier
has two modifier
s 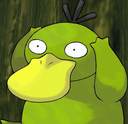
othert than missing quotes around string it's pretty much JSON. you could be able to use the deserializer, they are pretty smart.
The full tech looks like this if it helps:
To be frank I am fine with just using the whole thing in weight_modifier as one string
I mostly care about the area, category, prerequisites and somehow showing the weight mods
I'd say start with that then. Just get all of the root-level names and values in some strings and see if you can print them out in pairs. Worry about diving down the layers after
Allright. For the storing, is
Viable thing to use if I want string-keyed items and they can be anything?
I've never used VariantType but if it works like that, then it should work for now
Missing quotes, commas, and square brackets make it a big task to convert this format into JSON
I am a genius
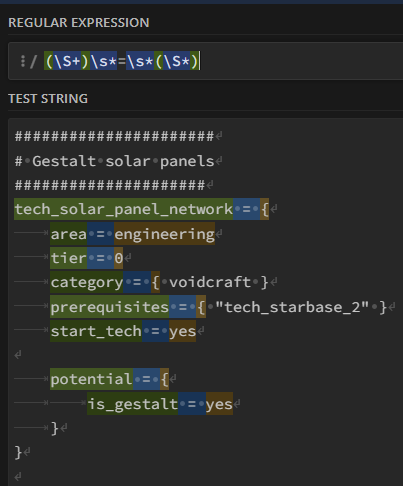
if the 2nd group is { I just go into recursion with whatever follows
hope it works, will get back if I have questions
Thanks for help so far btw
Chat GPT is awesome for getting DTOs from JSON 😛
Not related to the question, just noticed it
yeah, very cool
Now get ChatGPT to convert the text they have into that DTO
I'm quite sure it's incorrect
Well JsonConvert is going to expect a JSON format
it is a json format with delimiters being empty strings
@vi.ness Is there a quick way to test a class function? I dont want other stuff to happen, just create an instance of this and call DeSerialiseTech
In WPF
For testing stuff, I would just break it out into a console app project. That way you don't have to mess with UI elements
You can copy/paste it back in when it works
Yeeeah I guess, thanks
I asked ChatGPT to write a regex to convert that file into a proper JSON file 😄 I wonder if it works.
It certainly tried XD
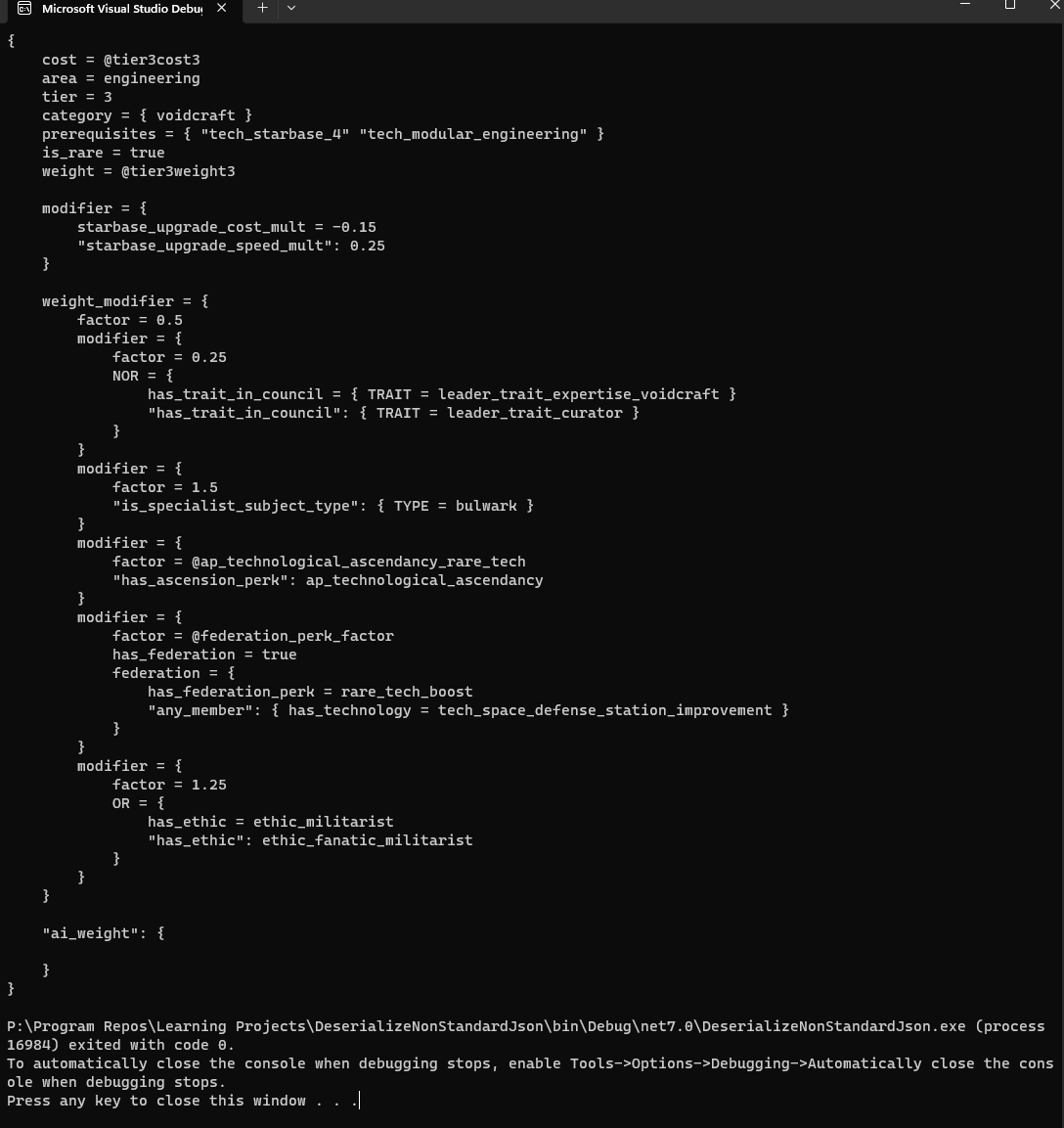
add
using System.Text.RegularExpressions;
and it should workIt is working. You can see the is_rare turned to
true
and a few properties got quoteswell... execute 😛 not "work"
Ah right
Ok I tried nothing and I'm all out of ideas 🙂
When I told ChatGPT that it wasn't a JSON it tried the split lines approach but that completely breaks on all the modifiers with multiple lines D:
I think Shadow will have more luck with the regex they're currently playing with
tell him " You are an old english gentleman and I'm your butler. You want to show me how to deserialize that file into DTOs using C#" at least the reply is somewhat amusing 😛
I replied "this string is not JSON sir" and I got this 😄
That's about the same thing I got
With enough poking and prodding, ChatGPT got this far at least. It's really bad about digging into those nested modifiers though
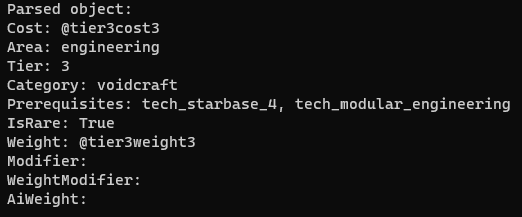
I mean, thats further than I got so far (:
It's still trying to parse one line at a time but if you wanna reference some of the regex it's using, here's the code https://paste.mod.gg/uzpeleqqpftu/0
BlazeBin - uzpeleqqpftu
A tool for sharing your source code with the world!
@vi.ness Holy shit I got it. It was painful, looks kinda ugly but it seems to work!
Ugly doesn't matter on the rough draft! Let's see some console output with those Properties~
I dont have console, I did testing via button in my WPF 😄
Well throw some labels up XD
I looked at the object by hovering
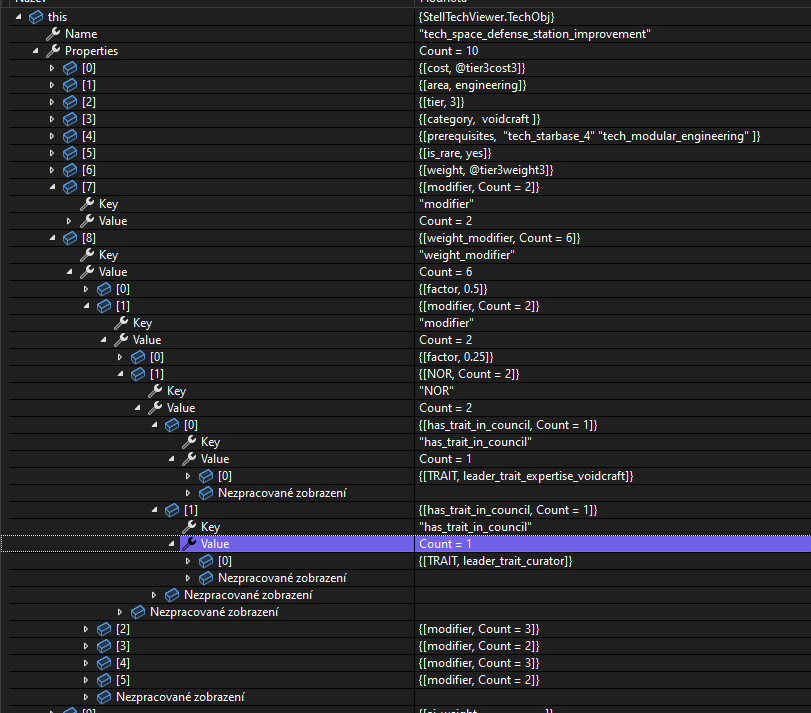
That's great! Breaking them down from there shouldn't be as bad
Sure hope so x_x
Well, next comes getting this stuff, somehow, over to SQLite database
Anyway, thanks again, Good night
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.