✅ Writing a scuffed way to use an "assignment" (=) operator
Obviously, there's no such thing as an assignment operator. I'd like to find some way around that.
I have a class that looks something like this:
I'd like to be able to assign values to this:
Is there any hacky, bodged, whatever way of doing this? I can't do it like this, obviously, because operators are static:
Any ideas are appreciated, thanks!
13 Replies
There is no such thing as assigment operator in C#
I mean, you can't modify it in any means
what would this be for 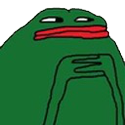
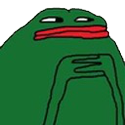
I just think it'd be nice not to type .Value everywhere, I use this type a lot.
Definitely not strictly necessary, just maybe a nice-to-have. It's just for brevity's sake.
yeah that doesn't make sense to me semantically
assigning a property of an object isn't the same as assigning a whole different object
In my case it doesn't really matter.
well, it matters to C# 😛
Also, just realized that I just typed class instead of struct.
Add an implicit conversion from double
For this particular case, it doesn't look like you actually have any extra state beyond
AField
If I'm misunderstand and you actually do have that state, then there is no wayThe implicit operator would have to deal with setting the AField because you could write something like
MyType<float> myType = 30.5f;
But it looks like they need to pass the AField into the constructor and use that when getting or setting ValueSuper duper bad coding practice. You'll make something that everyone (including future you) will assume is a float. This simply begs for a bug somewhere in the future.
Someone smart once wrote a rule for Python: "Explicit is better than implicit" this is also true in C#
What pattern is the
MyType
supposed to be in? Is it some sort of Guard? Because I don't see any other reason for it to look like that. Seems like the responsibilities are mixed.Also: https://xyproblem.info/
The XY Problem
Asking about your attempted solution rather than your actual problem
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.