getStaticProps & SSG helper data still being fetched on client.
Hello ya'll, my first time working on a new project using the T3 stack. Like it a lot so far.
I'm a bit stumped after trying to pre-fetch data for a static page though.
After looking through guides for SSG helpers, my assumption would be that the following code should work
I'm only going to need to revalidate my frontpage once per day for new jobs.
However, when deploying my app to Vercel, it's clearly not static.
I can see a network request made, and any new data in the db will be shown.
Appreciate any help or ideas 🙏
5 Replies
disabling the following did not do the trick either 🤔
const { data: jobs } = api.job.getAll.useQuery(undefined, { refetchOnMount: false, refetchOnWindowFocus: false })
Seems like adding Revalidate
did the trick...
I don't understand fully why though, as usually, if I did a good ol' normal fetch directly from the server it would be completely stale until I make a new deployment.
Oh well, I was planning to add a revalidate either way ! :3I think you're mixing the things
The requests always happen, what doesn't happen always is the update of the components, by default
You could try something like this
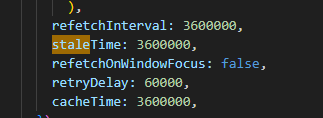
I'm doing something similar in this code^
It won't let RQ refetch until 1h has passed
but you'd do it to 24h
Hmm...yeah it's a bit confusing. Still trying to wrap my head around the dehydation and pre-fetching flow.
But, at least now when I added
revalidate
to GetStaticProps,
I don't see any request being made to the DB in the network tab at all, and it doesn't refetch any any new data.
So... seems to be working ?