Passing and Accessing Authentication Tokens from External Backend to Client-side Components in Next.
How can I pass the authentication token obtained from an external backend to client-side components in Next.js when using Next-Auth for authentication? I am currently using const session = await getServerSession(authOptions); in server-side components, but how can I access the session in client-side components?
dir app/api/auth/[...nextauth]
5 Replies
the code that i used for page.tsx
Client API | NextAuth.js
The NextAuth.js client library makes it easy to interact with sessions from React applications.
thanks for answering
i have a form which i am using "use client" how can i access the session token when i submit
maybe that can be done with provider but it provides session data inside network tab in browser inspect if it is possible to not show the session
the problem is i have to send token in headers each time i fetch and i dont like calling getServerSession on every page is there a better way to handle it ? @rocawear @ranthom-merge I maybe not asking the problem correctly i am pretty new to nextjs world
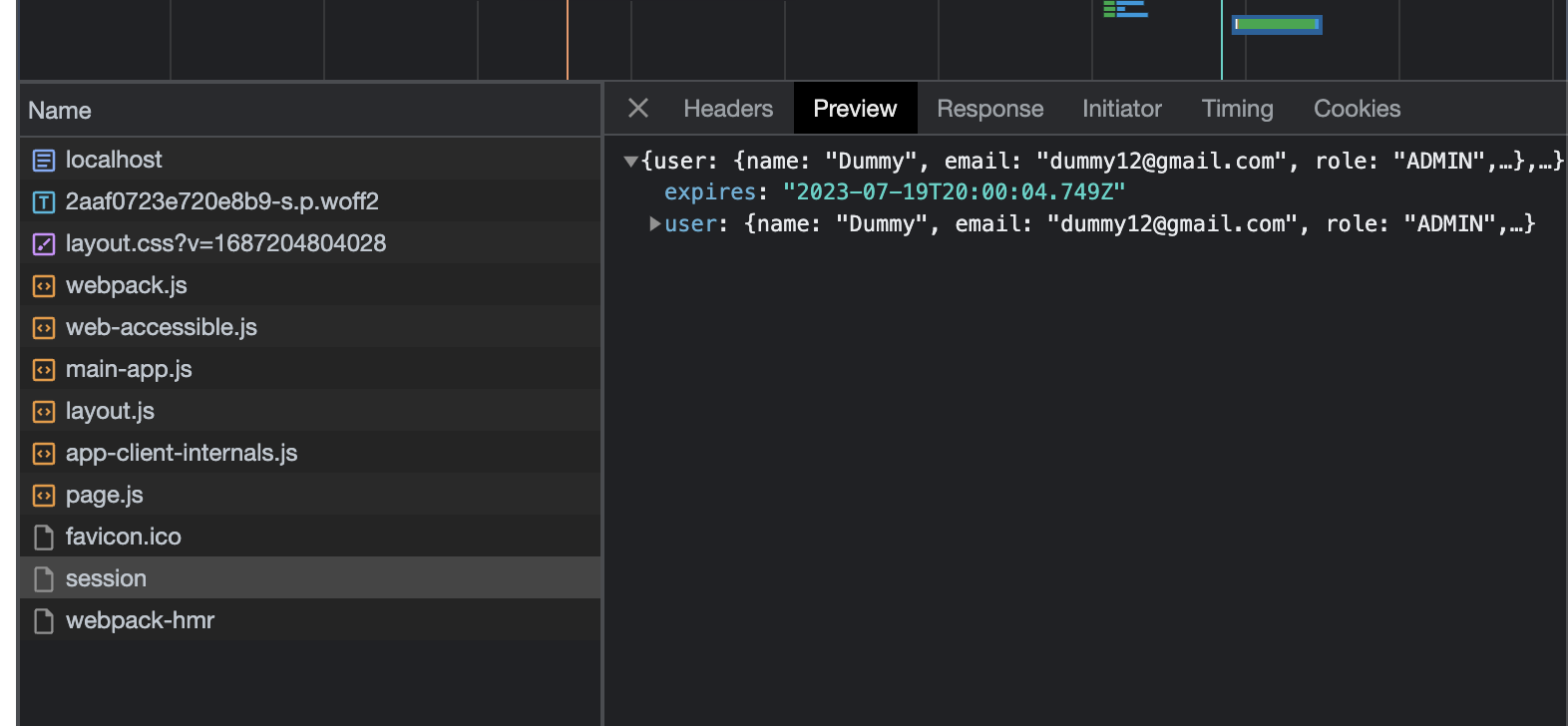
I would make function that adds token to it. Something like this:
i tried it but i think i need to handle some bits on client side and it is not possible to hide session
thanks you
i will try this
did not work that well but i will try figuring out this problem some other time thanks i am marking it as done