❔ Reading a process' memory - can't fix the issue
Hi guys,
For the last ~5 hours I've been trying to make a c# application, which will read a process memory and prints that in the console. Just that. Nothing big.
On the screenshot you can see the end-goal. I would like to see that value in the console. The problem is that the code I wrote doesn't work. Same thing with the help of chatGPT (gpt4) and many open-source copy&paste ideas I found on the internet. I simply have no idea what's wrong.
Code I am using:
I've already tried many other ways to handle that but the output is always the same.
I also have the python code...which actually works...and I have no idea why the hell the c# code doesn't work.
part of the python code:
Any ideas what's wrong?
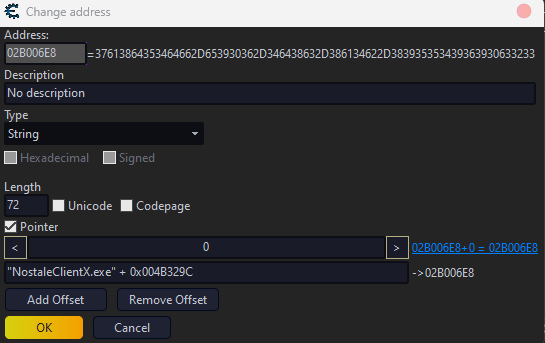
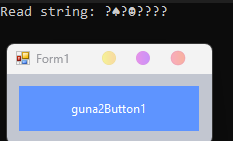
3 Replies
this looks way too close to cheating and/or breaking the game's TOS
i mean it's only reading
don't see anything wrong with that
but that
0x0
doesn't do what you think it does
if all it was were add 0
to the previous value, then what would even be the point in having it there
openprocess and closehandle are also unnecessary
the rpm pinvoke is technically not correct
and the fact that you're using winformsWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.