4 Replies
also how to check if a function (in this case
foo
) is recreated or not?ChatGPT:
More ChatGPT:
To check if a function is being recreated or not, you can add a console.log
statement inside the function and observe the logs in the browser's console. Here's an example:
When you run your React component with this code, you should see the message'foo function called'
logged in the console every time thefoo
function is executed. If the function is not recreated, you should see the message only once when the component is initially rendered.
If you see the message logged repeatedly, it means that the foo
function is being recreated on every render. However, if you see the message logged only once, it indicates that the function is correctly memoized and not recreated unnecessarily.
You may ask why all the ChatGPT... Simply because of this #2
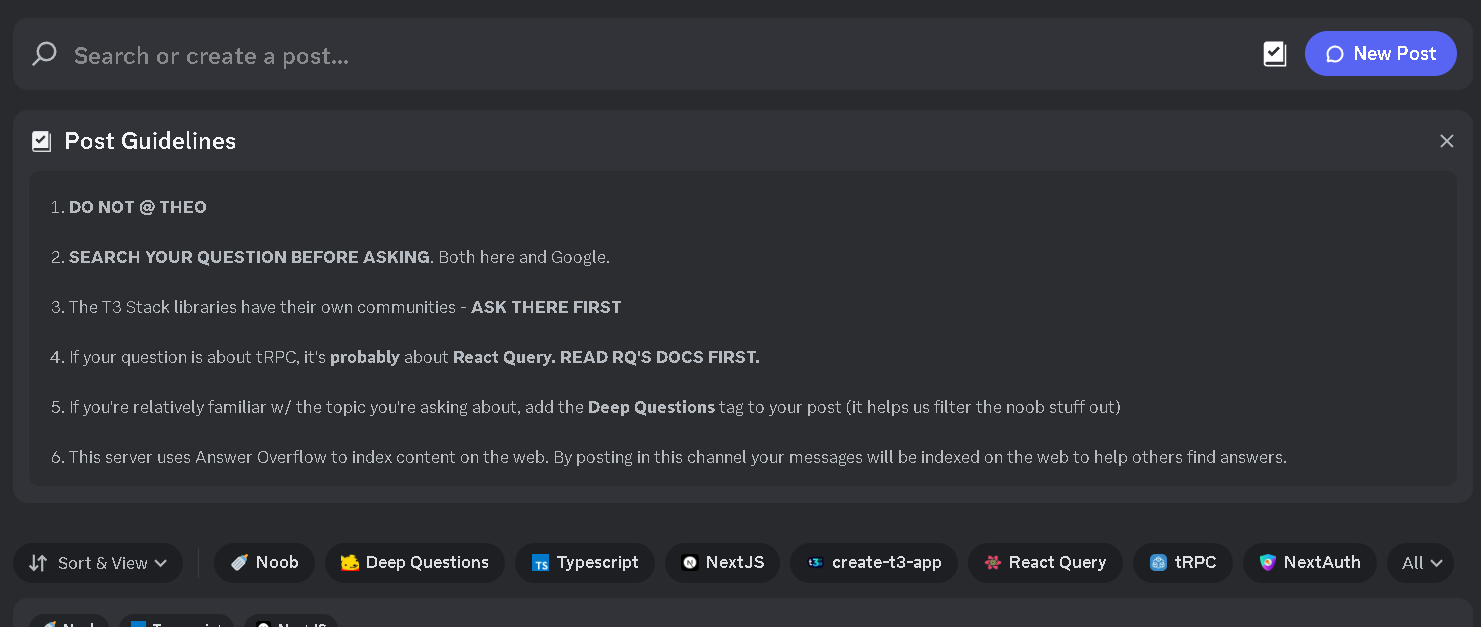
More indepth is actually the React Docs: https://react.dev/reference/react/useCallback
useCallback – React
The library for web and native user interfaces