❔ Verify Uniqueness in an Array
Does anyone know how to determine if an array contain unique names in an array without using previously defined methods. I have worked on this for days and finally just had to submit the homework without figuring it out.
The instructions:
"Given an array of string, names, verify that each name is unique meaning that none of the names are duplicated within the array. If the array is unique, return true; otherwise, return false."
My code:
7 Replies
would "without using previously defined methods" include not using
HashSet<T>
?
cause that'd be the easiest way
otherwise
Take a simple example to test with:
{ "John", "Amy", "Bob" }
. Your loops should be checking : "John" and "Amy"
, "John" and "Bob"
, and "Amy" and "Bob"
. There's not a need to check both "John" and "Bob"
and then "Bob" and "John"
. If a = b, then b = a so it's duplicate work.
Also the moment you find a duplicate, you can stop checking as you just need to return a true or false. For example in { "John", "John", "Amy", "Bob", "Alice", "Amy" }
once you determine that John
is duplicated, you know that the array is not unique and never can be, so there isn't a need to check for "Amy" and "Amy"
.sounds like they expect you to use nested loops and return early, so your solution is pretty close to what it should be
For some reason my professor had me set it up that way.
That is what my professor said however I haven't been able to figure it out.
So the first loop,
i
is going through all of the strings in array, and the inner nested loop is going through all of the strings in the array starting after the one your first loop is looking at. When i
gets to the end, it doesn't need to check the last string against the last string, though. i
will need to be the second to last element because j
is going to be the last element.When I first started learning programming, I would draw out each step to visualize what was happening like this:
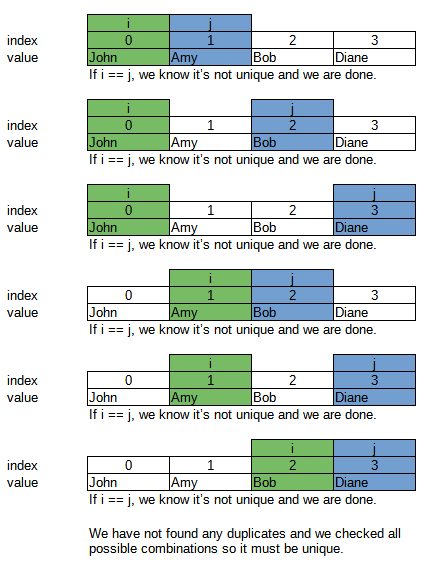
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.