❔ How to make a list of tuples that hold references?
I want to create a List that holds reference for two types of objects: one class object and one string. For this, I tried using tuples. However, it seems like when I create a tuple (with a constructor or with Tuple.Create), copies of those two objects will be created instead of references. What would be a solution for this?
15 Replies
that shouldn't be the case. can you show what you're doing and how you're testing it?
just_ero#0000
REPL Result: Success
Console Output
Compile: 691.652ms | Execution: 53.088ms | React with ❌ to remove this embed.
It's part of a larger project. The relevant code looks something like this:
I noticed the problem when placing a breakpoint inside the
Update()
function. Even though the contents of StartGameButtonString
were updated, the string inside localizedText.Item2
was not. Is the problem here or should I look somewhere else?you don't update
Item2
ever.My goal was to have
Item2
have a reference to the string so it would automatically update. Is that possible?to what string?
also it's probably best not trying to roll your own way of localizing your app
To clarify,
AddLocalizedText
is called with the parameter LanguageStrings.StartGameButtonString
, which is a string declared in the body of my class. The desired result is for the Item2
of LocalizedText
to update by itself whenever the value of StartGameButtonString
is modified. Sorry if I worded this poorly.that seems more like a major design flaw
I'm aware, it's a patchwork solution for a project with a deadline that is pretty close...
(and is not possible)
I'm aware...
Either way, unfortunate for me that I can't hold references like that. Thanks for the help, I'll see what I can do about it.
why not just clear the list and repopulate it?
that's basically what you're doing anyway
Oh, I think I could do that. And that usage of "nameof" gave me yet another idea on how I could implement this. Thanks!
strings have value type semantics even though they're reference types, that's why you don't see the changes "propagating" through other references set using the one you changed
i guess even then it wouldn't work without some kind of wrapper 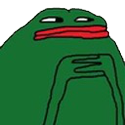
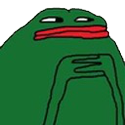
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.