❔ Python Decorators in C# ??? How do i intercept method calls with attributes?
Is is possible to intercept/prevent a method call in c# with attributes? i was thinking about something like python decorators, which can decide what happens with the method call, args and return value
that should throw a
ValueError
if the id
parameter is 69
, for example45 Replies
You can't
actually no you can but it takes the power of literal black magic
Bottom line is that Python-like decorators just aren't supported in regular C#.
if you have the ability to add an attribute to the method you also have the ability to add code that does the same thing, no?
This is probably a task for source generators or dynamic codegen
Not sure what python does to make this work
i got 100 database "middlewares" where i just want to add attributes to catch exceptions if env.IsDevelopment() for example
SGs can't do this because they can't modify source
Yeah that's true, they'd have to put a decorator around
Then you should probably do that using middleware/filters
So the code needs to be in a specific layout
this would be IL weaving
if anything
yeah, black magic
Yeah, that's going to work for everything
I'd put source gen a level lower on the magic scale at least, but it won't work everywhere
this is what we do at the moment
take a look at the last method
wtf are these type and parameter names 

we got a code generator ^^
make the code generator follow standard C# guidelines 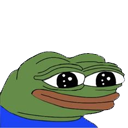
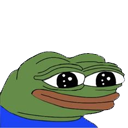
those are old database table names etc, can not change that
you use database table names for type names...?
for models, yes
our code generator creates c# classes from mssql database tables based on stored procedures for insert, select, update, delete, selectList
i'm glad i just use efc
Well you can do it without black magic, but it's extremely expensive performance wise
Assuming black magic is il weaving
hmm, how would you do it otherwise?
like... replace the method IL at runtime?
one of my old game projects made liberal use of runtime IL emission and monkey patching
https://github.com/TorchAPI/Torch/tree/master/Torch/Managers/PatchManager it's basically harmony from before harmony added a bunch of features
also i didn't write it so don't ask me questions about it 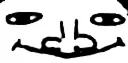
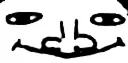
Some di frameworks support interception, with absurd performance costs
Would love to see how that is done
Doesn't that use something like Castle DynamicProxies?
Yes
And castle DP is a bit of a perf nightmare, couple that with transient DI, it can get nasty
i think this solves my problem
I'm not remotely a fan
You're going to swallow exceptions because it's prod??
sounds like a bad idea
log them at minimum
i want to return null to the frontend if an exception is raised in prod
So do that with an exception handler
default(T) is probably not the best solution
Is this aspnet core?
blazor wasm api yes
Handle errors in ASP.NET Core
Discover how to handle errors in ASP.NET Core apps.
Do you mean you have an api machine in wasm? Or you have a server side api you call?
server side api
I'd just use a global exception handler than try and muddy up the works
my goal is to get rid of try catch in every method, i was thinking about a wrapper which handles the exceptions and may return null on production, with logging or email sending
Just use the global exception handler and only add the middleware in prod
The handler will grab the exception and you decide how to handle it and pass back whatever you want
You can write your own middleware too if you want
Just don't make your code a hot mess of environment proxy wrappers everywhere.
kinda, yeah, but that's not implemented yet
Which?
probably the interceptor proposal: https://github.com/dotnet/csharplang/issues/7009
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.