❔ Help deserializing XML file
Hello,
I've been trying to deserialize the following XML, using
System.Xml
, no external libraries:
I haven't been able to get this value SOAP-ENV:encodingStyle='http://schemas.xmlsoap.org/soap/encoding/'
.
Some help would be great.71 Replies
I tried several possible solutions in a testing code like this:
all looks okay to me
is Body deserializing?
better question: is EncodingStyle deserializing?
its not
its always null
I haven't been able to get that SOAP-ENV:encodingStyle value at all
I have a break point after deserializing, so I always check, no result 😦
how do you k ow it's null?
er
scratch that
so, yeah, is Body null?
nope, body is not null
are you quite certain you're deserializing the right file?
yes
its the only xml file on the project (console project I made, with the only purpose of solving this problem)
well, now I have to ask
if it's part of the project
which copy of the file are you deserializing?
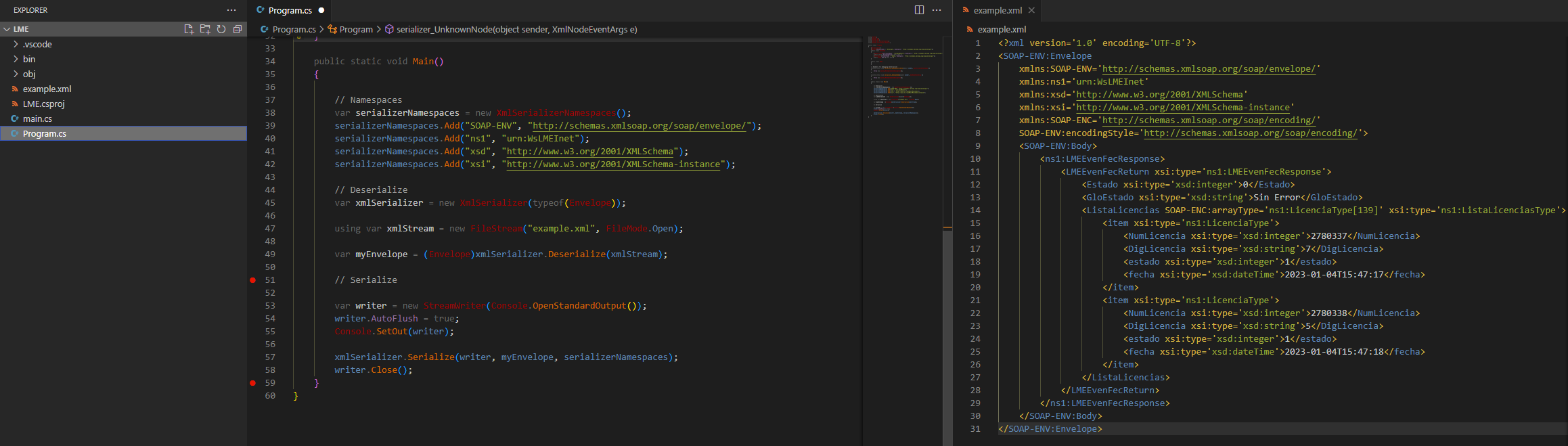
that screenshot is incomprehensible
what I meant sending the screenshot, its that its quite a simple project, Program.cs with the code, and example.xml
yes
which copy of example.xml?
what do mean which copy? there is only 1 version of the xml
if you have it as part of your project, it's likely getting copied and deployed to the bin folder
especially if you're referencing it via a relative path
oh I get it, 1 sec
it's likely opening the version in the bin folder, which may not be the one you've been editing
there is no copy of example.xml on bin
nor obj
how are you running the project, then?
how does that relative path of "example.xml" resolve?
let me delete these folders and try again, I recycled this project and have some leftovers
1 min
by default, that means it's going to look for the file in the same folder as the project exe/dll
which is the bin folder
unless you're changing the working directory, or launching the program from a different directory
I'm just using the default debugging tool on vscode
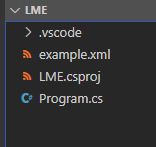
clean project
well, I know nothing about VSCode tooling
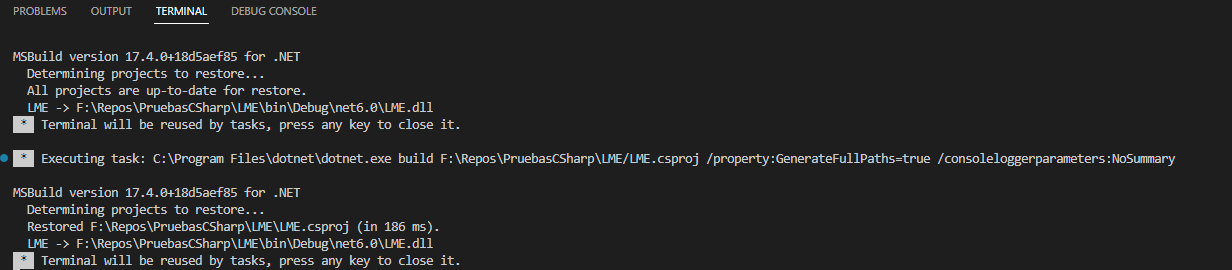
it doesnt generate a xml copy
also, obligatory recommendation to notbuse VSCode, if you don't have to
uses the same one I have in the root folder
alright, well that's really the only idea I have
your code looks proper
obviously, it's not, and it's almost certainly that you're not defining namespaces properly, somehow
yeah, it "looks" proper.. but I just can't read that damn namespaced attribute
but I don't see it
maybe because the namespace alias is at the same level as the attribute that's trying to use it?
does it work if you drop the namespace on the attribute?
or if you swap the namespace definition in your model to just
SOAP-ENV
?
maybe the engine just doesn't donnamespace translations for you? I would be rather appalled if it didn't, but maybe not surprisedSame result, null
dropped from both the file and the model, right?
only model
same, null
yeah, that's definitely not gonna work, the namespaces now don't match. Drop it from both
do you mean delete it from
example.xml
?yes
that wouldn't make much sense, I can't edit that file, since it comes from an API 😦
ill try tho, second
I'm aware, we're just experimenting
additional idea: deserialize the whole thing to an XmlDocument or whatever the DOM library is, and you can inspect EXACTLY how the engine is interpreting everything
it actually didnt work 😦


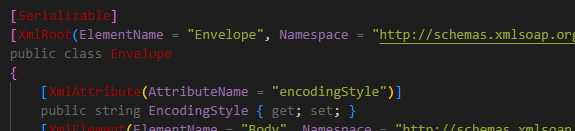
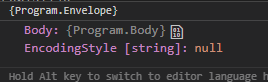
now, THAT makes no sense
little bit
I assumed it would work.
now I'm intrigued and wanna get my own hands on this
unfortunately, I'm not at home
yeah, thanks for the help. Much appreciated... this new finding can give me some room to test some new things.... there are way more examples of xml deserialization when the attribute has no namespace, maybe I can make it work, then I go back to try it with namespace
My bad, I didn't correctly change the example.xml file. since the code was running. It worked now (without namespace)

Adding the namespace back, it goes back to null
well, that's something
Did you try pasting xml as classes?
Im not familiar with the concept, what do you mean?
https://learn.microsoft.com/en-us/visualstudio/ide/reference/paste-json-xml
Also where are you getting the soap from?
https://learn.microsoft.com/en-us/dotnet/core/additional-tools/dotnet-svcutil-guide if it's a trusted web service
It seems I needed to specify
Form = System.Xml.Schema.XmlSchemaForm.Qualified
and now it is not null. Thanks a lot, that tool helped me find that problem. Its not perfect tho, now it throws another problem. Gotta check that out and ill post here again (maybe later/tomorrow)so, you need an extra annotation to tell the deserializer to interpret namespaces in attribute names?
Xml serialization can get really special really fast
Usually I just paste xml as classes and that gets me most | all of the way there
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.I was kinda busy and couldn't come back to ask my new problem. The thing is, I don't really knwo how to deserialize this part:

Paste XML as Classes doesn't work on this part, and when deserializing LMEEvenFecReturn it throws an error

Where are you getting the soap from?
It's a private company, they serve this api service for all their clients, the thing is, it's access restricted tho, I have that xml example, but can't really test it on realtime
I guess you wanted me to use the utility you posted above
Yah
I'm not in a great spot right now, but I think you posted the soap right?
that seems highly sus
cyclical references?
actually
more like
actually, I just don't know off-hand
haven't done XML serialization in this detail in a long time
dunno why the class generator tool is throwing an error, might be worth submitting a bug report
ultimately, I believe what's happening is that you have a property or something of type LMEEvenFecReturn, which is only a base/abstract class. LMEEvenFecResponse is annotated as the specific subclass that was serialized, so that annotation there is telling the deserializer what class to new up an instance of
and there's some kinda bug or limitation in the code-generator tool causing the issue, while the XML itself is fine
I mean, irrelevant of what the tag is supposed to be there for
the error is nonsense
the type ns1:LMEEvenFecResponse is clearly defined, in the exact same screenshot
Iirc the soap stuff does some horrifying stuff
for sure
it SEEMS to me what's happening here is that responses can be nested
which is perfectly fine, OOP-wise
like how exceptions can have an inner exception
Only only looked at the xml as a giant wall of text tbh
Thanks for the help, haven't really got it to work. I was trying to work around it by defining
LMEEvenFecResponse
as an array of 1 element, where LMEEvenFecReturn is a derived class. Something like it appears in the documentation: https://learn.microsoft.com/en-us/dotnet/standard/serialization/controlling-xml-serialization-using-attributes#serializing-derived-classes
I hope I get it to work like that... still no success tho, that type having a namespace kinda bothers me, however didn't have much time today to try.Controlling XML Serialization Using Attributes
Attributes can be used to control the XML serialization of an object or to create an alternate XML stream from the same set of classes.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.