26 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Alright i'll try it.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
MB, Thank you!!
Do i put the console.log like this?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Still NaNms
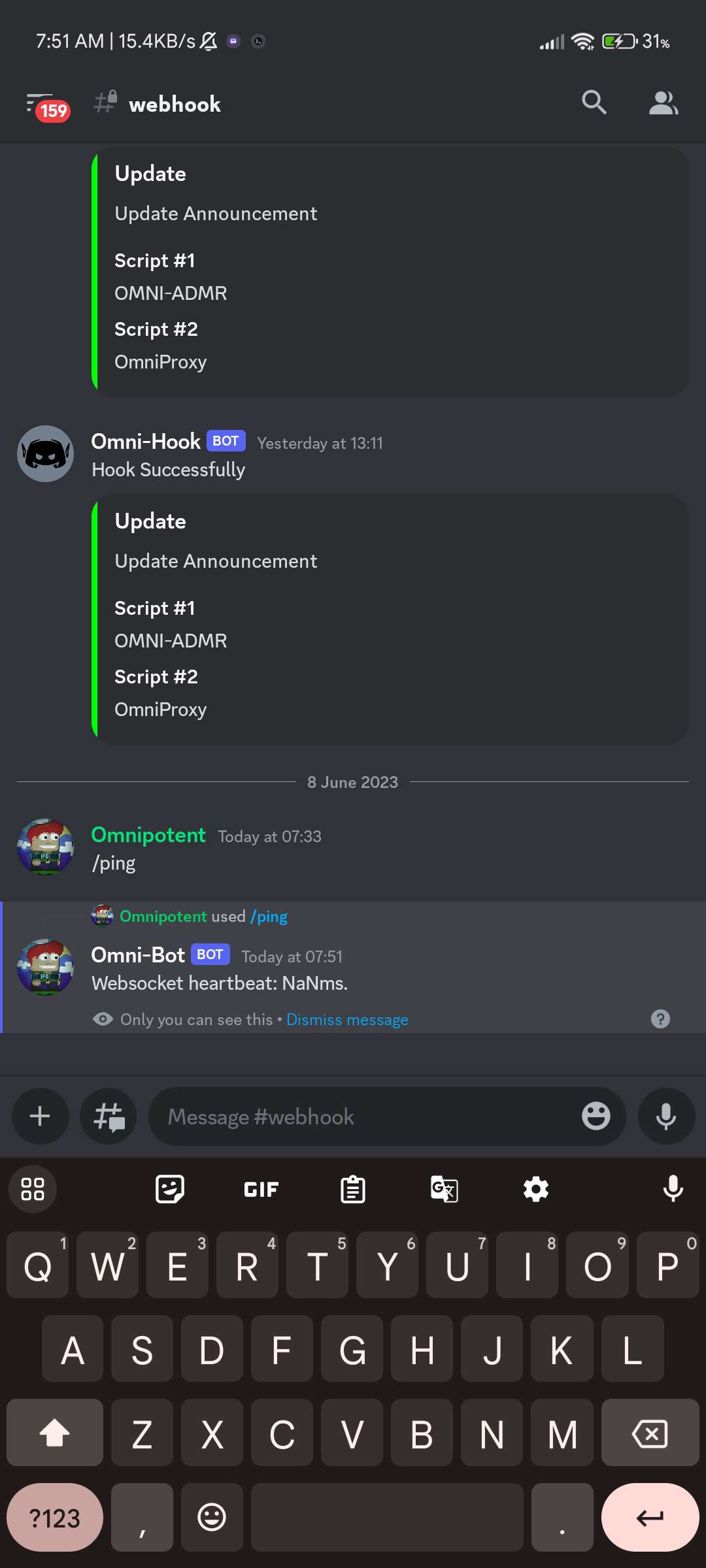
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
My main file is index.js
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
This index.js
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I changed the ping.ms into this
Is this good now?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I got warning when I deploy the commands.
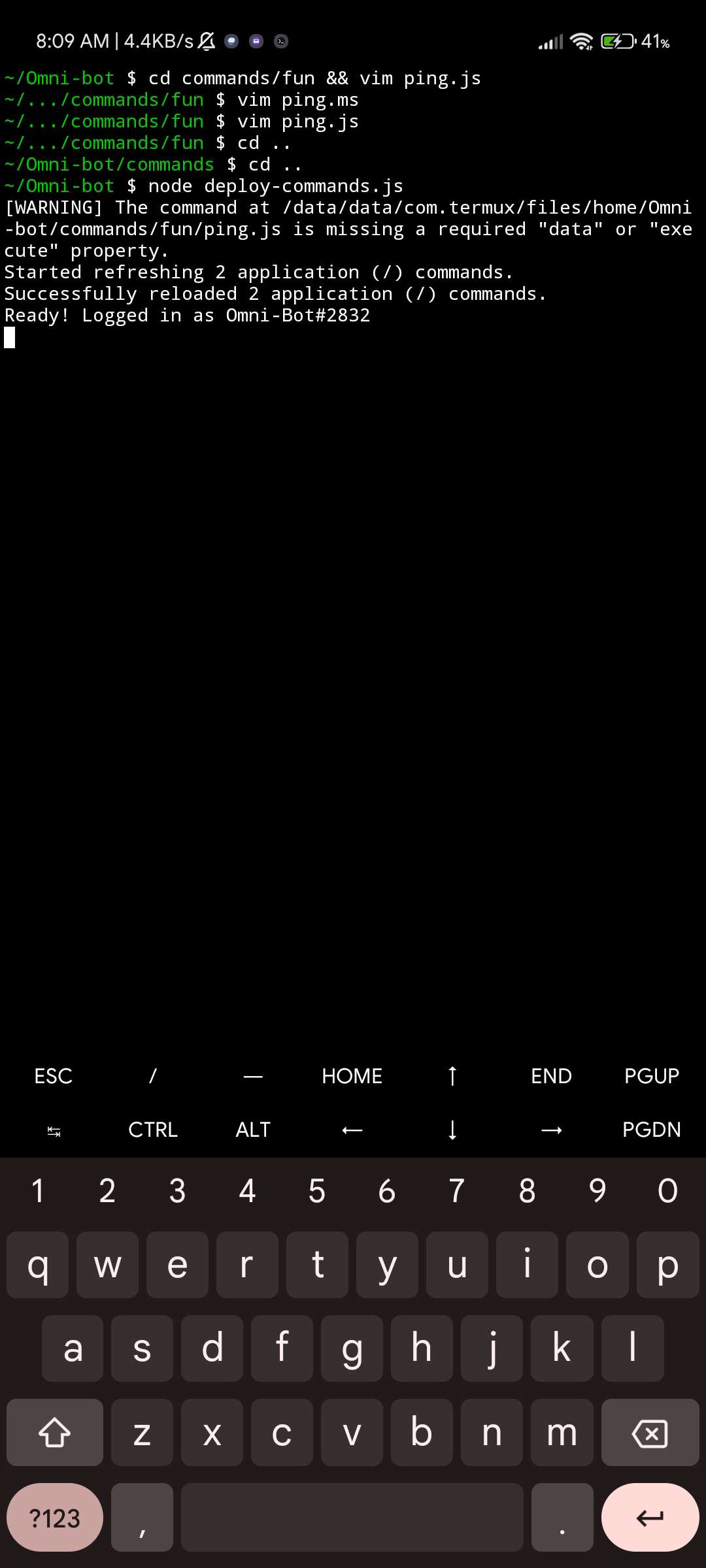
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Thank you, but my computer is down right now. Windows doesn't boot.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Thank you Jo
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
@Jô 🌈 🦄 @zer0cool0 it works now.
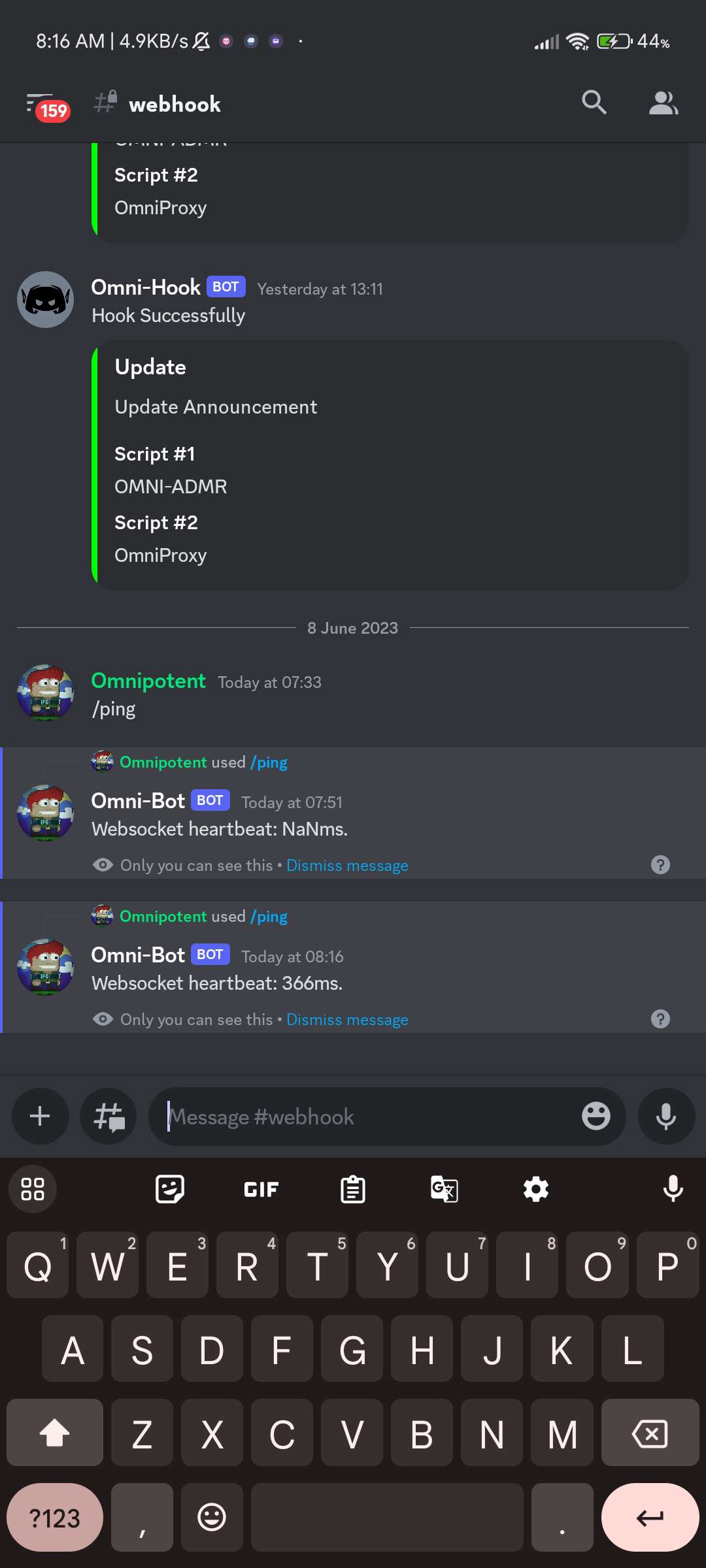
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I remove the client and i add interaction in client.ws.ping
Thanks!!