Need help with Strategy pattern for discounts in POS system
Hi everyone,
I'm working on an assignment where I have to implement two GoF patterns in a POS system. One of the patterns I chose is Strategy for calculating discounts based on criterias (customer ID, amount of products, etc.) The problem is that when I run Main.java, it doesn't show that the discount is applied on the products. I'm not sure what I'm doing wrong.
Here is some context of how my code works:
I have a Sale class that represents a sale in the POS system. It has a field called discounter that holds a reference to a Discounter interface. The Discounter interface declares a common method for all discount algorithms called applyDiscount, which takes a BigDecimal amount as a parameter and returns a BigDecimal amount after applying the discount.
I have two concrete classes that implement the Discounter interface: QuantityDiscounter and CustomerDiscounter. They both have their own logic for fetching the discount rate from a database and applying it to the amount. They also handle any exceptions that may occur when accessing the database.
In the Controller class, I have a method called createSaleAndApplyDiscount that creates a new sale and sets the discounter field to a new instance of QuantityDiscounter with a parameter of 3 (meaning 3 or more items bought will get a discount). Then it calls the applyDiscount method on the sale object and prints the discounted price.
In the View class, I have a method called simulateSaleProcess that simulates a sale process with three products and calls the createSaleAndApplyDiscount method on the controller.
The part of the assignment I'm stuck with is why the discount is not applied when I run Main.java. It should print something like "The discounted price is: ..." but instead it prints "The discounted price is: 0.000".
Can anyone help me figure out what's wrong with my code? Any hints or suggestions would be greatly appreciated.
Thanks in advance!
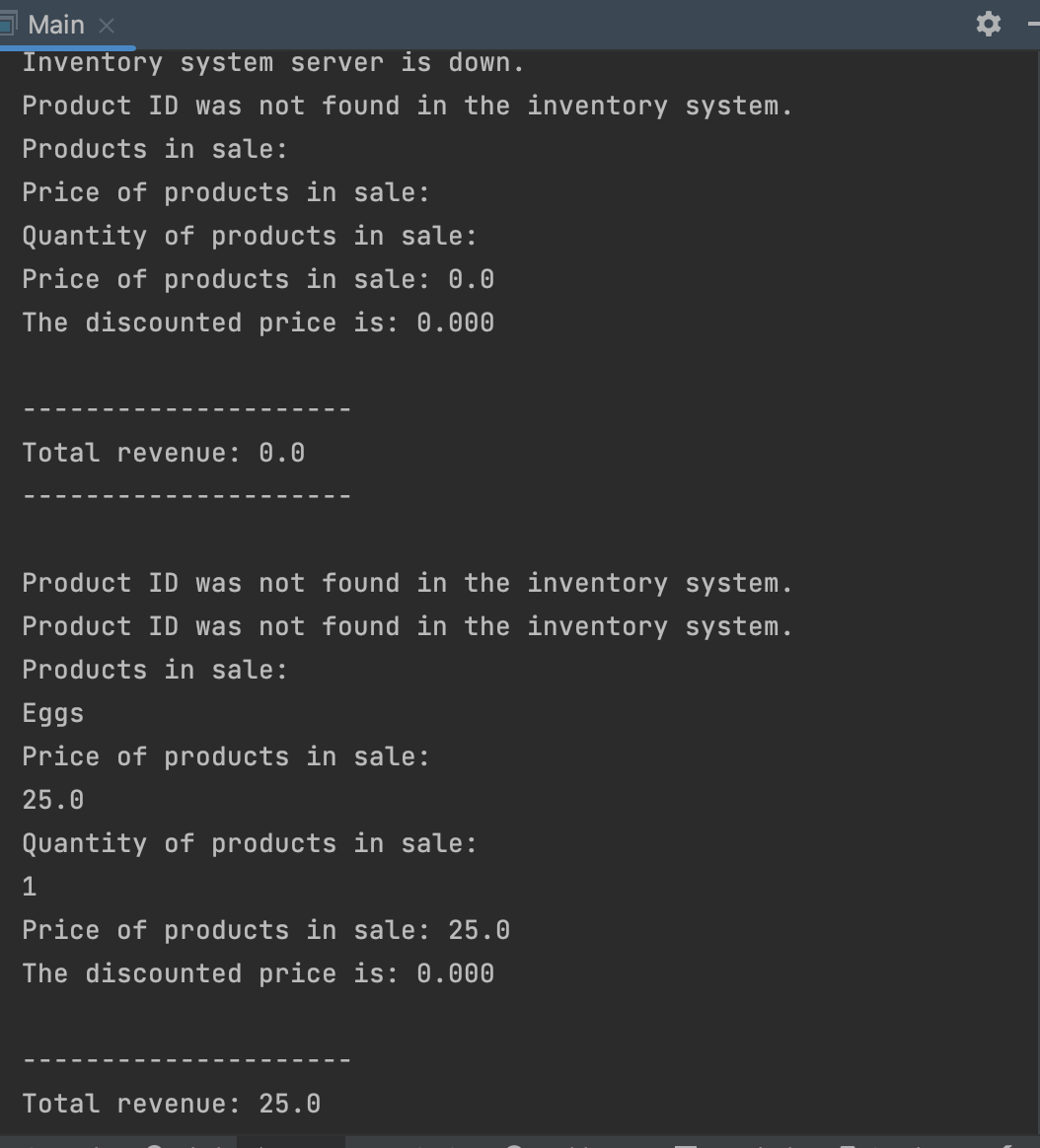
13 Replies
⌛
This post has been reserved for your question.
Hey @dghf! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when you're finished. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
@That_Guy977 https://sourceb.in/KzRuzbmzO5
This is my entire code
The code that calculates the discount is in the Sale class, in the applyDiscount method. Here is the code:
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
You think the issue is that
createSaleAndApplyDiscount
creates an empty sale and doesn't add any products to it?Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Generally speaking, you can't claim that you've tried to solve a problem, if you haven't first moved that problem to a 20 lines program
So I'd have to move the createSaleAndApplyDiscount method from the Controller class to the View class, and call it after adding the products to the sale?
So
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
But I am not sure if that'd break encapsulation or violate OOP principles and bring bad code smells
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived. If your question was not answered yet, feel free to re-open this post or create a new one.