Error Handling?
I want to supress only certain types of errors.
- Where can I find the documentation for different error.code values?
- Where can I find the structure of errors returned by Discord.js?
- What are the best practices for handling discord.js errors?
5 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.love ❤️
What's the difference between DiscordjsErrorCodes and RESTJSONErrorCodes and how do I know which is returned?
And are errors just structured as:
fab, that makes a lot of sense, thanks
I want to supress only certain types of errors.would the "best" way be something like: or to ignore RESTJSONErrorCodes.UnknownMessage but do error logic for other errors? I'm aware it's not strictly a d.js question, I was just wondering I want to avoid nested try {} catch {} hell perfect, thanks would you not end up with something like this? for example I can't help but think that there must be better ways Ahh, I see, bad example by me for example, I would be able to safely skip all "change nickname of x member" errors though when removing zalgo characters from all members in a guild i.e. user left the server, user's role is higher than the bot unless you mean that the best way would be to pre-empt every circumstance before instead of handling the errors but it seems equally tedious to check if the user exists, is in the server, has a role below the bot, etc.
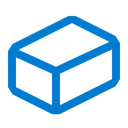
essentially I want to most errors
but obviously if it comes to an error that is absolutely unexpected, I want the whole bot to post the error to Sentry, for example, or terminate gracefully
for example, with the zalgo example, would it be best to abstract it into its own function?
sorry for all these silly questions
it just seems fairly tedious and mundane to do that for every action, but I guess that makes the code more mantainable
how would you return a boolean? by checking if the response of
await member.setNickname(...)
is false?
will .then not become deeply nested?
ah I see, so using
thanks so much by the way, and sorry for asking so many questions
on an even more vaguely-related d.js note, what would be the best way to:
- ensure an action is performed (even if the bot restarts)
- is it every relevant to retry an action if it fails the first time
I assume for the first I can use any persistent message queue system
actually, for the first it seems very unsafe
for context, the bot is similar to an LFG bot
it creates voice channels, text channels, and roles for users who are playing games
for example, by grouping together players who type the same command /join
say, if the bot loses power (magically) after creating the text channel but does not yet create the voice channel
is it best to store the required actions in a persistent queue and retrieve and complete those actions after restart
or is it safer to look up all games which were in the process of creation when the bot starts
and just inform those users that an error has occurred
the first would be ideal, but would seem complex to implement and would introduce bugs
perfect, thanks
is it every relevant to retry an action if it fails the first timedoes discord.js natively retry API requests? on a 5xx error I appreciate that those are rare thanks