[SOLVED] Playback ends after ~1 minute without any errors emitted
Title. It sometimes becomes Autopaused, sometimes not. It eventually becomes Idle after a few minutes.
No errors are emitted from the player nor the connection.
Any ideas on how I can get more information on what's going wrong or any solutions to problems I have overlooked? Thanks.
The bot's connection and player are being initialized like this:
And playing back with:
14 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.[email protected]
node v18.16.0
I believe I have found the fix from this: https://github.com/umutxyp/MusicBot/issues/97#issuecomment-1452607414
GitHub
SUCCESS: Bot stops playing after around 1 minute · Issue #97 · umut...
The bot stops playing after around 1 minute of playback... No error in the console. Any idea what could be causing this?
I needed to install the following event listener to the client (this is copy pasted from the comment modified a little to fit my code):
I put this directly below the call to joinVoiceChannel()
this fix should no longer be needed on the latest version of
@discordjs/voice
could you share your @discordjs/voice
version?0.11.0
latest is 0.16.0
consider updating
wow
i shall
thank you
also may be relevant to @sayed1
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
amazing!
though updating djs voice also fixes it
i updated to latest and i removed the code
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
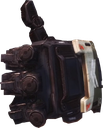
Solved my problem too, ty!
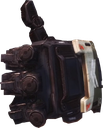
