✅ Add OAuth 2.0 in HttpClient Request Headers
Hi folks, I tried to request by Get using HttpClient, but in this case, I don't know how can add this "OAuth 2.0" in HttpClient to send. When I use this code I have the unauthorized 401.
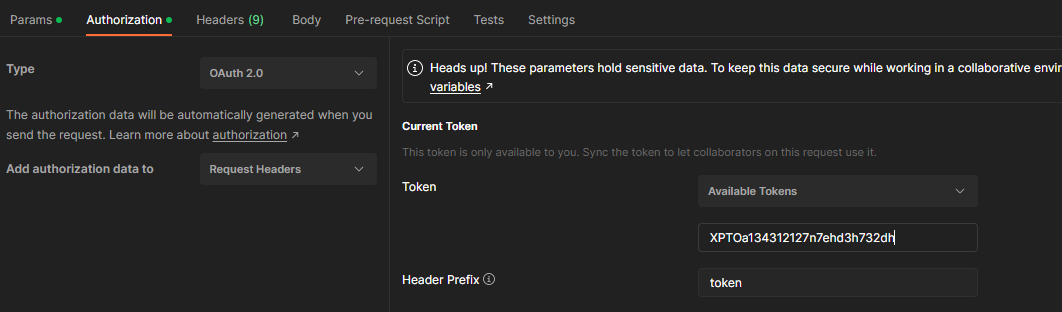
28 Replies
Not sure if I understood you correctly. What do you want to add?
How can I add "OAuth 2.0" in my Get, when I try to request with this code, I have the following unauthorized 401 error. My token is correct, because I tested the request in postman
You mean this?
That's the real token in the picture? You shouldn't post tokens publicly
No, my real token is another one, I put that one just to illustrate in the image
Good 😄
I tried this, but I got the same 401 😕
Sometimes I think this is a bug in .NET
So, when you tried stuff out in postman and it worked there, you could try to copy the headers from postman and add them to your request.
This is my Headers
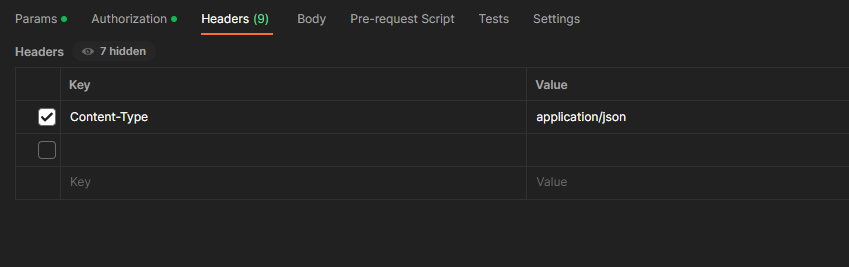
+7 hidden headers
Hummmmm give me a second
How can I add this in my headers?

I guess those wont be needed
The same again, 401 😕
Hmm, without seeing your code, I'd have to guess from here on. Maybe your url is wrong?
Have you tried using "Bearer" as token prefix?
Yes
I#m running out of ideas here
Is there more to the authorization process? Do you have to provide some client id or so in the url?

Unfortunately not, that's why I told you that I think it's some .NET bugs 😕
That's pretty unlikely imo. But no idea what else could be wrong then
So, yesterday I tried several solutions according to the documentation, according to StackOverFlow and today here with your tips, I find it very difficult for 3 different way to all go wrong when all 3 showed similar or identical solutions.
Must be something super obvious but small then
remember that I was requesting somthing from "https://..." when i just needed "http://...", but i doubt that this would return 401

I didn't understand what you meant
What exactly did you not understand?
About https and http
return 401
That was just an example that it could be a tiny detail you are missing
Humm ok
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.