✅ Need Help Passing Code Off To Helper Function
this is my current code https://pastebin.com/RFRQshGX. I'm trying to split it off into 1 or 2 helper functions to clean things up a bit. The first helper function needs to check if the users input is an integer and the second function needs to check if the integer is an index of the list, but I can't figure out how to separate it and get the input correct. The helper functions also need to return the correct values too please. Any errors that I have encountered are commented next to the line throwing the error. Thanks.
33 Replies
function
CheckInputIsInteger
needs to return an integer and function CheckIfCorrectIndex
needs to return a stringpublic bool CheckInputIsInteger(int tries, string userInput, out int value)
public bool CheckIfCorrectIndex(int tries, int userSelection, List<string> correctItems, out string correctItem)
it works like the picture
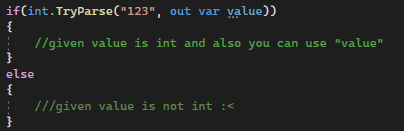
ok maybe I need help re-writing my stuff.
This is the "brain" function that gathers all of the input and then launches the wanted game screen.
changing the function to what you suggested, is still telling me that not all code returns a value so how would I write this helper function?
taking it one step down,
return value;
tells me that I cannot convert int to bool(sorry if i wrote something wrong, i'm not use google translate now) so, you made a little mistake, you just need to return something in every if else condition. just imagine situation if your function needs to return something where in "if" you return true, but what happens if there is no return in else? yes, this code won't compile, cus your function can't return you what you need
right. I get that part.
do not forget to add after if(){}
maybe you should take out tries as a variable? you use it in every method
This is my original code https://pastebin.com/WXJhPwBE. It works just how it is, but I'm trying to lighten the load of this function and clean the code up a bit. Specifically this part
This is 2 separate actions. The outer if statement is checking if the user entered an integer and if not, then it's allowing them to try again up to 3 times and the nested if statement is checking if the integer the user entered is of an allowable index. I'm wanting to put the outer if statement into one function and the nested if statement into a second function. The only difference is, the functions need to return the correct values.
can i get the whole script and do the refactoring?
so like
this function would match
and then the second function
this function would match
so I'm trying to break that giant if statement up into 2 functions to clean the code up a bit
so something like this, but this doesn't have a return value for all code lines so do I just return a random number?
ok I guess so. I answered my own question lol
just return 0;
and you didn't get how to use out
what do you mean?
?
I need the function to return the integer, not true/false
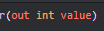
it returns value
that's why I need it to return the integer value, not true/false
........
dude
out userSelection is your value
or just use global variables
so you don't even need to return anything
ok so the one thing that i was missing was changing my variable cast.
ok so onto the next part
I implemented that
Pastebin
public void InputInvalid(int tries){ if (tries > 0) { ...
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
so add some code there cus i don't understand what to do
this is what I have so far, but I'm not sure how to write it so that I can get it to return correctly
what kind of stuff you need to return
stop doing this pls

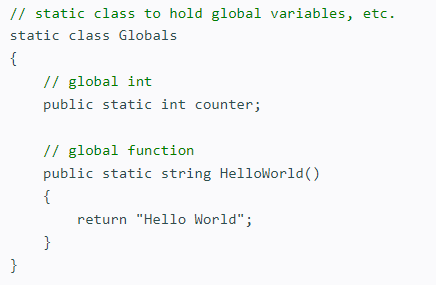
tries is instantiated in my first program file. it's passed around from the primary function throughout the entire program. It's not going to be global
than put in your class not in every method. sorry, but it's stupid
that's your opinion
this keeps telling me
The out parameter 'value' must be assigned to before control leaves the current method
so should I just put value=null;
above the return false;
line?anyway you can do what you want
ty for your help ❤️
to show what I mean about the tries variable is it's instantiated here and passed around. It's written once and re-used every time
