❔ EF Core Fluent API - Unidirectional one to many relationship
How can I implement a one to many unidirectional relationship using EF Core fluent API? (Image 1)
I'd like to avoid navigation properties in
Coordinate
as I believe Square (or any shape for that matter) needs to know about it's coordinates but the coordinates don't need to know about the shapes.
I've tried the code below but it's wrong. (Image 2)

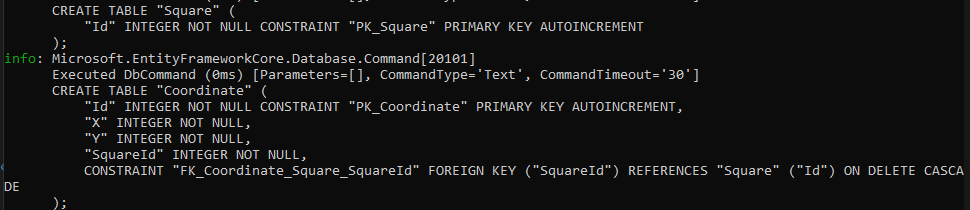
5 Replies
imo coordinate should be a valuetype, not an entity.
But what's wrong?
Yes, it could very well be a value object.
I'm just wondering how to, if possible, implement this relationship.
In the example above, I'd like to generate the relationship in
Square
and not in Coordinate
as Coordinate
does not need to know anything about the shape. However if you look at the second image, you can see that there's a foreign key on Coordinate
.EF is always going to put the FK on the child afaik
I see, thank you.
Looks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.