❔ XML Read XML and save as dictionary
Hi all. I want to read XML data and convert to a dictionary, I have given this go with:
This is how i write:
This is how I am reading:
I get an error
Pref
is an unexpected token. The expected token is =
Line 2, position 20.14 Replies
This is an example XML doc
well, its invalid xml, names (no matter if attribute or element) are not allowed to contain whitespaces
so u would have to rename the root element
Ribbon Visibility Preferences
to Ribbon_Visibility_Preferences
?
That simple?
Values the same then, i.e Archi Tecture
would be invalid?values can contain whitespaces, they are wrapped by the double quotes after all ;p
but yeah basically its that simple
names can contain letters, digits, hyphens, underscores, and periods
Awesome - Thank you will test it now 😄
@Curious r u there
Yes Hi
is it fixed
No still produces
error Pref is an unexpected token. The expected token is = Line 2, position 20.
and returns nulland
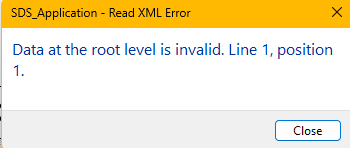
private const string StoredDataFile = "C:\\ProgramData\\SDS_TOOLS\\RibbonPreferences.xml";
private const string RibbonVisPref = "Ribbon_Visibility_Preferences";
the error is in there:
basically u need to get rid of the
xml.LoadXml(tagToRead);
lineOhhh
makes sense to be fair
thank you
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.