✅ Memory leaks and idk how to solve them
Why does the following code produce memory leaks? Shouldn't
bg
get deleted and then reallocated?
Why do these constantly allocate new memory and the old one doesn t get freed up? And how do i make it work?21 Replies
Does
bg
have other references?
Also note that the old one won't get deleted immediately when you set bg = new(), it will get deleted when the GC runs the next time.
Not a good idea generally but could try forcing a garbage collection immediately by calling GC.Collect to check if it does get deleted.you never even use
bg
? what's the point?
the Dispose
method also doesn't do anythingu probably have a memory leak in loading the texture on every frame
when the GC releases the memory for the not rooted
Background
instance (as in its not referenced anywhere anymore) is up to the GC itself, it could be hundres and thousand frames after unreferencing it.
but even that releasing memory, doesnt mean the physcally used memory will shrink. the .net runtime uses its own memory management model on top of the "normal" memory, so even if that heap is mostly empty, ur application could still consume like 2 gb worth of ram from the OS' perspective
https://learn.microsoft.com/en-us/dotnet/core/runtime-config/garbage-collector#high-memory-percent explains quite some stuff about itGarbage collector config settings - .NET
Learn about run-time settings for configuring how the garbage collector manages memory for .NET Core apps.
i assumed that that code runs somewhere every frame
its also not good to create new instances every frame, try to reuse them
u probably have a memory leak in loading the texture on every frame
Raylib.UnloadTexture(Texture2D)
(https://github.com/ChrisDill/Raylib-cs/blob/master/Raylib-cs/interop/Raylib.cs#L1587) seems to be the method u would need to use free the unmanaged resourceslook. i have a game class that is the whole match itself. it uses background, pipes and bird (these are created new every time i create a new instance of game)
when i want to start a new game, i just want to reinitialize that instance
game = new Game();
but this causes memory leaks
i need a way to delete the memory used by game, and then reallocate it
in cpp i would do
or i would use std::unique_ptr
as explained u cant really force the GC to do it when u want to, thats the whole point of managed memory systems.
as soon as the instance isnt referenced anymore, the GC will sooner or later collect it. when, is up to the GC
if u want to free underlying resources (because they somewhere down the line use native memory), u should use the
IDisposable
patternA GC doesn't immediately delete after it's no longer used like a RAII smart pointer does.
well, apparently it never deletes it
If it still leaks after the GC runs, then probably you have a reference to it somewhere that you aren't realising.
what makes u think that?
just the memory usage from taskmgr or which ever tool u use?
Try calling GC.Collect and check if it's gone.
(question was meant for Sound btw)
The memory usage window in the Visual Studio debugger can show all the managed objects not collected yet.
the memory usage graph in vs
that? that includes unused heap memory

At the bottom you should see a memory usage tab.
And then you can try hitting collect or snapshot (don't remember the name exactly).
on 3 a double click, then ya can inspect
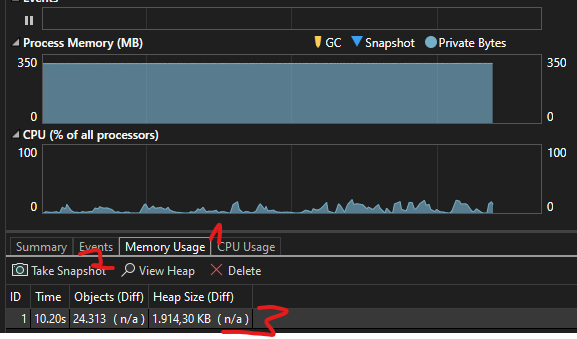
im not sure if
Raylib.LoadTexture("./resources/background.png");
allocates RAM down the line, but its at least a VRAM memory leak as explained earlierYou could click on the objects and see if an instance of Background is still there.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.