✅ Csharp serializing
Hello, I am really need this to work now and I am lost on how I can serialize because there are some errors which I do not really understand. I also am not sure where I should be serializing things.
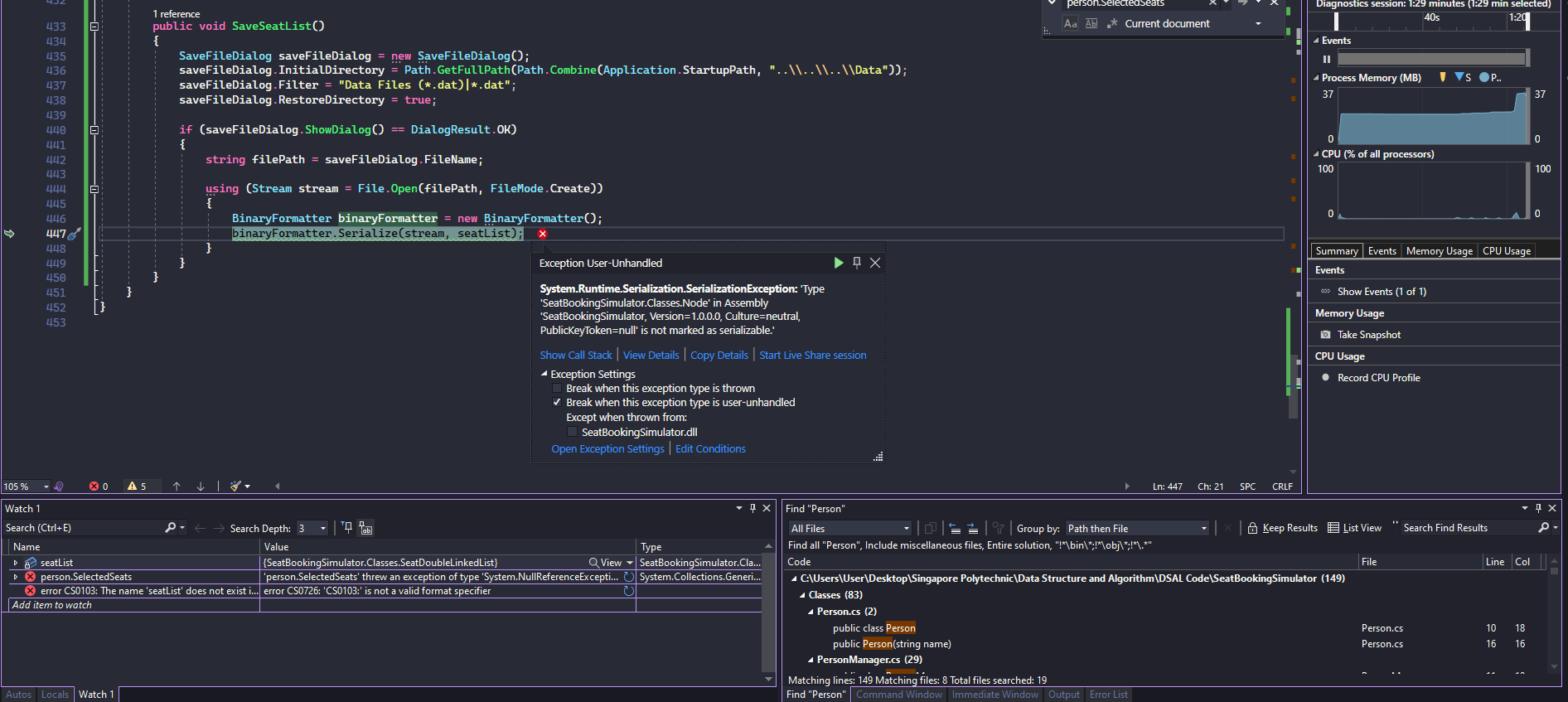
46 Replies
What is this for?
I want to serialize a seatDoubleLinkedList which contains Nodes for holding a seat class
ok first, don't use
BinarySerializer
the design of it is not very secure and it is known to have caused many problems
it is actively being discouraged from use; you should look for alternatives instead
what is the "seatDoubleLinkedList"?Oke, what should I use? and security not a problem since i just want to do a school homework
Yeah but overall it's very bad practice to use it
Haha oke, can i send my whole code?
Sure
Pls dun mind my bad coding
I guess looking at your directory structure you're practicing how to use linked list
so I won't really comment on that part
I just send all this?
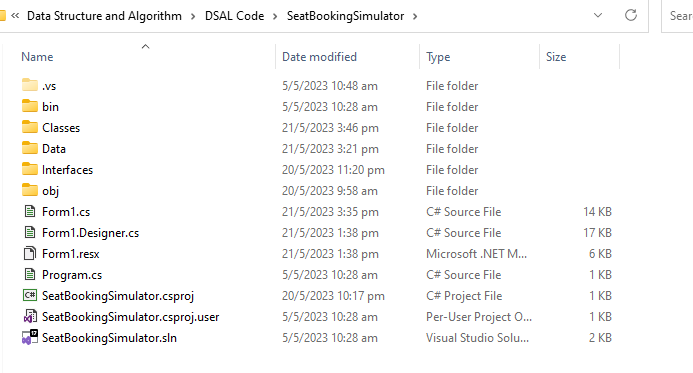
oh ok you don't need to send those
based on the description
seatList
is a doubly linked list?
it is completely unnecessary to serialize the list as a linked list, you could just walk over the list and just save the details of each seatYeh
one very common way to do that is to use something like
JsonSerializer
what does the list node look like?Hmm wait give me a moment
so really, what you want to store is the individual
Seat
s in the linked listits just to store seat class and see whos next and prev
right?
yeh
so what you could do
well actually before that
how does the
Seat
look like?hm ok I think you can use auto properties but that doesn't matter here
I dont know whats that 0.0
this can become
ooh
but anyway
I think you're better of making the linked list into an array
This is my files
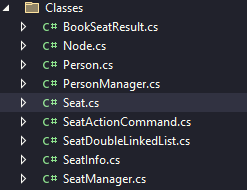
I cant my homework force me to use linkedlist
yes but
you can always read the array back into a linked list when you load the file
then once it's read into an array serializing the said array of
Book
s into some format like JSON is much simpler
there are probably solutions that can serialize the entire tree ("object graph" as they call it) but it seems completely unnecessary here
and you definitely do not want to use BinaryFormatter
does jsonSerializer can serialize into binary, its smhow also a requirement after looking
not sure what you mean by "binary"
does it actually tell you that as a requirement
show full text?
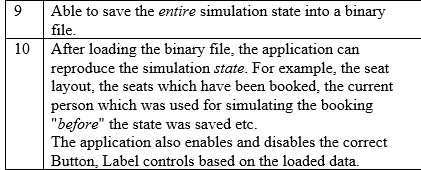
arguable what constitutes as a "binary file"
text files are also binary after all
hmm wait i got an example somewhere
actually idk, i think the teacher meant to use the binarySerializer
I just want to save the entire state of booking, specifically the Seat and Person class and also the Stack class but since In the Form i design it that Form can access the PersonManager and SeatManager, PersonManager have access to the list of Person class, while SeatManager have access to the doubleLinkedList which have Nodes which have Seat class.
So should I covert to array in the SeatManager class?
if you want a "more binary" version of json you could use bson 😜
I think i have to use binaryFormatter
I just dont understand why this is happening
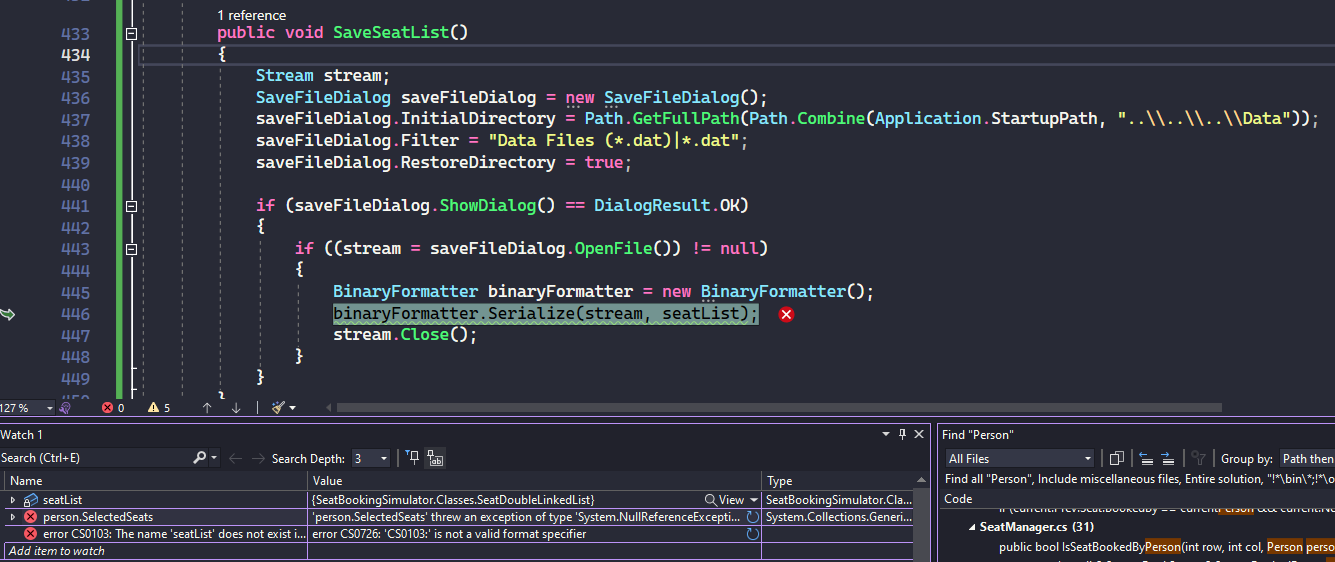
is it written in the requirements "use binary formatter"?
I guess in this.
The teacher also gave an example on how to use this and i used the exact code but i got error
would the implementation of using other formatter be different?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I tried different formatter but i still failed to serialize
am I doing something else wrong?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Oh no i cant serialize a class with Label?
Like this one?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
It alright already, it because Label is not serializable and the Seat class i have this
Ty guys