Issue turning a node linkedlist to generic type node linkedlist (this is what I tried)
public class LinkedListf<E> {
static class node <E>{
E data;
node<E> next;
node(E value) {
data = value;
next = null;
}
}
static node head;
// display the list
static void printList() {
node p = head;
System.out.print("\n[");
//start from the beginning
while(p != null) {
System.out.print(" " + p.data + " ");
p = p.next;
}
System.out.print("]");
}
//insertion at the beginning
void insertatbegin(E data) {
//create a link
node lk = new node(data);
// point it to old first node
lk.next = head;
//point first to new first node
head = lk;
//pointing old node to new node
}
void deletedata(E data){
node del = head;
node prev = null;
if(del != null && del.data == data){
head= del.next;
return;
}
while (del != null && del.data != data){
prev = del;
del= del.next;
}
prev.next=del.next;
}
void insertafter(E index, E data){
node sert = head;
//node prev = null;
node ins = new node(data);
while (sert != null){
if(sert.data == index){
ins.next = sert.next;
sert.next = ins;
break;
}
sert = sert.next;
}
}
public static void main(String args[]) {
int k=0;
insertatbegin(12);
insertatbegin(22);
insertatbegin(30);
deletedata(12);
insertafter(44, 2);
System.out.println("Linked List: ");
// print list
printList();
}
} //issue is im not sure if its correct, and I get a cannot run from a static point because I had to change the functions from static when trying to call the functions in MAIN as is.
100 Replies
ā
This post has been reserved for your question.
Hey @Alpha! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when you're finished. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
is it not the title?
or did I miss a part?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I put at the bottom of the code
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I got it to work
Am I allowed to post the code for you to see? here @That_Guy977
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
yes
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
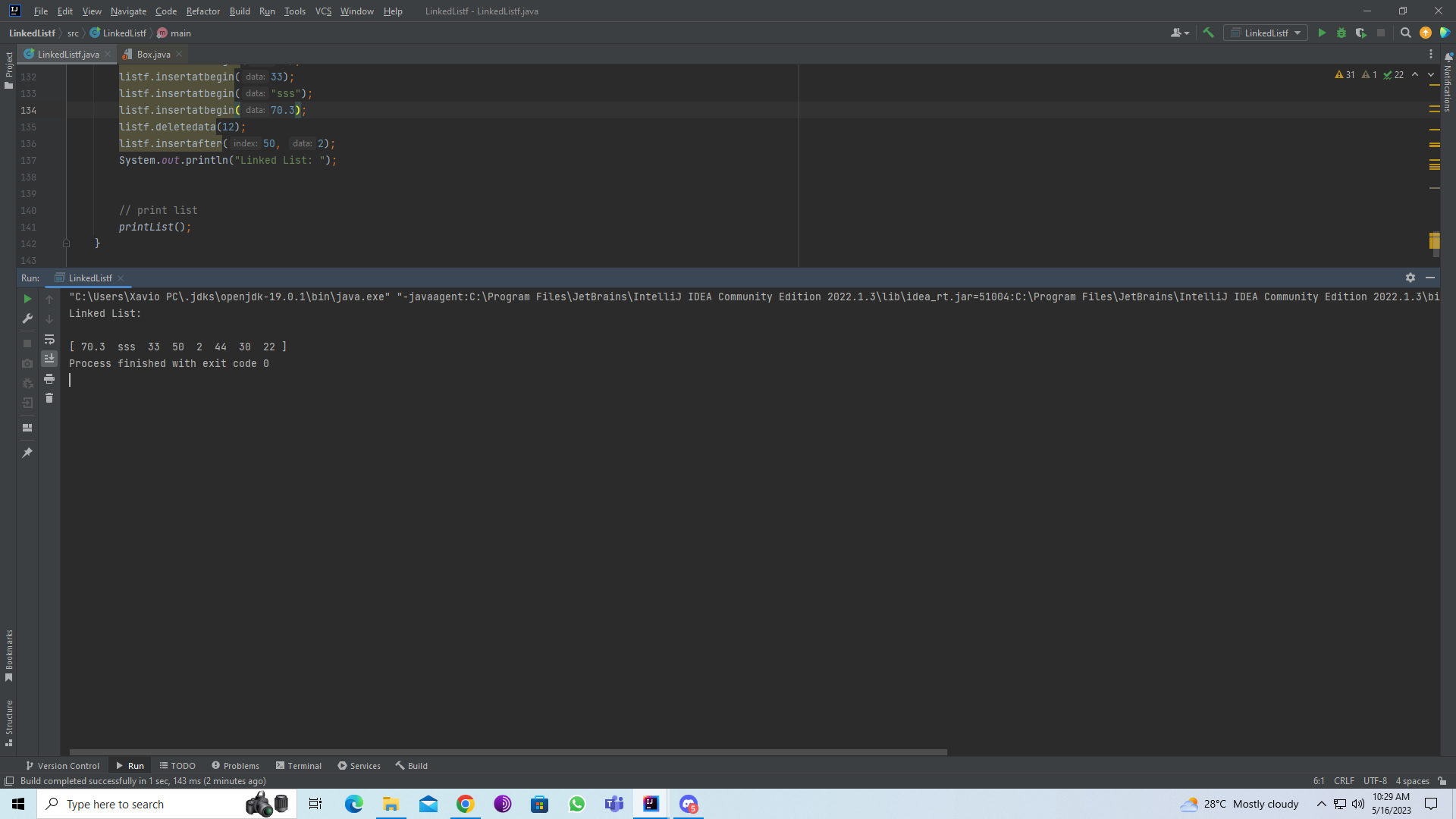
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I haven't learned PascalCase
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i usual call it camelBack notation, was confused but i get it
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
so it's Node not node?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
at the class or all of them?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
yes
i did it
also It needs to be able to input integers and strings
is it good as is or do I need to create a object for the list?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
oh sht I forgot i put that in
bare with me im kind of special
so its good ?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I'm doomed
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
oki doke
LinkedListf<String> StringList = new LinkedListf<>();
would that be generic instead of :
LinkedListf listf = new LinkedListf();
?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
ah ha i GET IT NOW
but would i get added to another list?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
so since it doesn't do this anymore
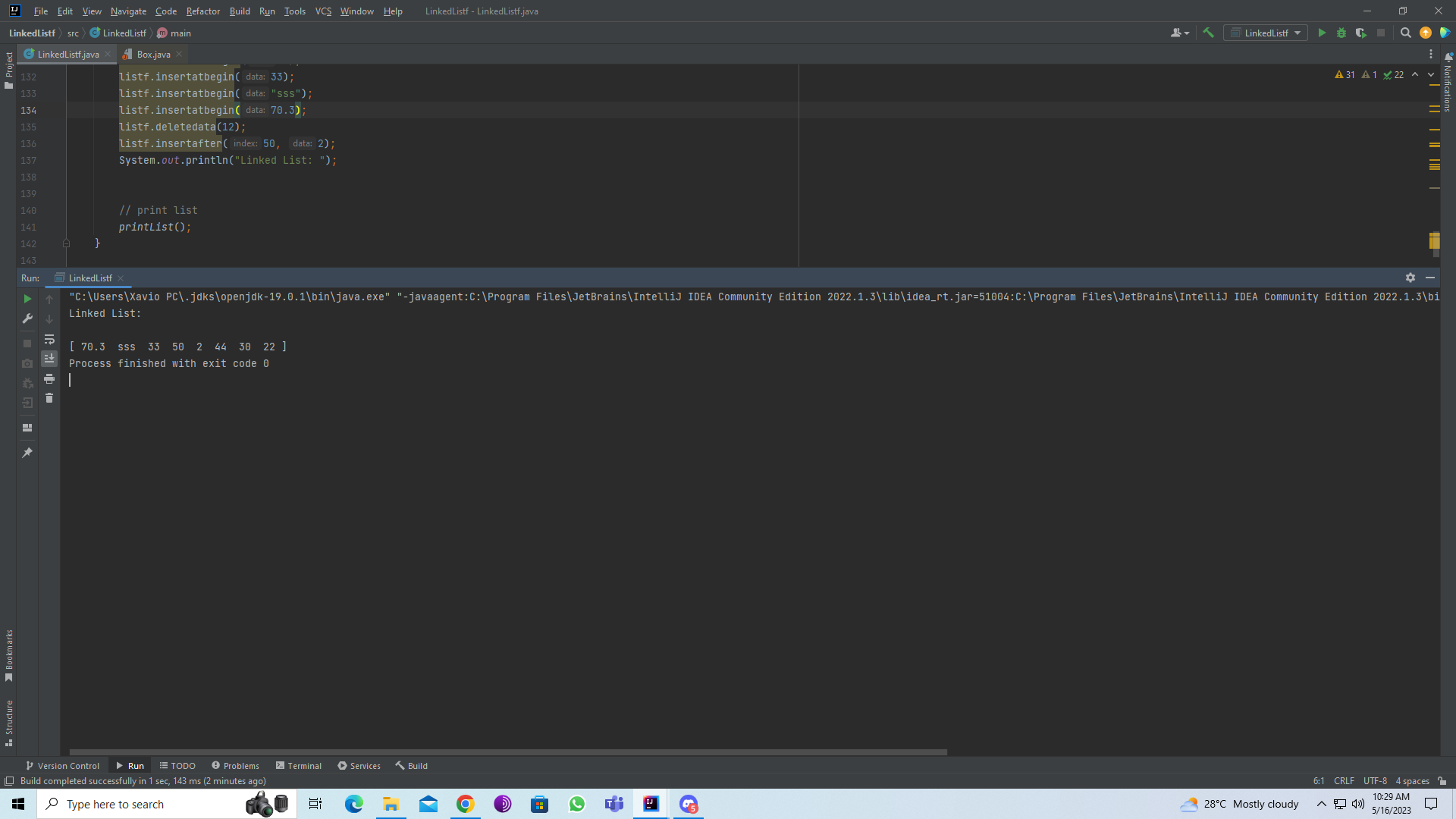
would one object list have all strings and another have all intergers?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
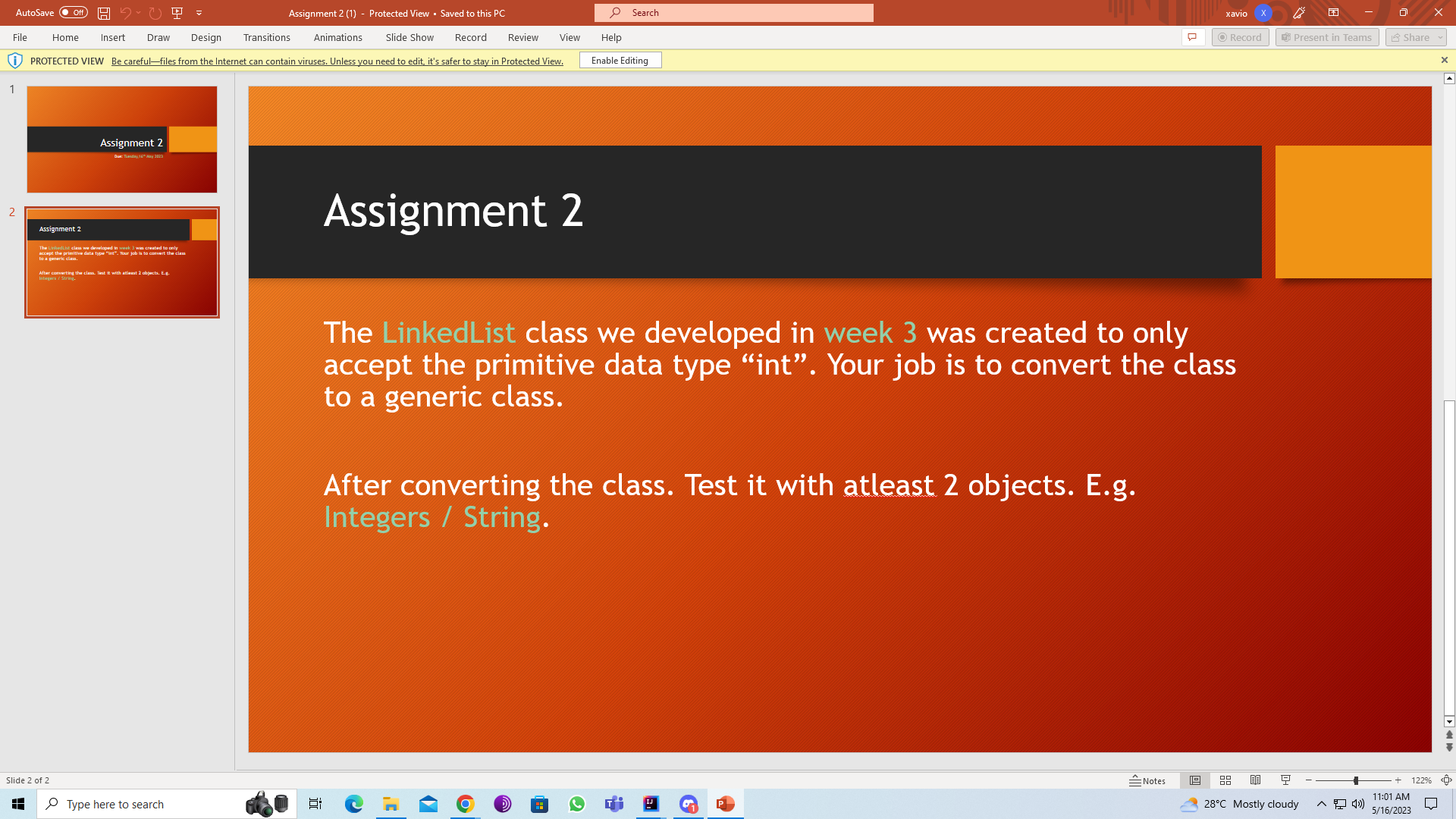
Im guessing that means two different lists
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
is this what it should do?
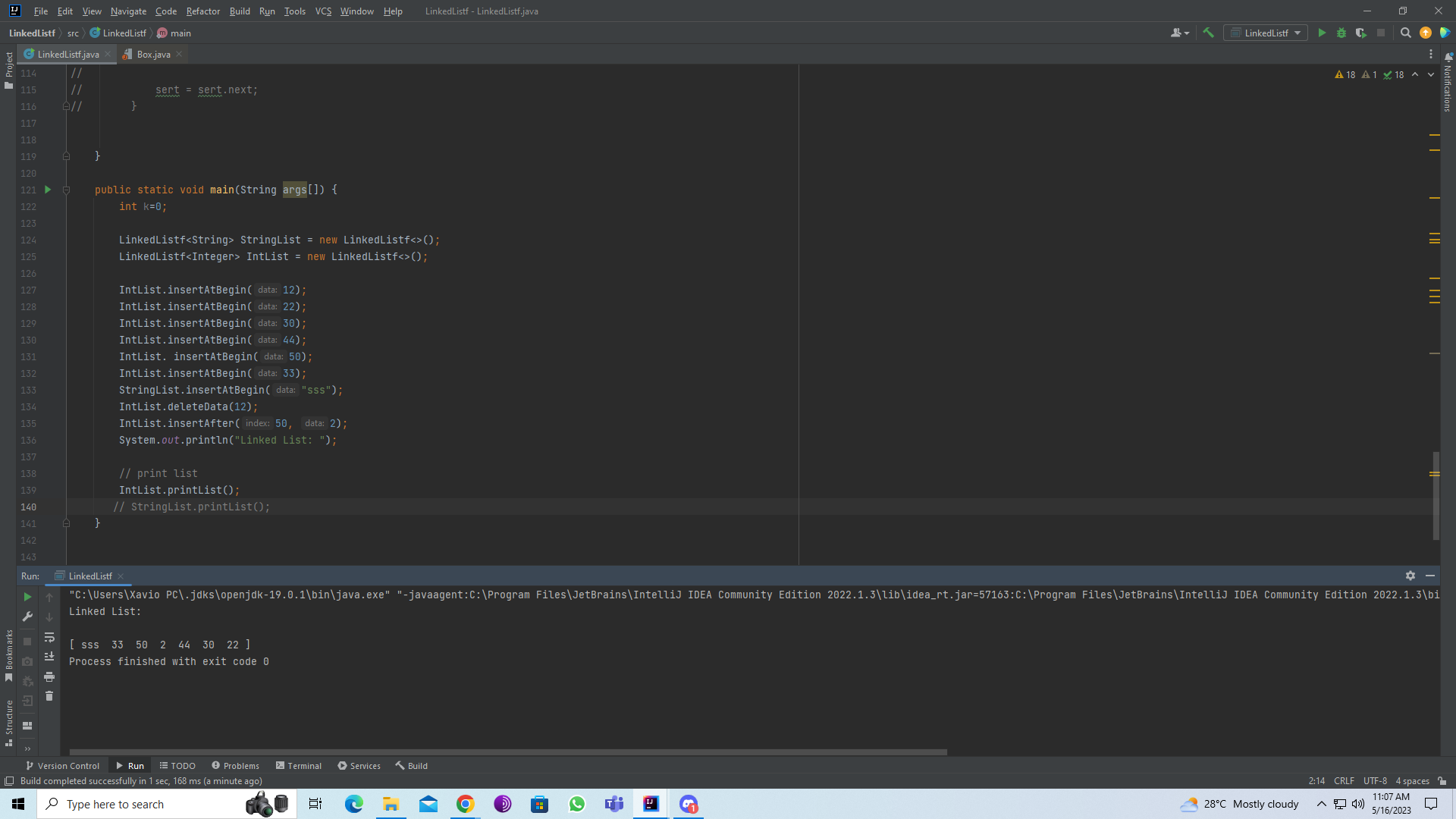
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I didn't even call String list
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
this is both intList and stringList
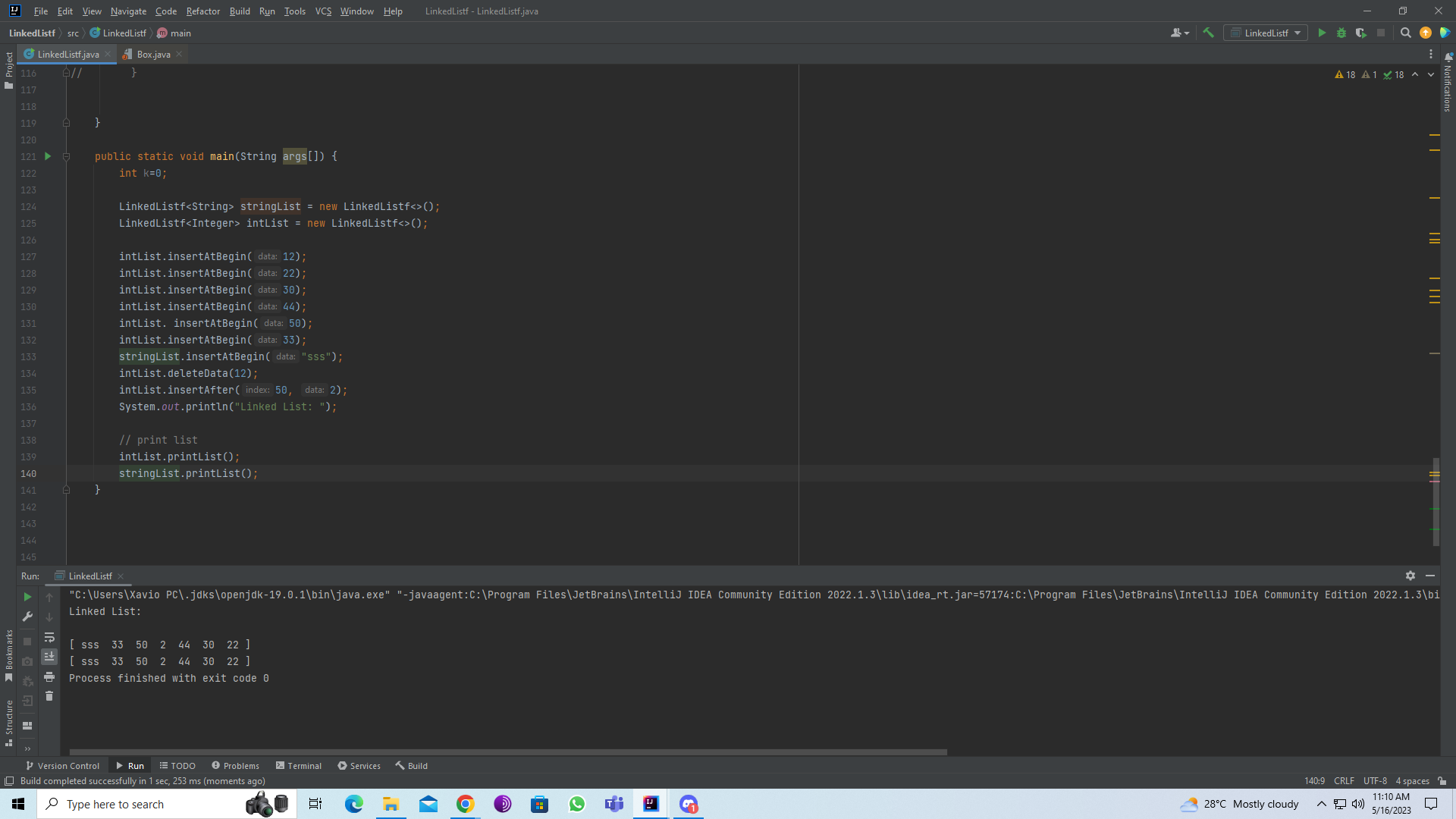
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I still had a static head
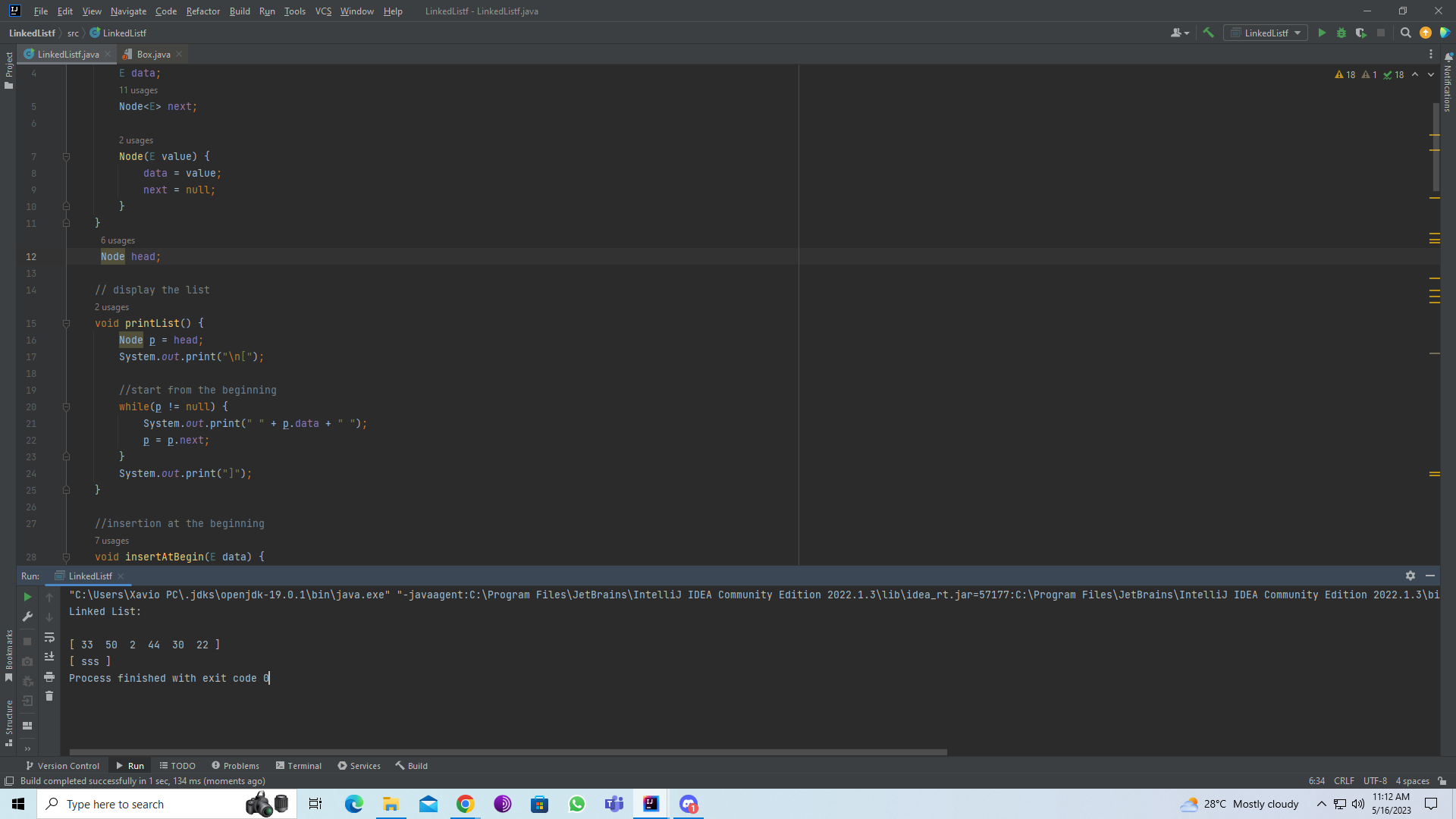
sorry lol
but its that correct?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
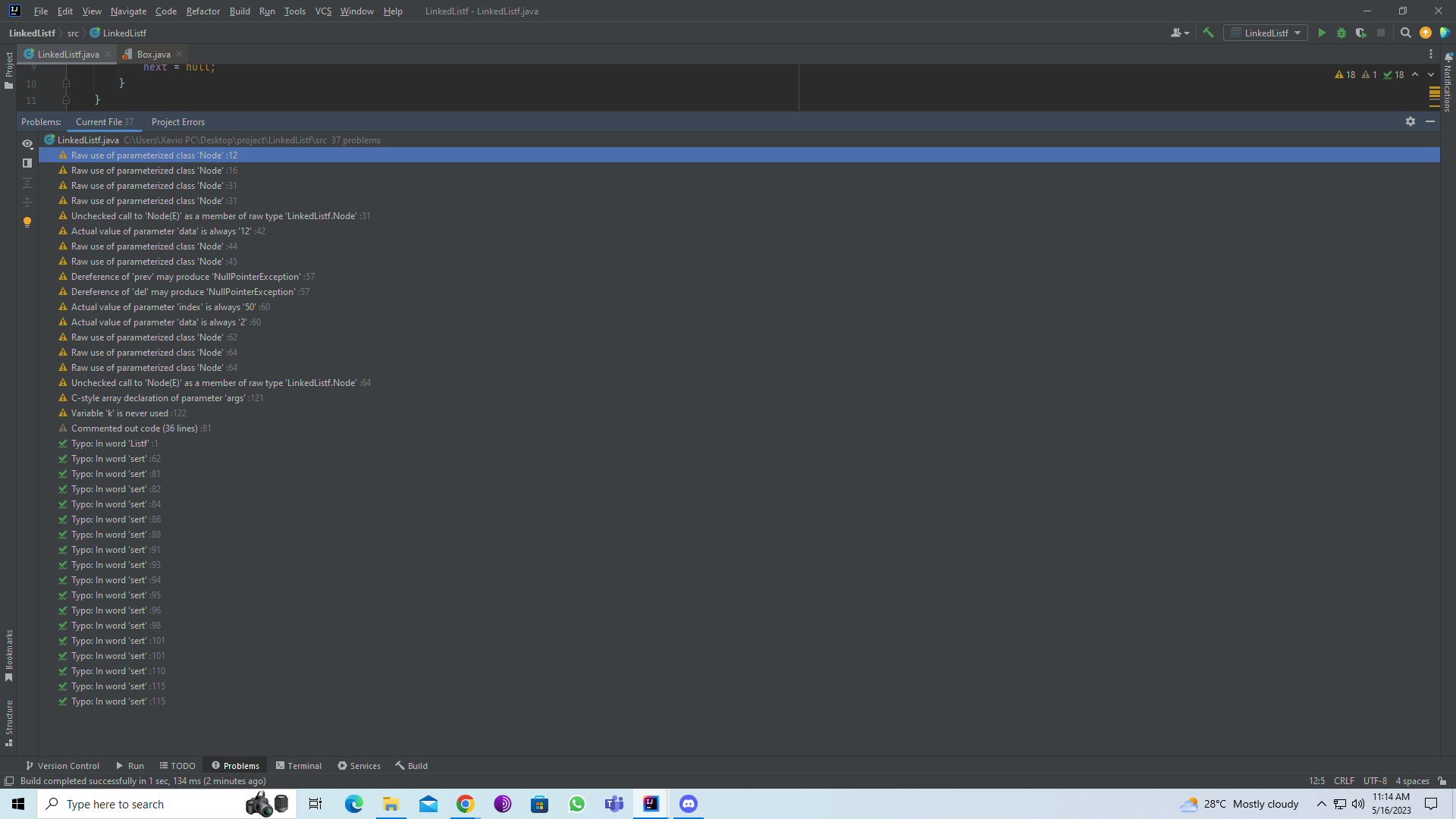
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
You're alot smarter than me idk how to fix it
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I was about to slap this bitch in for submission
did I?:pepesusthink:
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i can't even refractor lol give me some time
This is the best I could do
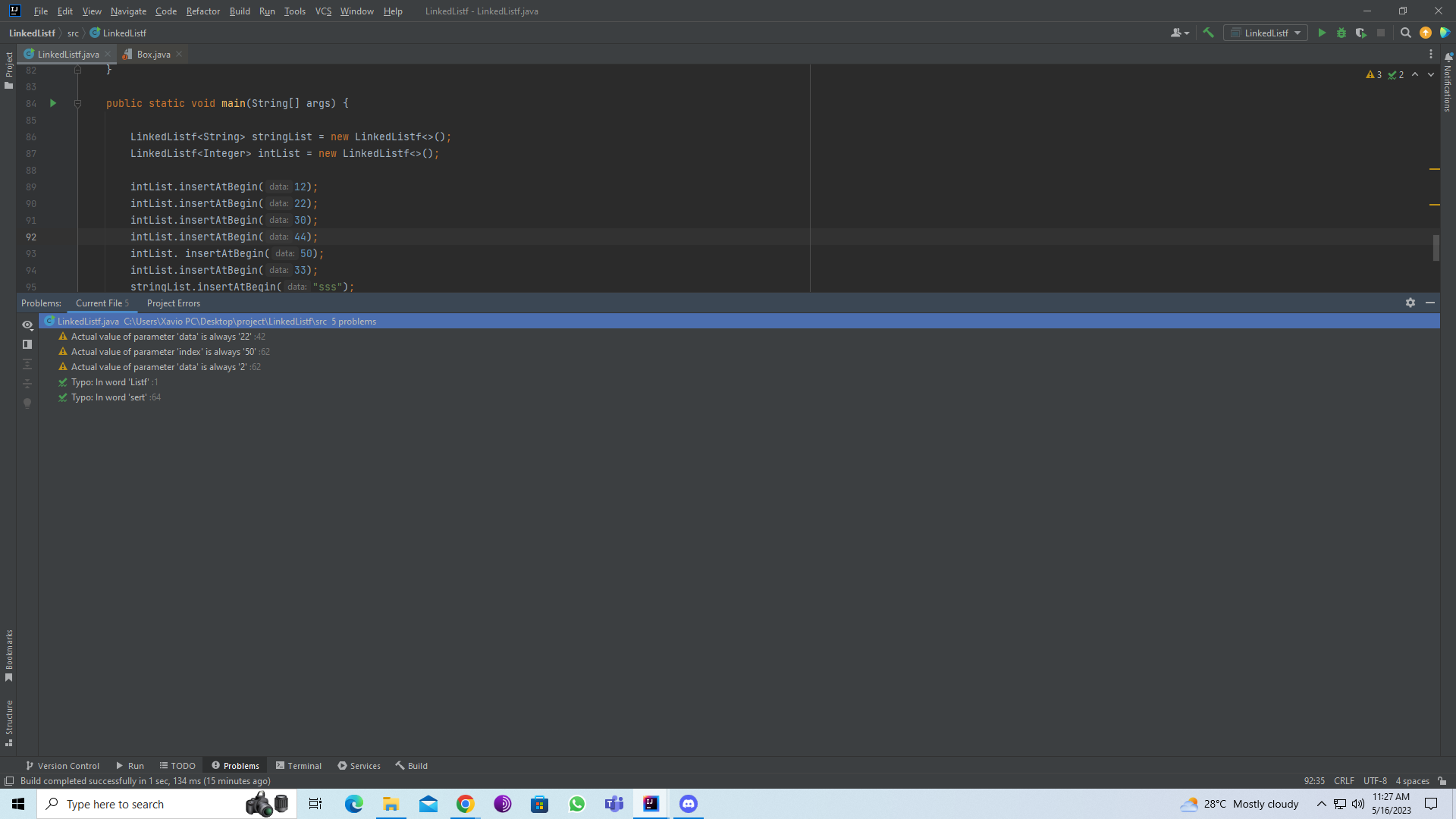
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
yes
It looks so good there i don't wanna change it š¦
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I don't know what indices are
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
maybe
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
whaaa
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
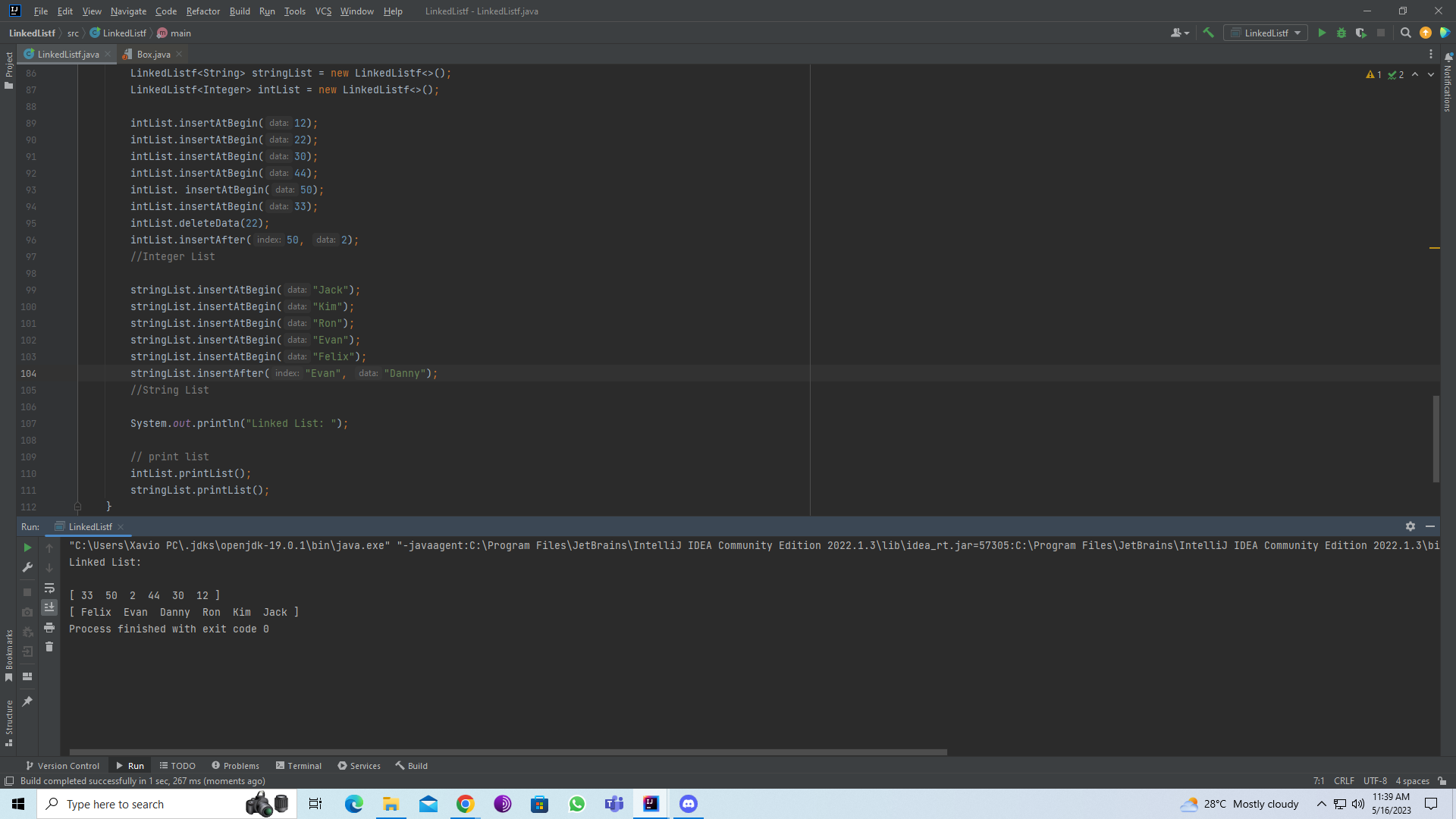
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
it won't allow me to put index function variable as int
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i have function(E index)
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i know i just randomly named it that
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i was suppose to name it replace
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i don't know what I mean ok
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
this is it
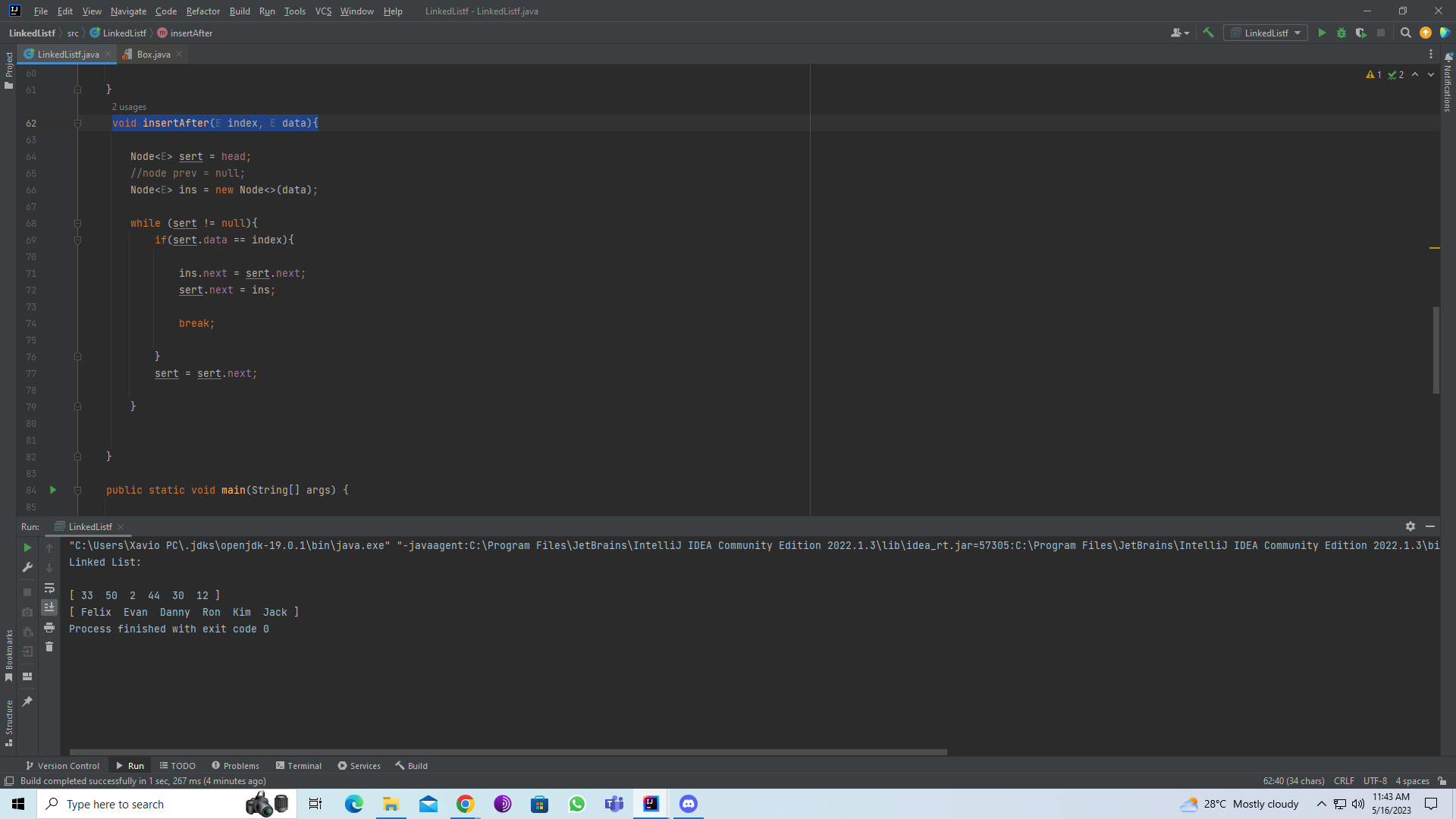
what would I name it?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
ok i'll change it
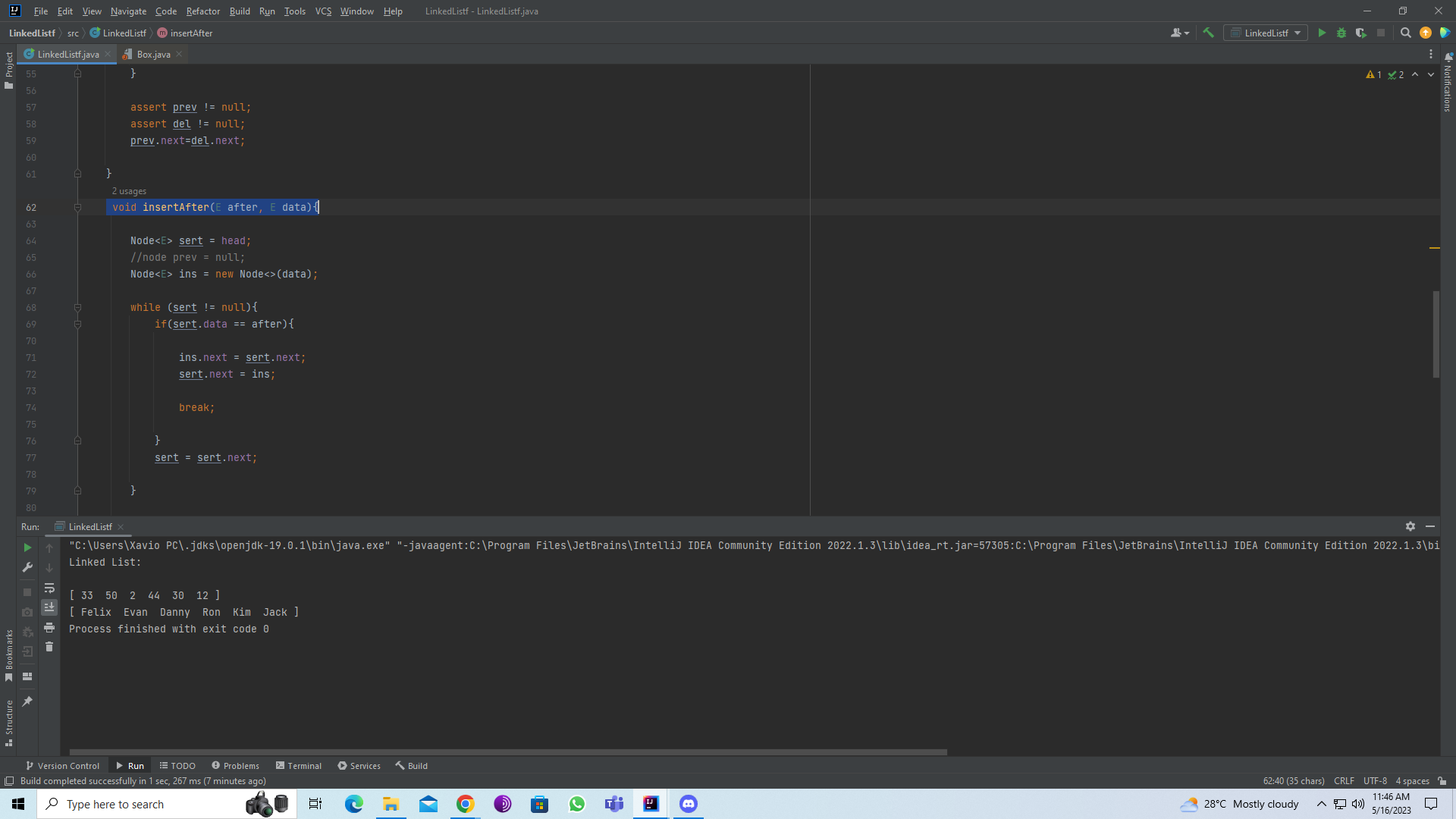
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
yes i refractored all of indices to after
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
i deleted that
i don't get how previous works
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
for the delete method
my teacher wrote that
i don't understand it
the delete function has a prev to go back through the list but I don't understand it
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
how i do dat?
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I debugged the prev variable
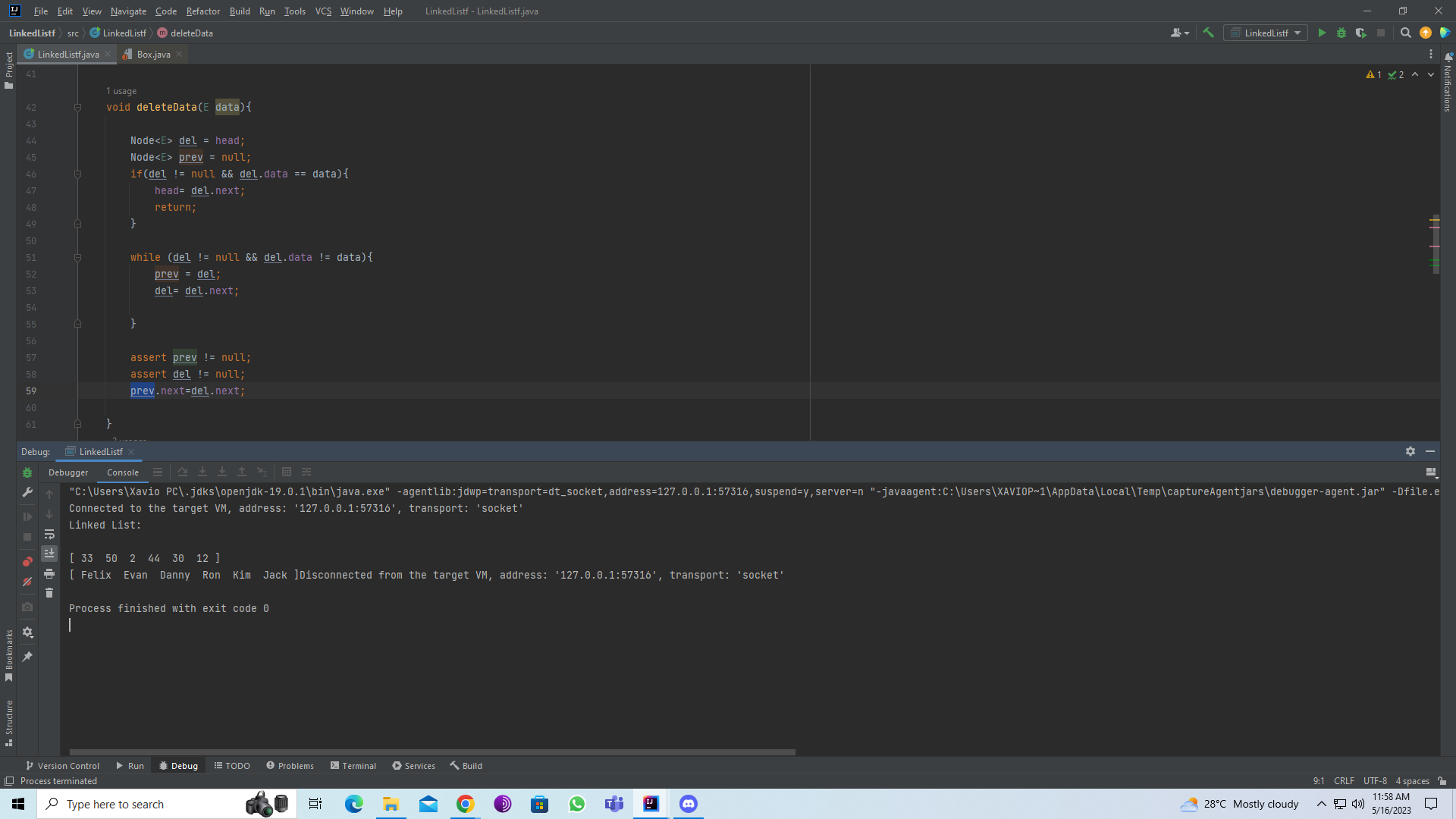
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
I've never really studied that
this?
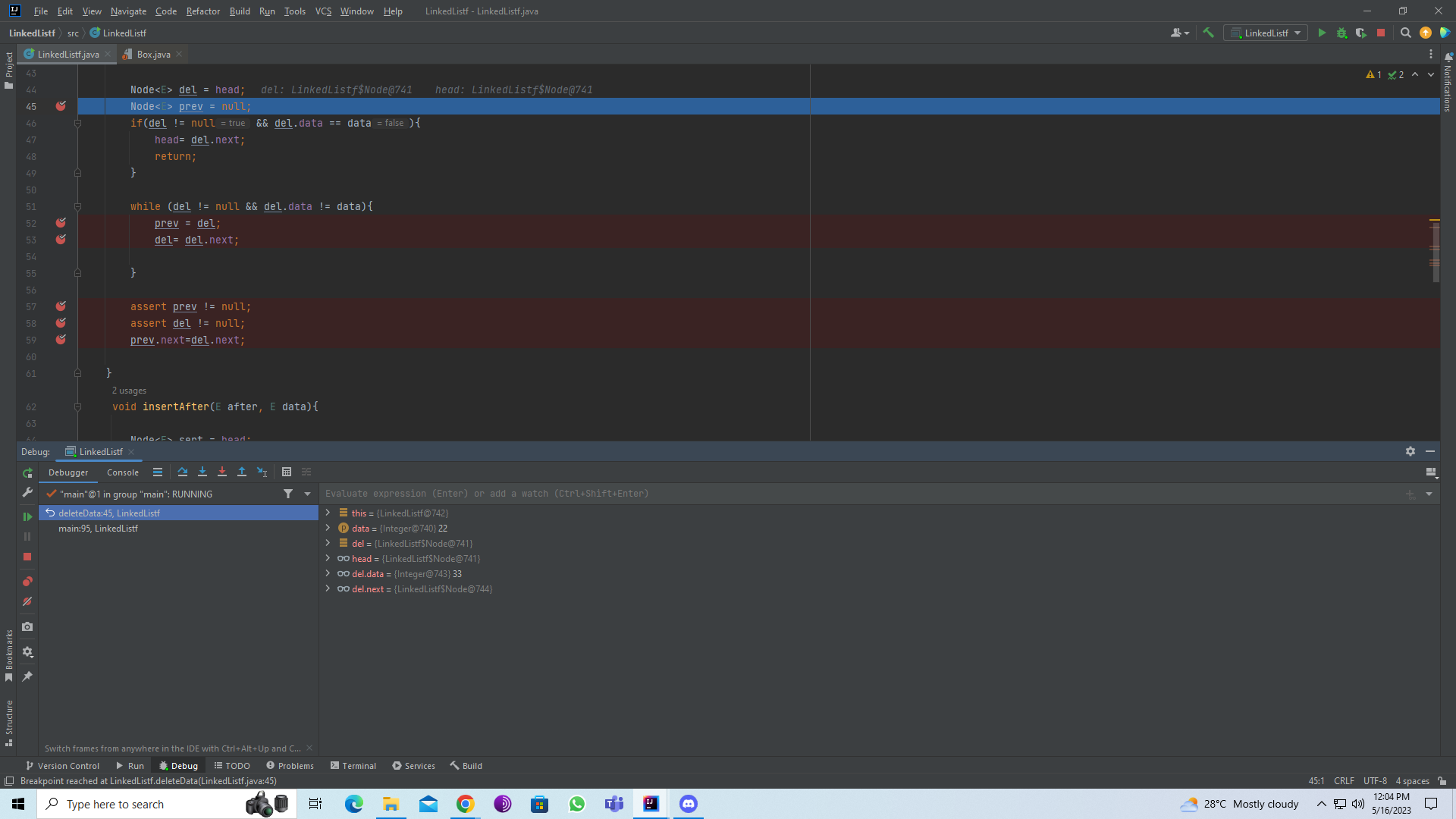
Unknown Userā¢2y ago
Message Not Public
Sign In & Join Server To View
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived. If your question was not answered yet, feel free to re-open this post or create a new one.