❔ How do I get item values from an array?
Basically, this is the array i have stored in a
var
:
[{"Example":{"updated":false,},] (i shortened it to make it more readable)
I want to get Example.updated and return it, how would i do that?74 Replies
Assuming it's not json you could do x[0].Updated where x is the name of your var
so x is my
var
, 0 is Example
and updated is updated?If this is a string then you'll have to deserialize it to a class then access that.
how would i deserialize it with Newtonsoft?
Any particular reason you're using Newtonsoft?
It's the only way I could find, should I use something else then?
thinker227#5176
REPL Result: Success
Console Output
Compile: 667.971ms | Execution: 134.660ms | React with ❌ to remove this embed.
System.Text.Json is a JSON library built into the standard library with about the same capabilities as Newtonsoft.
Alright
It says it doesn't exist
It's error CS0234
Also I'm using Windows Forms
So it happens when the form is loaded and on button click (currently only doing it on button click)
What .NET version are you using?
the latest one, idk how to view it tho
Open your csproj file
uhh where's that, in \repos?
ok opened
what next?
What is the
TargetFramework
?uh what
wheres that
in the csproj
Or like, show the entire file
i dont get what you mean

i opened it tho and its just my solution
Open it in a text editor
so vsc?
<TargetFrameworkVersion>v4.8.1</TargetFrameworkVersion>
ah, you're on Framework
which is not the latest version
k, should i just make a regular forms app?
$newproject
When creating a new project, prefer using .NET over .NET Framework, unless you have a very specific reason to be using .NET Framework.
.NET Framework is now legacy code and only get security fix updates, it no longer gets new features and is not recommended.
https://cdn.discordapp.com/attachments/569261465463160900/899381236617855016/unknown.png
You should practically never use Framework
kk
im going to use regular forms
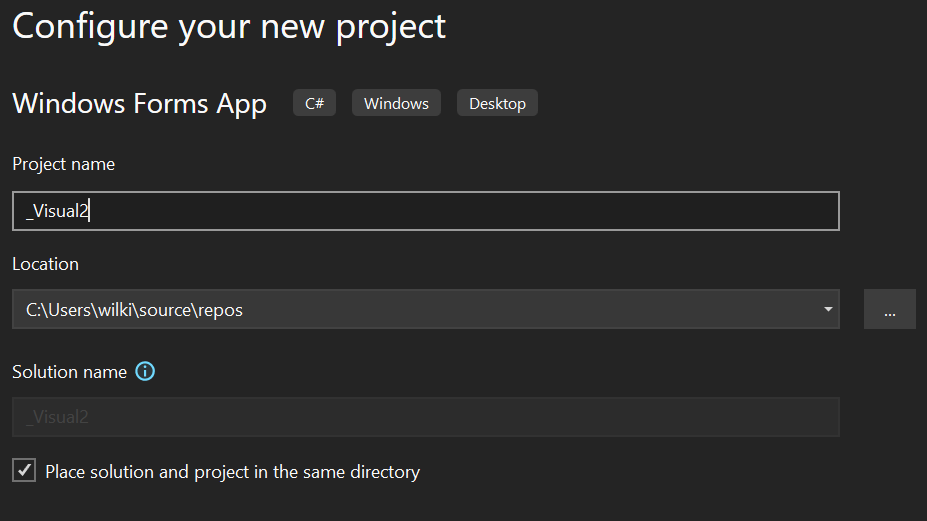
.NET 6.0 or .NET 7.0?
7 is the latest
okay, should i copy the code form my old project
I think that will work? probably
okay that worked
now how would i deserialize it with System.Text.Json?
oh god error: error CS0103: The name 'Form1_Load' does not exist in the current context
i know why but idk how to fix
ok fixed
how to deserialize?
How to serialize and deserialize JSON using C# - .NET
Learn how to use the System.Text.Json namespace to serialize to and deserialize from JSON in .NET. Includes sample code.
in the example with
WeatherForecast
, should i put it outside of the button
hmm im confused on this
@thinker227 most of the code works, but Console.WriteLine($"Example Updated: {Example.Updated}");
says that Example doesn't exist in the current context
How do I fix that?What does you class look like?
The issue is that
Example
doesn't contain a property named Updated
Looks fine
Hmm, it says an object reference is required now?
show your code
i used an api to get the json btw, thats why its different, but its definitely json as on the api docs it says it returns json
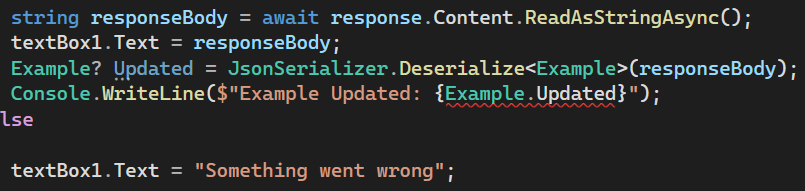
Well your variable is called
Updated
for some reason
Not Example
in the example class its Updated
Example
is a type, and Updated
is not static so Example.Updated
is not validoh ok
what should i do instead
You have
Example? Updated = JsonSerializer ...;
, so you should do Updated.Updated
Oh okay
Or you know, rename the variable to
example
I'm just trying to see if it works
So if it's an updated bool and its
Updated.Updated
will it return the value of Updated in Example class?
or would i have to do Updated.Value

how to fix?
Show your class
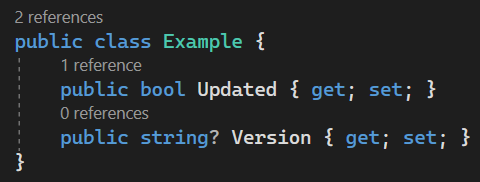
And what does your JSON string look like?
[{"Example":{"updated":false,},] shortened
That's an array first of all
oh
then why did it say it returned json
eh prob doc mistake
that is json
but the root of the json is an array
ah ok
so how to deserialize array then
It's an array containing objects containing an object called Example containing a boolean called updated
Example[]
probablyso i just need to do Example[updated]?
But the objects in the array have a property called Example
Yeah
ok so say the class is called
Example1
and the array is [{"Example2":{"updated":false,},]
what do i do to get updated from Example2?
and then change a textBox's text? (i know how to change text, just don't know the input required)If your json is then your class structure should be and you should be deserializing the string using
i'll try that out in a mo
@thinker227 error:
System.Text.Json.JsonException: ''<' is an invalid start of a value. Path: $ | LineNumber: 0 | BytePositionInLine: 0.'
on the line Root[] array = JsonSerializer.Deserialize<Root[]>(json);What does you json look like
this im pretty sure
Odd that it says something about an invalid
<
character in that case.yeah idk what the < error is
Try debugging your project and looking at what the data actually is
what
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
You're getting the error because your json is weird
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.