Zod conditional required/optional validation
I am using React Hook Form and Zod. I want make some fields optional/required based on other inputs.
Here's an example:
I want image to be required only when type: image is selected and vice-versa.
Solution:Jump to solution
if you have a more then two options this solution will be nicer
```ts
const enumToField = {
video: "videoFile",...
20 Replies
From the Zod docs:
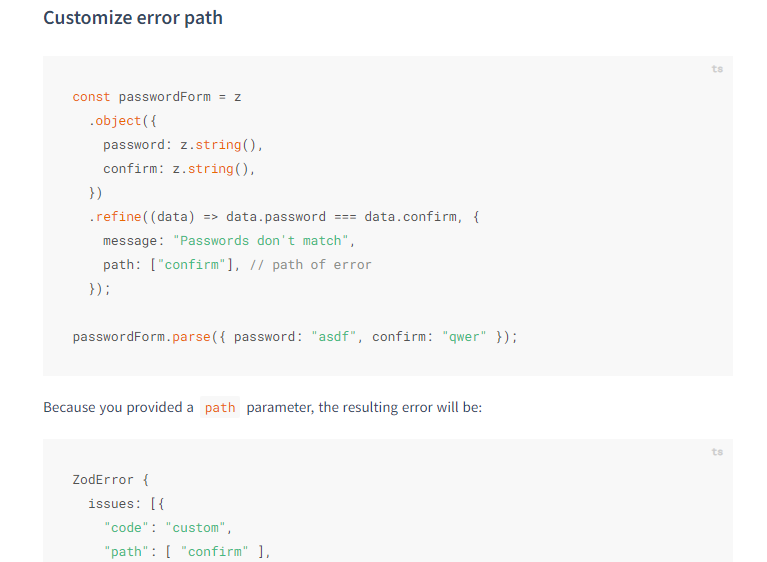
GitHub
TypeScript-first schema validation with static type inference
TypeScript-first schema validation with static type inference
In essence, you just have to use
z.refine
and check for the the conditions you want
But in any case, both will have to be optional for this to work, I believeI read it already. If you can provide an example that would be appreciated.
I want image to be required only when type: image is selected.
ok, let me see if I can do this, hold on
Something like this?
it will error if
data[data.type]
is undefinedOkay one more small addition to it, how will it work if keys does not match the enums? 👀
yeah that seems to work well
?
Then I think you could do manually?
I tested here and that seems to work
so, add a separate refine for each fields?
Sorry, I meant
No, that fails because it checks one, passes, then checks the next and fails. My bad.
The second solution should work though
Solution
if you have a more then two options this solution will be nicer
Yeah, I think in general it would just be better to match the enum
So you can oneline it like that
This seems like the best solution overall! 💯 🙏
You guys are awesome!! TYSM!!! ❤️
There is also z.discriminatedUnion
https://zod.dev/?id=discriminated-unions
So you could do something like
GitHub
TypeScript-first schema validation with static type inference
TypeScript-first schema validation with static type inference
And to include
otherNonMatchingKey
I've done it before via this sort of pattern
I used to do it via .refine
too but then I discovered that if you do it this way the type of z.infer<typeof schema>
is
which is very nice 🙂That is actually amazing, thank you for that naz
This is actually is the best way to handle this problem! tysm!! ❤️
Josh tried coding just released a video that shows this behavior very well:
https://www.youtube.com/watch?v=9i38FPugxB8
it's not in zod, naz's solution is essentially the same, but in zod
Josh tried coding
YouTube
How Did I Not Know This TypeScript Trick Earlier??!
This TypeScript trick to conditionally include types is so useful, especially for React props. I've asked myself how this is done many times before, and it's just really cool to learn something so simple yet useful.
-- links
Discord: https://discord.gg/4vCRMyzgA5
My GitHub: https://github.com/joschan21