Is it currently possible to use clerk auth with NextJS' 13.4 server actions?
I know it's currently in alpha, but I'd like to know if there is any way to use it right now.
Library versions are the latest.
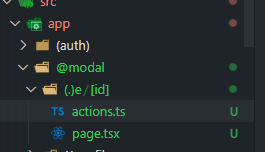
11 Replies
I feel like @James Perkins knows a lot about clerk 😄
I know too much about Clerk.
hahahaha
So there is a bug in Next.js that causes this problem. 🙂
Which hasn't been fixed by them yet, we reported it like 2 months ago or so.
But it can still be used, just gotta dig it up
Thats good to know! I was going crazy already. Thank you for your reply 🙂
Really? I'd love to know how 😄
So I think I remember correctly. you need to import auth() on the page that the action is also happening.
So for example, this should work as a server action. even though it's all on one page:
I'll try it and let you know it it works. Thanks James!
Yeah, it may or may not work it's very much a early early alpha a lot of usage seems to break even though it should work
When adding your server actions to a
actions.ts
file, it doesn't work. I like to use this pattern, bc it allows me to use the server actions in a client component.
When using clerk in a pure server component, I don't need to use auth()
to get it working.
I know it's still in alpha. Can't wait to use everything properly once it gets releasedI ran into this issue with my client component and the solution was to import the server action into the parent server component and pass it down to the client component.
When using client components you need to make sure you use prop drilling to ensure that headers are available.https://clerk.com/docs/nextjs/server-actions#with-client-components
Server Actions | Clerk
Learn how to use Clerk with Next.js Server Actions
Yup forgot to circle back. We release docs to explain how to use it today.