❔ Padding string from two sides
im trying to do padding of string from both side but it doesnt work.
The Code:
The ouput im getting:
The output i wanted
38 Replies
PadLeft
and PadRight
pads to the specified length
that is it adds #
until length of padding
is reached
if you do str.PadLeft(len, "#")
then str2.PadRight(len, "#")
does nothing
because length of len
is already reached in the first PadLeft
calloh
how can i fix that?
so
padding
is the amount of new characters to add on each side, right?yea
do
Hello.Length + padding
in the first call, then do str2.Length + padding
?
in the first one you add padding
amount on the left side
in the second one you add another padding
amount on the right side
Though I'd just avoid using PadLeft
and PadRight
altogetherwhat does
Hello.Length + padding
and str2.Length + padding
does?let's say you have
str
as the original string
and you get the amount of new characters to add on each side by doing (width - length) / 2, let's call that padding
(by the way, that wouldn't quite work with odd-number length strings)
var leftPadded = str.PadLeft(str.Length + padding, "#")
would be the original string + padding
amount of #s added on the left side
as I said above: the length you pass is the final length after padding
same goes for right padding
var padded = leftPadded.PadRight(leftPadded.Length + padding, "#")
adds another padding
amount of padding characters on the left padded stringwait u can use leftPadded() as first parameters of PadRight()?
why not
oh
isnt first parameter int?
oh
this is why you don't code without ide
nvm
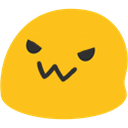
its just an console app XD
ig
though as I said above
You do need to consider this
i kinda think it may give same output as last time?
second padding would be of the whole length of the console
?
what's the doubt
apart that probably i wouldn't use padding for this
just add characters
Code i gave automatically find amount to chars to pad from both side to center main string
but it only pads from one side
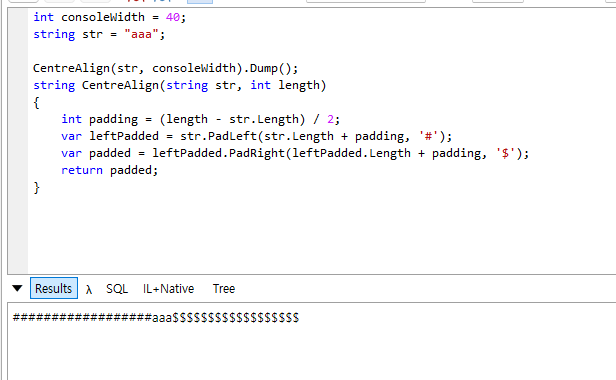
seems to work fine
but as I said this gives a string that's 39 characters in total
you'd need to add an extra character on either left or right if the string length is odd-numbered
what's the code you currently have?
the full code
you have
Hello.Length
in both the PadLeft and PadRight callsyou have already done the work yourself
where by work i mean calculating the length of the padding
you can just avoid calling pad and call instead
new string('#', padding)
that's itoutput

the second call should have
Hello_Mod.Length
what does it do?
oh
it gives you a '#' character repeated
padding
times
but that's more allocation!OHH

Thank you so much <3
Ahh can i ask something?
sure?
about this line
how actually this help?
basically using my example
with the result string being
##################aaa$$$$$$$$$$$$$$$$$$
the first PadLeft
gives you ##################aaa
which in this case would correspond to your Hello_Mod
variable
and we want to add another padding on the right side padding
times, to that Hello_Mod
variablewe could say that pad left goes from 0 to console center, and pad right goes from center to console width
so that means we want the resulting string to be
Hello_Mod.Length + padding
characters long
you did Hello.Length + padding
originally which has no effect because Hello_Mod
is already made to be at that lengthoh
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.