170 Replies
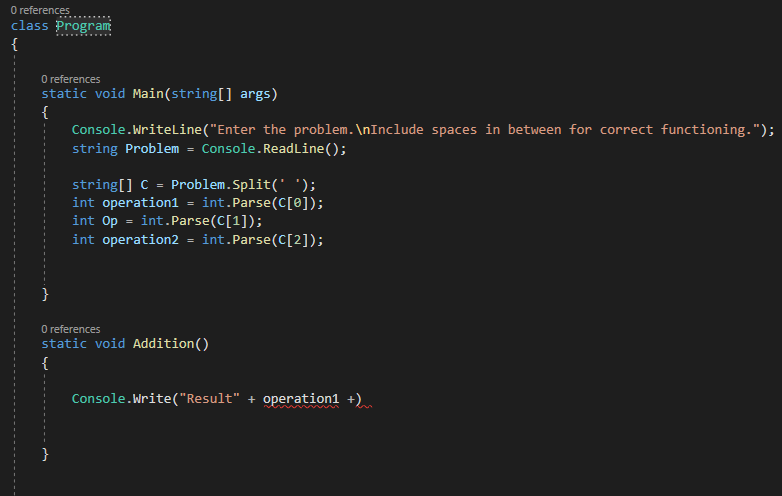
Is it possible to use the variable operation1 from the Main method in this other method?
yes. you don't show where you call addition but you could just call Addition and pass it the variables you need.
you'd need to update the function definition to take those variables as well
Addition(int x, int y)
ususally u would pass it as parameter
so I can do that every time I want to pass a variable on?
Like this?
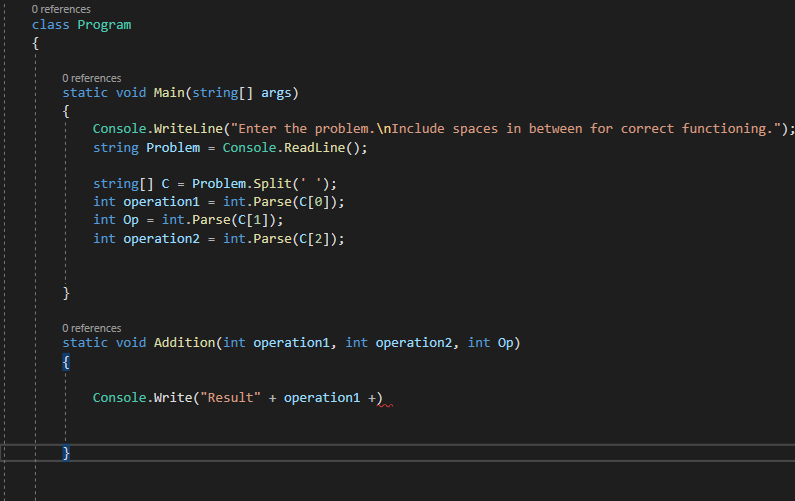
yeah you can pass parameters around functions all you want. its a fundamental part of any (most) programming language
Alright, got it, thanks.
this is how to do it right?
it seems to have worked
yes that syntax is correct.
u should rethink the
Adttion
method in a semantical way.
what is an addition? (it takes 2 operands and sums them, then returns a value)
thus ur method should take 2 parameters and return a value based on thatYeah, I can do it in the if/else way but I am trying to learn the methods
the
Op
input (i assume it stands for operator) would then be data to decide which method to call,
so u have one method for each possible operation, and decide which to call based on thatyes it is operator
Yes that is exactly what I have in mind
lets start with the methods then, and forget about user input for now ;p
If the Op is +,
Addition();
if the Op is * and vice versa
from that info, how would u write the
Addition
method in $code?To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/
I am thinking of this
but what if you want to do something with that number after adding it
I dont think int Op needs to be transferred
it does not, and operator also wouldn't be an int
I haven't thought about it
lol
Oh
this method hasissues in my opinion:
1) its called
Addition
so its very clear what it should do, thus the Op
parameter should be needed, right?
2) the method does something with the calculated value, thats not its job. its job is to calculate the value and return itwait what does this mean?
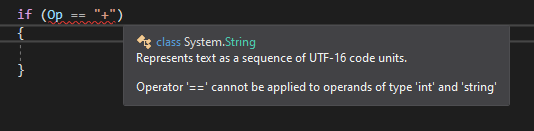
imagine having this line of code:
int someValue = Addition(1, 2);
,l there u would expect that someValue
is 3 afterwards
u also would not expect that something is printed to the console at that time
well, '"+"' is a string, basically zero or more characters (basically some text), Op
is an integer value. its simply not defined how to compare these different typesI want it so that in the main method, if the Op = + or * or anything else, it will perform that specific method applied to it
that was my initial conception but it seems to be failing miserably
u need to think about how to split ur task into minor task
1) take input
2) process the input
3) display the results
So how do I fix that
what type is Op right now
its an int
thats the order in which ur program will execute, but from the programming side, u should first think about which methods u should write and how to write them
(afterwards we talk about handling the user input)
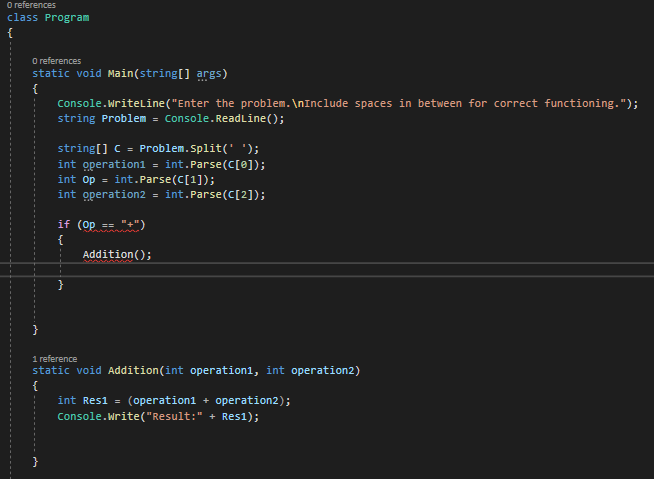
getting errors here
so you're suggesting I write the other methods for all the operators first?
you can't really compare a number to a string. because they are just completely different. What type do you think Op should be?
basically yes, but lets stick to
Addition
for nowString
as earlier explained, that method should only have one job. doing the addition and returning its value
do u know how to return a value from a method?
(i do not mean printing it)
return; ?
the
return
keyword is use yes
return;
only works for methods that return nothing (void
), but thats not what ya want right? u want to return the sum of these 2 operands, so u want to return an int
Ohh I get it now
then show the code ;p
So I should get rid of the Console.Writeline from the
Addition
method, return the int to the Main
method, and show the result there?yep
how do I return the integer? :P
first of all u have to rewrite the method header
static void Addition(...)
-> the void
says there is no return value, so if u change that to another type, u declare that the method returns that type (in ur case an int
)
this?
yes
yep
now you have to return an int
I get this
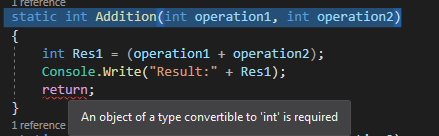
return;
which value do u return here?
none ;pthe Result
Res1
return (Res1);
yep
u dont have to use the parenthesis tho
Oh okay
return Res1;
would be enough
and totally get of the Console.Write()
call thereConsole.Write("Result:" + Res1);
So this should work in the main method now?
yeah I did it
well, dont think that the
Res1
in the Addition
method and in the Main
method are the same variables
they just happen to have the same name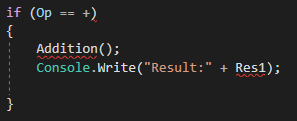
the method takes 2 integers as parameters and returns 1 integer as result
Yeah
so
int result = Addition(1, 29);
would be a way to call the method and storing its result in result
what's the 1, 29?
afterwards u can use
Console.Write("Result:" result);
to print out the result
just example values for the parametersdo you mean something like this?
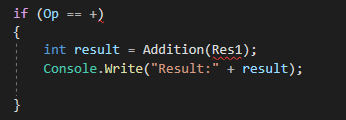
these can either be values (as shown already) or variables of the type
int
yeah they are variables
the operands, the user inputs them
yeah, i simplified it for the explanation ;p
would basically the same
I am confused, shouldnt this work?
beucase in the
Addition
method I already assigned the sum of the operands to the variable Res1 and returned itnow u would just have to replace the numbers with
int.Parse(...)
to get the actual user input for the operandsyes I've done it
this is the code
but what value do those numbers actually have? you haven't told the function yet
the
Res1
in that method is another variable that just happens to have the same namethey are variables that will be gathered from ReadLine
yes, but you never told the function that, you gave it one parameter
Res1
thats not defined in that scope. A variable created in a function doesn't exist outside that function unless you return it and store itthese are so called local variables. local as in that they only exist in their scope (basically in the stuff between the { and } )
Okay so it seems like I have returned it, but haven't stored it
is that it?
int result = Addition(Res1);
u stored it in result
int result = Addition(Res1);
this is you storing the result, but addition takes two numbers and adds them, you need to give it two numbers Addition(x, y)
yeah the private class and public class?
tho u did not pass the correct parameters
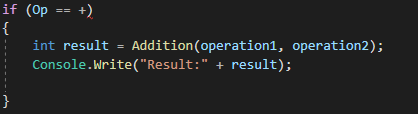
Is this what you mean?
public and private is something else, forget about it for now
thats correct
Oh I got it, the method doesnt store in the variables, but just the function
it's like a recipe book
sorta, eye
*yes
so in the main class, I use the variables as ingredients and use the methods as the recipe book to quickly cook it
that is correct, now Op is a string, and + is the addition symbol, you need to compare Op to the string "+" as thats what the user will be inputting
quite literally
yep, but one addition: its not the main class but the main method ;p
yeah I miscalled it
Wdym compare?
ill quickly smoke outdoors and return for the rest of the stuff (will take ~5min)
Op == "+"
compareAlright, you have helped a lot, thank you!
I am grateful
if (Op == "+") so like this?
yes
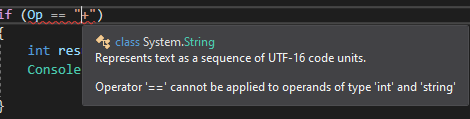
What type do you have Op set to?
int
and what should it be
its not a number, thats for sure
string
ints are only numbers
yeah
but if I do that it messes up my int.parse
I cant split the operands and operator then
maybe you shouldn't Int.Parse that one
because its not an int
you know ahead of time its never going to be an int so just don't try parsing an int out of it

Oh
will this work?

parenthesis not needed, but yes
I think it should, it will still take in the array
okay let me give it a try
It works!!
back from smoking ;p
so whats ur current code?
I added the 4 basic operators
it works perfectly
thanks a lot both of you!
I am really grateful
glad to serve ya o7

i have some hints on that
you can use
result
for all of them btw.Oh
because they are all the same if statement only one of those would ever run
oh correct I forgot
the operator can't be two different things
first of all, dont use these
ResX
variables in each (else) if
branchI am stupid
also I think I can just use Res for the methods too, right?
or will it mess it up
that way u will have one variable that stores ur data
you could, but since you aren't doing anything else with the variable, i wouldn't even store it
return operation1 + operation2
and after these if else stuff u can use
result
to further process it (like Console.WriteLine("Your result is" + result);
)That would make it shorter, good idea
yeah there is only 1 function
so no need to store it
yeah as clear and concise as you can make it is best
often shorter is better, but sometimes it makes it a lot harder to read
So I can have one for all of them?
also in ur different methods for calculating (
Addition
and the others) u have these ResX
variables
they only exist in the method, so they all can have the same name
these are totally valid, and the result
from Addition
and the result
from Substraction
are completely different variables that only exist within their scopes/methodsis this what you meant?
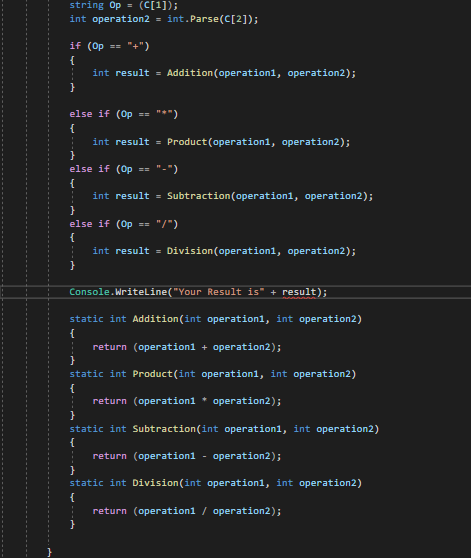
of course the
return a + b;
variant as LIVE suggested is valid as well and u dont even have to introduce a variableit cant find result though
almost
variables exist in the scopes where they are declared
what's the mistake
a scope is basically everything between the { and the }
yeah
because u want
result
"outside" of the if/else if stuff, u need to declare it before that in the outer scopeI hate dealing with them, it always messes up
so I can declare it as 0?
and that value will get updated
in the if and elifs
(im too lazy to write it out, replace the ... with code xD)
yep
and then afterwards u can still use it
for ur
Console.WriteLine
call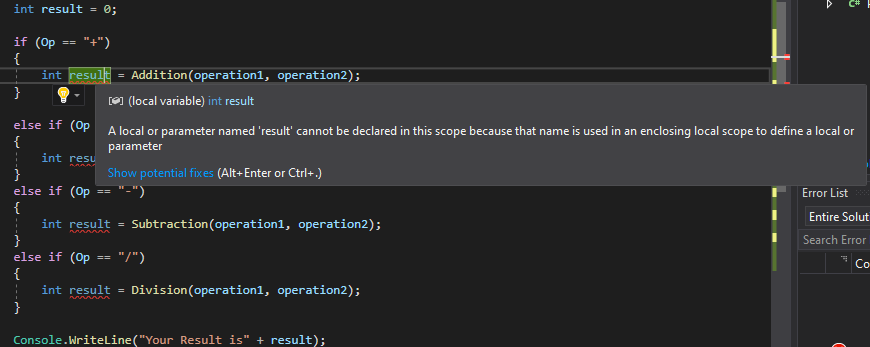
should I remove the int
yeah, u are trying to declare a variable again there.
Type VariableName
is a declaration (in ur case int result
) but it already exists, so it complainsI think that is it
yeah
correct
I was just thinking that
u just want to use it there now, so just use the variable name
result = ...
(no int
there)
there we go
it works perfectly too
that should be correct, but i still have 2 things to mention
Hold on I'll be back in 10 mins
first of all: variables should always be camelCase (basically starting with lower letter (a instead of A), and only on word boundaries start with a higher letter)
so
op
instead of op
.
actually the naming has more to it: Addition(int operation1, int operation2)
, these variables arent operations, but operands, so they should be called that
additionally, because this is a common math thingy, its usually lhs and rhs (left/right hand side)
and dont be lazy on names, op should be operation in this caseyeah thats an example where shorter also makes it worse code, because its less readable
the last thing:
u defined the methods Addition and alike inside of Main, u should not do this, do it in the outer scope
another thing i'd add, what if you were to do "5 / 2" what answer would you get?
like
(currently just like variables, these methods only exist within the scope of the
Main
method, which is a bad design choice)
there is ofc more to it, but thats far away from the beginner level ;p
so all in all i would rename Problem
to problem
, C
probably to something like component
and the operationX
to operandX
(where X is the number)
if u have adjusted that, please show ur code again ;p
(there is more stuff but im not sure if im overexerting then)Okay, I got it
yeah I wasn't sure what to name them so I just put some jibberish
I'll have to use the %
yeah I fixed it now
thats the point, always give it a meaningful name, even if its long.
imagine more complex code u revisist afte 6-12 months, u would not understand a thing with weird naming ;P
*cough*
You could, that would allow you to stick with integers.
But there are some different types that handle decimals better ( better said, they handle floating-point operations)
there are 3:
float
, double
, and decimal
but for these purposes I would just start with float, its the smallest of the 3 and has the least amount of precision but its best practices to only use the types you need to use.Yeah I've used float in python before
I guess it's similar here
yeah python just doesn't enforce typing so its a bit different
float result = (operand1 / operand2);
I hated how stupid it is to create an UI and executable
python is good to learn programming basics on, but overall its a worse language for actual programs. I like it for scripting and light repeatable tasks
its also so much slower, even though it uses a lot of c
Yeah true
should I change all these integers to floats?
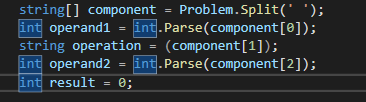
you could, it depends on what you want to support. If we are talking full calculator functionality it would have to be all floats, a calculator can handle
0.3 * 1.2
just fine.well it works now
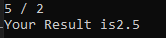
Well, gonna go get some sleep now.
Thanks for the help guys, it means a lot
learnt alot today!
u can do really pretty stuff with python, simply because most modules r c binaries instead of pure python
its also so much slower, even though it uses a lot of c*nods* glad i could help o7 if ur questions here are answered please $close the thread to mark it as answered 😉
Use the
/close
command to mark a forum thread as answered
Use the
/close
command to mark a forum thread as answered