JS functions
Hi again!
Why is this code printing undefined at the end?
The 'Hello ...' text is printed first, then underneath undefined is printed. I really don't understand what is not defined in this code.
23 Replies
alienShip.invade()
isn't returning anything, so the argument to console.log
on the last line is undefined
and in fact, that comment about the console.log not being printed is invalid, it doesn't matter where you call console.log, it always gets printed to the consoleBut if I comment out
console.log(alienShip.invade());
then nothing is printed to the console ...because you're not calling
alienShip.invade()
anymore
remove the console.log from that line instead of commenting it out, and it'll print the 'Hello!' text
while keeping alienShip.invade()
I meanYeah! Now it works.
I mean ... this codecademy course is good in terms of understanding the building blocks, which is obviously good for beginners. But it is not helping me understand how to read code – at least that's my feeling. Obviously I also have to do this a billion times.
Especially functions where you have to go back up and re-read it to understand the path of operations ...
the thing you seem to have missed here is that function bodies (whether defined as a property of an object, using
function
or arrow notation () => { ... }
) don't run until they are called. When it's defined, that just creates something you can use later to run the code insideYes! Cause I was also spending waaay to much time on understanding the global vs. local scope aspect when they went through that.
Jumping in a bit after-the-fact, but one important thing to keep in mind is that, in JavaScript, functions always return a value of some kind. If you don't explicitly
return
a value it'll return undefined
because no return value was defined.
Since your alienShip.invade()
method has no return
value the console.log
is logging undefined
. If you instead return
ed the string inside the method instead of logging it, then the outer log would log the text.If you haven't yet read https://javascript.info I highly recommend it. It's one of the best free resources for learning JS to date, in my opinion.
So with regards to this – where do you put return, and can you then use
console.log(alienShip.invade());
at the bottom?Yes! You've mentioned this before, and my plan is to finish this intro course (objects is the last part of it), and then go on to your JS tutorial suggestion. Just because I had started the codecademy one already ...
With that, every call to
alienShip.invade()
will return the string instead of logging it to the console. What you do with it after that is up to you. You could log it yourself, as is in the last line, add it to another object, combine it with other strings, etc.Which is also dependent on what you want to use it for later I guess. But if this was the only code that was being used. Is it best practice to do the one without
return
and only call alienShip.invade();
, or the one you now wrote?There really is no "best practice" for this b/c it's a simple example designed to show you how to use methods. In production code more often than not you'll want to return some info so the calling scope can use it how it's needed. Logging random text to the console isn't a function you'd have in prod
Logging is almost always used for debugging purposes and is a side effect of the main point of the function. You log stuff to know what's what when the function is being run, but other things happen within the function and that's the main point of it.
That makes sense, since they constantly tell us to log things to understand things ...
Another side effect of a intro course, which obviously is useful for now.
Thanks again guys. I feel it's very basic, but hey – in a years time ... 🤓
looking at the inside of your code is a really good habit to have, and console.log is the simplest way to do that for a beginner. It's just not something that should ever really run on the client's system (not that it's the end of the world if one or two slip through)
If you don't get the basics down you won't be able to get to the advanced stuff. Start basic. Ask questions. Learn!
You're doing it right, 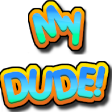
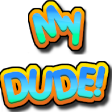
once you get a bit more experience, it's worth checking out the debugger in the dev tools, but knowing what variable values are at which points of your code is so important. Sometimes I think half the questions on here would be solved if people learned to inspect the state of their code better
Yeah, and I had to come back to the functions part because I was overwhelmed by them when they became methods in objects. I thought I understood them first time around, but that was not the case. 😅 So another take away, especially with beginners courses is that they, obviously, tend to focus more on the parts, and not the context so much.
it's one of the concepts I see a lot of people struggle with, honestly. You're definitely not alone in having to take a couple passes at functions 🙂
and like Beck said, you're doing the right thing in asking for clarification, it's the best way to learn
🙌
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
They've introduced it, but I think it was so abstract that I missed that I didn't really understand any of it. I'm excited to get back into it. 😅