❔ How would I implement a toroidal universe?
A while ago I had started a project to hopefully create John Conway's Game of Life. I have everything else figured out except how to implement a toroidal universe to where the boxes wrap to the other side of the console window. I genuinely don't understand the math behind it and I need a bit of help.
14 Replies
Since cells in Game of Life only check their surroundings neighbors, you could just check whether a cell is on the edge and then pick cells from the other side a neighbors, if I'm understanding the problem right
Sounds like a modulo usecase
my brain hurts already
would you like to see my code for the finite portion of the game of life?
Arithmetic operators - C# reference
Learn about C# operators that perform multiplication, division, remainder, addition, and subtraction operations with numeric types.
thats the finite universe.. thats how i implemented the math there.. now i am so unsure how to do the toroidal
(btw it's a c# server and you're using c++, but there's barely anything c++ specific in your code and c++ has modulo too)
Im.. oh wow
I genuinely didnt notice its been so long since i opened this project
Like since January. I have had a lot more projects lol. Ill close this then. Thank you!
Did you get this (besides the C#/C++ divide) figured out? If you still have questions about the actual implementation, happy to talk about it (though maybe tomorrow lol)
I did not get this figured out no. Im genuinely so confused
Do you understand the basics of modular arithmetic? If not, that’s totally alright, we can go through it! (If you want)
Tossing this out there while I remember
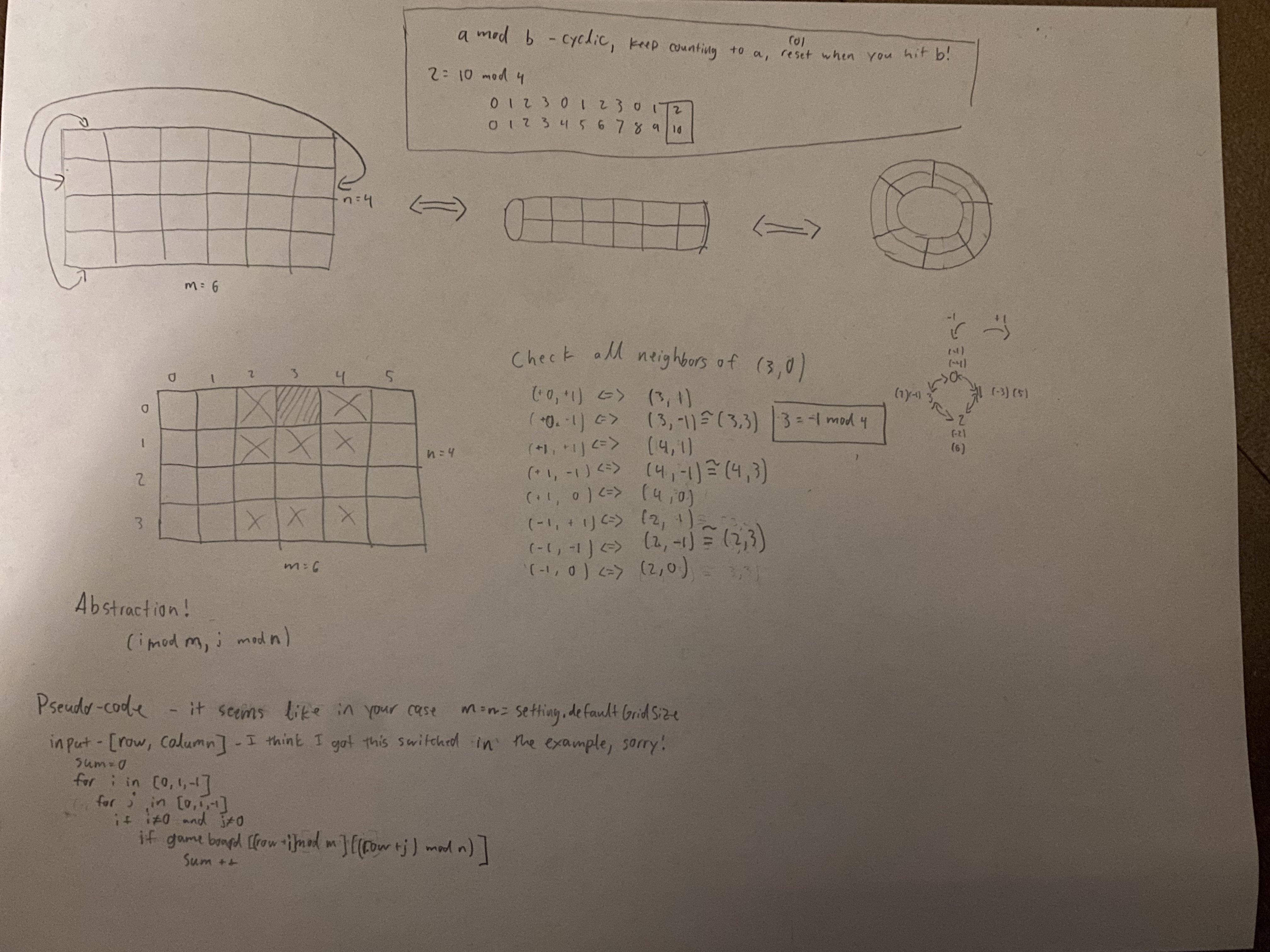
We should probably do this tomorrow im sorrg im super tired
Haha yeah same for me. But insomnia so I figured I’d at least take a look and jot down some stuff
Basically, if I'm understand correctly, the main thing you are trying to do is get the count of all live squares near a coordinate, including wrapping around to the other side of the board. The concepts behind modular arithmetic are pretty simple, you just keep counting and reset to 0 every time you hit, very similar to if you were counting on a clock which is the same as mod 12.
In your case, it looks like you have a grid of size
settings.defaultGridSize
and it is a square, yay! That means we have less numbers to keep track of.
Honestly, most of the time working in this sort of space makes code a bit simpler. You can reduce a lot of your checks because you don't ever need to worry about a "surrounding square" being out of bounds. So, for example, taking
You could just check and see if gameboard[(row - 1) % settings.defaultGridSize][(column + 1) % settings.defaultGridSize]
exists, and then increment the total (I think this is how you use modulo in c++, I'm not really a dev so please take any random code snippets with a grain of salt)
Because you are taking the remainder, even if, say, column + 1
is outside the range of the board, it will "reset" the value, effectively "wrapping" it over to the other end. So if you were in a 10x10
grid (which means we will mod by 10), numbered 0-9
, and your column value is 9, then (column + 1) mod 10 = 10 mod 10 = 0
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.