❔ Using inheritance in my project
I'm building a book shelf project and I need help with modifying my ctor so I could assign each input for one of the subclasses or the books https://gist.github.com/Programmer-Faraj/9a2f90285e21996c4cf7577505ce6853
71 Replies
what's the question?
After taking both user inputs for the title & author, I would need to use the public string Type; field to assign one of the se books objects from my sub-classes
what's an "se book"?
also, what
public string Type;
field
also, why would that be a field?sorry, I meant these not the se.
ah
so, you've got some UI pulling Title and Author values from the user
and then you need to create some
Book
instance for theseIn my base class you can see that I have defined a field
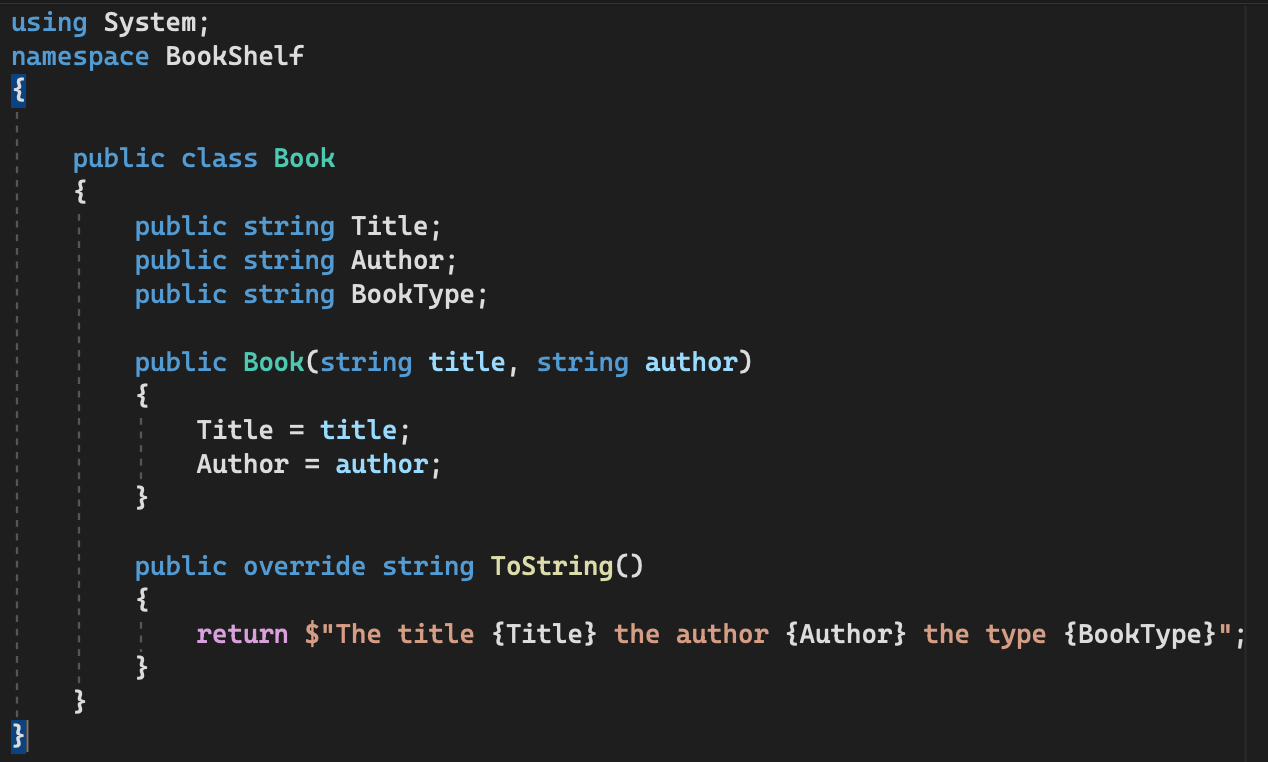
first point: don't make public fields
make public properties
and for something like this
Kind of
I'm not using properties at this point yet
time to start
and
BookType
is redundant when the class is already named Book
, make it Type
Ok! But in my assignment they haven't mentioned it
Look
ah, okay
if you're following an assignment, then yeah, stick to those constraints
just be aware, that's shitty code
don't do that in any real scenario
(public fields)
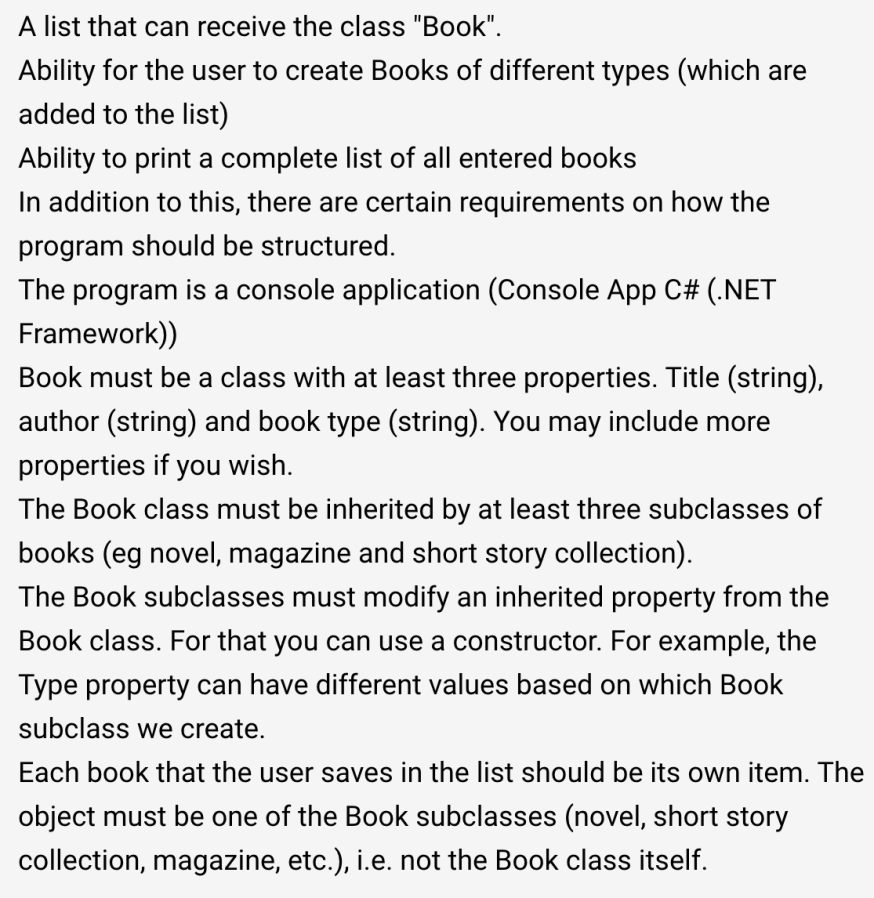
okay, so
it states RIGHT there that the
Book
class should have "properties"Really, why my friend?
because it allows classes better control over their state
the most specific example....
if your book class exposes a
Title
field directly
and then wants to change the behavior of that field, in the future
swapping that field out for a property is a breaking change
if it's already a property, adding logic to a getter that already exists would not (necessarily) be a breaking change
beyond that, it's just general practiceMany say the same, that those should be private access modifiers and not public, is it because of the accessibility level where whoever can come and change the values from the outside of the class and when they're private, basically no one is able to access & change them, right?
to the point of interoperability with other systems
like serializers
virtually all serializers will serialize your class's properties
and ignore fields
that's just the convention of what data models should be
What, I didn't know that 🙆🏻♂️
If we're JUST talking about the difference between properties and fields, then no, fields can be readonly just as much as properties can
it's a semantic convention, one which costs you nothing to implement
so
let's start with the
Book
class
per the requirements, it needs to have 3 properties, and a constructor that sets them
write that out for me, pleaseWow, I'm just amazed on the level of knowledge that you're capable of currently. That's amazing bro.
What do you mean by breaking change, I didn't quite understand this btw?
a breaking change is with regard to version control and publishing code
Bingo
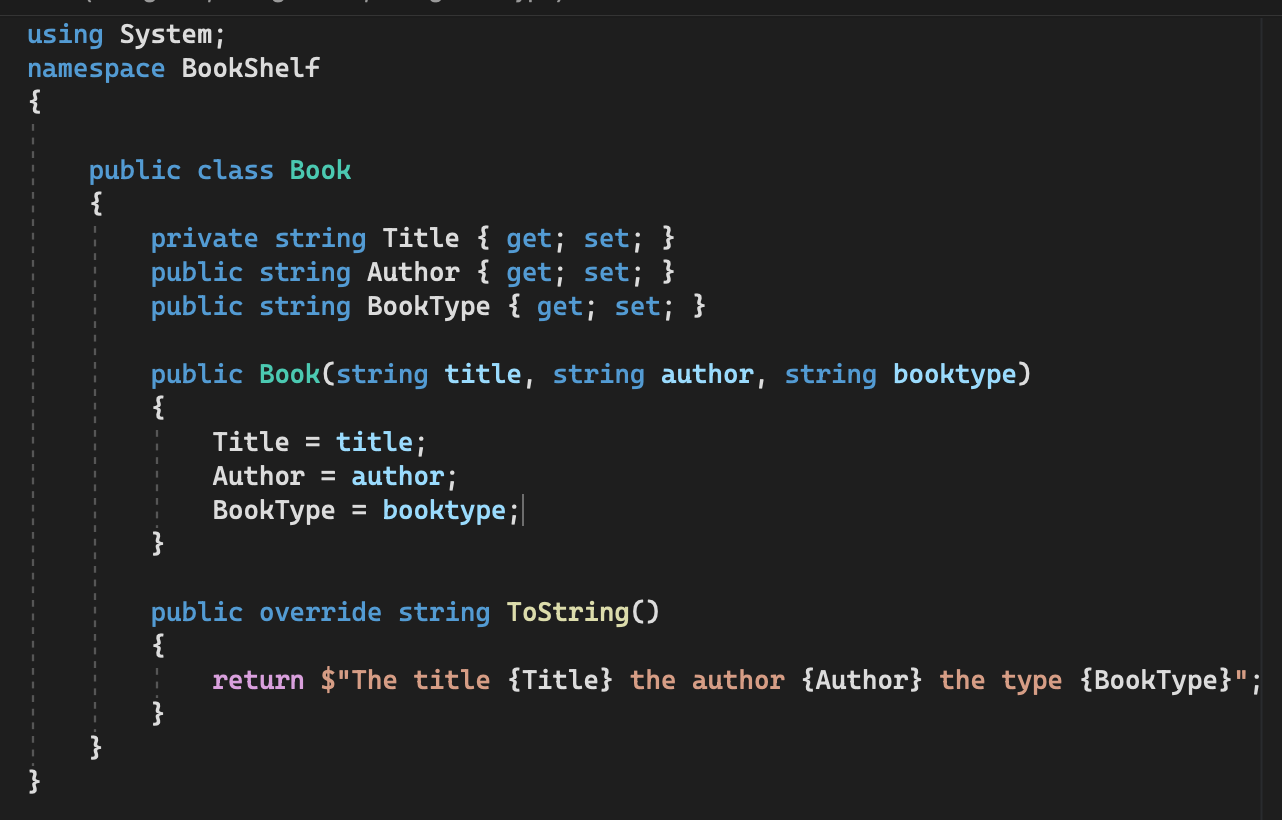
bingo indeed
well, mostly
A)
Title
should be public
B) all of those should not be set
able
C) Type
still makes more sense than BookType
I should read about it, because I'm not sure what version control means in a coding sense.
indeed you should
C# Versioning - C# Guide
Understand how versioning works in C# and .NET
Aha! Ok! Thanks a lot for teaching me these concpts.
Why title should be a public property and not private same thing with the Author?
Sorry for asking too many questions, I just want to learn using this thread 😃
per the assignment requirements
Ability to print a complete list of all entered bookspresumably, this should mean printing all the information ABOUT each book ergo, it needs to be accessible now
Yes, this is what they want me to implement pretty much.
technically, you could probably get away with keeping them as
private
fields, given that you're overriding ToString()
but that's a poor way to expose information in code
it limits your consumer
per the "Open-Closed Principle" of Object-Oriented Programming:
Entites should be open for extension, but closed for modificationand this really just plays into that make yourself as freely-usable as possible, while maintaining consistency so instead of forcing the consumers of the
Book
class to only be able to "print" the book info one way, expose the info as granularly as possible, to allow consumers to piece it together however they like
but restrict the ways they can create and modify book info
I.E. your requirements don't specify ANY need to make books mutable (I.E. changeable)
so, don't give them any
and that plays into the book type propertiy
don't allow consumers to set that to anything
if your data model says "only three types of books exist" set things up so that only those three values can ever be assigned
that's where the subclassing will play inOk! I have reviewed all of your content now.
Although this is a bit advanced topic for my level, but still very worth to learn all these.
Man I wish if I could've call you, otherwise we could continue to write here.
Now, I see why you didn't use any
set
in the properties, because basically we are the one who set the book type for the consumer.
Are you available?I say not to use
set
ters because you don't need to
the requirements of your problem don't specify that books need to be editable after they've been created
that's what a set
ter doesI noticed you're replaying to many threads, man you're awesome at helping other people and solving their code issues 😀
Aha! Ok! You're totally right.
This how it looks currently
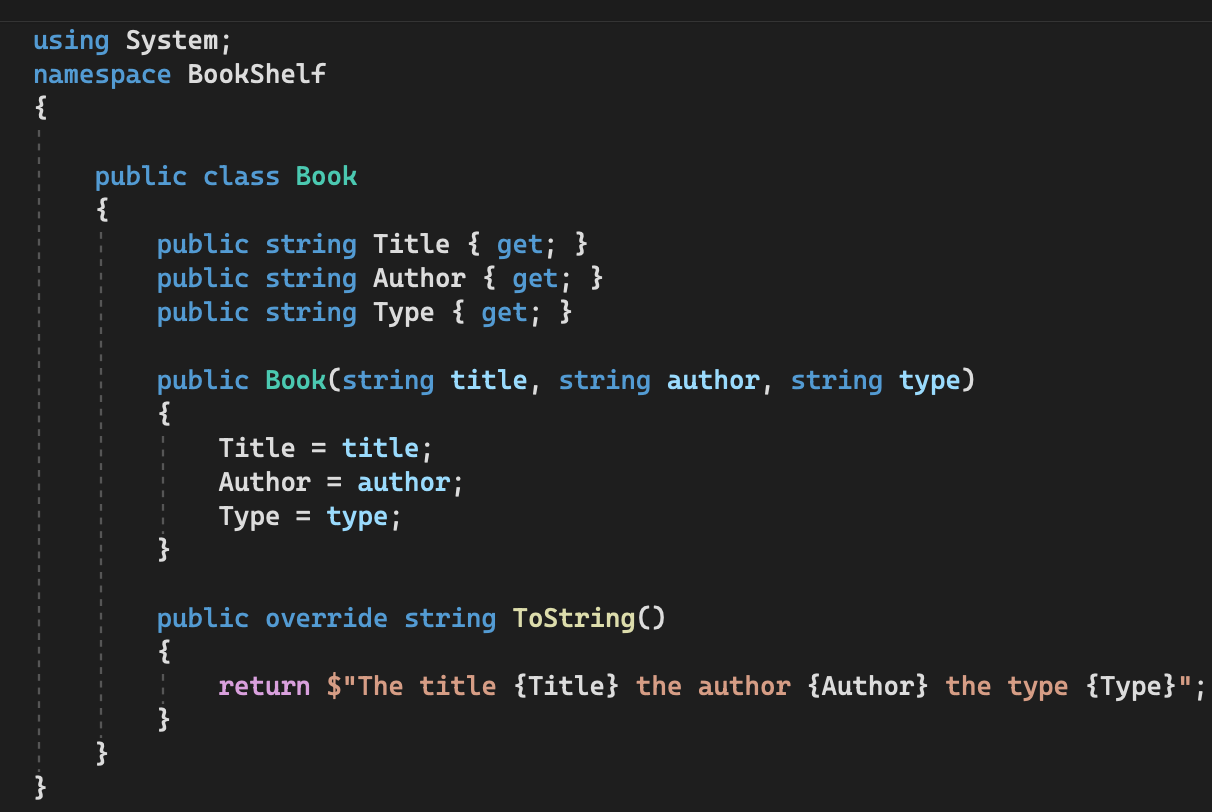
sweet
now
subclassing
requirements state
A) you need at least 3
B) you should leverage the constructor to set the type
Now, I have issues with the subclasses where they ask for passing a full arguments based on the params my friend 🤷🏻♂️
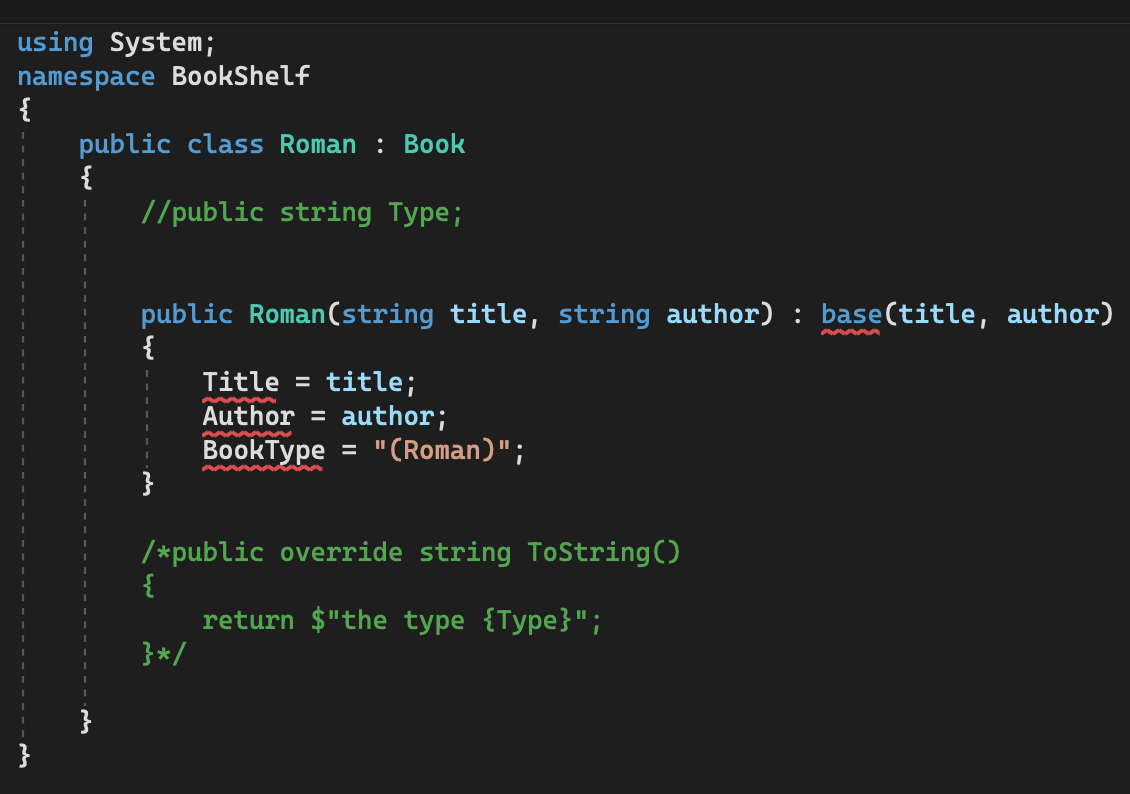
k
what are those errors?
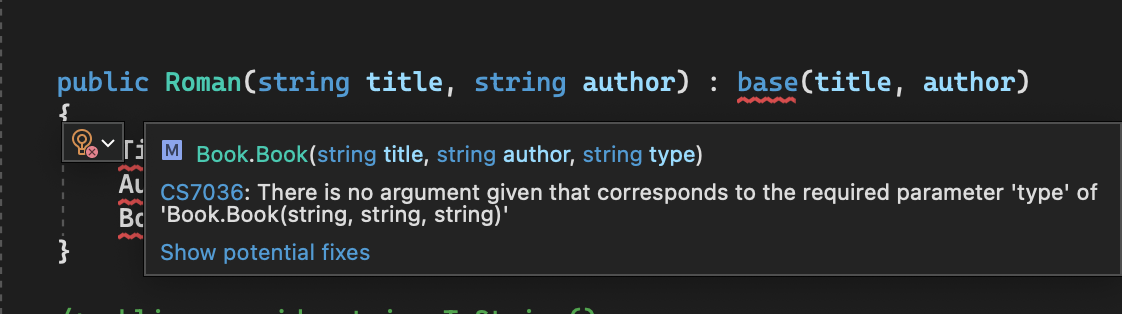
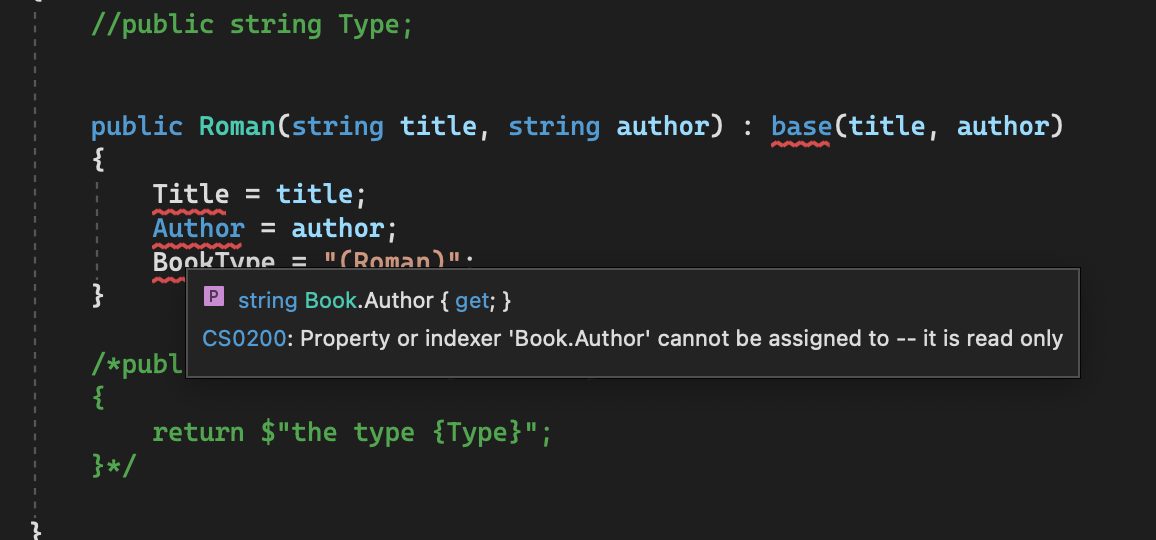
I don't need screenshots, just read them
or post the text
so, what does that error tell you
I have created these three subclasses if you look at my gist 😉 https://gist.github.com/Programmer-Faraj/9a2f90285e21996c4cf7577505ce6853
would if I could
well, okay, that one seems to work
the earlier one didn't
regardless, that's not really the point
I don't care what your other subclasses are
I'm asking about this one
what does that error tell you?
Ok! Well! The first error says you need to pass an argument for the
string type
parameterright
cause that's how you defined the
Book
constructor that you're callingI'm passing three params in the base class, so I need to pass three arguments in the sub-classes too
it requires a
type
parameter
so give it
so I need to pass three arguments in the sub-classes toonope not necessarily the only thing you need to pass 3 arguments to is the thing that you've defined to take 3 arguments the
Book
constructorsee these are things very tricky to learn for beginners and only people like you knows the solution
Aha
yes and no
you just need to learn how to think about your problems
You mean only when creating object of a class then we need to pass three arguments?
once you learn how to properly model your problems, the solutions are self-explanatory
I mean what I said
the only thing you need to pass 3 arguments to is the thing that you've defined to take 3 arguments the Book constructor
That's a good one
My friend, I work at a school and I need to go out, I will catch up withy you when I get my lunch hour 😃 thank you sooo much for your unlimited help in teaching me 🤗
sure thing
Alright. But what would be the thing that takes 3 arguments my friend?
I'm new to inheritance and still at the surface and haven't got deeper yet.
the Book constructor, as I've said
Ok!
So is that mean I shouldn't pass three arguments to the sub-classes or what?
Wait a minute, I've noticed something. base class (Book)'s ctor is already handling title and author, so I don't need to do it again over here in the sub-classes 💁🏻♂️
I mean logically, the sub-classes are for only the public string Type; why would I pass the other properties for inheritance, right?
uhhhhhh
no
the opposite of what you said
Sorry, I said wrongly yesterday until I learned from a member in the server that I would need to pass the same parameters except for the
string type
Klarth#1705
Because in your initial example, you didn't supply the third parameter. You used a hardcoded string, so there's no need for the caller to pass a parameter.
Quoted by
<@!973964186956726313> from #help-0 (click here)
React with ❌ to remove this embed.
so, where we at with this?
Great to see again
I just modified my sub-classes
Yesterday, I was getting two different errors, one for not passing a param for the
string type
in the base ctor and the other one for being readonly, then I learned those errors from Klarthbeautiful
Yep
Although, this seems a bit weird to me. What should I do inside the curly brackets? I mean we usually initialize parameters inside these brackets, but in my case, I'm passing "(Roman)" in the ctor directly.
What do you think?
Are you available?
Are you sleeping or something? 😁
working
What should I do inside the curly brackets?nothing you said it yourself the one thing the subclass needs to do in the constructor, you're already doing
Nice to see you again
It's seems to me that you're from US that's why you show up when it's evening over here in Sweden. Different timezones.
Aha! Are you working as a software developer or sort of?
Ok! Awesome. I guess this is the implementation that is required for the assignment so it shouldn't go beyond it's limitation.
.
Man, previously you mentioned very interesting tips here and I really really would like to learn how to do that. I wish if I could call you and get some more tips, like even a half hour whenever you're available!
ask question, receive answer
Aha! So this is all about learning how to model problems?
that's my take, yeah
that's a big part of software development, and C# in general, I think
Ok! I understand that.
I think asking a specific question for a specific problem is very worth it instead of looking at the screen for hours/days, since a concept in c# can be used/implemented in many different ways depending on the problem.
Are you working as a software developer?
yes
Wow, awesome.
What are the lest requirements to be a junior developer?
uhh
I dunno
depends who you ask
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.