❔ Simple blog page (Homework)
Hi!
(Forgot to mention, this is console app)
got into a block in my head, trying a try catch phase to prevent a crash for wrong input of a blog title, now I am stuck..
on case1
Trying to:
Tell the user to write a blog title
Get the user input, store it and send it of to list above or an vector but blank in my head for the moment..
122 Replies
isnt it supposed to be
userBlogInput = Console.ReadLine()
instead of Console.ReadLine(userBlogInput)
?
Thank you, tried a little with this.
Getting an issue that it goes onto catches, not saving the title into index 0
that could be because the list myBlog doesnt actually have a value at index 0

change
myBlog[i] = Console.ReadLine();
to myBlog.Add(Console.ReadLine());
and also you probably want for (int i = 0; i < 1; i++)
instead of for (int i = 0; i < 1;)
and also isRunning is always true
so that means you will only be able to enter the same case you initially enteredI moved it up, then would you recommend using a for loop for this if I want to add more posts without overwriting the old one?
Changed it to this instead of a for loop
This allowed me to store the values on index positions + changed the list to this:
looks like it should work
although just to nitpick, i would probably change blogTitle to something like blogPost
Then I saw that you mentioned .add, so using:
myBlogVectorList.Add(blogTitle);
yeah since the list initially doesnt have any values youll have to do that
Quick question regarding the .add, do I need it right after the user's input or only at the end once?
Will it then add both inputs if I use it at the end?
Ah. Now I know why this felt so similar.
Is this an NTI assignment?
oh i forgot this was homework
shouldve structured it as learning
and you are supposed to implement a binary search later on?
Yes!
Yeah, we had a fellow student of yours ask for help with this very assignment a week or two ago.
oh please dont make me remember that
Well, not sure. I find it very helpful. You just told me to change the title due to the name I chose. Made me think of why and keep in mind to name them more appealing
Would love to see that post, if you'd know where to find it
probably archived now
ah yes
but honestly, don't spend too much time digging in them
yeah id recommend trying to implement it yourself then if it doesnt work, ask
your program has a few issues as is already 🙂
this strikes me as the wrong order of things
you parse the input, once, and if it was valid, you enter a loop?
usually you have a loop surrounding your read, so that a failed read is "retried" so to speak
but bravo for
int.TryParse(Console.ReadLine(), out userInput)
, thats crispy cleanMy bad, It's updated. Noticed it while testing it out
(Prob should have cut this down)..
Have you covered methods yet?
Only a little, I played around with them yesterday after watching:
C# Tutorial - Full Course for Beginners by: freeCodeCamp.org
its fine if not, but this code would be so much nicer if each of your cases were split up a bit, as this will get nasty when you implement case 2 and 3 otherwise
its worth learning at least the basics for it
takes a lot of code out of main
Alright, give me a few. Will try it out
Alright, stuck
Okay so
lets look only on your save method first
this method takes a string in, that is... never interacted with at all, and then returned
you do however build a
string[] blogPost
that has values added to it, and then left to die alone
So my questions are as follows:
1. what is the purpose of string saveBlog
?
2. is saveBlog
the correct return value here?1. To use the return statement, I think I've misunderstood that.
Changed it to this:
Since we're trying to return blogPost's values/indexes
2. No, blogPost is the return we want
much better!
Not going to lie and take it as a W, this was a quick solution from Visual studio which helped me realize that I misunderstood the explanation of how to use a return statement!
Good progress though!
Alright, the statement should be alright.
Changed into this, this should allow us to enter the method and prompt from there to then return once finished.
Then, how would I use the add function to add it into the vectorList?
show me your entire
case 1:
nowsaveBlogPost
returns something
we need to use that returned valueIt returns blogPost, right?
it returns a
string[]
thats all you know at that point
but you are not using the return value
you are discarding it, throwing it awayAlright
So, then to grab that value. I'd simply have to:
yup
Not sure, it seems that it takes me into the method once more


Right, just remove the upper one and keep it as .add(saveBlogPost());
This should add the values, right?
yup
If i'd like to take the value on index 0 out just to check if it's stored, how would I do that?
myBlogVectorList[0]
would give you an array
you need to then index that array tooAlright, why is this needed?
its not, strictly speaking
its to make it easier to read
if you just want the blog title of the first post,
myBlogVectorList[0][0]
works
but its not very nice to readand then if you want the text as well youd have
myBlogVectorList[0][1]
Running that gives the last text only,
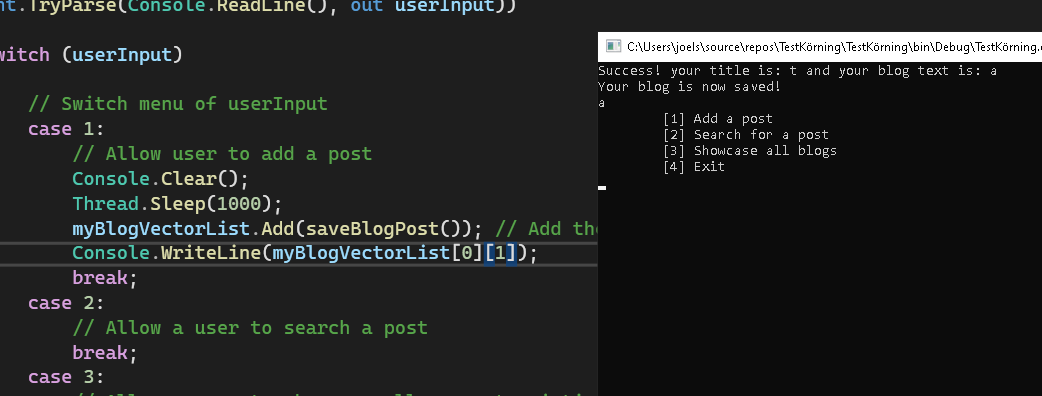
ofc
thats literally what haze said
the first index is accessing the
List
, the second index is for accessing what is stored there (the string[]
)i suppose i couldve worded it better
Oh!
if you wanted only the text youd have
myBlogVectorList[0][1]
Keeping the string inside, it does make it easier to 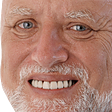
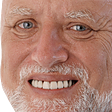

yup
Case 3
i dont see the need for an extra variable here, but it doesnt really matter
also another really nitpicky thing would be to possible rename item to blog, since you are looping through blogs
but that really doesnt matter
Thanks a lot so far, it's extremely helpful!
That's right. Luckily, I completed the project, submitted it 😆
@Jorikuit seems like you're taking the same course as I do or apparently different course, but same assignment!
Oh no way, hope you getting good grades!
I got some extra assignments to do for it for an A but don't think time exists for it. Got case 2 legt
Well! My grades looks good so far. I got some assignments too that I'm working on currently and they're getting harder and harder.
Yeah, so far only had A's up till last assignment where I started to struggle. They're getting extreme 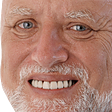
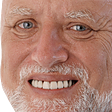
Ok! Same thing happened with me too. It's like playing a game and the level gets harder.
Are you from Brazilian?
Yeah, short explained. It just takes up your skill level
I am Swedish, prob shouldn't keep this help section into a conversation
Will return with Case 2 in a little

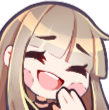
Aha! Ok! I'll dm you then.
Alright, stuck again
Using:

Well yes
What does Console.ReadLine() return?
The user input as a string, sorry. Went to gym and took some food.
and what are you trying to store it as?
I think I am trying to store it as the same as the vectorList..
so you are trying to store a string as a string array?
Trying to compare it, is what I am after at least
this line
string[] searchWord = Console.ReadLine();
what is it trying to store in searchWord?a string list
but what does Console.ReadLine() return?
a simple string
there you go then
you cannot store a string as a string array
Then what options do I have here?
well why are you trying to store it as a string array?
nowhere in your code uses indexes of the variable
Here's where my head collapsed
what does
string[]
mean to you?a list of strings, a place where you can store multiple strings
ok, but Console.ReadLine() returns only one string
so how can you declare a variable as a list (it's actually an array) when you are only passing in a singular string variable
This is where my head went into spinning, not sure what I am 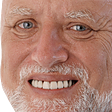
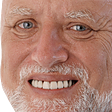
I'm trying to get you to understand what you are actually trying to do
So first off,
string[]
is a string array, not a list, if you do not know the difference I highly recommend you do some research
Secondly, reread the error message you receive when trying to run the code and really think about what it means
Thirdly, look through your current code and see if you really are using whatever data type you think you areThis
This is a list, right?
A list of strings with no set sequential
And an array, set sequentials
Or am I misunderstanding it completely
Or am I misunderstanding it completely
Yes, you are correct
Then if you only use string[], it's an array
And now, it's looking a little bit better
One question about this, though. While using the double [i]'s, why are what happens here?
It has to do with my list, right?
You are firstly indexing your list, which returns an array, then you are indexing that array with the second [i]
Although I don't know why you are using [i][i] instead of [i][0] since aren't you just trying to search the titles of the blogs?
So, the second i basically takes the value out of the array?
Right, since using 0 is the first Index. Thank you!
That just solved my crashes using 0 and 1 instead of i i with i++
So is it all sorted now?
Yeah, it's all sorted for now. Thank you!
great, and i hope you learned something
Alright, it's not done
Alright, I can't figure this out..
So, this is what I have so far.
Trying to give alternative options based on the first character of user input, if no post exists with the input of the user
Alright, back for some feedback and checks
So far, I updated the code to this
Questions: What can go wrong here, and is this acceptable as a linear search method?
Then, what I think this does to check If I am correct in most parts:
It takes the users input, searches loops through the vector list to find the search word. Ignores the ordinal case rule skipping need of toLower/Upper. Checks if both [0, 1] index are true and searches for a word match.
And if it doesn't exist, performs code below and informs that no character in the user's search matches a title nor text
comments like these are just useless noise. Unless your teachers force you to write them, remove them.
and yes, looks okay for a linear search
Update:
Added dates
And printing out as blogPost[2]
Is it just bad mobile formatting or am I seeing comments inside an if statement?
Uhm, caugh, caugh. Just bad mobile
let's see. How would I do to print out all existing blogs. Give them a number by taking 3 indexes for each as 1 then the next 3 indexes for number 2. So Create an ID for the stories?
This is to be able to remove a post by index 0 (title) text (index 1) and it's date (index 2) for each an every post. A simple remove function.
Then this will come in handy while allowing to edit that specific title or text aswell. Then just update the edit date by .new
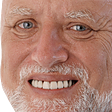
Posting the whole code since a lot has changed since last time.
Currently:
And I am done, finished up this task. I am not going for that A. Screw it, too complex xD
Thank you both for your help, it's been extremely useful and took hours extra of my already 4 days into this project.
Leaving the code public here, in case any one wants to look through it or needs it in the future.
How much longer do you have until it's due?
I'm guessing it was due 23:59 and they posted it at 23:48 😛
If they had more time I'd fully recommend at least trying to complete the other parts, since there's no real harm in doing so
Sorry, didn't see this. It's turned in, had till yesterday 23.59
ah okay
Yeah, I took 4 out of 6 extra parts. Sadly, had not time for the other two so I can't get an A, but at least a C/B
even if it doesnt mean anything, id still recommend you at least try the extra tasks
Yeah, starting a new project to finish up things and get some more repitation for the grounds
as long as you dont get into the habit of not doing stuff because its difficult you should be fine for the most part
Got the finals in 2 days only
So, the things left was:
- Binear search
- A sorting system
So, those are the things I'll be working on in the new project
thats good
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.